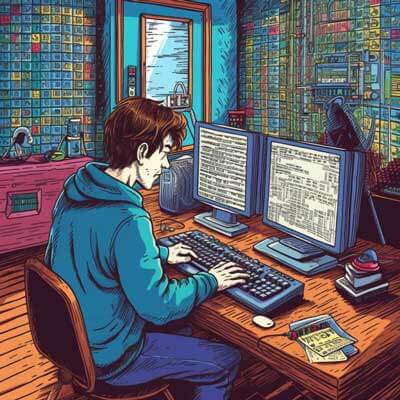
Types of Data Structures
Data structures are fundamental components of any programming language. They are used to organize and store data in a way that allows for efficient retrieval and manipulation. Python provides several built-in data structures that can be used to solve a wide range of problems. Some of the commonly used data structures in Python include lists, tuples, dictionaries, sets, arrays, stacks, queues, linked lists, hash tables, and binary trees.
Related Article: How To Convert a Dictionary To JSON In Python
Implementing Lists
Lists in Python are versatile data structures that can store an ordered collection of items. They are mutable, meaning that their elements can be modified after creation. Lists are implemented internally as dynamic arrays, which allows for efficient random access and insertion/deletion at the end of the list. Here is an example of creating a list in Python:
my_list = [1, 2, 3, 4, 5]
You can access individual elements of a list using indexing. For example, to access the first element of the list, you can use my_list[0]
. Lists also support slicing, which allows you to extract a portion of the list. For instance, my_list[1:3]
will return a new list containing the second and third elements of my_list
.
Implementing Tuples
Tuples are similar to lists but are immutable, meaning that their elements cannot be modified after creation. Tuples are typically used to store related pieces of information that should not be changed. Here is an example of creating a tuple in Python:
my_tuple = (1, 2, 3, 4, 5)
Tuples can be accessed using indexing, just like lists. For example, my_tuple[0]
will return the first element of the tuple. Tuples also support slicing, allowing you to extract a portion of the tuple. However, since tuples are immutable, you cannot modify their elements or append new elements to them.
Implementing Dictionaries
Dictionaries in Python are unordered collections of key-value pairs. They provide a way to store and retrieve data using a unique key. Dictionaries are implemented internally as hash tables, which allows for efficient insertion, deletion, and retrieval of elements. Here is an example of creating a dictionary in Python:
my_dict = {"name": "John", "age": 30, "city": "New York"}
You can access the value associated with a specific key by using indexing. For example, my_dict["name"]
will return the value “John”. Dictionaries also support adding new key-value pairs, modifying existing values, and removing key-value pairs.
Related Article: How to Sort a Dictionary by Key in Python
Implementing Sets
Sets in Python are unordered collections of unique elements. They are implemented internally as hash tables, which allows for efficient membership testing and set operations such as union, intersection, and difference. Here is an example of creating a set in Python:
my_set = {1, 2, 3, 4, 5}
You can check if an element is present in a set using the in
operator. For example, 2 in my_set
will return True
. Sets also support set operations such as union, intersection, and difference. For example, if you have two sets set1
and set2
, you can perform the union of the two sets using set1.union(set2)
.
Implementing Arrays
Arrays in Python are used to store a fixed-size sequence of elements of the same type. They are implemented internally as contiguous blocks of memory, which allows for efficient random access and element manipulation. Arrays in Python are provided by the array
module. Here is an example of creating an array in Python:
import array my_array = array.array('i', [1, 2, 3, 4, 5])
In this example, i
represents the type code for signed integers. You can access individual elements of an array using indexing, just like lists. Arrays also support slicing and various methods for manipulating their elements.
Implementing Stacks
A stack is a data structure that follows the Last-In-First-Out (LIFO) principle. It can be implemented using a list in Python. Here is an example of implementing a stack in Python:
stack = [] # Push operation stack.append(1) stack.append(2) stack.append(3) # Pop operation top_element = stack.pop()
In this example, append
is used to push an element onto the stack, and pop
is used to remove and return the top element of the stack. You can also use the len
function to check the size of the stack.
Related Article: How to Remove a Key from a Python Dictionary
Implementing Queues
A queue is a data structure that follows the First-In-First-Out (FIFO) principle. It can be implemented using a list in Python. However, since removing elements from the beginning of a list is inefficient, it is recommended to use the collections.deque
class from the collections
module. Here is an example of implementing a queue using collections.deque
:
from collections import deque queue = deque() # Enqueue operation queue.append(1) queue.append(2) queue.append(3) # Dequeue operation front_element = queue.popleft()
In this example, append
is used to enqueue an element, and popleft
is used to dequeue and return the front element of the queue. You can also use the len
function to check the size of the queue.
Implementing Linked Lists
A linked list is a data structure that consists of a sequence of nodes, where each node contains a value and a reference to the next node. Linked lists can be implemented using classes in Python. Here is an example of implementing a singly linked list in Python:
class Node: def __init__(self, value): self.value = value self.next = None class LinkedList: def __init__(self): self.head = None def add_node(self, value): new_node = Node(value) if self.head is None: self.head = new_node else: current_node = self.head while current_node.next is not None: current_node = current_node.next current_node.next = new_node def print_list(self): current_node = self.head while current_node is not None: print(current_node.value) current_node = current_node.next # Usage example linked_list = LinkedList() linked_list.add_node(1) linked_list.add_node(2) linked_list.add_node(3) linked_list.print_list()
In this example, the Node
class represents a node in the linked list, and the LinkedList
class manages the list operations such as adding nodes and printing the list.
Implementing Hash Tables
Hash tables, also known as hash maps, are data structures that allow for efficient insertion, deletion, and retrieval of key-value pairs. In Python, hash tables can be implemented using the dict
class. Here is an example of using a hash table in Python:
my_dict = {"name": "John", "age": 30, "city": "New York"} # Accessing values print(my_dict["name"]) # Output: John # Adding a new key-value pair my_dict["occupation"] = "Engineer" # Removing a key-value pair del my_dict["age"] # Iterating over key-value pairs for key, value in my_dict.items(): print(key, value)
In this example, the dict
class is used to create a hash table. You can access values using keys, add new key-value pairs, remove existing key-value pairs, and iterate over the key-value pairs using the items
method.
Related Article: How to Remove an Element from a List by Index in Python
Additional Resources
– Python Documentation – Lists
– Python Documentation – Tuples