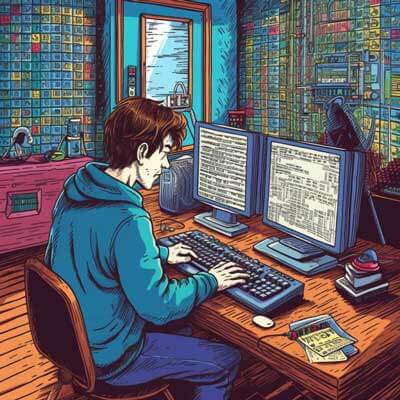
Reordering columns in a Pandas DataFrame can be done using various techniques provided by the Pandas library. In this answer, we will explore two common methods to achieve this.
Method 1: Using the reindex() function
The reindex() function in Pandas can be used to rearrange the columns of a DataFrame based on a specified order. Here are the steps to follow:
1. Define the desired order of the columns in a list.
2. Use the reindex() function to reorder the columns based on the defined list.
Here’s an example:
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Alice', 'Bob'], 'Age': [25, 30, 35], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Define the desired order of columns column_order = ['City', 'Name', 'Age'] # Reorder the columns using reindex() df = df.reindex(columns=column_order) # Display the updated DataFrame print(df)
Output:
City Name Age 0 New York John 25 1 London Alice 30 2 Paris Bob 35
In this example, we created a DataFrame with three columns: “Name”, “Age”, and “City”. We then defined the desired order of columns as [‘City’, ‘Name’, ‘Age’]. Finally, we used the reindex() function to reorder the columns based on the defined order.
Related Article: How To Convert a Python Dict To a Dataframe
Method 2: Using column indexing
Another way to reorder columns in a Pandas DataFrame is by directly indexing the columns in the desired order. Here are the steps to follow:
1. Define the desired order of the columns in a list.
2. Use the list to index the DataFrame columns.
Here’s an example:
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Alice', 'Bob'], 'Age': [25, 30, 35], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Define the desired order of columns column_order = ['City', 'Name', 'Age'] # Reorder the columns using column indexing df = df[column_order] # Display the updated DataFrame print(df)
Output:
City Name Age 0 New York John 25 1 London Alice 30 2 Paris Bob 35
In this example, we followed the same steps as in Method 1 but used column indexing instead of the reindex() function to reorder the columns.
Why would someone want to reorder columns in a Pandas DataFrame?
There can be several reasons why someone might want to reorder columns in a Pandas DataFrame. Here are a few potential reasons:
1. Improving readability: By reordering columns, you can arrange them in a more logical or intuitive sequence, making it easier for others to read and understand the data.
2. Aligning with external requirements: Sometimes, external systems or processes may expect the input data to be in a specific column order. Reordering the columns can help align the DataFrame with these requirements.
3. Performing calculations or analysis: Certain calculations or analysis tasks may require the data to be in a specific column order. Reordering the columns can simplify these tasks by ensuring the necessary data is readily available.
Suggestions and alternative ideas
While the methods described above are effective for reordering columns in a Pandas DataFrame, there are a few alternative approaches worth considering:
1. Using the loc[] accessor: The loc[] accessor in Pandas allows you to access and modify columns based on their labels. By specifying the desired order of column labels, you can achieve column reordering using loc[]. Here’s an example:
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Alice', 'Bob'], 'Age': [25, 30, 35], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Define the desired order of column labels column_order = ['City', 'Name', 'Age'] # Reorder the columns using loc[] df = df.loc[:, column_order] # Display the updated DataFrame print(df)
2. Creating a new DataFrame: Instead of modifying the existing DataFrame, you can create a new DataFrame with the desired column ordering. This approach ensures the original DataFrame remains unmodified, which can be useful in certain scenarios. Here’s an example:
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Alice', 'Bob'], 'Age': [25, 30, 35], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Define the desired order of columns column_order = ['City', 'Name', 'Age'] # Create a new DataFrame with the desired column ordering new_df = df[column_order] # Display the new DataFrame print(new_df)
Both the loc[] accessor and creating a new DataFrame offer alternative ways to achieve column reordering in Pandas.
Related Article: How To Filter Dataframe Rows Based On Column Values
Best practices
When reordering columns in a Pandas DataFrame, it is important to keep a few best practices in mind:
1. Document your column order: If you have a specific column order requirement, consider documenting it in the code or a separate documentation file. This can help others understand the intended column order and avoid confusion.
2. Avoid excessive column reordering: While it is easy to reorder columns in Pandas, excessive reordering can make the code harder to read and maintain. It is generally recommended to keep the column order as simple and intuitive as possible.
3. Consider using column labels: If your DataFrame has column labels, consider using them instead of numerical indices when reordering columns. This can make the code more readable and resilient to changes in column positions.