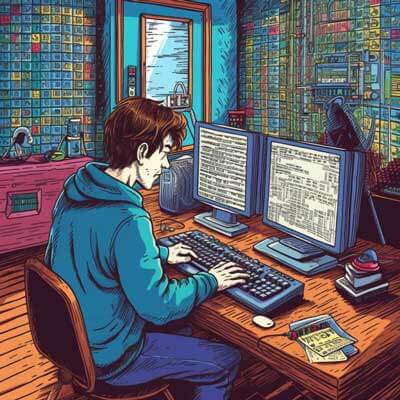
- Django Translation
- Setting up Django for Multiple Languages
- The Gettext Framework and Translations in Django
- Middleware and Context Processors for Language Preferences in Django
- Implementing Dynamic URL Routing Based on Languages in Django
- Handling Right-to-Left (RTL) Languages and Layout Considerations in Django
- Translation Management in Django
- Best Practices for Localization in Django
- Creating a Multilingual Website in Django
- Additional Resources
Django Translation
In today’s globalized world, it is essential for web applications to support multiple languages to cater to a diverse user base. Django, a popular Python web framework, provides useful tools and features for internationalization and localization. In this section, we will explore the various aspects of multilingual support in Django apps.
Related Article: Django 4 Best Practices: Leveraging Asynchronous Handlers for Class-Based Views
Setting up Django for Multiple Languages
To enable multilingual support in a Django app, we need to configure the necessary settings and provide translations for different languages.
First, we need to set the LANGUAGE_CODE
setting in the Django settings file (settings.py
). This setting specifies the default language for the app. For example, to set the default language to English, we can use the following code snippet:
LANGUAGE_CODE = 'en-us'
Next, we need to define the languages that our app supports. This can be done by setting the LANGUAGES
setting in the same settings file. The LANGUAGES
setting is a list of tuples, where each tuple represents a language and its corresponding language code. For example, to support English and Spanish, we can use the following code snippet:
LANGUAGES = [ ('en', 'English'), ('es', 'Spanish'), ]
Django uses the gettext
framework for translations, which we will explore in the next section.
The Gettext Framework and Translations in Django
The gettext
framework is a widely used tool for handling translations in software applications. Django leverages this framework to provide translation capabilities for its apps.
To start using the gettext
framework in Django, we need to import the gettext
function from the django.utils.translation
module. This function is used to mark the strings that need to be translated. Here’s an example:
from django.utils.translation import gettext as _ def hello_world(request): message = _('Hello, World!') return HttpResponse(message)
In the above code snippet, the _('Hello, World!')
statement marks the string “Hello, World!” for translation. The gettext
function extracts this string and prepares it for translation.
To generate translation files for different languages, we can use Django’s makemessages
management command. This command scans the codebase for strings marked for translation and creates or updates the corresponding translation files. For example, to generate translation files for English and Spanish, we can use the following command:
$ python manage.py makemessages -l en $ python manage.py makemessages -l es
This will create two translation files, django.po
, for each language in the locale
directory of the app. The translation files contain the original strings and empty placeholders for the translated strings.
Once the translation files are generated, we can start translating the strings. We can use any text editor or translation management tool to edit the translation files. Here’s an example of a translated string in the django.po
file:
msgid "Hello, World!" msgstr "¡Hola, Mundo!"
The msgid
field represents the original string, and the msgstr
field contains the translated string.
After translating the strings, we need to compile the translation files into binary format using the compilemessages
management command. This command creates .mo
files, which Django uses for runtime translations. Here’s the command to compile the translation files:
$ python manage.py compilemessages
With the translation files compiled, Django will automatically load the appropriate translations based on the user’s language preference, which we will explore in the next section.
Middleware and Context Processors for Language Preferences in Django
In a multilingual Django app, it is important to allow users to choose their preferred language. Django provides middleware and context processors to handle language preferences.
Middleware is a component in Django that sits between the web server and the view, allowing us to process requests and responses. The LocaleMiddleware
is a built-in middleware that enables language preference handling in Django.
To use the LocaleMiddleware
, we need to add it to the MIDDLEWARE
setting in the Django settings file. Here’s an example:
MIDDLEWARE = [ ... 'django.middleware.locale.LocaleMiddleware', ... ]
Once the LocaleMiddleware
is added, Django will automatically set the language based on the user’s preferences. The language is determined by checking the Accept-Language
header in the HTTP request.
In addition to middleware, Django also provides context processors that allow us to add variables to the context of every template rendering. The django.core.context_processors.i18n
context processor adds the LANGUAGES
and LANGUAGE_CODE
variables to the context, which can be used in templates to display language-related information.
To use the i18n
context processor, we need to add it to the TEMPLATES
setting in the Django settings file. Here’s an example:
TEMPLATES = [ { ... 'OPTIONS': { 'context_processors': [ ... 'django.template.context_processors.i18n', ... ], }, }, ]
With the i18n
context processor added, we can access the LANGUAGES
and LANGUAGE_CODE
variables in our templates. For example, to display the current language in a template, we can use the following code snippet:
{% for lang in LANGUAGES %} {% if lang.0 == LANGUAGE_CODE %} <strong>{{ lang.1 }}</strong> {% else %} {{ lang.1 }} {% endif %} {% endfor %}
This will display the languages supported by the app, with the current language highlighted.
With the language preferences set up, we can now move on to implementing dynamic URL routing based on languages.
Related Article: How to Replace Strings in Python using re.sub
Implementing Dynamic URL Routing Based on Languages in Django
In a multilingual Django app, it is common to have different URLs for different languages. Django allows us to implement dynamic URL routing based on languages using language prefixes.
To enable language-based URL routing, we need to modify the urlpatterns
list in the Django urls.py file. Here’s an example:
from django.urls import path from django.conf.urls.i18n import i18n_patterns urlpatterns = [ ... ] urlpatterns += i18n_patterns( ... path('about/', views.about, name='about'), ... )
In the above code snippet, the i18n_patterns
function wraps the URL patterns that should be language-specific. This function takes care of adding the language prefix to the URLs based on the user’s language preference.
For example, if the user’s preferred language is set to Spanish, the URL /es/about/
will be used for the about
view. If the user’s preferred language is English, the URL /en/about/
will be used.
It’s important to note that the language prefix is automatically added to all URLs within the i18n_patterns
function. This includes any URLs defined in included URL configuration files.
With dynamic URL routing in place, we can now move on to handling right-to-left (RTL) languages and other layout considerations.
Handling Right-to-Left (RTL) Languages and Layout Considerations in Django
In a multilingual Django app, it is important to consider the layout and design aspects when dealing with right-to-left (RTL) languages, such as Arabic or Hebrew.
Django provides support for RTL languages through the django.utils.translation.get_language_bidi
function. This function returns True if the current language is a RTL language, and False otherwise.
To handle RTL languages in templates, we can use conditional statements to apply specific styles or layout changes. Here’s an example:
{% if LANGUAGE_BIDI %} {% else %} {% endif %}
In the above code snippet, the LANGUAGE_BIDI
variable is used to check if the current language is RTL. If it is, the appropriate CSS file (rtl.css
) is loaded, otherwise the default CSS file (ltr.css
) is loaded.
Similarly, we can apply RTL-specific styles to elements using conditional statements. Here’s an example:
{% if LANGUAGE_BIDI %} <div class="rtl-specific-style">...</div> {% else %} <div class="ltr-specific-style">...</div> {% endif %}
In the above code snippet, the LANGUAGE_BIDI
variable is used to conditionally apply different styles to the div
element based on the language direction.
Handling RTL languages also involves considering the order of elements, such as text alignment, list numbering, and form layout. Django provides built-in filters and template tags to handle these layout considerations. For example, the add_internationalization
template filter can be used to format numbers according to the current language. Here’s an example:
{{ number|add_internationalization }}
This will format the number
variable based on the current language’s numbering system.
Translation Management in Django
Managing translations in a Django app involves various tasks, such as generating translation files, translating strings, and updating translations when the app’s codebase changes.
Django provides a set of management commands to simplify the translation management process.
To generate translation files for different languages, we can use the makemessages
management command. This command scans the codebase for strings marked for translation and creates or updates the corresponding translation files. For example, to generate translation files for English and Spanish, we can use the following command:
$ python manage.py makemessages -l en $ python manage.py makemessages -l es
This will create two translation files, django.po
, for each language in the locale
directory of the app. The translation files contain the original strings and empty placeholders for the translated strings.
Once the translation files are generated, we can start translating the strings. We can use any text editor or translation management tool to edit the translation files. Here’s an example of a translated string in the django.po
file:
msgid "Hello, World!" msgstr "¡Hola, Mundo!"
The msgid
field represents the original string, and the msgstr
field contains the translated string.
After translating the strings, we need to compile the translation files into binary format using the compilemessages
management command. This command creates .mo
files, which Django uses for runtime translations. Here’s the command to compile the translation files:
$ python manage.py compilemessages
With the translation files compiled, Django will automatically load the appropriate translations based on the user’s language preference.
When the app’s codebase changes, it is important to update the translation files to include any new or modified strings. This can be done using the makemessages
command with the --all
option. For example, to update all translation files in the app, we can use the following command:
$ python manage.py makemessages --all
This will scan the entire codebase and update the translation files accordingly.
Related Article: Advanced Querying and Optimization in Django ORM
Best Practices for Localization in Django
Localization is a complex process that requires careful planning and attention to detail. Here are some best practices for localization in Django:
1. Use the gettext
framework: Django’s integration with the gettext
framework provides a robust and efficient solution for translations. By using the gettext
function to mark strings for translation, we can easily extract and manage translations.
2. Separate translation files: It is recommended to keep the translations separate from the codebase. Storing translations in dedicated files allows for easier management and collaboration with translators.
3. Use language-specific URL routing: Implementing language-specific URLs using language prefixes improves the user experience and makes it easier for search engines to index the different language versions of the app.
4. Consider layout and design for RTL languages: When supporting RTL languages, pay attention to layout considerations such as text alignment, list numbering, and form layout. Use conditional statements and Django’s built-in filters and template tags to handle RTL-specific styles and layout.
5. Test translations thoroughly: Localization involves more than just translating strings. It is important to thoroughly test the app’s functionality and layout in different languages to ensure a seamless user experience.
6. Update translations regularly: As the app evolves, new strings may be added or existing strings may change. It is important to update the translations regularly to keep them up to date with the latest version of the app.
Creating a Multilingual Website in Django
Now that we have explored the various aspects of multilingual support in Django, let’s put it all together and create a simple multilingual website.
First, we need to set up Django for multiple languages by configuring the LANGUAGE_CODE
and LANGUAGES
settings in the Django settings file.
Next, we can start marking the strings that need to be translated using the gettext
function. For example, in our views.py file, we can mark the string “Hello, World!” for translation:
from django.shortcuts import render from django.utils.translation import gettext as _ def home(request): message = _('Hello, World!') return render(request, 'home.html', {'message': message})
In our template (home.html), we can display the translated string using the trans
template tag:
<h1>{% trans message %}</h1>
Once we have marked all the strings for translation, we can generate the translation files using the makemessages
management command:
$ python manage.py makemessages -l en $ python manage.py makemessages -l es
This will create two translation files, django.po
, for English and Spanish, in the locale
directory.
We can then provide translations for the marked strings in the django.po
files. For example, in the English translation file, we can add the following entry:
msgid "Hello, World!" msgstr "Hola, Mundo!"
After translating the strings, we need to compile the translation files using the compilemessages
management command:
$ python manage.py compilemessages
With the translation files compiled, Django will automatically load the appropriate translations based on the user’s language preference.
To implement language preferences, we can add the LocaleMiddleware
to the MIDDLEWARE
setting and the i18n
context processor to the TEMPLATES
setting, as described earlier.
Finally, we can implement dynamic URL routing based on languages by modifying the urlpatterns
list in the Django urls.py file. We can use the i18n_patterns
function to wrap the language-specific URLs.
With these steps in place, we have created a multilingual website in Django. Users can select their preferred language, and the app will display the content in the chosen language.
Additional Resources
– Django documentation – Internationalization
– Django documentation – Translation
– Django i18n and L10n Cookbook