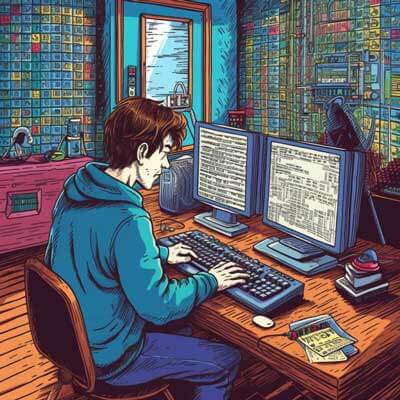
Converting a string to lowercase in Python is a common task that can be easily accomplished using built-in string methods. In this guide, we will explore different approaches to convert a string to lowercase in Python.
Using the lower() method
One simple and straightforward way to convert a string to lowercase in Python is by using the lower()
method. The lower()
method returns a new string where all the characters are converted to lowercase.
Here’s an example:
string = "Hello, World!" lowercase_string = string.lower() print(lowercase_string)
Output:
hello, world!
In the above example, the original string “Hello, World!” is converted to lowercase using the lower()
method, resulting in the lowercase string “hello, world!”.
It’s important to note that the lower()
method does not modify the original string. Instead, it returns a new string with the lowercase characters. If you want to store the lowercase string, you need to assign it to a new variable, as shown in the example.
Related Article: How To Limit Floats To Two Decimal Points In Python
Using the casefold() method
In addition to the lower()
method, Python also provides the casefold()
method to convert a string to lowercase. The casefold()
method is similar to the lower()
method but performs additional transformations that make it suitable for case-insensitive comparisons.
Here’s an example:
string = "Hello, World!" lowercase_string = string.casefold() print(lowercase_string)
Output:
hello, world!
In the above example, the casefold()
method is used to convert the original string “Hello, World!” to lowercase, resulting in the lowercase string “hello, world!”.
Like the lower()
method, the casefold()
method does not modify the original string and returns a new string with the lowercase characters.
Handling Special Characters
When converting a string to lowercase, it’s important to consider how special characters and non-ASCII characters are handled. The behavior may vary depending on the Python version and the specific characters involved.
For example, in some languages, the character “ß” (German sharp s) is converted to “ss” when using lower()
, but to “ss” or “ß” when using casefold()
. It’s important to be aware of these differences and choose the appropriate method based on your specific requirements.
Alternative Approaches
While the lower()
and casefold()
methods are the standard ways to convert a string to lowercase in Python, there are alternative approaches that can be used in certain scenarios.
Using the str.translate() method
The str.translate()
method can be used to perform character-level transformations on a string. By creating a translation table that maps uppercase characters to their lowercase counterparts, we can convert a string to lowercase.
Here’s an example:
string = "Hello, World!" translation_table = str.maketrans("ABCDEFGHIJKLMNOPQRSTUVWXYZ", "abcdefghijklmnopqrstuvwxyz") lowercase_string = string.translate(translation_table) print(lowercase_string)
Output:
hello, world!
In the above example, the str.maketrans()
method is used to create a translation table that maps uppercase characters to lowercase characters. This translation table is then passed to the str.translate()
method to perform the conversion.
While this approach can be useful in certain situations, it is generally less efficient and less readable compared to using the lower()
or casefold()
methods.
Using the str.join() method with a list comprehension
Another alternative approach to convert a string to lowercase is by using the str.join()
method in combination with a list comprehension. This approach involves iterating over each character in the string, converting it to lowercase, and then joining the characters back together.
Here’s an example:
string = "Hello, World!" lowercase_string = ''.join([character.lower() for character in string]) print(lowercase_string)
Output:
hello, world!
In the above example, a list comprehension is used to iterate over each character in the string, convert it to lowercase using the lower()
method, and store the lowercase characters in a list. The str.join()
method is then used to join the characters back together into a single string.
While this approach can be useful in certain scenarios, it is generally less efficient and less concise compared to using the lower()
or casefold()
methods.
Related Article: How To Rename A File With Python
Best Practices
When converting a string to lowercase in Python, consider the following best practices:
1. Use the lower()
method for most cases where you simply need to convert a string to lowercase.
2. Use the casefold()
method if you need to perform case-insensitive comparisons or want consistent behavior across different languages.
3. Be aware of how special characters and non-ASCII characters are handled by the chosen method, as the behavior may differ in specific cases.
4. Avoid using alternative approaches, such as the str.translate()
method or the str.join()
method with a list comprehension, unless there is a specific requirement that cannot be met by the standard methods.