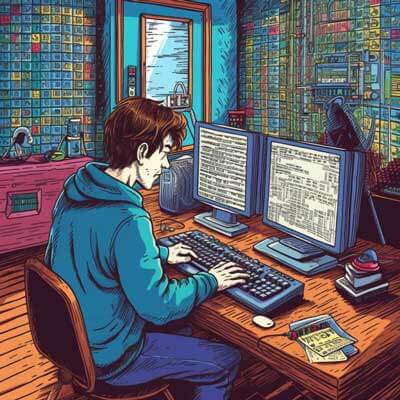
To check if a file exists in Python, you can make use of the os
module. The os
module provides a variety of functions for interacting with the operating system, including file operations. In particular, the os.path
submodule provides methods for working with file paths and file system.
Using the os.path.exists()
function
One way to check if a file exists is by using the os.path.exists()
function. This function takes a file path as an argument and returns True
if the file exists, and False
otherwise.
Here’s an example that demonstrates how to use the os.path.exists()
function:
import os file_path = '/path/to/file.txt' if os.path.exists(file_path): print("File exists") else: print("File does not exist")
In this example, we first import the os
module. Then, we define the file_path
variable with the path to the file we want to check. We use the os.path.exists()
function to check if the file exists, and print an appropriate message based on the result.
Related Article: How To Limit Floats To Two Decimal Points In Python
Using the os.path.isfile()
function
Another way to check if a file exists is by using the os.path.isfile()
function. This function takes a file path as an argument and returns True
if the path points to a regular file, and False
otherwise. This function can be useful if you want to specifically check if the path points to a file, rather than a directory or a symbolic link.
Here’s an example that demonstrates how to use the os.path.isfile()
function:
import os file_path = '/path/to/file.txt' if os.path.isfile(file_path): print("File exists") else: print("File does not exist or is not a regular file")
In this example, we again import the os
module and define the file_path
variable with the path to the file we want to check. We use the os.path.isfile()
function to check if the file exists and is a regular file, and print an appropriate message based on the result.
Why the question is asked
The question “How to check if file exists in Python” is commonly asked because file existence checking is a fundamental operation in many file-related tasks. When working with files, it is often necessary to determine whether a file exists before performing further operations on it, such as reading its contents, writing to it, or deleting it. By checking if a file exists, you can avoid potential errors or unexpected behavior that may occur when trying to work with a non-existent file.
Potential reasons for checking file existence
There are several potential reasons why you might need to check if a file exists in Python:
1. Input validation: If your Python program expects a file path as input from the user or from another part of the program, it is important to validate the input by checking if the file exists. This can help prevent errors and handle invalid or non-existent file paths gracefully.
2. Conditional execution: In certain situations, you may want to conditionally execute a block of code based on whether a file exists or not. For example, you might want to perform different actions based on the presence or absence of a configuration file, a log file, or a data file.
3. Error handling: When working with files, it is important to handle potential errors that may occur, such as file not found errors. By checking if a file exists before performing file operations, you can catch and handle such errors appropriately, for example by displaying an error message or taking alternative actions.
Related Article: How To Rename A File With Python
Suggestions and alternative ideas
When checking if a file exists in Python, there are a few additional considerations and alternative ideas to keep in mind:
1. File permissions: In addition to checking if a file exists, you may also need to consider file permissions. Even if a file exists, you may not have the necessary permissions to read, write, or execute it. In such cases, you may need to handle permission errors separately.
2. Handling directories: The methods described above (os.path.exists()
and os.path.isfile()
) can be used to check if a file exists. However, they do not differentiate between files and directories. If you specifically need to check if a path points to a directory, you can use the os.path.isdir()
function.
3. Avoid race conditions: When checking if a file exists and subsequently performing file operations, it is important to avoid race conditions. A race condition can occur if another process or thread modifies or deletes the file between the time you check its existence and the time you perform file operations. To mitigate this, you can use file locking mechanisms or handle potential errors when accessing the file.
Best practices
When checking if a file exists in Python, it is a good practice to follow these best practices:
1. Use the appropriate function: Choose the appropriate function (os.path.exists()
, os.path.isfile()
, or os.path.isdir()
) based on your specific requirements. If you only need to check if a file exists, using os.path.exists()
or os.path.isfile()
is usually sufficient.
2. Handle exceptions: Even if you check if a file exists before performing file operations, it is still possible for errors to occur. It is important to handle potential exceptions that may be raised when working with files, such as FileNotFoundError
or PermissionError
. Use try-except blocks to catch and handle exceptions appropriately.
3. Use absolute file paths: To ensure consistency and avoid ambiguity, it is recommended to use absolute file paths when checking file existence. Absolute paths provide an unambiguous reference to a file, regardless of the current working directory.
4. Consider file path validation: In addition to checking if a file exists, you may also want to validate the file path itself. This can help prevent potential security vulnerabilities, such as path traversal attacks. Use appropriate methods or libraries to validate and sanitize file paths.