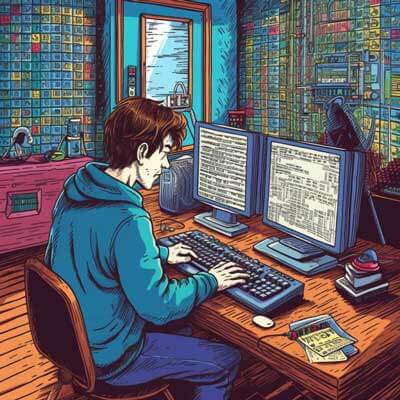
Deleting a file or folder is a common task in any programming language, including Python. In this article, we will explore different methods to delete files and folders using Python. We will cover both basic file deletion and more advanced scenarios, such as deleting non-empty folders and handling exceptions.
Why is this question asked?
The question of how to delete a file or folder in Python is often asked by developers who need to manage files and directories in their applications. There are several reasons why this question may come up:
1. Cleaning up temporary files: Applications often generate temporary files that need to be deleted once they are no longer needed. Knowing how to delete files programmatically allows developers to automate this process and avoid cluttering the file system.
2. Removing unwanted files or folders: Sometimes, you may need to delete specific files or folders as part of your application logic. For example, you might want to remove log files, outdated backups, or any other types of files that are no longer needed.
3. Automating file system management: In some cases, you may need to interact with the file system programmatically to perform tasks such as cleaning up old files, archiving, or organizing files into different folders. Being able to delete files and folders using Python enables you to automate these tasks efficiently.
Related Article: How To Limit Floats To Two Decimal Points In Python
Possible Answers
Answer 1: Using the os module
Python provides the os
module, which offers a wide range of functions for interacting with the operating system. One of the functions provided by this module is os.remove()
, which allows you to delete a file.
Here’s an example of how to use os.remove()
to delete a file:
import os # Specify the path of the file to be deleted file_path = '/path/to/file.txt' # Check if the file exists before attempting to delete it if os.path.exists(file_path): os.remove(file_path) print(f"The file {file_path} has been deleted.") else: print(f"The file {file_path} does not exist.")
In this example, we first check if the file exists using os.path.exists()
. If the file exists, we call os.remove()
with the file’s path to delete it. Finally, we print a message indicating whether the file was deleted or if it does not exist.
It’s important to note that os.remove()
can only delete files and not directories. If you try to delete a directory using os.remove()
, a IsADirectoryError
will be raised.
Answer 2: Using the shutil module
In addition to the os
module, Python also provides the shutil
module, which offers higher-level file operations. The shutil
module includes a function called shutil.rmtree()
that allows you to delete a directory and all its contents recursively.
Here’s an example of how to use shutil.rmtree()
to delete a directory:
import shutil # Specify the path of the directory to be deleted directory_path = '/path/to/directory' # Check if the directory exists before attempting to delete it if os.path.exists(directory_path): shutil.rmtree(directory_path) print(f"The directory {directory_path} has been deleted.") else: print(f"The directory {directory_path} does not exist.")
In this example, we first check if the directory exists using os.path.exists()
. If the directory exists, we call shutil.rmtree()
with the directory’s path to delete it. The function shutil.rmtree()
removes the entire directory tree, including all files and subdirectories.
Related Article: How To Rename A File With Python
Best Practices
When deleting files or folders in Python, it’s important to consider the following best practices:
1. Check if the file or directory exists before attempting to delete it. This helps avoid exceptions and unnecessary processing.
2. Handle exceptions that may occur during the deletion process. For example, if the file is in use by another process or if the user does not have the necessary permissions to delete the file, an exception may be raised. By handling exceptions gracefully, you can provide appropriate error messages to the user and prevent your application from crashing.
3. Be cautious when deleting files or folders recursively. Deleting a directory and its contents can have unintended consequences if not done carefully. Always double-check the path and ensure that you are deleting the correct files or folders.
4. Consider using the send2trash
module instead of permanently deleting files. The send2trash
module moves files to the operating system’s trash or recycle bin instead of deleting them permanently. This provides an extra layer of safety, allowing users to recover accidentally deleted files.