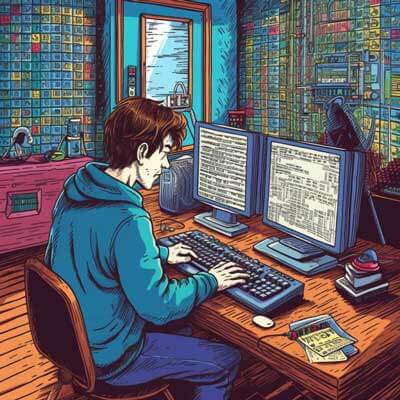
- The Importance of Unicode in Python and Django
- Understanding the Difference Between ASCII and Unicode
- Exploring Character Encoding and its Relationship to Data Formats
- Distinguishing Character Set from Character Encoding
- Comparing UTF-8, UTF-16, and ISO-8859-1
- Handling Special Characters, Diacritics, and Emojis in Python and Django
- Handling Special Characters
- Handling Diacritics
- Handling Emojis
- Common Issues with Input Validation and Sanitization for Multilingual Data
- Handling Input Validation
- Handling Input Sanitization
- Configuring a Database to Support Multilingual Data in Python and Django
- Choosing the Right Database Collation
- Indexing and Querying Multilingual Data
- Encoding Issues in Forms, Templates, and Views
- Encoding Issues in Forms
- Encoding Issues in Templates
- Encoding Issues in Views
- Methods for Handling Encoding Issues in Python and Django
- Explicit Encoding and Decoding
- Using Unicode Literals
- Using Encoding and Decoding Libraries
- Additional Resources
The Importance of Unicode in Python and Django
Unicode is a character encoding standard that aims to encompass all characters from all writing systems in the world. It allows computers to represent and manipulate text in any language, including multilingual data. In the context of Python and Django, Unicode plays a crucial role in handling and managing multilingual data effectively.
Python has built-in support for Unicode, making it a useful tool for working with different languages and character sets. The Unicode string type in Python, represented by the str
class, allows developers to store and manipulate text in any language.
Django, being a popular web framework built on top of Python, also has excellent support for Unicode. It encourages developers to use Unicode strings throughout their applications, ensuring consistent handling of multilingual data.
To work with Unicode in Python and Django, it is important to understand the difference between ASCII and Unicode.
Related Article: 16 Amazing Python Libraries You Can Use Now
Understanding the Difference Between ASCII and Unicode
ASCII (American Standard Code for Information Interchange) is a character encoding standard that represents characters using 7 bits, allowing for a total of 128 characters. It was originally designed for the English language and does not support characters from other languages.
Unicode, on the other hand, is a superset of ASCII and supports a much wider range of characters. It uses a variable number of bytes to represent characters, allowing for the representation of characters from all writing systems.
In Python, ASCII strings are represented by the str
class, while Unicode strings are represented by the unicode
class. However, starting from Python 3, the str
class is used to represent Unicode strings, and the bytes
class is used to represent ASCII and binary data.
In Django, Unicode strings are used extensively throughout the framework. Django models and templates, for example, expect Unicode strings as input and output.
Let’s take a look at an example of working with Unicode strings in Python:
# Python 2 # Define a Unicode string unicode_str = u"Hello, 世界" print(unicode_str) # Python 3 # Define a Unicode string unicode_str = "Hello, 世界" print(unicode_str)
In this example, we define a Unicode string that contains characters from different languages. The u
prefix is used in Python 2 to indicate that the string is Unicode, while in Python 3, Unicode strings are represented by default.
Exploring Character Encoding and its Relationship to Data Formats
Character encoding is the process of representing characters as binary data for storage or transmission. It is closely related to data formats, as different data formats may require different character encodings to represent text correctly.
In the context of Python and Django, understanding character encoding is crucial for handling multilingual data effectively. Let’s explore character encoding and its relationship to data formats in more detail.
Distinguishing Character Set from Character Encoding
Before diving into character encoding, it is important to distinguish between a character set and a character encoding.
A character set is a collection of characters, such as the Latin alphabet, the Cyrillic alphabet, or the Chinese character set. It defines the characters that can be used in a particular language or writing system.
A character encoding, on the other hand, is a mapping between a character set and the binary representation of those characters. It defines how characters are represented as binary data.
For example, the character set for the English language is the ASCII character set, and the ASCII character encoding maps each ASCII character to a unique 7-bit binary representation.
In the case of multilingual data, Unicode is the character set that encompasses characters from all writing systems. Various character encodings, such as UTF-8, UTF-16, and ISO-8859-1, can be used to represent Unicode characters.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
Comparing UTF-8, UTF-16, and ISO-8859-1
UTF-8, UTF-16, and ISO-8859-1 are popular character encodings used to represent Unicode characters. Let’s compare these encodings and understand their differences.
– UTF-8: UTF-8 is a variable-length character encoding that uses 8-bit code units to represent characters. It is backward-compatible with ASCII and can represent any Unicode character. UTF-8 is widely used and recommended for web applications due to its compatibility and efficiency in representing characters.
– UTF-16: UTF-16 is a variable-length character encoding that uses 16-bit code units to represent characters. It can represent any Unicode character and is commonly used in environments that require fixed-width characters.
– ISO-8859-1: ISO-8859-1, also known as Latin-1, is a character encoding that represents the first 256 Unicode characters. It is a fixed-length encoding that uses 8-bit code units. ISO-8859-1 is commonly used for Western European languages but does not support characters from other writing systems.
When working with multilingual data in Python and Django, UTF-8 is the recommended character encoding due to its compatibility, efficiency, and support for all Unicode characters.
Handling Special Characters, Diacritics, and Emojis in Python and Django
Special characters, diacritics, and emojis are common in multilingual data and require special handling to ensure proper representation and manipulation. In Python and Django, there are various techniques and libraries available to handle these characters effectively.
Handling Special Characters
Special characters, such as punctuation marks or symbols, can be represented using Unicode escape sequences in Python and Django. Unicode escape sequences start with a backslash followed by the letter ‘u’ and four hexadecimal digits representing the Unicode code point of the character.
Let’s take an example of representing a special character using a Unicode escape sequence in Python:
# Representing a special character using a Unicode escape sequence special_char = '\u00A9' print(special_char) # Output: ©
In this example, the special character ‘©’ is represented using the Unicode escape sequence ‘\u00A9’. When the code is executed, the special character is correctly displayed.
In Django, special characters can be used in templates by directly including them in the template files. Django’s template engine automatically handles the correct encoding and rendering of special characters.
Related Article: Django 4 Best Practices: Leveraging Asynchronous Handlers for Class-Based Views
Handling Diacritics
Diacritics are marks or signs added to characters to modify their pronunciation or meaning. In Python and Django, diacritics can be handled using Unicode normalization techniques and libraries such as unicodedata
and unidecode
.
Unicode normalization is the process of transforming Unicode text into a canonical form, ensuring that equivalent characters are represented in a consistent way. The unicodedata
module in Python provides functions for normalizing Unicode strings.
Let’s take an example of normalizing a Unicode string in Python:
import unicodedata # Normalize a Unicode string unicode_str = 'Héllo' normalized_str = unicodedata.normalize('NFKD', unicode_str) print(normalized_str) # Output: Hello
In this example, the Unicode string ‘Héllo’ is normalized using the NFKD (Normalization Form KD) normalization form. The resulting string ‘Hello’ is in a normalized form with diacritics removed.
In Django, the unidecode
library can be used to convert Unicode strings with diacritics into ASCII equivalents. This is useful for generating slugs or URLs from user-provided text.
Handling Emojis
Emojis are pictorial representations of emotions, objects, or symbols and have become an integral part of modern communication. In Python and Django, emojis can be handled using Unicode strings and libraries such as emoji
.
Unicode includes a wide range of emojis, and Python and Django provide built-in support for handling them.
Let’s take an example of working with emojis in Python:
# Using emojis in Python emoji_str = "I ❤️ Python 🐍" print(emoji_str) # Output: I ❤️ Python 🐍
In this example, the Unicode emojis ❤️ and 🐍 are used in a Python string. When the code is executed, the emojis are correctly displayed.
In Django, emojis can be used in templates by directly including them in the template files. Django’s template engine automatically handles the correct encoding and rendering of emojis.
Common Issues with Input Validation and Sanitization for Multilingual Data
Input validation and sanitization are crucial steps in handling multilingual data effectively. They help prevent security vulnerabilities, data corruption, and other issues. In Python and Django, there are common issues that need to be addressed when validating and sanitizing multilingual data.
Related Article: String Comparison in Python: Best Practices and Techniques
Handling Input Validation
Input validation is the process of verifying that user input meets specific criteria or constraints. When dealing with multilingual data, input validation becomes more complex due to the different character sets and encodings involved.
One common issue in input validation for multilingual data is ensuring that the input contains valid characters for the intended language or writing system. This can be done by defining a whitelist of allowed characters or using regular expressions to match the input against valid patterns.
Let’s take an example of validating input for a specific language in Python:
import re # Validate input for English text def validate_english_input(input_str): # Define a regular expression pattern for English text pattern = re.compile(r'^[a-zA-Z0-9\s!@#$%^&*()_+=-]+$') # Check if the input matches the pattern if not pattern.match(input_str): raise ValueError("Invalid input. Only English characters, numbers, and special characters are allowed.") # Validation passed, do further processing # ...
In this example, the validate_english_input
function validates the input for English text. It uses a regular expression pattern that matches English characters, numbers, and a set of allowed special characters. If the input does not match the pattern, a ValueError
is raised.
In Django, input validation can be performed using form validation or custom validators. Django provides various form fields and validators that can be used to validate multilingual data.
Handling Input Sanitization
Input sanitization is the process of removing or escaping potentially malicious or unintended characters from user input. It helps prevent security vulnerabilities, such as SQL injection or cross-site scripting (XSS) attacks.
When working with multilingual data, input sanitization becomes more challenging due to the different character sets and encodings involved. It is important to properly handle and sanitize input to ensure data integrity and security.
One common issue in input sanitization for multilingual data is the handling of special characters and control characters. Special characters, such as quotes or backslashes, can be escaped or removed from the input to prevent them from being interpreted as commands or control characters.
Let’s take an example of sanitizing input in Python using the html
module:
import html # Sanitize input by escaping special characters def sanitize_input(input_str): return html.escape(input_str)
In this example, the sanitize_input
function uses the html.escape
function from the html
module to escape special characters in the input. This helps prevent HTML injection or XSS attacks by converting special characters to their HTML entity representations.
In Django, input sanitization can be performed using built-in functions or libraries such as bleach
or django-bleach
. These libraries provide sanitization functions that can be used to clean user input before storing or displaying it.
Configuring a Database to Support Multilingual Data in Python and Django
Supporting multilingual data in a database requires proper configuration and consideration of character encodings, collations, and indexing. In Python and Django, configuring a database to handle multilingual data effectively involves specific steps and considerations.
Related Article: How to Replace Strings in Python using re.sub
Choosing the Right Database Collation
Collation is a set of rules that determines how characters are sorted and compared in a database. When working with multilingual data, it is important to choose the right collation to ensure proper sorting and comparison of characters.
In Django, the database collation can be specified in the database settings. For example, to use the UTF-8 collation for a PostgreSQL database, the following configuration can be added to the settings file:
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'mydatabase', 'USER': 'myuser', 'PASSWORD': 'mypassword', 'HOST': 'localhost', 'PORT': '5432', 'OPTIONS': { 'options': '-c collation_server=utf8_general_ci', }, } }
In this example, the options
parameter is used to specify the collation for the PostgreSQL database as utf8_general_ci
. This collation supports case-insensitive sorting and comparison of UTF-8 characters.
It is important to choose a collation that matches the character encoding used in the database and the application. Inconsistent collations can lead to sorting and comparison issues when working with multilingual data.
Indexing and Querying Multilingual Data
Indexing is an important aspect of optimizing database performance for multilingual data. Proper indexing allows for efficient querying and sorting of data, especially when dealing with large datasets.
When working with multilingual data in Python and Django, it is important to create indexes that consider the specific needs of the application and the data being stored.
In Django, indexes can be defined in models using the db_index
attribute. For example, to create an index on a field that stores multilingual data, the following configuration can be added to the model:
from django.db import models class MyModel(models.Model): my_field = models.CharField(max_length=100, db_index=True)
In this example, the db_index=True
attribute is set on the my_field
field to create an index. This allows for faster querying and sorting of data based on the my_field
field.
It is important to carefully consider the fields that require indexing based on the specific requirements of the application. Over-indexing can lead to decreased performance and increased storage requirements.
Encoding Issues in Forms, Templates, and Views
Handling encoding issues is crucial when working with multilingual data in forms, templates, and views in Python and Django. Encoding issues can lead to data corruption, display problems, or security vulnerabilities if not handled properly.
Related Article: How to Work with CSV Files in Python: An Advanced Guide
Encoding Issues in Forms
Forms in Django are used to handle user input and validate data. When working with multilingual data, encoding issues can arise when processing form data that contains characters from different languages.
One common encoding issue in forms is the encoding of form data received from the client. By default, Django uses the encoding specified in the DEFAULT_CHARSET
setting to decode form data. It is important to ensure that the DEFAULT_CHARSET
setting matches the encoding used by the client.
For example, if the client sends form data encoded in UTF-8, the DEFAULT_CHARSET
setting should be set to 'utf-8'
in the Django settings file:
DEFAULT_CHARSET = 'utf-8'
Additionally, it is important to specify the correct encoding in the HTML form tag to ensure that the form data is sent with the correct encoding. The accept-charset
attribute can be used to specify the encoding:
<!-- form fields here -->
Encoding Issues in Templates
Templates in Django are used to generate HTML, XML, or other output formats based on data provided by views. When working with multilingual data, encoding issues can arise when rendering templates that contain characters from different languages.
One common encoding issue in templates is the encoding of variables that contain multilingual data. By default, Django uses the encoding specified in the DEFAULT_CHARSET
setting to encode template variables. It is important to ensure that the DEFAULT_CHARSET
setting matches the encoding used by the client.
For example, if the client expects the output to be encoded in UTF-8, the DEFAULT_CHARSET
setting should be set to 'utf-8'
in the Django settings file.
DEFAULT_CHARSET = 'utf-8'
Additionally, it is important to specify the correct encoding in the HTML meta tag to ensure that the rendered output is displayed correctly. The charset
attribute can be used to specify the encoding:
<!-- other meta tags and head elements --> <!-- body content here -->
Encoding Issues in Views
Views in Django handle the processing of HTTP requests and generate responses. When working with multilingual data, encoding issues can arise when processing request data or generating response data.
One common encoding issue in views is the encoding of data sent in the response. By default, Django uses the encoding specified in the DEFAULT_CHARSET
setting to encode response data. It is important to ensure that the DEFAULT_CHARSET
setting matches the encoding expected by the client.
For example, if the client expects the response to be encoded in UTF-8, the DEFAULT_CHARSET
setting should be set to 'utf-8'
in the Django settings file.
DEFAULT_CHARSET = 'utf-8'
Additionally, it is important to specify the correct encoding in the Content-Type
header of the response to ensure that the client interprets the response correctly. The charset
parameter can be used to specify the encoding:
from django.http import HttpResponse def my_view(request): response = HttpResponse(content_type='text/html; charset=utf-8') # generate response content return response
Related Article: How to Work with Lists and Arrays in Python
Methods for Handling Encoding Issues in Python and Django
When working with multilingual data in Python and Django, there are several methods and techniques available to handle encoding issues effectively. Let’s explore some of these methods.
Explicit Encoding and Decoding
One method for handling encoding issues is to explicitly encode and decode strings using the encode()
and decode()
methods. These methods allow you to specify the desired encoding when converting between Unicode strings and byte strings.
For example, to encode a Unicode string to UTF-8, you can use the encode()
method:
unicode_str = "Hello, 世界" utf8_bytes = unicode_str.encode('utf-8')
In this example, the encode()
method is used to encode the Unicode string unicode_str
to UTF-8, resulting in a byte string utf8_bytes
.
To decode a byte string to a Unicode string, you can use the decode()
method:
utf8_bytes = b'Hello, \xe4\xb8\x96\xe7\x95\x8c' unicode_str = utf8_bytes.decode('utf-8')
In this example, the decode()
method is used to decode the byte string utf8_bytes
to a Unicode string, resulting in the Unicode string unicode_str
.
Using Unicode Literals
In Python, you can use Unicode literals to specify Unicode characters directly in your code. Unicode literals start with the u
prefix and allow you to include Unicode characters using their code points or escape sequences.
For example, to define a Unicode string containing the Euro sign, you can use a Unicode literal:
euro_sign = u'\u20AC'
In this example, the Unicode literal u'\u20AC'
represents the Euro sign character.
Using Unicode literals can help avoid encoding issues when working with multilingual data, as the characters are directly represented in their Unicode form.
Related Article: How to Use Switch Statements in Python
Using Encoding and Decoding Libraries
Python provides several libraries that can be used to handle encoding and decoding of strings, such as chardet
and iconvcodec
. These libraries can automatically detect the encoding of a string or convert between different encodings.
For example, the chardet
library can be used to detect the encoding of a byte string:
import chardet byte_str = b'Hello, \xe4\xb8\x96\xe7\x95\x8c' result = chardet.detect(byte_str) print(result['encoding']) # Output: utf-8
In this example, the chardet.detect()
function is used to detect the encoding of the byte string byte_str
. The detected encoding is then printed.