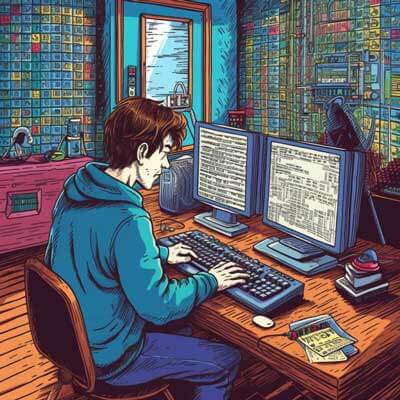
To check the type of a variable in Python, you can use the built-in type()
function. The type()
function returns the type of the variable as a string. Here’s how you can use it:
x = 5 print(type(x)) # Output: y = "Hello, world!" print(type(y)) # Output: z = [1, 2, 3] print(type(z)) # Output:
In the example above, we define three variables x
, y
, and z
with different types: an integer, a string, and a list. By calling the type()
function and passing the variable as an argument, we can determine the type of each variable.
It’s important to note that the type()
function returns the exact type of the variable, including any custom classes or modules. For example:
import math circle_radius = 5.0 print(type(circle_radius)) # Output: print(type(math)) # Output:
In this example, we import the math
module and define a variable circle_radius
with a floating-point value. The type()
function correctly identifies the type of circle_radius
as a float. Similarly, it recognizes the math
module as a module.
Using the isinstance() Function
In addition to the type()
function, Python provides the isinstance()
function to check if a variable is an instance of a particular class or type. The isinstance()
function returns True
if the variable is an instance of the specified class or type, and False
otherwise.
Here’s an example that demonstrates the usage of the isinstance()
function:
x = 5 print(isinstance(x, int)) # Output: True print(isinstance(x, float)) # Output: False y = [1, 2, 3] print(isinstance(y, list)) # Output: True print(isinstance(y, tuple)) # Output: False
In this example, we use the isinstance()
function to check if the variables x
and y
are instances of specific classes or types. The first print()
statement returns True
because x
is an instance of the int
class. The second print()
statement returns False
because x
is not an instance of the float
class.
Similarly, the third print()
statement returns True
because y
is an instance of the list
class. The fourth print()
statement returns False
because y
is not an instance of the tuple
class.
Related Article: String Comparison in Python: Best Practices and Techniques
Best Practices
When checking the type of a variable, it’s good practice to use the isinstance()
function instead of the type()
function in most cases. The isinstance()
function allows you to perform more specific type checks and is more flexible when working with inheritance and class hierarchies.
Here are some best practices to keep in mind:
1. Use the isinstance()
function when you need to check if a variable is an instance of a specific class or type.
2. Use the type()
function when you only need to determine the general type of a variable.
3. Avoid relying too heavily on type checking in your code. Python is a dynamically-typed language, and it’s often more effective to use duck typing and rely on the behavior of objects rather than their specific types.
4. If you need to perform different actions based on the type of a variable, consider using polymorphism and object-oriented design principles instead of explicit type checks.
Related Article: How To Limit Floats To Two Decimal Points In Python