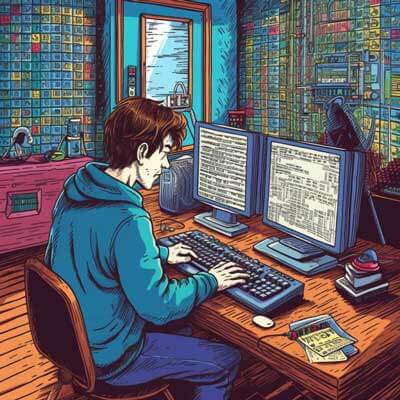
Moving a file in Python is a common task that you may need to perform while working with files and directories. In this guide, we will explore different ways to move a file using Python.
Why is the question asked?
The question of how to move a file in Python is often asked by developers who want to automate file management tasks or need to organize files within their applications. Moving a file can be useful in scenarios where you want to reorganize files, create backups, or perform specific operations on files in a different location.
Related Article: How To Limit Floats To Two Decimal Points In Python
Possible Solutions
1. Using the shutil module
Python provides the shutil
module, which offers a high-level interface for file operations. To move a file using shutil
, you can make use of the shutil.move()
function.
Here’s an example that demonstrates how to move a file using shutil
:
import shutil # Specify the source file path source_file = '/path/to/source/file.txt' # Specify the destination directory path destination_directory = '/path/to/destination/' # Move the file shutil.move(source_file, destination_directory)
In the above code, you need to provide the source file path and the destination directory path. The shutil.move()
function will move the file from the source location to the destination directory.
It’s important to note that if a file with the same name already exists in the destination directory, the existing file will be replaced by the moved file.
2. Using the os module
Another way to move a file in Python is by making use of the os
module. The os
module provides lower-level functions for interacting with the operating system.
Here’s an example that demonstrates how to move a file using os.rename()
:
import os # Specify the source file path source_file = '/path/to/source/file.txt' # Specify the destination directory path destination_directory = '/path/to/destination/' # Create the destination directory if it doesn't exist if not os.path.exists(destination_directory): os.makedirs(destination_directory) # Move the file os.rename(source_file, os.path.join(destination_directory, os.path.basename(source_file)))
In the above code, we first check if the destination directory exists. If it doesn’t, we create it using os.makedirs()
. Then, we use os.rename()
to move the file to the destination directory. The os.path.join()
function is used to construct the new file path in the destination directory.
Best Practices
When moving files in Python, it’s important to consider some best practices:
1. Handle exceptions: File operations can fail due to various reasons such as permission issues or file not found errors. It’s a good practice to handle exceptions when moving files and provide appropriate error messages or fallback strategies.
2. Check file existence: Before moving a file, it’s advisable to check if the file exists at the source location. You can use os.path.exists()
to check if the file exists before attempting to move it.
3. Verify file movement: After moving a file, it’s a good practice to verify that the file has been successfully moved to the desired location. You can use os.path.exists()
again to check if the file exists at the new location.
4. Use absolute file paths: When specifying file paths, it’s recommended to use absolute paths instead of relative paths. This ensures that the file is moved to the correct location regardless of the current working directory.
Alternative Ideas
Apart from using the shutil
and os
modules, there are other third-party libraries available that can help with file operations in Python. Some popular libraries include pathlib
and pyfilesystem
. These libraries provide additional features and abstractions for working with files and directories.
Here’s an example using the pathlib
library to move a file:
from pathlib import Path # Specify the source file path source_file = Path('/path/to/source/file.txt') # Specify the destination directory path destination_directory = Path('/path/to/destination/') # Move the file source_file.rename(destination_directory / source_file.name)
In the above code, we use the Path
class from the pathlib
module to represent file paths. The rename()
method is used to move the file to the destination directory.
Related Article: How To Rename A File With Python