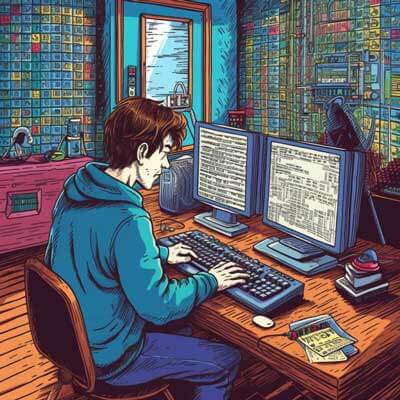
Regex, short for regular expression, is a tool used for pattern matching and search operations in strings. It provides a concise and flexible way to specify patterns that can be used to match, search, and manipulate text. However, there are scenarios where you may need to use a regex pattern to negate or exclude certain matches. In this guide, we will explore how to use regex to perform a “not match” operation.
Why would you need to use a “not match” operation?
There are several reasons why you might need to use a “not match” operation with regex:
1. Filtering: You may want to filter out certain patterns or matches from a larger set of data. For example, you might want to exclude all email addresses that contain a specific domain name or exclude lines in a log file that match a particular pattern.
2. Validation: In some cases, you may want to ensure that a string does not match a certain pattern. For instance, you might want to validate that a password does not contain any common patterns like sequential numbers or repeating characters.
3. Refactoring: When refactoring code, you may need to identify parts that do not match a specific pattern. This can help you find areas that need to be modified or updated.
Related Article: What is Test-Driven Development? (And How To Get It Right)
Using the caret (^) symbol
One way to perform a “not match” operation with regex is by using the caret (^) symbol. In regex, the caret symbol has a special meaning when used at the beginning of a character class. It negates the character class, effectively excluding any characters that match the pattern within the character class.
For example, the regex pattern [^0-9]
matches any character that is not a digit. This means it will exclude all digits from the string and match any other character. Here’s an example in Python:
import re string = "Hello123World" matches = re.findall("[^0-9]", string) print(matches) # Output: ['H', 'e', 'l', 'l', 'o', 'W', 'o', 'r', 'l', 'd']
In the example above, the regex pattern [^0-9]
matches all characters that are not digits in the string “Hello123World”. The re.findall()
function returns a list of all matches, which in this case are all the non-digit characters in the string.
Using negative lookaheads
Another way to perform a “not match” operation with regex is by using negative lookaheads. A negative lookahead is a zero-width assertion that allows you to specify a pattern that should not be present after the current position.
To use a negative lookahead, you can use the syntax (?!pattern)
. This asserts that the given pattern does not match at the current position. Here’s an example in JavaScript:
const string = "Hello123World"; const matches = string.match(/(?!123)\w/g); console.log(matches); // Output: ['H', 'e', 'l', 'l', 'o', 'W', 'o', 'r', 'l', 'd']
In the example above, the regex pattern (?!123)\w
matches any word character that is not followed by the sequence “123”. The string.match()
function returns an array of all matches, which in this case are all the word characters that are not followed by “123” in the string “Hello123World”.
Using the pipe (|) symbol
The pipe (|) symbol can also be used to perform a “not match” operation by specifying multiple patterns separated by the pipe symbol. This allows you to match any pattern except the ones specified.
For example, the regex pattern ^(?!dog$|cat$)
matches any word that is not exactly “dog” or “cat”. Here’s an example in Perl:
my $string = "Hello world"; my @matches = $string =~ /^(?!dog$|cat$)\w+/g; print "@matches"; # Output: Hello world
In the example above, the regex pattern ^(?!dog$|cat$)\w+
matches any word character that is not exactly “dog” or “cat” at the beginning of a line. The =~
operator is used to match the pattern against the string and the \w+
matches one or more word characters.
Related Article: 16 Amazing Python Libraries You Can Use Now