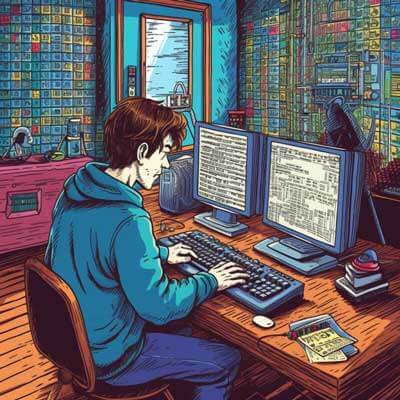
Table of Contents
When working with lists in Python, it is often necessary to check whether a list is empty or not. This can be useful in various scenarios, such as checking if a list has any elements before performing certain operations or validating user input. In this guide, we will explore different ways to check if a list is empty in Python.
Method 1: Using the len() function
One simple way to check if a list is empty is by using the built-in len()
function. The len()
function returns the number of elements in a list, and if the length of the list is zero, it means the list is empty.
Here's an example of how to use the len()
function to check if a list is empty:
my_list = [] if len(my_list) == 0: print("The list is empty") else: print("The list is not empty")
In this example, we define an empty list my_list
and then use the len()
function to check its length. If the length is equal to zero, we print "The list is empty". Otherwise, we print "The list is not empty".
Related Article: How to Add a Gitignore File for Python Projects
Method 2: Using the not operator
Another way to check if a list is empty is by using the not
operator. The not
operator is a logical operator that returns True
if the operand is False
, and False
if the operand is True
. In Python, an empty list evaluates to False
, so we can use the not
operator to check if the list is empty.
Here's an example of how to use the not
operator to check if a list is empty:
my_list = [] if not my_list: print("The list is empty") else: print("The list is not empty")
In this example, we use the not
operator to check if my_list
is empty. If my_list
is empty, the condition evaluates to True
, and we print "The list is empty". Otherwise, we print "The list is not empty".
Why is this question asked?
The question "How to check if a list is empty in Python?" is commonly asked by Python developers, especially those who are new to the language. Checking if a list is empty is a fundamental operation in Python programming, and understanding how to do it correctly is important for writing reliable and bug-free code.
Some potential reasons why this question is asked include:
- Validating user input: When receiving input from users, it is important to check if the input is empty or not before processing it further. This helps to ensure that the program handles empty input gracefully without causing any errors.
- Conditional execution: In certain cases, it may be necessary to perform certain operations only if a list is not empty. By checking if the list is empty, the program can decide whether to execute a particular block of code or not.
- Error handling: When working with data structures like lists, it is important to handle edge cases, such as empty lists, to avoid potential errors or exceptions. Checking if a list is empty allows the program to handle such cases appropriately.
Suggestions and Alternative Ideas
While the methods mentioned above are the most common ways to check if a list is empty in Python, there are a few alternative ideas and suggestions worth considering:
1. Using the if
statement directly: In Python, the if
statement can be used directly to check if a list is empty. Since an empty list evaluates to False
, we can use the list itself as the condition in the if
statement.
my_list = []
if my_list:
print("The list is not empty")
else:
print("The list is empty")
This code is equivalent to the previous examples but uses the
if
statement directly instead of using thenot
operator orlen()
function.
2. Using the
bool()
function: Thebool()
function can be used to convert any value to its corresponding boolean representation. In the case of lists, an empty list will be converted toFalse
, while a non-empty list will be converted toTrue
.
my_list = []
if bool(my_list):
print("The list is not empty")
else:
print("The list is empty")
This approach is similar to using the
not
operator but explicitly converts the list to a boolean value using thebool()
function.
Related Article: How to Implement a Python Foreach Equivalent
Best Practices
When checking if a list is empty in Python, it is important to follow some best practices to ensure clean and maintainable code:
1. Use the most concise and readable method: In Python, there are multiple ways to check if a list is empty. It is recommended to use the method that is most concise and readable for your specific use case. Using the
if
statement directly or thenot
operator is generally preferred over using thelen()
function, as they convey the intent more clearly.
2. Comment the code if necessary: If the purpose of the code is not immediately clear, it can be helpful to add comments explaining why the check is being performed. This can make the code more understandable for other developers who may work on the project in the future.
3. Consider the context: When checking if a list is empty, it is important to consider the context in which the check is being performed. If the list is expected to be empty in certain cases, it may be necessary to handle those cases separately and provide appropriate feedback to the user or perform alternative actions.