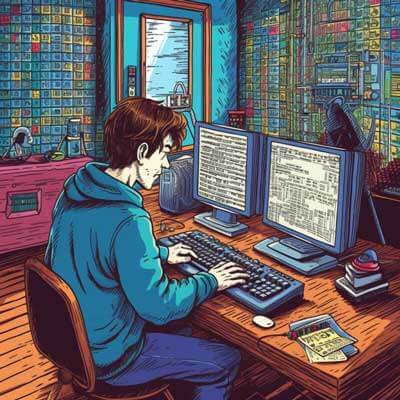
Table of Contents
Importing other Python files in your code is a common practice that allows you to reuse code and organize your project more effectively. There are a few different ways to import files in Python, depending on your specific needs. In this answer, we will explore the different import statements and techniques available to you.
1. Importing a Python Module
The most common way to import other Python files is by importing them as modules. A module is a file containing Python definitions and statements that can be used in other Python programs. To import a module, you can use the import
statement followed by the name of the module. Here's an example:
import module_name
Once you've imported a module, you can access its functions, classes, and variables using the dot notation. For example, if the module contains a function called my_function
, you can access it like this:
module_name.my_function()
If you want to import only specific functions or variables from a module, you can use the from
keyword followed by the module name and the names of the functions or variables you want to import. Here's an example:
from module_name import function_name, variable_name
Now you can use the imported functions and variables directly without having to prefix them with the module name.
Related Article: How to Manipulate Strings in Python and Check for Substrings
2. Importing a Python File as a Script
Sometimes you may want to import a Python file as a script rather than as a module. When you import a file as a script, any code in the file that is not inside a function or a class will be executed immediately. To import a file as a script, you can use the import
statement followed by the name of the file. Here's an example:
import file_name
When you import a file as a script, any code in the file will be executed. This can be useful for running initialization code or defining global variables. However, it's important to note that importing a file as a script can have side effects and may not be the best practice in all situations.
3. Importing a Python File from a Different Directory
In some cases, you may need to import a Python file from a different directory. To do this, you can add the directory containing the file to the Python module search path. You can modify the module search path using the sys.path
list. Here's an example:
import sys sys.path.append('/path/to/directory')
Once you've added the directory to the module search path, you can import the file using the regular import
statement. Python will search for the file in the directories listed in sys.path
.
It's important to note that modifying sys.path
can have unintended consequences and should be used with caution. It's generally recommended to use relative imports or package structures to organize your code instead.
Best Practices
When importing other Python files in your code, it's important to follow some best practices to ensure clean and maintainable code:
1. Import modules at the beginning of your code: It's a good practice to import all the required modules at the beginning of your code. This makes it easier for other developers to understand the dependencies of your code.
2. Use explicit imports: Instead of using wildcard imports (from module_name import *
), it's better to import only the specific functions, classes, or variables that you need. This makes your code more readable and avoids namespace clashes.
3. Use clear and descriptive module and variable names: Choose meaningful names for your modules and variables to make your code more readable and maintainable. Avoid using generic names like utils
or helpers
as they can easily clash with other modules.
4. Organize your code into packages: If you have a large project with multiple files, consider organizing your code into packages. Packages allow you to group related modules together and provide a logical structure to your codebase.
5. Follow PEP 8 style guide: PEP 8 is the official Python style guide that provides guidelines on how to format your code. Following consistent coding style helps maintain readability and makes collaboration easier.