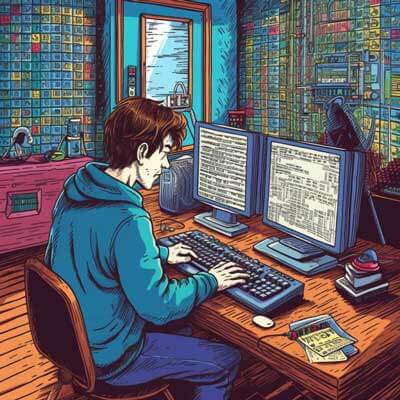
To limit floats to two decimal points in Python, you can make use of the built-in format()
function or the round()
function. These functions provide different ways to achieve the desired result based on your specific requirements. In this guide, we will explore both methods and explain when to use each one.
Method 1: Using the format() function
The format()
function in Python allows you to format a float value to a specific number of decimal places. To limit a float to two decimal points using this method, you can specify the desired format as follows:
num = 3.14159 formatted_num = format(num, ".2f") print(formatted_num)
Output:
3.14
In the above example, the format()
function is used to format the num
variable with the format specifier ".2f"
. The ".2f"
format specifier indicates that the float should be formatted with two decimal places. The resulting formatted value is stored in the formatted_num
variable and then printed.
Related Article: How To Rename A File With Python
Method 2: Using the round() function
The round()
function in Python allows you to round a float value to a specified number of decimal places. To limit a float to two decimal points using this method, you can use the round()
function with the desired number of decimal places specified as the second argument:
num = 3.14159 rounded_num = round(num, 2) print(rounded_num)
Output:
3.14
In the above example, the round()
function is used to round the num
variable to two decimal places. The resulting rounded value is stored in the rounded_num
variable and then printed.
Why would you want to limit floats to two decimal points?
Limiting floats to two decimal points is a common requirement in many applications, especially those involving financial calculations or displaying numeric values to users. By limiting the number of decimal places, you can ensure that the output remains concise and easy to read. Additionally, it can help avoid potential rounding errors or unnecessary precision in certain scenarios.
Some potential reasons for limiting floats to two decimal points include:
– Consistency: Limiting floats to two decimal points ensures that all values are displayed in a consistent manner throughout the application or system.
– Presentation: Limiting floats to two decimal points can improve the visual presentation of numeric values, making them easier to understand for users.
– Data storage: In some cases, limiting the number of decimal places can help optimize data storage and reduce memory usage.
Suggestions and alternative ideas
While the format()
and round()
functions are the most straightforward and commonly used methods to limit floats to two decimal points in Python, there are alternative approaches you can consider depending on your specific needs.
1. String formatting: Another way to limit floats to two decimal points is by using string formatting. You can achieve this by using the %
operator or the newer str.format()
method:
Using %
operator:
num = 3.14159 formatted_num = "%.2f" % num print(formatted_num)
Using str.format()
method:
num = 3.14159 formatted_num = "{:.2f}".format(num) print(formatted_num)
Both of these approaches produce the same result as the format()
function or the round()
function.
2. Decimal module: If you are dealing with precise decimal calculations, you may want to consider using the decimal
module in Python. The decimal
module provides more control over decimal places and rounding methods:
from decimal import Decimal, ROUND_HALF_UP num = Decimal("3.14159") rounded_num = num.quantize(Decimal("0.00"), rounding=ROUND_HALF_UP) print(rounded_num)
In the above example, the Decimal
class from the decimal
module is used to create a decimal object. The quantize()
method is then used to limit the decimal places to two using the Decimal("0.00")
argument. The rounding
parameter is set to ROUND_HALF_UP
to round the decimal value.
Related Article: How To Check If List Is Empty In Python
Best practices
When limiting floats to two decimal points in Python, it is important to consider the following best practices:
1. Use the appropriate method based on your requirements: The format()
function and the round()
function are suitable for most cases where limiting floats to two decimal points is required. However, if you need more control over decimal places or rounding methods, consider using the decimal
module.
2. Be aware of floating-point precision: Floating-point numbers in Python (and most programming languages) have limited precision due to the way they are represented internally. This can lead to rounding errors or unexpected results when performing calculations. If high precision is crucial, consider using the decimal
module or other specialized libraries.
3. Consider localization: In some cases, you may need to format floats with two decimal points according to the specific locale or regional settings. Python provides the locale
module for handling localization and formatting numbers based on the user’s locale preferences.
4. Document your formatting choices: When working on a project with multiple developers or maintaining code over time, it is helpful to document the formatting choices you make, especially if they deviate from the default behavior. This can help others understand the reasoning behind the formatting and avoid confusion.