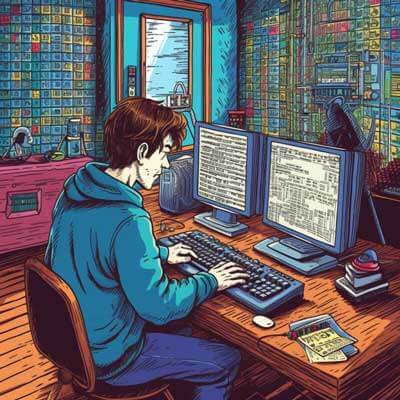
Table of Contents
Introduction to Python Data Types
Python is a versatile programming language that offers a wide range of data types to store and manipulate different kinds of data. Understanding the various data types is crucial for effective programming and data manipulation in Python. In this chapter, we will explore the basic Python data types and their usage.
Related Article: How to Work with Lists and Arrays in Python
List of Python Data Types and Their Use Cases
Python provides several built-in data types that can be used to store and manipulate different kinds of data. Some of the commonly used data types include:
1. Integer: Used to represent whole numbers, such as 1, 2, -5, etc.
Example:
num = 10 print(num)
2. Float: Used to represent decimal numbers, such as 3.14, 2.5, -0.75, etc.
Example:
pi = 3.14 print(pi)
3. String: Used to represent a sequence of characters enclosed in single quotes ('') or double quotes ("").
Example:
name = "John Doe" print(name)
4. List: Used to store a collection of items in a specific order. Lists are mutable, meaning they can be modified.
Example:
fruits = ["apple", "banana", "orange"] print(fruits)
5. Tuple: Similar to lists, but tuples are immutable, meaning they cannot be modified once created.
Example:
point = (3, 5) print(point)
6. Dictionary: Used to store key-value pairs. Each value is associated with a unique key.
Example:
person = {"name": "John Doe", "age": 30, "city": "New York"} print(person)
7. Set: Used to store a collection of unique items. Sets do not allow duplicate values.
Example:
fruits = {"apple", "banana", "orange"} print(fruits)
These are just a few examples of Python's built-in data types. Each data type has its own specific use cases, and understanding them is essential for effective programming.
Working with Float in Python
Float is a built-in data type in Python that represents decimal numbers. Floats are useful for dealing with numbers that require fractional precision. In Python, floats can be manipulated using various arithmetic operators.
Example 1: Basic Arithmetic Operations
x = 3.14 y = 2.5 # Addition sum = x + y print(sum) # Subtraction difference = x - y print(difference) # Multiplication product = x * y print(product) # Division quotient = x / y print(quotient)
Example 2: Rounding Floats
x = 3.141592653589793238 rounded = round(x, 2) print(rounded)
In the first example, we perform basic arithmetic operations on two float variables. The result of each operation is printed using the print() function.
In the second example, we use the round() function to round a float number to a specified number of decimal places. In this case, we round the number x to 2 decimal places.
Working with Decimal in Python
While floats are useful for most decimal calculations, they have limited precision. Python provides the decimal module to handle decimal numbers with arbitrary precision.
Example 1: Basic Decimal Operations
from decimal import Decimal x = Decimal('3.14') y = Decimal('2.5') # Addition sum = x + y print(sum) # Subtraction difference = x - y print(difference) # Multiplication product = x * y print(product) # Division quotient = x / y print(quotient)
Example 2: Rounding Decimals
from decimal import Decimal, ROUND_HALF_UP x = Decimal('3.141592653589793238') rounded = x.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP) print(rounded)
In the first example, we import the Decimal class from the decimal module. We then perform basic arithmetic operations using Decimal objects instead of regular floats.
In the second example, we use the quantize() method to round a decimal number to a specified number of decimal places. We also specify the rounding method using the ROUND_HALF_UP constant.
Related Article: How to Work with CSV Files in Python: An Advanced Guide
Working with Time and Date in Python
Python provides the datetime module for working with dates and times. This module allows us to create, manipulate, and format dates and times in various ways.
Example 1: Current Date and Time
from datetime import datetime current_datetime = datetime.now() print(current_datetime)
Example 2: Formatting Dates
from datetime import date today = date.today() formatted_date = today.strftime("%Y-%m-%d") print(formatted_date)
In the first example, we import the datetime class from the datetime module. We then create a datetime object representing the current date and time using the now() method. The result is printed using the print() function.
In the second example, we import the date class from the datetime module. We then create a date object representing the current date using the today() method. We format the date as a string using the strftime() method and print the formatted date.
Creating Custom Data Types in Python
Python allows us to create custom data types using classes. By defining a class, we can create objects with their own attributes and behaviors.
Example 1: Creating a Custom Class
class Rectangle: def __init__(self, width, height): self.width = width self.height = height def area(self): return self.width * self.height # Create an instance of the Rectangle class rectangle = Rectangle(3, 4) print(rectangle.area())
Example 2: Adding Methods to a Custom Class
class Circle: def __init__(self, radius): self.radius = radius def area(self): return 3.14 * self.radius ** 2 def circumference(self): return 2 * 3.14 * self.radius # Create an instance of the Circle class circle = Circle(5) print(circle.area()) print(circle.circumference())
In the first example, we define a custom class called Rectangle. The class has an initializer method (__init__) that sets the width and height attributes of the object. We also define an area() method that calculates and returns the area of the rectangle.
In the second example, we define a custom class called Circle. Similar to the Rectangle class, it has an initializer method and two additional methods: area() and circumference(). These methods calculate and return the area and circumference of the circle, respectively.
Working with Dict in Python
Python's built-in dict type is used to store key-value pairs. Dicts are unordered collections of items, where each item is accessed by its key.
Example 1: Creating a Dictionary
person = { "name": "John Doe", "age": 30, "city": "New York" } print(person)
Example 2: Accessing Dictionary Values
person = { "name": "John Doe", "age": 30, "city": "New York" } print(person["name"]) print(person.get("age"))
In the first example, we create a dictionary called person that stores information about a person. The keys are "name", "age", and "city", and the corresponding values are "John Doe", 30, and "New York", respectively. The entire dictionary is printed using the print() function.
In the second example, we access the values of the dictionary. We can access a value by specifying its key in square brackets ([]), or by using the get() method. In both cases, the value is printed using the print() function.
Working with Arrays in Python
In Python, arrays are implemented using the array module. Arrays are similar to lists, but they can only store elements of the same type.
Example 1: Creating an Array
from array import array numbers = array("i", [1, 2, 3, 4, 5]) print(numbers)
Example 2: Accessing Array Elements
from array import array numbers = array("i", [1, 2, 3, 4, 5]) print(numbers[0]) print(numbers[2:4])
In the first example, we import the array class from the array module. We then create an array called numbers, which stores integers (specified by the "i" argument). The initial values of the array are provided as a list. The entire array is printed using the print() function.
In the second example, we access the elements of the array using indexing. We can access a specific element by specifying its index in square brackets ([]). We can also access a range of elements using slicing, as shown in the example. The selected elements are printed using the print() function.
Related Article: How to Use Python Named Tuples
Working with Sets in Python
Python provides the built-in set type to store a collection of unique elements. Sets are useful for tasks that involve finding unique values or performing set operations, such as union, intersection, and difference.
Example 1: Creating a Set
fruits = {"apple", "banana", "orange"} print(fruits)
Example 2: Set Operations
set1 = {1, 2, 3, 4, 5} set2 = {4, 5, 6, 7, 8} # Union union = set1.union(set2) print(union) # Intersection intersection = set1.intersection(set2) print(intersection) # Difference difference = set1.difference(set2) print(difference)
In the first example, we create a set called fruits that stores the names of various fruits. The entire set is printed using the print() function.
In the second example, we perform set operations on two sets: set1 and set2. The union() method returns a new set that contains all the unique elements from both sets. The intersection() method returns a new set that contains the common elements between the two sets. The difference() method returns a new set that contains the elements from set1 that are not present in set2. The results of each operation are printed using the print() function.
Advanced Python Data Types
In addition to the basic data types covered earlier, Python also provides several advanced data types that are commonly used in more complex scenarios. These advanced data types include:
1. Deque: A double-ended queue that supports adding and removing elements from both ends efficiently.
2. NamedTuple: A subclass of tuples with named fields, allowing for more readable and self-explanatory code.
3. Counter: A dictionary subclass for counting hashable objects. It provides easy and efficient counting of elements.
4. OrderedDict: A dictionary subclass that remembers the order in which items were added. Iterating over it returns items in the order they were added.
5. DefaultDict: A dictionary subclass that provides a default value for missing keys. It simplifies handling missing keys and avoids KeyError exceptions.
6. Heap: A data structure that maintains a heap order. It allows efficient insertion, deletion, and retrieval of the smallest (or largest) element.
These advanced data types offer additional functionality and flexibility compared to the basic data types, and they are often used in more complex data manipulation tasks.
Code Snippet 1: Advanced Example
from collections import deque # Creating a deque queue = deque() # Adding elements queue.append(1) queue.append(2) queue.append(3) # Removing elements print(queue.popleft()) # Output: 1 print(queue.popleft()) # Output: 2
This code snippet demonstrates the usage of the deque data type from the collections module. Deques are useful for implementing queues efficiently. In this example, we create a deque called queue and add elements to it using the append() method. We then remove elements from the left side of the deque using the popleft() method. The removed elements are printed using the print() function.
Code Snippet 2: Advanced Example
from collections import namedtuple # Creating a named tuple Point = namedtuple("Point", ["x", "y"]) # Creating an instance p = Point(3, 5) # Accessing fields print(p.x) # Output: 3 print(p.y) # Output: 5
This code snippet demonstrates the usage of named tuples from the collections module. Named tuples are useful for creating more readable and self-explanatory code. In this example, we define a named tuple called Point with two fields: x and y. We then create an instance of the named tuple and access its fields using dot notation. The field values are printed using the print() function.
Related Article: Implementing a cURL command in Python
Error Handling in Python Data Types
Error handling is an important aspect of programming, including when working with data types in Python. Python provides several mechanisms for handling errors, such as exception handling and error codes.
Example: Exception Handling
try: result = 10 / 0 except ZeroDivisionError: print("Error: Division by zero")
In this example, we use a try-except block to handle the ZeroDivisionError exception that occurs when dividing a number by zero. The code inside the try block is executed, and if an exception occurs, the code inside the except block is executed. In this case, the except block prints an error message.
Use Cases for Python Data Types
Python data types are used in a wide range of scenarios, including:
1. Storing and manipulating numeric data, such as integers and floats.
2. Managing collections of items, such as lists, tuples, dictionaries, and sets.
3. Working with dates and times, using the datetime module.
4. Creating custom data structures using classes and objects.
5. Handling errors and exceptions in a structured manner.
6. Performing advanced data manipulation tasks using specialized data types.
These are just a few examples of the many use cases for Python data types. The versatility and flexibility of Python's data types make them suitable for a wide range of programming tasks.
Best Practices for Python Data Types
When working with Python data types, it is important to follow some best practices to ensure clean and efficient code:
1. Use meaningful variable and function names to improve code readability.
2. Choose the appropriate data type for the task at hand to ensure efficient memory usage and performance.
3. Use built-in functions and methods whenever possible to avoid reinventing the wheel.
4. Avoid using global variables whenever possible to prevent naming conflicts and improve code maintainability.
5. Handle errors and exceptions gracefully to provide informative error messages and prevent crashes.
6. Document your code using comments and docstrings to make it easier for others (and yourself) to understand and maintain.
Real World Examples of Python Data Types
Python data types are used in various real-world applications, including:
1. Web development: Python data types are used to store and manipulate data in web applications, such as user information, product details, and database records.
2. Data analysis: Python data types are used in data analysis tasks, such as storing and processing large datasets, performing statistical calculations, and visualizing data.
3. Machine learning: Python data types are used in machine learning algorithms to store and process training data, model parameters, and prediction results.
4. Scientific computing: Python data types are used in scientific computing applications, such as numerical simulations, data visualization, and data processing.
5. Game development: Python data types are used to store game state, player information, and game assets in game development projects.
These are just a few examples of how Python data types are used in real-world scenarios. Python's versatility and wide range of data types make it a popular choice for various domains and industries.
Related Article: How To Fix The 'No Module Named Pip' Error
Performance Considerations for Python Data Types
While Python's built-in data types are convenient to use, they may not always offer the best performance in certain scenarios. Here are some performance considerations to keep in mind when working with Python data types:
1. Lists vs. arrays: If you need to perform numerical computations on large datasets, consider using arrays from the NumPy library instead of lists. Arrays offer better performance and memory efficiency for numerical computations.
2. Dict vs. namedtuple: If you need to store a large number of objects with named fields, consider using namedtuples instead of dictionaries. Namedtuples are more memory-efficient and offer faster attribute access.
3. String concatenation: When concatenating multiple strings, it is more efficient to use the join() method instead of the + operator. The join() method avoids creating unnecessary intermediate string objects.
4. Set operations: Performing set operations (union, intersection, difference) on large sets can be computationally expensive. Consider using the set data type from the built-in collections module for efficient set operations.
5. Data serialization: When working with large datasets, consider using efficient data serialization formats, such as Protocol Buffers or Apache Avro, to reduce storage and transfer overhead.
Advanced Techniques for Python Data Types
In addition to the basic usage of Python data types, there are several advanced techniques that can be applied to enhance their functionality:
1. Comprehensions: Python comprehensions allow concise creation of lists, dictionaries, and sets based on existing sequences or iterables.
- List comprehension example: [x for x in range(10) if x % 2 == 0]
- Dictionary comprehension example: {x: x**2 for x in range(5)}
- Set comprehension example: {x for x in range(10) if x % 2 == 0}
2. Generators: Generators are functions that can be paused and resumed, allowing for efficient memory usage when working with large datasets or infinite sequences.
- Generator function example: def fibonacci(): yield 0; yield 1; a, b = 0, 1; while True: a, b = b, a + b; yield b
- Generator expression example: (x for x in range(10) if x % 2 == 0)
3. Context managers: Context managers provide a way to manage resources (such as files or database connections) by automatically allocating and releasing them when needed.
- Context manager example using the with
statement: with open("file.txt") as f: print(f.read())
4. Decorators: Decorators allow modifying the behavior of functions or classes without changing their source code. They are often used for adding additional functionality or applying cross-cutting concerns.
- Decorator example: def logger(func): def wrapper(*args, **kwargs): print("Calling function:", func.__name__); return func(*args, **kwargs); return wrapper
- Usage of decorator: @logger def add(a, b): return a + b
These advanced techniques can greatly enhance the functionality and expressiveness of Python data types, allowing for more concise and efficient code.