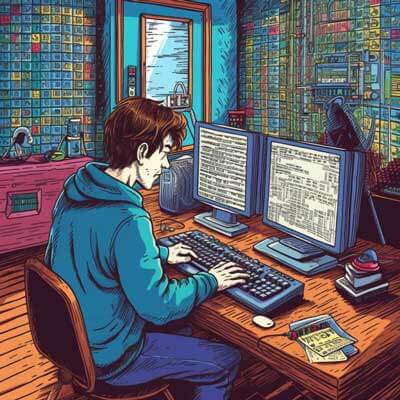
- Introduction to Operators
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Assignment Operators
- Bitwise Operators
- Identity Operators
- Membership Operators
- Operator Precedence
- Use Case: Using Arithmetic Operators
- Use Case: Using Comparison Operators
- Use Case: Using Logical Operators
- Use Case 1: Checking if a Year is a Leap Year
- Use Case 2: Validating User Credentials
- Use Case: Using Assignment Operators
- Use Case 1: Calculating Simple Interest
- Use Case 2: Updating a Shopping Cart Total
- Use Case: Using Bitwise Operators
- Use Case 1: Checking if a Number is Even or Odd
- Use Case 2: Flipping the Case of a String
- Use Case: Using Identity Operators
- Use Case: Checking if a Number is Positive
- Use Case: Using Membership Operators
- Use Case: Checking if a Username is Available
- Best Practice: Using Operator Precedence
- Real World Example: Arithmetic Operators in a Calculator Application
- Real World Example: Logical Operators in a User Authentication System
- Performance Consideration: Efficiency of Different Operators
- Advanced Technique: Implementing Custom Operators
- Error Handling: Common Operator Errors
- Code Snippet: Performing Calculations with Arithmetic Operators
- Code Snippet: User Verification with Logical Operators
- Code Snippet: Assigning Values with Assignment Operators
- Code Snippet: Bit Manipulation with Bitwise Operators
- Code Snippet: Checking Object Identity with Identity Operators
- Code Snippet: Checking Membership with Membership Operators
Introduction to Operators
Operators are special symbols or keywords in Python that perform various operations on one or more operands. These operands can be variables, constants, or expressions. Python provides a wide range of operators that can be used to manipulate data and control program flow.
Related Article: How To Limit Floats To Two Decimal Points In Python
Arithmetic Operators
Arithmetic operators are used to perform mathematical operations such as addition, subtraction, multiplication, division, modulus, and exponentiation. Here are some examples:
# Addition result = 10 + 5 print(result) # Output: 15 # Subtraction result = 10 - 5 print(result) # Output: 5 # Multiplication result = 10 * 5 print(result) # Output: 50 # Division result = 10 / 5 print(result) # Output: 2.0 # Modulus result = 10 % 3 print(result) # Output: 1 # Exponentiation result = 10 ** 2 print(result) # Output: 100
Arithmetic operators can be used with different data types, including integers, floating-point numbers, and complex numbers.
Comparison Operators
Comparison operators are used to compare the values of two operands and return a Boolean value (True or False). These operators are commonly used in conditional statements and loops. Here are some examples:
# Equal to result = 10 == 5 print(result) # Output: False # Not equal to result = 10 != 5 print(result) # Output: True # Greater than result = 10 > 5 print(result) # Output: True # Less than result = 10 = 5 print(result) # Output: True # Less than or equal to result = 10 <= 5 print(result) # Output: False
Comparison operators can be used with various data types, including numbers, strings, and objects.
Logical Operators
Logical operators are used to combine multiple conditions and evaluate the overall result. These operators are typically used in conditional statements to make decisions based on multiple conditions. Here are the three logical operators in Python:
– and
: Returns True if both conditions are True.
– or
: Returns True if at least one of the conditions is True.
– not
: Returns the opposite of the condition.
# Logical AND result = (10 > 5) and (5 5) or (5 5) print(result) # Output: False
Logical operators can be used with Boolean values or expressions that evaluate to Boolean values.
Related Article: How To Rename A File With Python
Assignment Operators
Assignment operators are used to assign values to variables. They combine the assignment operation with another operation, such as addition or multiplication. Here are some examples:
# Simple assignment x = 10 print(x) # Output: 10 # Add and assign x += 5 print(x) # Output: 15 # Subtract and assign x -= 3 print(x) # Output: 12 # Multiply and assign x *= 2 print(x) # Output: 24 # Divide and assign x /= 3 print(x) # Output: 8.0 # Modulus and assign x %= 5 print(x) # Output: 3.0 # Exponentiation and assign x **= 2 print(x) # Output: 9.0
Assignment operators can be used with any data type, including numbers, strings, and objects.
Bitwise Operators
Bitwise operators are used to perform operations on individual bits of binary numbers. They are often used in low-level programming and for manipulating binary data. Here are the bitwise operators available in Python:
– &
: Bitwise AND
– |
: Bitwise OR
– ^
: Bitwise XOR
– ~
: Bitwise NOT
– <>
: Bitwise right shift
# Bitwise AND result = 10 & 5 print(result) # Output: 0 # Bitwise OR result = 10 | 5 print(result) # Output: 15 # Bitwise XOR result = 10 ^ 5 print(result) # Output: 15 # Bitwise NOT result = ~10 print(result) # Output: -11 # Bitwise left shift result = 10 <> 2 print(result) # Output: 2
Bitwise operators are used with integer values and perform operations at the binary level.
Identity Operators
Identity operators are used to compare the memory addresses of two objects. They are typically used to check if two variables refer to the same object. Here are the two identity operators in Python:
– is
: Returns True if the operands refer to the same object.
– is not
: Returns True if the operands refer to different objects.
# Identity check x = [1, 2, 3] y = x result = x is y print(result) # Output: True # Identity check (different objects) x = [1, 2, 3] y = [1, 2, 3] result = x is y print(result) # Output: False # Non-identity check x = [1, 2, 3] y = [1, 2, 3] result = x is not y print(result) # Output: True
Identity operators are typically used with objects and can help in checking object references.
Related Article: How To Check If List Is Empty In Python
Membership Operators
Membership operators are used to check if a value or an object is present in a sequence, such as a list, tuple, or string. They are often used in conditional statements and loops. Here are the two membership operators in Python:
– in
: Returns True if the value is found in the sequence.
– not in
: Returns True if the value is not found in the sequence.
# Membership check my_list = [1, 2, 3, 4, 5] result = 3 in my_list print(result) # Output: True # Membership check (not found) my_list = [1, 2, 3, 4, 5] result = 6 in my_list print(result) # Output: False # Non-membership check my_list = [1, 2, 3, 4, 5] result = 6 not in my_list print(result) # Output: True
Membership operators are commonly used with sequences and can simplify the process of searching for values.
Operator Precedence
Operator precedence determines the order in which operators are evaluated in an expression. It helps in avoiding ambiguity and ensuring that expressions are evaluated correctly. Here is the general order of precedence for the operators discussed so far (from highest to lowest):
1. Parentheses: ()
2. Exponentiation: **
3. Bitwise NOT: ~
4. Multiplication, Division, Modulus: *
, /
, %
5. Addition, Subtraction: +
, -
6. Bitwise Shifts: <>
7. Bitwise AND: &
8. Bitwise XOR: ^
9. Bitwise OR: |
10. Comparison Operators: ==
, !=
, >
, =
, <=
11. Logical NOT: not
12. Logical AND: and
13. Logical OR: or
14. Identity Operators: is
, is not
15. Membership Operators: in
, not in
16. Assignment Operators: =
, +=
, -=
, *=
, /=
, %=
, **=
, &=
, |=
, ^=
, <>=
Use Case: Using Arithmetic Operators
Arithmetic operators are frequently used in mathematical calculations and manipulating numerical data. Here are two use cases that demonstrate the usage of arithmetic operators:
Use Case 1: Calculating the Area of a Rectangle
# User inputs the length and width of a rectangle length = float(input("Enter the length of the rectangle: ")) width = float(input("Enter the width of the rectangle: ")) # Calculate the area using the formula: length * width area = length * width # Display the result print("The area of the rectangle is:", area)
Use Case 2: Converting Temperature from Celsius to Fahrenheit
# User inputs the temperature in Celsius celsius = float(input("Enter the temperature in Celsius: ")) # Convert Celsius to Fahrenheit using the formula: (Celsius * 9/5) + 32 fahrenheit = (celsius * 9/5) + 32 # Display the result print("The temperature in Fahrenheit is:", fahrenheit)
These use cases showcase how arithmetic operators can be used to perform calculations in real-world scenarios.
Related Article: How To Check If a File Exists In Python
Use Case: Using Comparison Operators
Comparison operators are commonly used in conditional statements and loops to make decisions based on the comparison of values. Here are two use cases that demonstrate the usage of comparison operators:
Use Case 1: Checking if a Number is Positive, Negative, or Zero
# User inputs a number number = float(input("Enter a number: ")) # Check if the number is positive, negative, or zero if number > 0: print("The number is positive.") elif number < 0: print("The number is negative.") else: print("The number is zero.")
Use Case 2: Finding the Maximum of Three Numbers
# User inputs three numbers num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) num3 = float(input("Enter the third number: ")) # Find the maximum using comparison operators if num1 >= num2 and num1 >= num3: max_num = num1 elif num2 >= num1 and num2 >= num3: max_num = num2 else: max_num = num3 # Display the result print("The maximum number is:", max_num)
These use cases demonstrate how comparison operators can be used to make decisions and perform comparisons in real-world scenarios.
Use Case: Using Logical Operators
Logical operators are frequently used to combine multiple conditions and evaluate the overall result. Here are two use cases that demonstrate the usage of logical operators:
Use Case 1: Checking if a Year is a Leap Year
# User inputs a year year = int(input("Enter a year: ")) # Check if the year is a leap year if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0: print("The year is a leap year.") else: print("The year is not a leap year.")
Related Article: How to Use Inline If Statements for Print in Python
Use Case 2: Validating User Credentials
# User inputs their username and password username = input("Enter your username: ") password = input("Enter your password: ") # Check if the username and password are valid if username == "admin" and password == "password": print("Login successful.") else: print("Invalid credentials.")
These use cases illustrate how logical operators can be used to combine conditions and perform logical evaluations in real-world scenarios.
Use Case: Using Assignment Operators
Assignment operators are commonly used to assign values to variables. Here are two use cases that demonstrate the usage of assignment operators:
Use Case 1: Calculating Simple Interest
# User inputs the principal, rate, and time principal = float(input("Enter the principal amount: ")) rate = float(input("Enter the interest rate: ")) time = float(input("Enter the time period (in years): ")) # Calculate the simple interest using the formula: (principal * rate * time) / 100 simple_interest = (principal * rate * time) / 100 # Display the result print("The simple interest is:", simple_interest)
Related Article: How to Use Stripchar on a String in Python
Use Case 2: Updating a Shopping Cart Total
# User adds items to the shopping cart cart_total = 0 item_price = float(input("Enter the price of the first item: ")) cart_total += item_price item_price = float(input("Enter the price of the second item: ")) cart_total += item_price item_price = float(input("Enter the price of the third item: ")) cart_total += item_price # Display the updated cart total print("The updated cart total is:", cart_total)
These use cases showcase how assignment operators can be used to assign and update values in real-world scenarios.
Use Case: Using Bitwise Operators
Bitwise operators are commonly used in low-level programming, networking, and data manipulation. Here are two use cases that demonstrate the usage of bitwise operators:
Use Case 1: Checking if a Number is Even or Odd
# User inputs a number number = int(input("Enter a number: ")) # Check if the number is even or odd using bitwise AND if number & 1: print("The number is odd.") else: print("The number is even.")
Related Article: How To Delete A File Or Folder In Python
Use Case 2: Flipping the Case of a String
# User inputs a string string = input("Enter a string: ") # Flip the case of the string using bitwise XOR flipped_string = "" for char in string: flipped_char = chr(ord(char) ^ 32) flipped_string += flipped_char # Display the flipped string print("The flipped string is:", flipped_string)
These use cases demonstrate how bitwise operators can be used for various operations in real-world scenarios.
Use Case: Using Identity Operators
Identity operators are commonly used to compare object references and check if two variables refer to the same object. Here is a use case that demonstrates the usage of identity operators:
Use Case: Checking if a Number is Positive
# User inputs a number number = float(input("Enter a number: ")) # Check if the number is positive using identity operators if number is 0: print("The number is zero.") elif number > 0: print("The number is positive.") else: print("The number is negative.")
This use case showcases how identity operators can be used to compare object references in real-world scenarios.
Related Article: How To Move A File In Python
Use Case: Using Membership Operators
Membership operators are commonly used to check if a value or an object is present in a sequence. Here is a use case that demonstrates the usage of membership operators:
Use Case: Checking if a Username is Available
# List of existing usernames existing_usernames = ["john", "mary", "alex", "emma"] # User inputs a username username = input("Enter a username: ") # Check if the username is available using membership operators if username in existing_usernames: print("Sorry, the username is already taken.") else: print("Congratulations, the username is available.")
This use case illustrates how membership operators can be used to check for the presence of a value in a sequence.
Best Practice: Using Operator Precedence
Understanding operator precedence is crucial for writing correct and efficient code. It helps in avoiding unexpected results and ensures that expressions are evaluated in the intended order. Here are some best practices for using operator precedence:
– Use parentheses to explicitly specify the order of evaluation, especially when dealing with complex expressions.
– Familiarize yourself with the operator precedence table and refer to it when in doubt.
– Use whitespace and line breaks to improve code readability, especially in expressions with multiple operators.
Here is an example that showcases the importance of operator precedence:
result = 10 + 5 * 2 print(result) # Output: 20
In this example, the multiplication operation is evaluated first due to its higher precedence, resulting in 10 + 10 = 20. If the addition operation was evaluated first, the result would have been 15.
Related Article: How to Implement a Python Foreach Equivalent
Real World Example: Arithmetic Operators in a Calculator Application
Arithmetic operators are commonly used in calculator applications to perform mathematical calculations. Here is a simplified example of a calculator application that utilizes arithmetic operators:
# User inputs two numbers num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) # User selects an operation operation = input("Select an operation (+, -, *, /): ") # Perform the operation based on user input if operation == "+": result = num1 + num2 elif operation == "-": result = num1 - num2 elif operation == "*": result = num1 * num2 elif operation == "/": result = num1 / num2 else: result = "Invalid operation" # Display the result print("The result is:", result)
This example demonstrates how arithmetic operators can be used to perform different calculations based on user input.
Real World Example: Logical Operators in a User Authentication System
Logical operators are commonly used in user authentication systems to evaluate multiple conditions and determine if a user should be granted access. Here is a simplified example of a user authentication system that utilizes logical operators:
# User inputs their username and password username = input("Enter your username: ") password = input("Enter your password: ") # Check if the username and password match the expected values if username == "admin" and password == "password": print("Login successful.") else: print("Invalid credentials.")
In this example, the logical and
operator is used to check if both the username and password match the expected values. If the condition evaluates to true, the user is granted access.
Performance Consideration: Efficiency of Different Operators
While Python provides a wide range of operators for various operations, it’s important to consider the efficiency of different operators, especially in performance-critical scenarios. Here are some general considerations:
– Arithmetic operators (+
, -
, *
, /
) are generally efficient and have a low computational cost.
– Comparison operators (==
, !=
, >
, =
, <=
) are also efficient and have a low computational cost.
– Logical operators (and
, or
, not
) can have short-circuit evaluation, which can improve performance in certain cases.
– Bitwise operators (&
, |
, ^
, ~
, <>
) are efficient for manipulating binary data but may not provide significant performance benefits in non-binary operations.
– Assignment operators (=
, +=
, -=
, *=
, /=
, %=
, **=
, &=
, |=
, ^=
, <>=
) have a negligible performance impact compared to the operation they combine.
– Identity operators (is
, is not
) and membership operators (in
, not in
) have a similar performance cost to comparison operators.
It’s important to note that the performance impact of different operators can vary depending on the specific use case and the scale of the operations being performed. Therefore, it’s recommended to profile and benchmark your code to identify potential bottlenecks and optimize accordingly.
Related Article: How to Use Slicing in Python And Extract a Portion of a List
Advanced Technique: Implementing Custom Operators
Python allows the creation of custom operators by defining special methods in classes. These methods are known as “magic methods” or “dunder methods” and enable the customization of operator behavior. Here is an example of a custom operator implemented using magic methods:
class ComplexNumber: def __init__(self, real, imag): self.real = real self.imag = imag def __add__(self, other): real = self.real + other.real imag = self.imag + other.imag return ComplexNumber(real, imag) def __str__(self): return f"{self.real} + {self.imag}i" # Create complex numbers c1 = ComplexNumber(2, 3) c2 = ComplexNumber(4, 5) # Add two complex numbers using the custom operator result = c1 + c2 # Display the result print(result) # Output: 6 + 8i
In this example, the __add__
method is defined to customize the behavior of the +
operator for the ComplexNumber
class. This allows complex numbers to be added using the +
operator.
Error Handling: Common Operator Errors
While using operators in Python, it’s important to be aware of common errors that can occur and handle them appropriately. Here are some common operator-related errors and how to handle them:
– ZeroDivisionError
: Occurs when dividing by zero. To handle this error, you can use a try-except block to catch the exception and handle it gracefully.
– TypeError
: Occurs when using operators with incompatible data types. To handle this error, you can check the data types before performing the operation or use try-except blocks to catch and handle specific types of errors.
– NameError
: Occurs when using an undefined variable. To handle this error, ensure that all variables are properly defined before using them in expressions.
It’s good practice to anticipate and handle potential errors to prevent program crashes and improve the robustness of your code.
Code Snippet: Performing Calculations with Arithmetic Operators
Here is a code snippet that demonstrates various calculations using arithmetic operators:
# Addition result = 10 + 5 print(result) # Output: 15 # Subtraction result = 10 - 5 print(result) # Output: 5 # Multiplication result = 10 * 5 print(result) # Output: 50 # Division result = 10 / 5 print(result) # Output: 2.0 # Modulus result = 10 % 3 print(result) # Output: 1 # Exponentiation result = 10 ** 2 print(result) # Output: 100
This code snippet showcases how arithmetic operators can be used to perform calculations.
Related Article: How to Check a Variable's Type in Python
Code Snippet: User Verification with Logical Operators
Here is a code snippet that demonstrates user verification using logical operators:
# User inputs their username and password username = input("Enter your username: ") password = input("Enter your password: ") # Check if the username and password match the expected values if username == "admin" and password == "password": print("Login successful.") else: print("Invalid credentials.")
This code snippet illustrates how logical operators can be used to evaluate multiple conditions and make decisions based on them.
Code Snippet: Assigning Values with Assignment Operators
Here is a code snippet that demonstrates assigning values using assignment operators:
# Simple assignment x = 10 print(x) # Output: 10 # Add and assign x += 5 print(x) # Output: 15 # Subtract and assign x -= 3 print(x) # Output: 12 # Multiply and assign x *= 2 print(x) # Output: 24 # Divide and assign x /= 3 print(x) # Output: 8.0 # Modulus and assign x %= 5 print(x) # Output: 3.0 # Exponentiation and assign x **= 2 print(x) # Output: 9.0
This code snippet showcases how assignment operators can be used to assign and update values.
Code Snippet: Bit Manipulation with Bitwise Operators
Here is a code snippet that demonstrates bit manipulation using bitwise operators:
# Bitwise AND result = 10 & 5 print(result) # Output: 0 # Bitwise OR result = 10 | 5 print(result) # Output: 15 # Bitwise XOR result = 10 ^ 5 print(result) # Output: 15 # Bitwise NOT result = ~10 print(result) # Output: -11 # Bitwise left shift result = 10 <> 2 print(result) # Output: 2
This code snippet showcases how bitwise operators can be used for bit manipulation.
Related Article: How to Use Increment and Decrement Operators in Python
Code Snippet: Checking Object Identity with Identity Operators
Here is a code snippet that demonstrates checking object identity using identity operators:
# Identity check x = [1, 2, 3] y = x result = x is y print(result) # Output: True # Identity check (different objects) x = [1, 2, 3] y = [1, 2, 3] result = x is y print(result) # Output: False # Non-identity check x = [1, 2, 3] y = [1, 2, 3] result = x is not y print(result) # Output: True
This code snippet illustrates how identity operators can be used to compare object references.
Code Snippet: Checking Membership with Membership Operators
Here is a code snippet that demonstrates checking membership using membership operators:
# Membership check my_list = [1, 2, 3, 4, 5] result = 3 in my_list print(result) # Output: True # Membership check (not found) my_list = [1, 2, 3, 4, 5] result = 6 in my_list print(result) # Output: False # Non-membership check my_list = [1, 2, 3, 4, 5] result = 6 not in my_list print(result) # Output: True
This code snippet demonstrates how membership operators can be used to check for the presence of a value in a sequence.