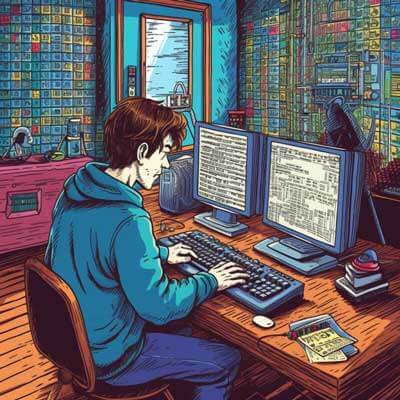
Table of Contents
Getting Started with CSV Files
CSV (Comma-Separated Values) files are a widely used format for storing tabular data. In Python, processing CSV files is made easy with the built-in csv
module. Let's explore how to get started with CSV file handling in Python.
Related Article: How to Send an Email Using Python
What are CSV Files and their Structure?
CSV files consist of rows and columns, where each line represents a row and the values are separated by a delimiter, typically a comma. However, other delimiters like tabs or semicolons can also be used. The first row often contains the column headers.
To begin, we need to import the csv
module in Python:
import csv
Reading CSV Files using Python's csv
Module
To read data from a CSV file, we can use the csv.reader()
function. This function takes a file object and returns an iterable reader object. Each iteration returns a row of the CSV file as a list.
Here's an example of reading a CSV file named "data.csv":
with open("data.csv", "r") as file: csv_reader = csv.reader(file) for row in csv_reader: print(row)
This code opens the "data.csv" file in read mode and iterates over each row, printing it to the console.
Handling Different Delimiters and Quoting Styles
The csv.reader()
function assumes a comma as the default delimiter. However, we can specify a different delimiter by passing the delimiter
parameter. For example, to handle tab-separated values (TSV), we can set the delimiter as follows:
with open("data.tsv", "r") as file: csv_reader = csv.reader(file, delimiter="\t") for row in csv_reader: print(row)
In some cases, values in CSV files may be enclosed in quotes. To handle such cases, we can set the quotechar
parameter. For instance, if values are enclosed in double quotes, we can use:
with open("data.csv", "r") as file: csv_reader = csv.reader(file, quotechar='"') for row in csv_reader: print(row)
Related Article: How To Find Index Of Item In Python List
Dealing with Header Rows and Accessing Column Data
CSV files often have a header row that contains column names. We can skip the header row while reading the file by using the next()
function before iterating over the remaining rows. This allows us to access the data without including the header.
with open("data.csv", "r") as file: csv_reader = csv.reader(file) headers = next(csv_reader) # Skip the header row for row in csv_reader: print(row)
To access specific columns, we can refer to the elements of each row by their index. For example, to access the second column:
with open("data.csv", "r") as file: csv_reader = csv.reader(file) headers = next(csv_reader) for row in csv_reader: print(row[1]) # Print the second column value
This allows us to extract and work with specific columns of interest.
Basic Data Manipulation Techniques
In this section, we will dive into some fundamental data manipulation techniques for working with CSV files in Python. These techniques will help us filter, sort, aggregate, and transform data within the CSV file.
Filtering and Selecting Specific Rows
To filter and select specific rows based on certain conditions, we can use conditional statements and loop through the rows of the CSV file.
For example, let's say we have a CSV file with student data, and we want to select only the rows where the student's age is above 18:
with open("data.csv", "r") as file: csv_reader = csv.reader(file) headers = next(csv_reader) for row in csv_reader: age = int(row[2]) # Assuming age is in the third column if age > 18: print(row)
By applying a condition within the loop, we can filter out the rows that don't meet the criteria.
Sorting CSV Data based on Columns
To sort CSV data based on specific columns, we can utilize the sorted()
function and provide a key argument that specifies the column to sort on. The key
argument can be a lambda function that extracts the desired column value.
Here's an example of sorting a CSV file based on a numeric column in ascending order:
with open("data.csv", "r") as file: csv_reader = csv.reader(file) headers = next(csv_reader) sorted_rows = sorted(csv_reader, key=lambda row: int(row[3])) # Sort based on fourth column for row in sorted_rows: print(row)
In this code snippet, we sort the rows based on the fourth column (assuming it contains numeric values) using a lambda function as the key.
Related Article: Build a Chat Web App with Flask, MongoDB, Reactjs & Docker
Aggregating and Summarizing Data using pandas
For more advanced data manipulation tasks, we can leverage the pandas
library, which provides powerful tools for working with structured data, including CSV files.
To aggregate and summarize data in a CSV file using pandas
, we need to install the library first:
pip install pandas
Once pandas
is installed, we can import it and load the CSV data into a DataFrame for further processing:
import pandas as pd df = pd.read_csv("data.csv")
Now, we can utilize pandas
functions to perform various operations on the DataFrame. For example, to calculate the average age of students:
average_age = df["Age"].mean() print(average_age)
The above code calculates the mean value of the "Age" column in the DataFrame.
Applying Transformations and Calculations on CSV Columns
To apply transformations and perform calculations on CSV columns, we can directly operate on the DataFrame using pandas
.
For instance, let's say we have a CSV file with a "Price" column, and we want to calculate the total price after applying a discount of 10% to each value:
df["Price"] = df["Price"] * 0.9
In this example, we multiply the "Price" column by 0.9 to apply the discount.
Advanced CSV Processing Techniques
In this section, we'll delve into advanced CSV processing techniques that go beyond basic manipulation. We'll explore joining and merging CSV files, handling missing data, working with large CSV files, and optimizing CSV processing performance.
Joining and Merging Multiple CSV Files
When working with multiple CSV files, it's often necessary to combine their data based on common keys. We can achieve this by joining or merging the CSV files.
To join CSV files, we can use the pandas
library. Let's consider two CSV files, "file1.csv" and "file2.csv," both containing a "ProductID" column. We can perform an inner join to merge the data based on the shared "ProductID" values:
import pandas as pd df1 = pd.read_csv("file1.csv") df2 = pd.read_csv("file2.csv") merged_df = pd.merge(df1, df2, on="ProductID", how="inner")
The resulting merged_df
DataFrame will contain the rows that have matching "ProductID" values in both files.
Related Article: How to Convert Bytes to a String in Python 3
Handling Missing Data and Data Imputation
Real-world CSV data may contain missing values, represented by NaN (Not a Number) or other markers. To handle missing data, we can use various techniques such as dropping rows with missing values or imputing the missing values with appropriate replacements.
To drop rows with missing values, we can use the dropna()
function in pandas
:
df.dropna(inplace=True)
This will remove any rows containing missing values from the DataFrame.
To impute missing values, we can use methods such as mean imputation or forward/backward filling. Here's an example of forward filling missing values:
df.fillna(method="ffill", inplace=True)
This fills missing values with the previous non-missing value in the DataFrame.
Working with Large CSV Files using Chunking and Streaming
Large CSV files can exceed available memory, making it challenging to process them as a whole. To handle large CSV files, we can use chunking and streaming techniques.
pandas
allows us to read CSV files in chunks, enabling us to process one chunk at a time. We can specify the chunk size using the chunksize
parameter:
chunk_size = 10000 for chunk in pd.read_csv("large_file.csv", chunksize=chunk_size): # Process each chunk # Example: calculate statistics, perform transformations, etc. print(chunk.head())
This code reads the "large_file.csv" in chunks of 10,000 rows and processes each chunk separately.
Streaming libraries like csv
provide an alternative for processing large CSV files without loading the entire file into memory. We can process the file row by row, which is memory-efficient. Here's an example:
import csv with open("large_file.csv", "r") as file: csv_reader = csv.reader(file) for row in csv_reader: # Process each row # Example: perform calculations, extract data, etc. print(row)
Optimizing CSV Processing Performance with Parallelization
To improve the performance of CSV processing, we can leverage parallelization. Parallel processing allows us to distribute the workload across multiple cores or processes, significantly reducing the processing time.
The multiprocessing
module in Python provides tools for parallel execution. We can divide the CSV data into chunks and process them simultaneously using multiple processes.
Here's a simplified example of parallelizing CSV processing using multiprocessing
import csv from multiprocessing import Pool def process_row(row): # Process each row # Example: perform calculations, extract data, etc. print(row) with open("data.csv", "r") as file: csv_reader = csv.reader(file) # Define the number of processes to use num_processes = 4 with Pool(num_processes) as pool: # Map the process_row function to each row in parallel pool.map(process_row, csv_reader)
In this example, we use the Pool
class from the multiprocessing
module to create a pool of processes. We specify the number of processes to use (in this case, 4). The pool.map()
function maps the process_row
function to each row in parallel, distributing the workload across the available processes.
Real-World Examples
In this section, we'll explore some real-world examples that demonstrate the practical application of processing CSV files in Python. These examples will showcase how CSV file handling can be used in data analysis, data cleaning, and data integration tasks.
Related Article: Building Flask Web Apps: Advanced Features
Example 1: Data Analysis and Visualization
CSV files are commonly used for data analysis and visualization tasks. Let's consider an example where we have a CSV file containing sales data for different products. We can use Python libraries like pandas
and matplotlib
to analyze and visualize the data.
First, we read the CSV file and load it into a DataFrame:
import pandas as pd df = pd.read_csv("sales_data.csv")
Next, we can perform various analysis tasks on the data, such as calculating total sales, average prices, or identifying the top-selling products:
total_sales = df["Quantity"].sum() average_price = df["Price"].mean() top_selling_products = df.groupby("Product")["Quantity"].sum().nlargest(5)
We can also create visualizations to gain insights from the data. For example, we can plot a bar chart of product sales:
import matplotlib.pyplot as plt product_sales = df.groupby("Product")["Quantity"].sum() product_sales.plot(kind="bar") plt.xlabel("Product") plt.ylabel("Quantity Sold") plt.title("Product Sales") plt.show()
These analysis and visualization techniques allow us to explore and understand the data from the CSV file effectively.
Example 2: Data Cleaning and Preprocessing
CSV files often contain inconsistencies, missing values, or incorrect formats. Python can be used to clean and preprocess such data for further analysis. Let's consider an example where we have a CSV file with customer data that needs cleaning.
We can use pandas
to read the CSV file and identify missing values:
import pandas as pd df = pd.read_csv("customer_data.csv") missing_values = df.isnull().sum()
The isnull()
function identifies missing values, and the sum()
function calculates the total number of missing values in each column.
To handle missing values, we can choose to drop the rows or columns with missing data, or we can impute the missing values with appropriate replacements:
# Drop rows with missing values df.dropna(inplace=True) # Impute missing values with the mean df["Age"].fillna(df["Age"].mean(), inplace=True)
These cleaning techniques ensure that the data is in a consistent and usable format.
Example 3: Data Integration and Transformation
CSV files are often used for data integration tasks, where data from multiple sources needs to be combined or transformed. Let's consider an example where we have two CSV files, one containing customer information and the other containing purchase history.
We can merge the two CSV files based on a common key, such as the customer ID, to create a consolidated dataset:
import pandas as pd customer_df = pd.read_csv("customer_data.csv") purchase_df = pd.read_csv("purchase_history.csv") merged_df = pd.merge(customer_df, purchase_df, on="CustomerID", how="inner")
The resulting merged_df
DataFrame will contain the combined data from both CSV files based on the matching customer IDs.
We can also perform transformations on the data, such as calculating total purchase amounts, aggregating data by customer, or creating new derived columns:
total_purchase_amounts = merged_df.groupby("CustomerID")["Amount"].sum() customer_agg = merged_df.groupby("CustomerID").agg({"TotalPurchaseAmount": ("Amount", "sum"), "AveragePurchaseAmount": ("Amount", "mean"), "PurchaseCount": ("Amount", "count")})
These transformations allow us to integrate and transform the data from different CSV files into a consolidated and meaningful format.
Example 4: Exporting Processed Data to CSV
After processing and analyzing CSV data, it's often useful to export the results back to a CSV file for further use or sharing. Python provides straightforward methods to export data from DataFrames to CSV files.
For instance, let's say we have performed data analysis and derived some insights from a DataFrame. We can export the results to a new CSV file as follows:
import pandas as pd # Perform data analysis and obtain results in a DataFrame results_df = ... # Export results to a CSV file results_df.to_csv("analysis_results.csv", index=False)
The to_csv()
function allows us to save the DataFrame as a CSV file. The index=False
parameter ensures that the index column is not included in the exported CSV file.
Related Article: Working with Linked Lists in Python
Error Handling and Data Validation
When processing CSV files in Python, it's crucial to have robust error handling and data validation mechanisms in place. This section will explore techniques for handling exceptions, ensuring data integrity, and implementing error logging and reporting mechanisms.
Handling Exceptions during CSV File Processing
During CSV file processing, various exceptions can occur, such as file not found errors, incorrect file formats, or issues with data parsing. It's essential to handle these exceptions gracefully to prevent program crashes and provide informative error messages to users.
One way to handle exceptions is by using the try-except
block. For example, consider the following code snippet that reads a CSV file and performs some operations on the data:
import csv try: with open("data.csv", "r") as file: csv_reader = csv.reader(file) # Perform operations on the CSV data ... except FileNotFoundError: print("File not found. Please provide a valid CSV file.") except csv.Error as e: print("CSV file error:", e)
In this example, we use the try-except
block to catch specific exceptions. If a FileNotFoundError
occurs, we display a user-friendly error message. If a csv.Error
occurs, we print the specific error message provided by the csv
module.
By handling exceptions effectively, we can anticipate and address potential issues during CSV file processing.
Validating Data Integrity and Ensuring Data Quality
Data integrity and quality are crucial aspects of CSV file processing. It's important to validate the data and ensure that it meets specific criteria or constraints before further processing.
One common approach is to perform data validation using conditional statements or regular expressions. For example, if we have a CSV file containing customer information, we can validate that the email addresses are in the correct format:
import csv import re with open("customer_data.csv", "r") as file: csv_reader = csv.DictReader(file) for row in csv_reader: email = row["Email"] if not re.match(r"[^@]+@[^@]+\.[^@]+", email): print("Invalid email format:", email)
In this example, we use regular expressions to validate the email addresses. Any invalid email addresses are flagged and displayed as error messages.
By implementing data validation techniques, we can ensure the integrity and quality of the data being processed.
Implementing Error Logging and Reporting Mechanisms
To effectively manage errors and troubleshoot issues during CSV file processing, it's essential to implement error logging and reporting mechanisms. These mechanisms help capture and document errors, making it easier to identify and resolve issues.
One way to implement error logging is by using Python's built-in logging
module. Here's an example of how we can log errors to a file:
import logging logging.basicConfig(filename="error.log", level=logging.ERROR) try: # CSV file processing code ... except Exception as e: logging.error("An error occurred: %s", str(e))
In this example, we configure the logging module to write error messages to a file named "error.log" at the ERROR
level. When an exception occurs during CSV file processing, we log the error message along with additional details.
Additionally, you can consider implementing a reporting mechanism to notify users or system administrators about errors. This can be done through email notifications, system alerts, or other communication channels.
By implementing error logging and reporting mechanisms, you can track and address errors efficiently, leading to more reliable and robust CSV file processing.