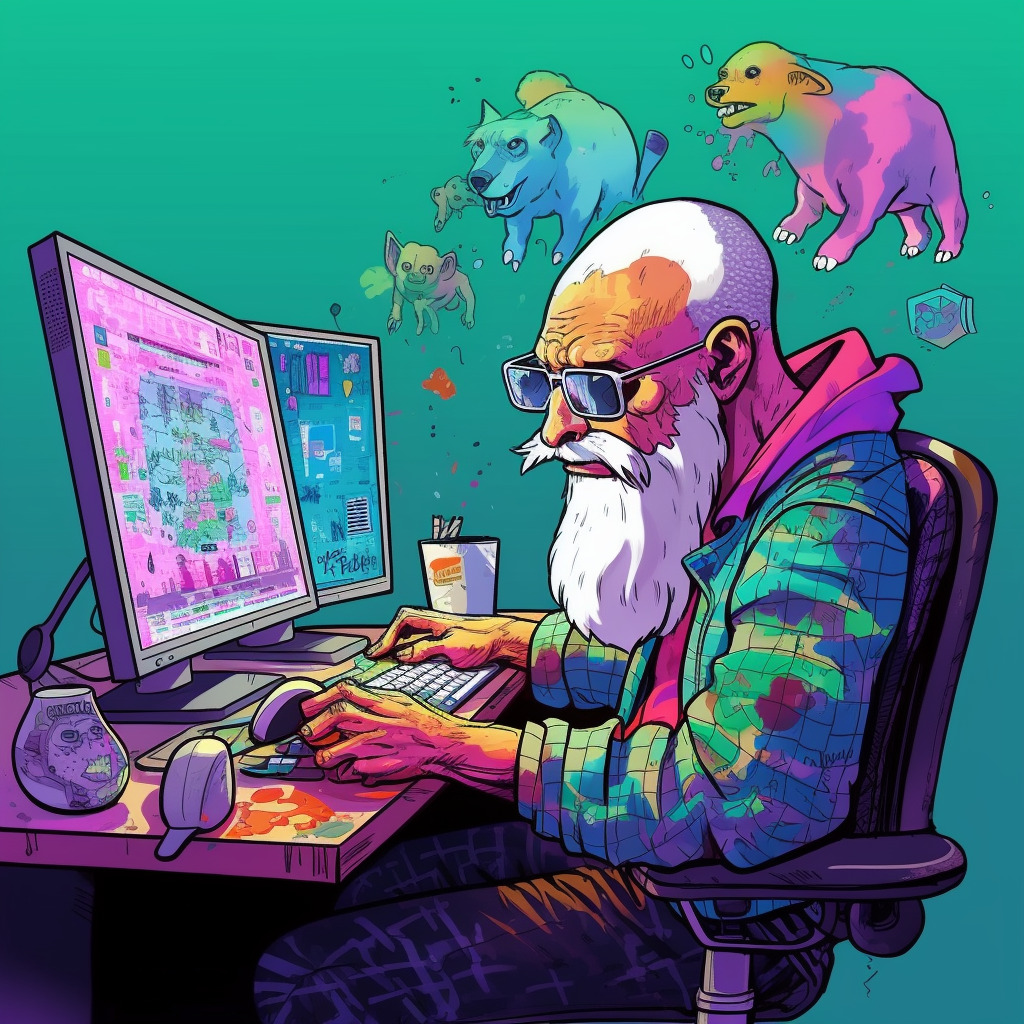
- Understanding Strings in Python
- Creating Strings
- Accessing Characters in a String
- Slicing Strings
- String Concatenation
- String Length
- String Methods
- String Contains
- Checking if a String Contains a Substring
- Using the in Operator
- Using the index() Method
- Ignoring Case Sensitivity
- Extracting Substrings
- Using Slicing to Extract Substrings
- Using Regular Expressions
- Matching Patterns
- Extracting Matches
- Replacing Matches
- Modifiers and Special Characters
- Replacing Substrings
- Replacing All Occurrences
- Replacing Case Insensitive
- Splitting a String
- Splitting at a Specific Character
- Splitting with Regular Expressions
- Joining Strings
- Using the + Operator
- Using the join() Method
- Joining Strings with Other Data Types
- Joining with a Specific Separator
- Joining with a Custom Separator
- Converting Strings to Upper or Lower Case
- Removing Leading and Trailing Whitespace
- 1. Using the strip() method
- 2. Using the lstrip() method
- 3. Using the rstrip() method
- Removing Leading and Trailing Characters
- 1. Using the strip() method
- 2. Using the lstrip() and rstrip() methods
- 3. Using regular expressions
- Checking if a String Starts or Ends with a Substring
- Counting Occurrences of a Substring
- Checking if a String Contains Only Digits
- Using the isdigit() Method
- Using Regular Expressions
- Using a Custom Function
- Checking if a String Contains Only Letters
- Checking if a String Contains Only Alphanumeric Characters
- Formatting Strings
- 1. Using the {{EJS402}} method
- 2. Using f-strings
- 3. Using the {{EJS407}} operator
- Using f-strings
- Using the format() Method
- Using String Interpolation
- Escaping Characters in Strings
- Using Raw Strings
Understanding Strings in Python
In Python, a string is a sequence of characters enclosed in single quotes (”) or double quotes (“”). Strings are one of the most commonly used data types in Python and are used to represent text.
Related Article: How To Limit Floats To Two Decimal Points In Python
Creating Strings
To create a string in Python, simply enclose the characters within quotes. Here are a few examples:
string1 = 'Hello, World!' string2 = "Python is awesome"
Accessing Characters in a String
You can access individual characters in a string by using indexing. Indexing starts at 0. For example, to access the first character of a string, you would use index 0.
string = "Hello, World!" print(string[0]) # Output: H
Slicing Strings
Slicing allows you to extract a portion of a string. You can specify a range of indices to extract a substring. The syntax for slicing is string[start:end]
, where start
is the index where the slice starts and end
is the index where the slice ends.
string = "Hello, World!" print(string[7:12]) # Output: World
Related Article: How To Rename A File With Python
String Concatenation
Python provides the +
operator to concatenate strings. You can concatenate two or more strings together to create a new string.
string1 = "Hello" string2 = "World" result = string1 + " " + string2 print(result) # Output: Hello World
String Length
To find the length of a string, you can use the built-in len()
function. It returns the number of characters in the string.
string = "Hello, World!" print(len(string)) # Output: 13
String Methods
Python provides numerous built-in methods to manipulate strings. Some commonly used string methods include:
– lower()
: Converts all characters in a string to lowercase.
– upper()
: Converts all characters in a string to uppercase.
– strip()
: Removes leading and trailing whitespace from a string.
– replace()
: Replaces a specified substring with another substring.
string = " Hello, World! " print(string.lower()) # Output: hello, world! print(string.upper()) # Output: HELLO, WORLD! print(string.strip()) # Output: Hello, World! print(string.replace("Hello", "Hi")) # Output: Hi, World!
Related Article: How To Check If List Is Empty In Python
String Contains
To check if a string contains a specific substring, you can use the in
keyword. It returns True
if the substring is found, and False
otherwise.
string = "Hello, World!" print("Hello" in string) # Output: True print("Python" in string) # Output: False
Now that you have a basic understanding of strings in Python, you can start using them in your programs to manipulate and process text data.
Checking if a String Contains a Substring
You can easily check if a string contains a specific substring using the in
keyword. The in
keyword is used to check if a value exists in a sequence, such as a string.
Here’s a simple example that demonstrates how to use the in
keyword to check if a string contains a specific substring:
string = "Hello, World!" if "Hello" in string: print("Substring found!") else: print("Substring not found!")
In this example, we have a string variable string
that contains the value “Hello, World!”. We use the in
keyword to check if the substring “Hello” exists in the string
variable. If the substring is found, we print “Substring found!”. Otherwise, we print “Substring not found!”.
You can also use the in
keyword to check if a string contains multiple substrings. Here’s an example:
string = "The quick brown fox jumps over the lazy dog." if "fox" in string and "dog" in string: print("Both substrings found!") elif "fox" in string: print("Only 'fox' substring found!") elif "dog" in string: print("Only 'dog' substring found!") else: print("Neither substring found!")
In this example, we check if the string contains both the substrings “fox” and “dog”. If both substrings are found, we print “Both substrings found!”. If only one of the substrings is found, we print the corresponding message. If neither substring is found, we print “Neither substring found!”.
Sometimes, you may want to perform a case-insensitive check for substring existence. Python provides a lower()
method that converts a string to lowercase, allowing you to perform a case-insensitive check. Here’s an example:
string = "Hello, World!" if "hello" in string.lower(): print("Case-insensitive substring found!") else: print("Case-insensitive substring not found!")
In this example, we convert the string
variable to lowercase using the lower()
method before checking if the substring “hello” exists. This allows us to perform a case-insensitive check.
Now that you know how to check if a string contains a substring in Python, you can use this knowledge to perform various string manipulations and validations in your programs.
Using the in Operator
The in
operator is a powerful tool in Python for checking if a string contains a specific substring. It returns True
if the substring is found, and False
otherwise.
Here’s a simple example:
sentence = "The quick brown fox jumps over the lazy dog" if "fox" in sentence: print("The string contains 'fox'") else: print("The string does not contain 'fox'")
Output:
The string contains 'fox'
In the above code snippet, we check if the string variable sentence
contains the substring “fox”. Since it does, the condition is evaluated as True
and the message “The string contains ‘fox'” is printed.
We can also use the in
operator with variables:
substring = "quick" sentence = "The quick brown fox jumps over the lazy dog" if substring in sentence: print("The string contains 'quick'") else: print("The string does not contain 'quick'")
Output:
The string contains 'quick'
In this example, we assign the substring “quick” to the variable substring
and then check if it is present in the string variable sentence
.
The in
operator can be used to check for the presence of multiple substrings as well. We can use it in conjunction with the and
or or
operators to combine multiple conditions.
sentence = "The quick brown fox jumps over the lazy dog" if "fox" in sentence and "dog" in sentence: print("The string contains both 'fox' and 'dog'") elif "fox" in sentence or "cat" in sentence: print("The string contains either 'fox' or 'cat'") else: print("The string does not contain 'fox' or 'dog'")
Output:
The string contains both 'fox' and 'dog'
In this example, we check if the string variable sentence
contains both the substrings “fox” and “dog”. Since it does, the first condition is evaluated as True
and the message “The string contains both ‘fox’ and ‘dog'” is printed.
As you can see, the in
operator provides a simple and efficient way to check if a string contains a specific substring. It is a valuable tool for string manipulations in Python.
Continue reading to learn more about other useful string manipulation techniques in Python.
Using the find() Method
The find()
method is used to search for a specified substring within a string. It returns the index of the first occurrence of the substring, or -1 if the substring is not found.
Here’s the syntax for using the find()
method:
string.find(substring, start, end)
– string
is the string in which you want to search for the substring.
– substring
is the substring you want to find within the string.
– start
(optional) is the starting index for the search. By default, it starts from the beginning of the string.
– end
(optional) is the ending index for the search. By default, it searches to the end of the string.
Let’s see some examples to better understand how to use the find()
method:
Example 1: Finding a substring in a string
sentence = "Python is a powerful programming language." index = sentence.find("powerful") print(index) # Output: 10
In this example, the find()
method is used to search for the substring “powerful” within the sentence
string. Since “powerful” is found at index 10, the output is 10.
Example 2: Specifying the starting and ending indices
word = "manipulations" index = word.find("ip", 3, 10) print(index) # Output: 6
In this example, the find()
method is used to search for the substring “ip” within the word
string, but only between indices 3 and 10. Since “ip” is found at index 6 within that range, the output is 6.
Example 3: Handling non-existent substrings
text = "Hello, world!" index = text.find("Python") print(index) # Output: -1
In this example, the find()
method is used to search for the substring “Python” within the text
string. Since “Python” is not found in the string, the output is -1.
The find()
method can be very useful when you need to check if a string contains a specific substring or when you want to find the position of a substring within a larger string.
You can find more information about the find()
method in the Python documentation: https://docs.python.org/3/library/stdtypes.html#str.find.
Related Article: How To Check If a File Exists In Python
Using the index() Method
The index() method in Python is used to find the first occurrence of a substring within a string. It returns the index position of the substring if found, otherwise it raises a ValueError.
The syntax for using the index() method is as follows:
string.index(substring, start, end)
Here, string
is the original string, substring
is the substring we want to find, and start
and end
are optional parameters that specify the range of the string to be searched.
Let’s look at an example:
sentence = "The quick brown fox jumps over the lazy dog." index = sentence.index("fox") print(index)
Output:
16
In this example, we have a sentence and we want to find the index position of the substring “fox”. The index() method returns 16, which is the starting position of the substring “fox” within the sentence.
If the substring is not found, the index() method raises a ValueError. For example:
sentence = "The quick brown fox jumps over the lazy dog." index = sentence.index("cat")
Output:
ValueError: substring not found
In this example, the substring “cat” is not found within the sentence, so the index() method raises a ValueError.
It’s important to note that the index() method only returns the index of the first occurrence of the substring. If you want to find all occurrences of a substring within a string, you can use a loop or the find() method.
Now that you understand how to use the index() method in Python, you can easily find the index position of a substring within a string. This can be helpful when you need to manipulate or extract specific parts of a string in your Python programs.
Ignoring Case Sensitivity
Strings are case-sensitive by default. This means that when you compare two strings, Python considers uppercase and lowercase letters as distinct characters. However, there may be situations where you want to perform case-insensitive comparisons or manipulations on strings.
To ignore case sensitivity, you can convert both strings to lowercase or uppercase before comparing or manipulating them. Python provides two methods for this purpose: lower()
and upper()
.
The lower()
method converts all characters in a string to lowercase, while the upper()
method converts them to uppercase. Let’s see some examples:
string1 = "Hello" string2 = "hello" if string1.lower() == string2.lower(): print("The strings are equal (ignoring case sensitivity)") else: print("The strings are not equal") # Output: The strings are equal (ignoring case sensitivity)
In this example, we convert both strings to lowercase using the lower()
method before comparing them. As a result, the strings are considered equal, ignoring the difference in case.
You can also use these methods to manipulate strings with case-insensitive behavior. For example, if you want to search for a substring within a string without considering case sensitivity, you can convert both the substring and the string to lowercase or uppercase before searching. Here’s an example:
string = "Hello, World!" substring = "WORLD" if substring.lower() in string.lower(): print("Substring found") else: print("Substring not found") # Output: Substring found
Convert both the substring and the string to lowercase using the lower()
method before searching for the substring. As a result, the substring is found within the string, ignoring the difference in case.
Ignoring case sensitivity can be useful in various scenarios, such as user input validation, searching for keywords or patterns in text, or performing case-insensitive sorting. It provides flexibility and makes string manipulations more inclusive.
Keep in mind that when you ignore case sensitivity, you may lose some information about the original case of the characters. Therefore, use this approach judiciously according to your specific requirements.
Next, we’ll explore more string manipulations in Python.
Extracting Substrings
You can easily extract substrings from a string using a combination of indexing and slicing. Substrings are simply smaller parts of a larger string.
To extract a single character from a string, you can use square brackets with the index of the character you want to extract. Remember that Python uses zero-based indexing, so the first character has an index of 0.
string = "Hello, World!" first_character = string[0] print(first_character) # Output: H
You can also extract a range of characters from a string using slicing. Slicing allows you to specify a start index and an end index, separated by a colon. The start index is inclusive, while the end index is exclusive.
string = "Hello, World!" substring = string[7:12] print(substring) # Output: World
If you omit the start index in a slice, Python will assume it to be the beginning of the string. Similarly, if you omit the end index, Python will assume it to be the end of the string.
string = "Hello, World!" first_five_characters = string[:5] last_five_characters = string[7:] print(first_five_characters) # Output: Hello print(last_five_characters) # Output: World!
You can also use negative indexing to extract characters from the end of a string. In negative indexing, -1 refers to the last character, -2 refers to the second last character, and so on.
string = "Hello, World!" last_character = string[-1] second_last_character = string[-2] print(last_character) # Output: ! print(second_last_character) # Output: d
To extract a substring from a string based on a specific pattern or condition, you can use the split()
method. This method splits a string into a list of substrings based on a specified delimiter.
string = "Hello, World!" words = string.split(", ") print(words) # Output: ['Hello', 'World!']
You can then access the desired substring by using the index of the list.
string = "Hello, World!" words = string.split(", ") second_word = words[1] print(second_word) # Output: World!
Python provides a wide range of string manipulation methods and functions that can be used to extract and manipulate substrings. By mastering these techniques, you can efficiently work with strings in Python.
Related Article: How to Use Inline If Statements for Print in Python
Using Slicing to Extract Substrings
We can use slicing to extract substrings from a larger string. Slicing allows us to access a portion of a string by specifying a range of indices.
The syntax for slicing a string is as follows:
string[start:end:step]
– start
is the index where the slice starts (inclusive). If not specified, it defaults to the beginning of the string.
– end
is the index where the slice ends (exclusive). If not specified, it defaults to the end of the string.
– step
is the increment between indices. If not specified, it defaults to 1.
Let’s look at some examples to understand how slicing works:
string = "Hello, World!" # Extract a substring starting from index 7 to the end substring = string[7:] print(substring) # Output: "World!" # Extract a substring from index 0 to 5 (exclusive) substring = string[:5] print(substring) # Output: "Hello" # Extract a substring from index 0 to 5 with a step of 2 substring = string[::2] print(substring) # Output: "Hlo ol" # Extract a substring starting from index 7 to the end with a step of 2 substring = string[7::2] print(substring) # Output: "Wrd"
In the first example, we use slicing to extract a substring starting from index 7 to the end of the string. The resulting substring is “World!”.
In the second example, we use slicing to extract a substring from the beginning of the string to index 5 (exclusive). The resulting substring is “Hello”.
In the third example, we use slicing to extract a substring from the beginning of the string to the end with a step of 2. This means that we skip every second character in the resulting substring, resulting in “Hlo ol”.
In the fourth example, we use slicing to extract a substring starting from index 7 to the end with a step of 2. This means that we skip every second character in the resulting substring, resulting in “Wrd”.
Slicing also allows us to make modifications to the original string. For example, we can change the case of a substring:
string = "Hello, World!" # Convert the substring "World" to uppercase substring = string[7:].upper() print(substring) # Output: "WORLD!" # Replace the substring "World" with "Python" new_string = string[:7] + "Python" print(new_string) # Output: "Hello, Python!"
In the first example, we extract the substring “World” using slicing and convert it to uppercase using the upper()
method. The resulting substring is “WORLD!”.
In the second example, we use slicing to extract the substring “Hello,” and concatenate it with the string “Python” to create a new string “Hello, Python!”.
Slicing is a powerful feature in Python that allows us to manipulate strings efficiently. It provides a convenient way to extract substrings and perform various operations on them.
Using Regular Expressions
Regular expressions, often referred to as regex, are powerful tools for pattern matching and manipulation of strings in Python. They provide a concise and flexible way to search, extract, and manipulate text based on patterns.
Python provides built-in support for regular expressions through the re
module. This module contains functions and methods that allow you to work with regular expressions.
To use regular expressions in Python, you first import the re
module:
import re
Matching Patterns
The most basic operation with regular expressions is pattern matching. You can search for a specific pattern within a string using the re.search()
function. If the pattern is found, it returns a match object; otherwise, it returns None.
Here’s an example that searches for the word “apple” in a string:
import re text = "I love apples" pattern = r"apple" match = re.search(pattern, text) if match: print("Pattern found!") else: print("Pattern not found.")
In this example, we use the regular expression pattern r"apple"
to search for the word “apple” in the text
string. The re.search()
function returns a match object if the pattern is found.
Related Article: How to Use Stripchar on a String in Python
Extracting Matches
Regular expressions also allow you to extract specific parts of a string that match a pattern. You can use the re.findall()
function to find all occurrences of a pattern in a string and return them as a list of strings.
Here’s an example that extracts all the email addresses from a given string:
import re text = "Contact us at info@example.com or support@example.com" pattern = r"b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+.[A-Za-z]{2,}b" matches = re.findall(pattern, text) for match in matches: print(match)
In this example, we use the regular expression pattern r"b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+.[A-Za-z]{2,}b"
to match email addresses. The re.findall()
function returns all matches as a list of strings.
Replacing Matches
Regular expressions also allow you to replace specific parts of a string that match a pattern. You can use the re.sub()
function to search for a pattern in a string and replace it with a specified string.
Here’s an example that replaces all occurrences of the word “apple” with “orange” in a given string:
import re text = "I love apples. Apples are delicious." pattern = r"apple" new_text = re.sub(pattern, "orange", text) print(new_text)
In this example, we use the regular expression pattern r"apple"
to search for the word “apple” in the text
string. The re.sub()
function replaces all occurrences of the pattern with the specified string, “orange”.
Modifiers and Special Characters
Regular expressions support various modifiers and special characters that allow you to create more complex patterns. Some common modifiers and special characters include:
– .
– Matches any character except a newline.
– *
– Matches zero or more occurrences of the preceding character or group.
– +
– Matches one or more occurrences of the preceding character or group.
– ?
– Matches zero or one occurrence of the preceding character or group.
– ^
– Matches the start of a string.
– $
– Matches the end of a string.
– []
– Matches any single character within the brackets.
– – Escapes a special character.
– |
– Matches either the expression before or after the pipe symbol.
For a complete list of modifiers and special characters, refer to the Python documentation on regular expressions.
Regular expressions are a powerful tool for string manipulation in Python. They allow you to search, extract, and replace patterns in strings efficiently. By mastering regular expressions, you can greatly enhance your string handling capabilities in Python.
Now that you have learned the basics of using regular expressions in Python, you can start applying them to your own projects and explore more advanced features and techniques.
Related Article: How To Delete A File Or Folder In Python
Replacing Substrings
You can easily replace substrings within a string using the replace()
method. This method allows you to replace all occurrences of a substring with a new string. Here’s the syntax:
new_string = original_string.replace(old_substring, new_substring)
Let’s say we have a string called sentence
and we want to replace all occurrences of the word “apple” with the word “orange”. We can do it like this:
sentence = "I have an apple, a red apple, and a green apple." new_sentence = sentence.replace("apple", "orange") print(new_sentence)
Output:
I have an orange, a red orange, and a green orange.
As you can see, all occurrences of the word “apple” have been replaced with “orange” in the new_sentence
string.
The replace()
method also allows you to specify the maximum number of replacements to be made by providing an optional third parameter. For example, if we only want to replace the first occurrence of the word “apple” with “orange”, we can do it like this:
sentence = "I have an apple, a red apple, and a green apple." new_sentence = sentence.replace("apple", "orange", 1) print(new_sentence)
Output:
I have an orange, a red apple, and a green apple.
In this case, only the first occurrence of “apple” is replaced with “orange”, while the other occurrences remain unchanged.
It’s important to note that the replace()
method doesn’t modify the original string. Instead, it returns a new string with the replacements made.
If you want to replace multiple substrings at once, you can chain multiple replace()
calls. Each call will return a new string, which can be used as the input for the next replacement. Here’s an example:
sentence = "I have an apple, a red apple, and a green apple." new_sentence = sentence.replace("apple", "orange").replace("red", "blue") print(new_sentence)
Output:
I have an orange, a blue orange, and a green orange.
In this example, both the word “apple” and the word “red” are replaced, resulting in the new_sentence
string.
That’s it for replacing substrings in Python strings using the replace()
method. It’s a simple yet powerful way to manipulate strings and make changes as needed.
Replacing All Occurrences
You can replace all occurrences of a substring within a string using the replace()
method. This method returns a new string where all occurrences of the specified substring are replaced with a new substring.
The syntax for the replace()
method is as follows:
new_string = old_string.replace(substring, new_substring)
Here, old_string
is the original string, substring
is the substring you want to replace, and new_substring
is the new substring that will replace all occurrences of substring
in old_string
.
Let’s take a look at an example to understand how this works:
sentence = "I love apples, they are my favorite fruit" new_sentence = sentence.replace("apples", "bananas") print(new_sentence)
Output:
I love bananas, they are my favorite fruit
In the above example, we replaced all occurrences of the word “apples” with “bananas” in the sentence
string using the replace()
method. The resulting string new_sentence
is then printed, which shows the replaced string.
It’s important to note that the replace()
method is case-sensitive. This means that if you want to replace a substring regardless of its case, you need to convert the string to lowercase or uppercase using the lower()
or upper()
methods before calling the replace()
method.
Here’s an example that demonstrates how to replace all occurrences of a substring case-insensitively:
sentence = "I love Apples, they are my favorite fruit" new_sentence = sentence.lower().replace("apples", "bananas") print(new_sentence)
Output:
i love bananas, they are my favorite fruit
In this example, we first convert the sentence
string to lowercase using the lower()
method, and then replace all occurrences of the lowercase substring “apples” with “bananas”. The resulting string new_sentence
is printed, which shows the replaced string.
That’s all you need to know about replacing all occurrences of a substring in a string using Python!
Replacing Case Insensitive
You can replace substrings within a string using the replace()
method. By default, this method performs case-sensitive replacements.
To perform a case-insensitive replacement, you can use the re
module in Python, which provides support for regular expressions. Regular expressions allow you to search for patterns within strings and perform various operations, including case-insensitive replacements.
Here’s an example that demonstrates how to perform a case-insensitive replacement using regular expressions:
import re string = "The quick brown fox jumps over the lazy dog" pattern = r"fox" replacement = "cat" new_string = re.sub(pattern, replacement, string, flags=re.IGNORECASE) print(new_string)
Output:
The quick brown cat jumps over the lazy dog
In the example above, we import the re
module and define the input string, pattern, and replacement. The re.sub()
function is used to perform the case-insensitive replacement. The flags=re.IGNORECASE
parameter ensures that the replacement is case-insensitive.
Another approach to achieve case-insensitive replacements is by converting the string to lowercase (or uppercase) before performing the replacement. This approach can be useful when you know the specific case you are dealing with.
Here’s an example that demonstrates this approach:
string = "The quick brown fox jumps over the lazy dog" pattern = "fox" replacement = "cat" new_string = string.lower().replace(pattern.lower(), replacement) print(new_string)
Output:
The quick brown cat jumps over the lazy dog
In the example above, we convert both the input string and the pattern to lowercase using the lower()
method. Then, we use the replace()
method to perform the case-insensitive replacement.
Both approaches described above can be used to replace substrings in a case-insensitive manner. Choose the approach that best suits your specific case and requirements.
Now that you understand how to perform case-insensitive replacements in Python, you can apply this knowledge to manipulate strings in various scenarios.
Related Article: How To Move A File In Python
Splitting a String
Splitting a string is a common operation in Python when you need to separate a string into multiple parts based on a delimiter. Python provides a built-in method called split()
that allows you to split a string into a list of substrings.
The split()
method takes an optional parameter called delimiter
, which specifies the character or sequence of characters to use as the delimiter. If no delimiter is provided, the method splits the string at whitespace characters by default.
Here’s an example that demonstrates how to use the split()
method:
sentence = "Hello, world! How are you today?" words = sentence.split() print(words)
Output:
['Hello,', 'world!', 'How', 'are', 'you', 'today?']
In this example, we split the sentence
string into a list of words by calling the split()
method without any arguments. The resulting list, words
, contains each word from the original string as separate elements.
You can also specify a custom delimiter to split the string based on a specific character or sequence of characters. For example:
numbers = "1-2-3-4-5" digits = numbers.split("-") print(digits)
Output:
['1', '2', '3', '4', '5']
In this case, we split the numbers
string using the hyphen -
as the delimiter. The resulting list, digits
, contains each individual number as separate elements.
If you want to split the string into a limited number of parts, you can use the optional maxsplit
parameter. This parameter specifies the maximum number of splits to perform. Here’s an example:
sentence = "I love Python programming" words = sentence.split(" ", 2) print(words)
Output:
['I', 'love', 'Python programming']
In this example, we split the sentence
string into three parts using the space character as the delimiter. The resulting list, words
, contains the first three words from the original string.
It’s worth noting that the split()
method always returns a list, even if the original string doesn’t contain the delimiter. If the delimiter is not found in the string, the method returns a list with a single element, which is the original string itself.
Splitting a string is a powerful operation that can be used in various scenarios, such as parsing CSV files, extracting specific parts of a string, or tokenizing text for natural language processing tasks.
Splitting at a Specific Character
You can split a string into multiple parts by using a specific character as the delimiter. The result will be a list of substrings.
To split a string at a specific character, you can use the split()
method. This method takes the delimiter as an argument and returns a list of substrings.
Here’s an example:
string = "Hello, World! How are you?" parts = string.split(",") print(parts)
Output:
['Hello', ' World! How are you?']
In this example, we split the string at the comma (,
) character. The resulting list contains two substrings: 'Hello'
and ' World! How are you?'
.
You can also split a string at a specific character multiple times. For example:
string = "apple,banana,orange,grape" fruits = string.split(",") print(fruits)
Output:
['apple', 'banana', 'orange', 'grape']
In this case, we split the string at the comma (,
) character and get a list of four substrings: 'apple'
, 'banana'
, 'orange'
, and 'grape'
.
If the delimiter is not found in the string, the split()
method will return a list with a single element, which is the original string itself. For example:
string = "Hello, World!" parts = string.split(";") print(parts)
Output:
['Hello, World!']
In this case, since the semicolon (;
) is not found in the string, the split()
method returns a list with a single element: 'Hello, World!'
.
It’s worth noting that the split()
method does not modify the original string. Instead, it returns a new list of substrings.
Now that you know how to split a string at a specific character, you can use this knowledge to manipulate and process strings effectively in Python.
Splitting with Regular Expressions
Regular expressions (regex) are a powerful tool for pattern matching and manipulation of strings. Python provides the re
module, which allows you to work with regular expressions.
To split a string using a regular expression pattern, you can use the re.split()
function. This function takes two arguments: the regex pattern and the string to be split. It returns a list of substrings that match the pattern.
Here’s a simple example that splits a string using a regular expression:
import re sentence = "I love Python programming" words = re.split(r"s", sentence) print(words)
Output:
['I', 'love', 'Python', 'programming']
In this example, we split the string sentence
into a list of words by using the regular expression pattern r"s"
. The pattern s
matches any whitespace character.
You can also split a string based on multiple patterns by using the |
(pipe) character. The |
character acts as an OR operator in regular expressions.
Here’s an example that splits a string based on either a comma or a space:
import re data = "apple,banana,orange apple banana orange" items = re.split(r"s|,", data) print(items)
Output:
['apple', 'banana', 'orange', 'apple', 'banana', 'orange']
In this example, the regular expression pattern r"s|,"
matches either a comma or a space.
You can also use more complex regular expressions to split strings. For example, you can split a string by a specific pattern or a sequence of characters.
Here’s an example that splits a string based on a sequence of digits:
import re text = "I have 123 apples and 456 bananas" numbers = re.split(r"d+", text) print(numbers)
Output:
['I have ', ' apples and ', ' bananas']
In this example, the regular expression pattern r"d+"
matches one or more digits.
Regular expressions provide a flexible and powerful way to split strings based on patterns. They are particularly useful when dealing with complex string manipulations.
To learn more about regular expressions in Python, you can refer to the official documentation.
Related Article: How to Implement a Python Foreach Equivalent
Joining Strings
You can join two or more strings together using the +
operator or the join()
method. Joining strings is a common operation when working with text data or when you need to concatenate multiple strings into one.
Using the + Operator
The simplest way to join strings is by using the +
operator. This operator concatenates the strings together, resulting in a new string that contains the combined content of the original strings. Here’s an example:
string1 = "Hello" string2 = "world" result = string1 + " " + string2 print(result) # Output: Hello world
In the above code snippet, we use the +
operator to concatenate string1
, a space character, and string2
. The result is stored in the result
variable, which we then print to the console.
Using the join() Method
Another way to join strings is by using the join()
method. This method is called on a string object and takes an iterable (such as a list or tuple) as its argument. It concatenates all the elements of the iterable, using the string on which it was called as the separator. Here’s an example:
words = ["Hello", "world"] result = " ".join(words) print(result) # Output: Hello world
In the above code snippet, we create a list called words
that contains the strings “Hello” and “world”. We then call the join()
method on the string " "
, specifying it as the separator. The method concatenates all the elements of the words
list with a space character in between, resulting in the string "Hello world"
. Finally, we print the result to the console.
Related Article: How to Use Slicing in Python And Extract a Portion of a List
Joining Strings with Other Data Types
You can also join strings with other data types, such as integers or floats. However, before doing so, you need to convert the non-string data types to strings using the str()
function. Here’s an example:
number = 42 message = "The answer is: " + str(number) print(message) # Output: The answer is: 42
In the above code snippet, we convert the integer number
to a string using the str()
function. We then concatenate it with the string "The answer is: "
, resulting in the string "The answer is: 42"
. Finally, we print the message to the console.
Remember that the join()
method requires all elements in the iterable to be strings. If you try to join a list that contains non-string elements without converting them to strings, a TypeError
will be raised.
Joining strings is a powerful tool in Python that allows you to combine text in various ways. Whether you use the +
operator or the join()
method, understanding how to join strings will be beneficial in many programming scenarios.
Joining with a Specific Separator
You can join multiple strings together using a specific separator by using the join()
method. This method takes an iterable as its argument and returns a new string that is the concatenation of all the strings in the iterable, separated by the specified separator.
Here’s the syntax for using the join()
method:
separator = "" joined_string = separator.join(iterable)
In the above syntax, represents the separator string that you want to use to join the strings together. The
iterable
argument is any iterable object, such as a list, tuple, or string, that contains the strings you want to join.
Let’s look at a few examples to understand how the join()
method works:
Example 1: Joining a list of strings
fruits = ["apple", "banana", "orange"] separator = ", " joined_string = separator.join(fruits) print(joined_string)
Output:
apple, banana, orange
In the above example, we have a list of strings called fruits
. We want to join these strings together with a comma and a space as the separator. By using the join()
method, we get the desired output.
Example 2: Joining a tuple of strings
names = ("John", "Jane", "Doe") separator = " | " joined_string = separator.join(names) print(joined_string)
Output:
John | Jane | Doe
In this example, we have a tuple of strings called names
. We want to join these strings together with a pipe symbol and a space as the separator. The join()
method helps us achieve this.
Example 3: Joining a string with no separator
text = "Hello World" joined_string = "".join(text) print(joined_string)
Output:
Hello World
In this example, we have a string called text
. We want to join the characters of this string together with no separator. By passing an empty string as the separator to the join()
method, we get the original string as the output.
The join()
method is a powerful tool for joining strings with a specific separator. By understanding how to use this method, you can manipulate and format strings in Python with ease.
Joining with a Custom Separator
You can use the join()
function to concatenate a list of strings with a custom separator. This can be useful when you want to create a single string from a list of elements, separating them with a specific character or string.
The join()
function is a string method that takes a list as its argument and returns a new string. The elements of the list are concatenated together, with the separator specified in the string used to call the function.
Here’s an example that demonstrates how to use the join()
function:
names = ['John', 'Jane', 'Joe'] separator = ', ' joined_string = separator.join(names) print(joined_string)
Output:
John, Jane, Joe
In this example, we have a list of names and a separator string ', '
. We use the join()
function to concatenate the elements of the list together, separating them with the separator. The resulting string is then printed to the console.
You can use any character or string as a separator with the join()
function. For example, if you want to separate the elements with a hyphen, you can specify '-'
as the separator.
numbers = ['1', '2', '3', '4'] separator = '-' joined_string = separator.join(numbers) print(joined_string)
Output:
1-2-3-4
In this example, we have a list of numbers and a separator string '-'
. The join()
function concatenates the elements of the list together, separating them with the hyphen character. The resulting string is then printed to the console.
The join()
function is a powerful tool for manipulating and formatting strings in Python. It allows you to easily concatenate a list of strings with a custom separator, making it useful for various applications such as creating CSV files or formatting output for display.
For more information on the join()
function, you can refer to the official Python documentation on str.join().
Related Article: How to Check a Variable's Type in Python
Converting Strings to Upper or Lower Case
You can easily convert strings to either upper or lower case using built-in methods. These methods are particularly useful when you want to normalize your string data or when you need to perform case-insensitive comparisons.
To convert a string to uppercase, you can use the upper()
method. This method returns a new string with all the characters converted to uppercase. Here’s an example:
message = "hello, world!" uppercase_message = message.upper() print(uppercase_message)
Output:
HELLO, WORLD!
As you can see, the upper()
method converted all the lowercase characters in the string to uppercase.
Similarly, to convert a string to lowercase, you can use the lower()
method. This method returns a new string with all the characters converted to lowercase. Here’s an example:
message = "HELLO, WORLD!" lowercase_message = message.lower() print(lowercase_message)
Output:
hello, world!
The lower()
method converted all the uppercase characters in the string to lowercase.
It’s important to note that both the upper()
and lower()
methods return a new string and do not modify the original string. If you want to modify the original string, you need to assign the result back to the original variable.
These methods are particularly handy when you need to perform case-insensitive comparisons. For example, you can compare two strings without worrying about the difference in case:
string1 = "Hello" string2 = "hello" if string1.lower() == string2.lower(): print("The strings are equal (case-insensitive)") else: print("The strings are not equal (case-insensitive)")
Output:
The strings are equal (case-insensitive)
In the above example, even though the strings have different cases, the case-insensitive comparison using the lower()
method returns True.
Converting strings to upper or lower case in Python is simple and can be done using the upper() and lower() methods. These methods are useful for normalizing string data and performing case-insensitive comparisons.
Removing Leading and Trailing Whitespace
Strings often contain leading and trailing whitespace characters such as spaces, tabs, or newlines. These whitespace characters can sometimes be problematic when working with strings, especially when comparing or manipulating them. Fortunately, Python provides several built-in methods to remove leading and trailing whitespace from strings.
1. Using the strip() method:
The strip()
method removes leading and trailing whitespace characters from a string. It returns a new string with the whitespace characters removed.
Here’s an example:
text = " hello world " stripped_text = text.strip() print(stripped_text) # Output: "hello world"
In this example, the original string ” hello world ” has leading and trailing whitespace characters. The strip()
method removes these whitespace characters and returns the modified string “hello world”.
Related Article: How to Use Increment and Decrement Operators in Python
2. Using the lstrip() method:
The lstrip()
method removes only the leading whitespace characters from a string. It returns a new string with the leading whitespace characters removed.
Here’s an example:
text = " hello world " stripped_text = text.lstrip() print(stripped_text) # Output: "hello world "
In this example, the original string ” hello world ” has leading whitespace characters. The lstrip()
method removes these leading whitespace characters and returns the modified string “hello world “.
3. Using the rstrip() method:
The rstrip()
method removes only the trailing whitespace characters from a string. It returns a new string with the trailing whitespace characters removed.
Here’s an example:
text = " hello world " stripped_text = text.rstrip() print(stripped_text) # Output: " hello world"
In this example, the original string ” hello world ” has trailing whitespace characters. The rstrip()
method removes these trailing whitespace characters and returns the modified string ” hello world”.
It’s important to note that all three methods mentioned above return a new string without modifying the original string. If you want to modify the original string in-place, you can assign the result back to the same variable.
Removing leading and trailing whitespace from strings is a common task when handling user input, reading data from files, or comparing strings. Understanding these methods will help you clean up strings and ensure consistent string comparisons and manipulations.
Removing Leading and Trailing Characters
You can remove leading and trailing characters from a string using various built-in string manipulation methods. These methods allow you to remove specific characters or remove characters based on a certain condition.
Related Article: How to Import Other Python Files in Your Code
1. Using the strip() method:
The strip()
method removes leading and trailing characters from a string. By default, it removes whitespace characters including spaces, tabs, and newline characters.
Here’s an example that demonstrates how to use the strip()
method:
string = " hello world " stripped_string = string.strip() print(stripped_string) # Output: "hello world"
In the example above, the leading and trailing whitespace characters are removed from the string using the strip()
method.
If you want to remove specific characters instead of whitespace, you can pass a string of characters to the strip()
method. It will remove any leading and trailing occurrences of those characters.
string = "!!hello world!!" stripped_string = string.strip("!") print(stripped_string) # Output: "hello world"
In this example, the strip()
method is called with the argument “!”, so it removes any leading and trailing exclamation marks from the string.
2. Using the lstrip() and rstrip() methods:
If you only want to remove leading or trailing characters, you can use the lstrip()
method to remove leading characters and the rstrip()
method to remove trailing characters.
Here’s an example that demonstrates how to use these methods:
string = " hello world " left_stripped_string = string.lstrip() right_stripped_string = string.rstrip() print(left_stripped_string) # Output: "hello world " print(right_stripped_string) # Output: " hello world"
In the example above, the lstrip()
method removes the leading whitespace characters, while the rstrip()
method removes the trailing whitespace characters from the string.
Both the lstrip()
and rstrip()
methods can also accept a string of characters to remove specific leading or trailing occurrences of those characters.
3. Using regular expressions:
If you need more complex pattern matching to remove leading and trailing characters, you can use regular expressions with the re
module.
Here’s an example that demonstrates how to use regular expressions to remove leading and trailing characters:
import re string = "XXhello worldXX" pattern = r"^X+|X+$" stripped_string = re.sub(pattern, "", string) print(stripped_string) # Output: "hello world"
In the example above, the regular expression pattern ^X+|X+$
matches one or more occurrences of “X” at the start of the string (^X+
) or at the end of the string (X+$
). The re.sub()
function is then used to replace the matched pattern with an empty string, effectively removing the leading and trailing “X” characters from the string.
These are some of the methods you can use to remove leading and trailing characters from a string in Python. Choose the method that best fits your requirements and manipulate your strings accordingly.
Related Article: How to Use Named Tuples in Python
Checking if a String Starts or Ends with a Substring
You can check if a string starts or ends with a specific substring using built-in methods. This can be useful in many scenarios, such as validating user input or manipulating text data.
To check if a string starts with a specific substring, you can use the startswith()
method. This method returns True
if the string starts with the specified substring, and False
otherwise. Here’s an example:
text = "Hello, world!" if text.startswith("Hello"): print("The string starts with 'Hello'.") else: print("The string does not start with 'Hello'.")
Output:
The string starts with 'Hello'.
Similarly, to check if a string ends with a specific substring, you can use the endswith()
method. This method returns True
if the string ends with the specified substring, and False
otherwise. Here’s an example:
text = "Hello, world!" if text.endswith("world!"): print("The string ends with 'world!'.") else: print("The string does not end with 'world!'.")
Output:
The string ends with 'world!'.
Both startswith()
and endswith()
methods are case-sensitive, meaning that the substring must match exactly in terms of case. If you want to perform a case-insensitive check, you can convert both the string and the substring to lowercase (or uppercase) using the lower()
(or upper()
) method before performing the check.
Here’s an example of performing a case-insensitive check using the lower()
method:
text = "Hello, world!" if text.lower().startswith("hello"): print("The string starts with 'Hello' (case-insensitive check).") else: print("The string does not start with 'Hello' (case-insensitive check).")
Output:
The string starts with 'Hello' (case-insensitive check).
Similarly, you can perform a case-insensitive check using the endswith()
method.
In addition to checking if a string starts or ends with a specific substring, you can also use these methods to check multiple substrings. Simply pass a tuple of substrings as the argument to the startswith()
or endswith()
method. The methods will return True
if the string starts or ends with any of the substrings in the tuple.
text = "Hello, world!" if text.startswith(("Hello", "Hi")): print("The string starts with 'Hello' or 'Hi'.") else: print("The string does not start with 'Hello' or 'Hi'.")
Output:
The string starts with 'Hello' or 'Hi'.
Similarly, you can pass a tuple of substrings to the endswith()
method for checking multiple substrings.
Counting Occurrences of a Substring
You can count the number of occurrences of a substring within a string using the count()
method. This method returns the number of non-overlapping occurrences of the substring in the given string.
Here’s an example that demonstrates how to use the count()
method:
sentence = "I love Python programming, Python is my favorite language" substring = "Python" count = sentence.count(substring) print(f"The substring '{substring}' appears {count} times in the sentence.")
Output:
The substring 'Python' appears 2 times in the sentence.
In the above example, we have a sentence that contains the word “Python” twice. We use the count()
method to count the number of occurrences and store the result in the variable count
. Finally, we print the result using an f-string.
It’s important to note that the count()
method is case-sensitive. This means that it will only count occurrences that match the exact case of the substring. For example, if we search for the substring “python” instead of “Python” in the above example, the count would be 0.
If you want to perform a case-insensitive search, you can convert both the string and the substring to lowercase (or uppercase) before using the count()
method. Here’s an example:
sentence = "I love Python programming, Python is my favorite language" substring = "python" count = sentence.lower().count(substring.lower()) print(f"The substring '{substring}' appears {count} times in the sentence.")
Output:
The substring 'python' appears 2 times in the sentence.
In this example, both the sentence
and substring
are converted to lowercase using the lower()
method before applying the count()
method. This ensures that the search is case-insensitive.
With the count()
method, you can easily determine the number of occurrences of a substring within a string. This can be useful in various scenarios, such as text analysis, data processing, and pattern matching.
Checking if a String Contains Only Digits
There are several approaches to accomplish this task. Let’s explore a few of them.
Related Article: How to Work with CSV Files in Python: An Advanced Guide
Using the isdigit() Method:
The easiest way to check if a string contains only digits is by using the isdigit()
method. This method returns True
if all characters in the string are digits, and False
otherwise. Here’s an example:
string = "12345" if string.isdigit(): print("The string contains only digits.") else: print("The string contains non-digit characters.")
This will output: The string contains only digits.
Using Regular Expressions:
Another approach is to use regular expressions, which provide a powerful way to match patterns in strings. The re
module in Python allows you to work with regular expressions. Here’s an example of how to check if a string contains only digits using regular expressions:
import re string = "12345" if re.match("^d+$", string): print("The string contains only digits.") else: print("The string contains non-digit characters.")
This will also output: The string contains only digits.
Using a Custom Function:
If you prefer a custom function, you can create one that iterates over each character in the string and checks if it is a digit. Here’s an example:
def contains_only_digits(string): for char in string: if not char.isdigit(): return False return True string = "12345" if contains_only_digits(string): print("The string contains only digits.") else: print("The string contains non-digit characters.")
Again, this will output: The string contains only digits.
These are just a few ways to check if a string contains only digits in Python. Choose the approach that suits your needs and the complexity of your task.
For more information on string manipulation in Python, you can refer to the official Python documentation on string methods.
Related Article: String Comparison in Python: Best Practices and Techniques
Checking if a String Contains Only Letters
One way to check if a string contains only letters is by using the isalpha() method. This method returns True if all characters in the string are alphabetic, meaning they are either uppercase or lowercase letters. Here’s an example:
string1 = "Hello" string2 = "Hello World!" string3 = "123" string4 = "abc123" print(string1.isalpha()) # Output: True print(string2.isalpha()) # Output: False print(string3.isalpha()) # Output: False print(string4.isalpha()) # Output: False
As you can see, the isalpha() method correctly identifies that string1 contains only letters, while the other strings contain non-alphabetic characters.
Another approach is to use regular expressions. The re module in Python provides a powerful way to perform pattern matching operations on strings. You can use the match() function from re to check if a string matches a specified pattern. To check if a string contains only letters, you can use the pattern “[a-zA-Z]+” which matches one or more alphabetical characters. Here’s an example:
import re string1 = "Hello" string2 = "Hello World!" string3 = "123" string4 = "abc123" pattern = "[a-zA-Z]+" print(re.match(pattern, string1)) # Output: print(re.match(pattern, string2)) # Output: None print(re.match(pattern, string3)) # Output: None print(re.match(pattern, string4)) # Output:
In this example, the match() function returns a match object if the string matches the pattern, otherwise it returns None. As you can see, the match() function correctly identifies that string1 and string4 contain only letters, while the other strings do not match the pattern.
These methods should cover most scenarios when you need to check if a string contains only letters in Python. You can choose the one that fits your needs and use it in your projects accordingly. Remember to always validate user input to ensure your code behaves as expected.
Checking if a String Contains Only Alphanumeric Characters
The isalnum()
function returns True
if all characters in a string are alphanumeric and False
otherwise. It considers both uppercase and lowercase alphabets, as well as numbers.
Here’s an example that demonstrates the usage of the isalnum()
function:
string1 = "HelloWorld123" string2 = "Hello, World!" print(string1.isalnum()) # Output: True print(string2.isalnum()) # Output: False
In the above example, string1
consists of only alphanumeric characters, so isalnum()
returns True
. On the other hand, string2
contains a space and a punctuation mark, so isalnum()
returns False
.
If you want to check whether a string contains only letters or only digits, you can combine the isalpha()
or isdigit()
functions with the isalnum()
function.
Here’s an example that demonstrates this:
string3 = "HelloWorld" string4 = "12345" print(string3.isalpha()) # Output: True print(string4.isdigit()) # Output: True print(string3.isalnum()) # Output: True print(string4.isalnum()) # Output: True
In the above example, string3
consists of only alphabets, so isalpha()
returns True
. Similarly, string4
consists of only digits, so isdigit()
returns True
. Both string3
and string4
are considered alphanumeric since they contain only letters or digits, so isalnum()
returns True
for both.
Remember to handle empty strings appropriately, as they will also return False
when using the isalnum()
function.
Now that you know how to check if a string contains only alphanumeric characters using the isalnum()
function, you can easily validate user input or perform data cleaning operations in your Python programs.
Formatting Strings
This allows you to create dynamic output by inserting variables or values into a string. Here are some common techniques for formatting strings in Python:
Related Article: Python Operators Tutorial & Advanced Examples
1. Using the format()
method
The format()
method is a versatile way to format strings in Python. It allows you to insert variables or values into a string by using curly braces {}
as placeholders. Here’s an example:
name = "Alice" age = 25 message = "My name is {} and I am {} years old.".format(name, age) print(message)
Output:
My name is Alice and I am 25 years old.
You can also specify the position of the arguments passed to the format()
method:
name = "Bob" age = 30 message = "My name is {1} and I am {0} years old.".format(age, name) print(message)
Output:
My name is Bob and I am 30 years old.
2. Using f-strings
Starting from Python 3.6, you can use f-strings, also known as formatted string literals, to format strings. F-strings make it easier to embed expressions inside string literals by using curly braces {}
. Here’s an example:
name = "Charlie" age = 35 message = f"My name is {name} and I am {age} years old." print(message)
Output:
My name is Charlie and I am 35 years old.
You can also perform computations inside f-strings:
x = 10 y = 5 message = f"The sum of {x} and {y} is {x + y}." print(message)
Output:
The sum of 10 and 5 is 15.
3. Using the %
operator
Another way to format strings in Python is by using the %
operator. This operator is similar to the printf
function in C. Here’s an example:
name = "David" age = 40 message = "My name is %s and I am %d years old." % (name, age) print(message)
Output:
My name is David and I am 40 years old.
The %s
and %d
are conversion specifiers that indicate the type of the variable to be inserted into the string. %s
is used for strings, and %d
is used for integers.
These are just a few techniques for formatting strings in Python. Each method has its own advantages and use cases, so choose the one that fits your needs the best. You can find more information and examples in the official Python documentation on string formatting.
Related Article: How To Round To 2 Decimals In Python
Using f-strings
F-strings, short for formatted string literals, allow you to embed expressions inside string literals by prefixing the string with the letter ‘f’. This feature was introduced in Python 3.6 and has become the recommended way to format strings in Python.
To use an f-string, simply prefix the string literal with the letter ‘f’ and enclose the expressions you want to include inside curly braces {}. The expressions will be evaluated and their values will be inserted into the string at runtime.
Here’s a simple example to demonstrate the usage of f-strings:
name = "Alice" age = 25 print(f"My name is {name} and I am {age} years old.")
Output:
My name is Alice and I am 25 years old.
In the above example, the expressions {name}
and {age}
are replaced with their corresponding values when the string is printed.
F-strings also support various formatting options to control how the values are displayed. You can specify the width, precision, and alignment of the values, as well as format them as integers, floats, or even dates.
Here are a few examples of f-string formatting options:
– Specifying the width of a value:
number = 42 print(f"The answer is {number:5}.")
Output:
The answer is 42.
In this example, the value of number
is displayed in a field of width 5. The value is right-aligned by default.
– Specifying the precision of a float:
pi = 3.14159 print(f"Pi is approximately {pi:.2f}.")
Output:
Pi is approximately 3.14.
In this example, the value of pi
is displayed with a precision of 2 decimal places.
– Aligning values to the left:
name = "Bob" print(f"Hello, {name:<10}!")
Output:
Hello, Bob !
In this example, the value of name
is left-aligned within a field of width 10.
– Formatting dates:
from datetime import date today = date.today() print(f"Today's date is {today:%d-%m-%Y}.")
Output:
Today's date is 25-12-2022.
In this example, the value of today
is formatted as a date using the specified format %d-%m-%Y.
F-strings are a powerful and concise way to format strings in Python. They offer a lot of flexibility and make it easy to include expressions and format values within string literals. If you're using Python 3.6 or later, it's a good idea to start using f-strings in your code.
To learn more about f-strings and their formatting options, you can refer to the official Python documentation on f-strings: https://docs.python.org/3/reference/lexical_analysis.html#f-strings.
Using the format() Method
The format()
method is a powerful built-in function in Python that allows us to format strings by replacing placeholders with values. It provides a concise and flexible way to construct strings with dynamic content.
The syntax for using the format()
method is straightforward. We start with a string containing one or more placeholders, marked by curly braces {}
. Inside the curly braces, we can optionally include field names or format specifiers to control the formatting of the values.
Let’s look at a simple example to understand how the format()
method works:
name = "Alice" age = 25 message = "My name is {} and I am {} years old.".format(name, age) print(message)
Output:
My name is Alice and I am 25 years old.
In the example above, we have a string with two placeholders {}
. We call the format()
method on the string and pass the values we want to substitute for the placeholders. The format()
method returns a new string with the placeholders replaced by the provided values.
We can also use field names inside the placeholders to refer to specific values:
name = "Bob" age = 30 message = "My name is {name} and I am {age} years old.".format(name=name, age=age) print(message)
Output:
My name is Bob and I am 30 years old.
In the example above, we specify the field names name
and age
inside the placeholders. We pass the values for these fields as keyword arguments to the format()
method.
The format()
method also supports format specifiers, which allow us to control the formatting of the values. For example, we can specify the number of decimal places for a floating-point number:
pi = 3.141592653589793 formatted_pi = "Value of pi: {:.2f}".format(pi) print(formatted_pi)
Output:
Value of pi: 3.14
In the example above, we use the format specifier :.2f
to format the value of pi
with two decimal places.
We can also use format specifiers to control the width and alignment of the values, display numbers in different bases (e.g., binary, octal, hexadecimal), and apply various other formatting options. The format specifiers provide a lot of flexibility for customizing the output.
The format()
method is a versatile tool for string manipulation in Python. It allows us to easily substitute values into placeholders and control the formatting of the resulting string. By understanding the syntax and usage of the format()
method, we can enhance our string manipulation capabilities in Python.
Using String Interpolation
String interpolation refers to the process of embedding values or expressions within a string. It allows you to create dynamic strings by combining static text with variables or expressions. String interpolation can make your code more readable and maintainable, as it eliminates the need for string concatenation.
Python provides several ways to perform string interpolation:
1. Using the ‘%’ operator: This is the oldest and most commonly used method for string interpolation in Python. The ‘%’ operator allows you to substitute values into a string using placeholders.
name = "John" age = 25 message = "My name is %s and I am %d years old." % (name, age) print(message)
Output:
My name is John and I am 25 years old.
In the above example, the ‘%s’ and ‘%d’ are placeholders for the name
and age
variables, respectively. The values are substituted into the string using the ‘%’ operator.
2. Using the format()
method: This is a more modern and preferred method for string interpolation in Python. The format()
method allows you to substitute values into a string by using curly braces as placeholders.
name = "John" age = 25 message = "My name is {} and I am {} years old.".format(name, age) print(message)
Output:
My name is John and I am 25 years old.
In this example, the curly braces ‘{}’ act as placeholders. The values are substituted into the string by calling the format()
method on the string object and passing the values as arguments.
3. Using f-strings: Introduced in Python 3.6, f-strings provide a concise and readable way to perform string interpolation. They allow you to embed expressions inside curly braces prefixed with the ‘f’ character.
name = "John" age = 25 message = f"My name is {name} and I am {age} years old." print(message)
Output:
My name is John and I am 25 years old.
In this example, the expressions inside the curly braces are evaluated and the results are substituted into the string.
String interpolation is not limited to simple variables. You can also interpolate the results of function calls, perform arithmetic operations, and format the values using various formatting options.
Formatting options: String interpolation provides various formatting options to control the appearance of the interpolated values. These options can be specified inside the curly braces using a colon followed by the format specifier.
pi = 3.14159 formatted_pi = f"The value of pi is approximately {pi:.2f}" print(formatted_pi)
Output:
The value of pi is approximately 3.14
In this example, the ‘.2f’ format specifier is used to round the value of pi
to 2 decimal places.
String interpolation is a powerful feature in Python that simplifies the process of constructing dynamic strings. It offers flexibility and readability, making your code more concise and maintainable. Choose the method that suits your needs and coding style, and start using string interpolation in your Python projects.
Related Article: How To Set Environment Variables In Python
Escaping Characters in Strings
There are certain characters that have special meanings when used within a string. These characters include the backslash (), single quote (‘), and double quote (“). To include these special characters in a string, you need to escape them using the backslash.
For example, let’s say you want to include a backslash in a string. If you simply write “\” in your string, Python will interpret it as a single backslash. Here’s an example:
my_string = "This is a backslash: \" print(my_string)
Output:
This is a backslash:
Similarly, if you want to include a single quote or double quote in a string, you can escape them using the backslash. Here’s an example:
my_string = 'I'm using a single quote' print(my_string) my_string = "He said, "Hello!"" print(my_string)
Output:
I'm using a single quote He said, "Hello!"
Python also provides a way to represent special characters using escape sequences. For example, if you want to include a newline character in a string, you can use the escape sequence “n”. Here’s an example:
my_string = "This is a multi-line stringnwith a newline character" print(my_string)
Output:
This is a multi-line string with a newline character
Similarly, you can use other escape sequences like “t” for a tab character and “r” for a carriage return character.
It’s important to note that when using escape sequences, you need to be careful with how they are interpreted. For example, if you want to include a backslash followed by a letter, Python may interpret it as a special sequence. To avoid this, you can use raw strings by prefixing the string with the letter ‘r’. Here’s an example:
my_string = r"This is a raw stringn" print(my_string)
Output:
This is a raw stringn
In this example, the escape sequence “n” is not interpreted as a newline character because the string is marked as a raw string.
Understanding how to escape characters in strings is crucial when dealing with certain scenarios, such as when you need to include special characters or control characters in your strings. By using escape sequences or raw strings, you can ensure that your strings are interpreted correctly by Python.
Using Raw Strings
A raw string is a string that is prefixed with an ‘r’ character. It is used to represent string literals without having to escape special characters such as backslashes.
Let’s see an example to better understand the concept of raw strings. Suppose we have a string that contains a file path:
path = "C:\Users\John\Documents\file.txt"
In the above example, we have used double backslashes to escape the special characters. However, we can simplify this by using a raw string:
path = r"C:UsersJohnDocumentsfile.txt"
By using the ‘r’ prefix, Python treats the backslashes as literal characters and does not interpret them as escape sequences. This makes raw strings particularly useful when dealing with file paths, regular expressions, or any other strings that contain a large number of backslashes.
Another advantage of using raw strings is when working with regular expressions. Regular expressions often contain many backslashes, which can be cumbersome to write and read when using normal strings. By using raw strings, we can write regular expressions more concisely:
import re pattern = r"d{3}-d{3}-d{4}" result = re.match(pattern, "123-456-7890")
In the above example, the regular expression pattern is defined as a raw string using the ‘r’ prefix. This makes it easier to write and understand the regular expression pattern.
It is worth noting that raw strings still support escape sequences for special characters such as newline (‘n’) or tab (‘t’). For example:
message = r"HellonWorld!" print(message)
Output:
HellonWorld!
In the above example, the newline character ‘n’ is treated as a literal character and not as a newline character.
Raw strings provide a convenient way to represent string literals without the need to escape special characters. They are particularly useful when dealing with file paths, regular expressions, or any other strings that contain a large number of backslashes.