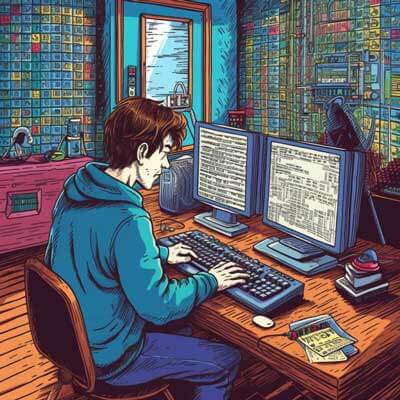
Rounding numbers to a specific number of decimal places is a common task in Python. There are several ways to achieve this in Python, depending on the desired output format and the specific requirements of your application. In this article, we will explore different approaches to rounding numbers to two decimal places in Python.
1. Using the round() function
The simplest way to round a number to two decimal places in Python is by using the built-in round()
function. This function takes two arguments: the number you want to round, and the number of decimal places to round to. Here’s an example:
number = 3.14159 rounded_number = round(number, 2) print(rounded_number) # Output: 3.14
In this example, we round the number 3.14159
to two decimal places by passing it as the first argument to the round()
function, and specifying 2
as the second argument. The resulting rounded number, 3.14
, is then printed to the console.
Related Article: String Comparison in Python: Best Practices and Techniques
2. Using string formatting
Another approach to rounding numbers to two decimal places in Python is by using string formatting. This method allows you to control the formatting of the rounded number, including the number of decimal places. Here’s an example:
number = 3.14159 rounded_number = "%.2f" % number print(rounded_number) # Output: 3.14
In this example, we use the string formatting syntax "%.2f"
to specify that we want to format the number with two decimal places. The %
operator is used to substitute the value of the number
variable into the string format. The resulting formatted string, "3.14"
, is then printed to the console.
This method can be useful when you need more control over the formatting of the rounded number, such as when you want to display it as a string in a specific format.
Why is rounding to two decimal places important?
Rounding numbers to two decimal places is important in many applications where precision is required, such as financial calculations, scientific calculations, or when presenting data to users. In these cases, it is often necessary to round numbers to a specific number of decimal places to ensure consistency and accuracy in the results.
For example, when performing financial calculations, rounding to two decimal places is commonly used to represent currency values. This helps to avoid rounding errors and ensures that calculations involving currency are accurate and consistent.
Similarly, in scientific calculations, rounding to a specific number of decimal places is important to maintain the desired level of precision and avoid introducing errors into the calculations.
Alternative ideas and suggestions
While rounding to two decimal places is a common requirement, there may be cases where you need to round to a different number of decimal places. In Python, you can easily adapt the approaches mentioned above to round to a different number of decimal places by changing the second argument of the round()
function or the format specifier in the string formatting method.
For example, to round a number to three decimal places using the round()
function, you can simply change the second argument to 3
:
number = 3.14159 rounded_number = round(number, 3) print(rounded_number) # Output: 3.142
Similarly, to round a number to three decimal places using string formatting, you can change the format specifier to "%.3f"
:
number = 3.14159 rounded_number = "%.3f" % number print(rounded_number) # Output: 3.142
By adjusting the number of decimal places, you can round numbers to the desired level of precision in your application.
Related Article: How To Limit Floats To Two Decimal Points In Python
Best practices
When rounding numbers in Python, it is important to be aware of potential rounding errors and the limitations of floating-point arithmetic. Due to the inherent nature of floating-point numbers, rounding errors can occur when performing calculations involving decimal numbers.
To mitigate rounding errors, it is recommended to use the decimal module in Python for precise decimal arithmetic. The decimal module provides a Decimal class that allows you to perform decimal arithmetic with arbitrary precision.
Here’s an example of rounding a number to two decimal places using the decimal module:
from decimal import Decimal, ROUND_HALF_UP number = Decimal("3.14159") rounded_number = number.quantize(Decimal("0.00"), rounding=ROUND_HALF_UP) print(rounded_number) # Output: 3.14
In this example, we create a Decimal object using the string representation of the number "3.14159"
. We then use the quantize()
method to round the number to two decimal places, using the rounding mode ROUND_HALF_UP
. The resulting rounded number, 3.14
, is then printed to the console.
Using the decimal module can help ensure the accuracy and precision of decimal calculations, especially in applications where precision is critical.