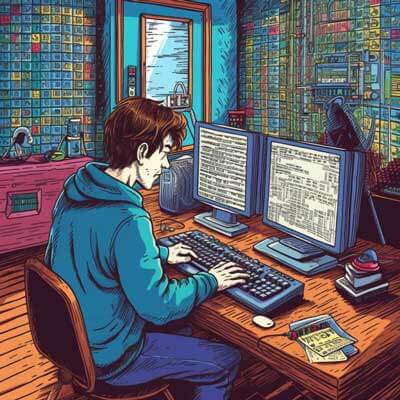
To list all the files in a directory using Python, you can use the os
module, which provides a way to interact with the operating system. Specifically, you can use the os.listdir()
function to retrieve a list of all the files and directories within a given directory.
Here are two possible ways to list all the files of a directory in Python:
Method 1: Using os.listdir()
The os.listdir()
function returns a list containing the names of all the files and directories within a specified directory. To list only the files, you can filter out the directories using the os.path.isfile()
function.
Here’s an example:
import os def list_files(directory): files = [] for filename in os.listdir(directory): if os.path.isfile(os.path.join(directory, filename)): files.append(filename) return files # Example usage directory = '/path/to/directory' file_list = list_files(directory) print(file_list)
In this example, the list_files()
function takes a directory path as input and returns a list of file names within that directory. The os.listdir()
function is used to retrieve all the names within the specified directory. The os.path.isfile()
function is then used to check if each name corresponds to a file or a directory. Only the file names are added to the files
list, which is then returned.
Related Article: How To Limit Floats To Two Decimal Points In Python
Method 2: Using os.scandir()
Starting from Python 3.5, the os.scandir()
function provides a more efficient and convenient way to list files and directories. It returns an iterator of DirEntry
objects, which provide more information about each entry, such as file attributes and file type.
Here’s an example:
import os def list_files(directory): files = [] with os.scandir(directory) as entries: for entry in entries: if entry.is_file(): files.append(entry.name) return files # Example usage directory = '/path/to/directory' file_list = list_files(directory) print(file_list)
In this example, the list_files()
function works similarly to the previous method, but instead of using os.listdir()
, it uses os.scandir()
. The with
statement is used to automatically close the directory after iterating through its entries. The entry.is_file()
method is used to check if each entry corresponds to a file.
Reasons for Asking
The question of how to list all files of a directory in Python may arise for various reasons. Some potential reasons include:
– Needing to process or analyze a large number of files within a directory.
– Implementing a file management or organization system.
– Automating a task that involves working with files in a specific directory.
Suggestions and Alternative Ideas
When listing all files of a directory in Python, you may also consider the following suggestions and alternative ideas:
– Filtering the files based on specific criteria: You can modify the code to filter the files based on specific file extensions, file sizes, or other criteria. This can be done by adding additional conditions within the if
statement.
– Sorting the file list: If you want the file list to be sorted, you can use the sorted()
function to sort the files
list before returning it. For example, return sorted(files)
will return the file list in alphabetical order.
– Recursive listing: If you need to list all files in a directory and its subdirectories recursively, you can use a recursive function that traverses the directory tree. This can be achieved by combining the os.walk()
function with the methods mentioned in the previous methods.
Related Article: How To Rename A File With Python
Best Practices
When listing all files of a directory in Python, it is good practice to:
– Use the os.path
module: The os.path
module provides functions for working with file paths, such as joining paths using os.path.join()
or checking if a path exists using os.path.exists()
. Utilizing these functions can make your code more robust and platform-independent.
– Handle exceptions: When working with directories and files, it is important to handle potential exceptions that may occur, such as PermissionError
or FileNotFoundError
. You can use a try-except
block to catch and handle these exceptions appropriately.
– Use context managers: When working with file-related operations, such as opening or iterating through files or directories, it is recommended to use context managers, such as the with
statement. Context managers ensure that resources are released properly, even if an exception occurs.