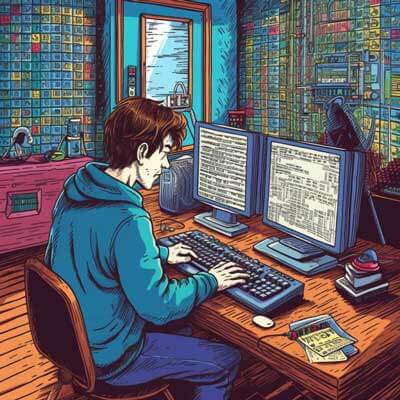
In Python, you can use inline if statements, also known as the ternary operator, to conditionally print values. The inline if statement has the following syntax:
value_if_true if condition else value_if_false
Here’s an example that demonstrates the usage of inline if statements for print:
x = 10 print("x is greater than 5") if x > 5 else print("x is less than or equal to 5")
This code will output “x is greater than 5” if x
is greater than 5, and “x is less than or equal to 5” otherwise.
Inline if statements are particularly useful when you want to print different values based on a condition without writing multiple if-else statements. They provide a concise and readable way to conditionally print values.
Multiple Inline If Statements
You can also chain multiple inline if statements together to handle more complex conditions. Here’s an example:
x = 10 print("x is greater than 5") if x > 5 else print("x is less than or equal to 5") if x < 5 else print("x is equal to 5")
In this example, the first inline if statement checks if x
is greater than 5. If it is, it prints "x is greater than 5". Otherwise, it moves to the next inline if statement, which checks if x
is less than 5. If it is, it prints "x is less than or equal to 5". Finally, if neither condition is true, it prints "x is equal to 5".
Related Article: How To Limit Floats To Two Decimal Points In Python
Using Inline If Statements with String Formatting
You can also use inline if statements in combination with string formatting to conditionally include values in a formatted string. Here’s an example:
x = 10 message = f"The value of x is {x} and it is {'greater than 5' if x > 5 else 'less than or equal to 5'}" print(message)
In this example, the inline if statement is used within the f-string to conditionally include the message “greater than 5” if x
is greater than 5, or “less than or equal to 5” otherwise. The resulting message will be printed accordingly.
Using inline if statements with string formatting allows you to dynamically construct strings based on conditions, making your code more flexible and concise.
Best Practices
When using inline if statements for print in Python, consider the following best practices:
1. Keep the code readable: While inline if statements can make your code more concise, avoid nesting too many conditions or writing complex expressions. This can make the code harder to understand and maintain. If your condition becomes too complex, consider using traditional if-else statements instead.
2. Use parentheses for clarity: When using inline if statements in complex expressions, it’s a good practice to enclose the condition within parentheses. This helps improve readability and avoids unexpected behavior due to operator precedence.
result = (value1 if condition1 else value2) + (value3 if condition2 else value4)
In this example, the use of parentheses makes it clear that the two inline if statements are separate conditions that are combined using the +
operator.
3. Consider using named variables: If the values to be printed are complex or require computation, consider assigning them to named variables before using them in the inline if statement. This can improve code readability and make it easier to debug.
x = 10 is_greater_than_5 = x > 5 message = "x is greater than 5" if is_greater_than_5 else "x is less than or equal to 5" print(message)
Related Article: How To Rename A File With Python