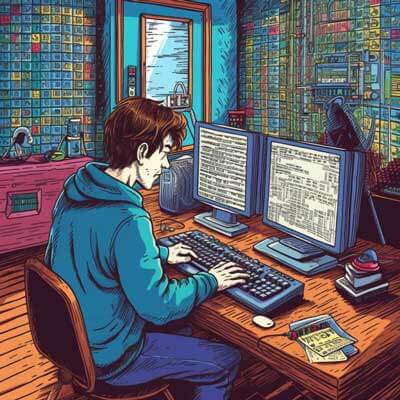
Printing a Python dictionary line by line can be achieved by iterating over the dictionary and printing each key-value pair on a separate line. There are multiple approaches to accomplish this task, and in this answer, we will explore two possible solutions.
Using a for loop
One way to print a Python dictionary line by line is to use a for loop to iterate over the dictionary items. Here’s an example:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} for key, value in my_dict.items(): print(key, value)
This code snippet uses the items()
method to retrieve the key-value pairs of the dictionary. The for
loop then iterates over each pair, assigning the key to the variable key
and the value to the variable value
. The print()
function is used to display the key-value pairs on separate lines.
The output of the above code will be:
key1 value1 key2 value2 key3 value3
This approach allows you to easily print the dictionary line by line, displaying both the keys and their corresponding values.
Related Article: How To Convert a Dictionary To JSON In Python
Using a list comprehension
Another way to print a Python dictionary line by line is to use a list comprehension in combination with the join()
method. Here’s an example:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} lines = [f'{key} {value}' for key, value in my_dict.items()] print('\n'.join(lines))
In this code snippet, a list comprehension is used to create a list of strings, where each string represents a key-value pair from the dictionary. The f-string syntax is used to format each pair as key value
. The join()
method is then used to concatenate the strings with a newline character (\n
), resulting in the desired output.
The output of the above code will be the same as the previous example:
key1 value1 key2 value2 key3 value3
Using a list comprehension can be a concise and efficient way to print a Python dictionary line by line.
Alternative ideas
– If you only want to print the keys or values of the dictionary, you can modify the code accordingly. For example, to print only the keys, you can replace print(key, value)
with print(key)
in the first solution. Similarly, to print only the values, you can use print(value)
instead.
– If you prefer a more formatted output, you can use string formatting techniques to align the keys and values. For example, you can use the str.ljust()
method to left-justify the keys and values within a fixed width.
– You can also explore third-party libraries such as pprint
(pretty-print) or tabulate
for more advanced printing options, especially if you are dealing with larger or nested dictionaries.
Keep in mind that dictionaries in Python are unordered, so the order of the key-value pairs may not necessarily match the order of insertion. If you need to preserve the order, consider using an ordered dictionary from the collections
module.
These are just a few examples of how you can print a Python dictionary line by line. Depending on your specific requirements and preferences, you may choose one of these approaches or explore other techniques.
Related Article: How to Sort a Dictionary by Key in Python