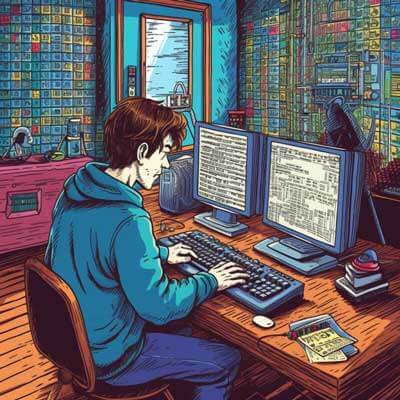
Table of Contents
To find a value in a Python list, you can use various approaches. In this answer, we will explore two common methods: using the index()
method and using a loop to iterate through the list.
Using the index() Method
The index()
method in Python returns the index of the first occurrence of a specified value in a list. Here's how you can use it:
my_list = [10, 20, 30, 40, 50] # Find the index of a specific value value_to_find = 30 index = my_list.index(value_to_find) print(f"The value {value_to_find} is found at index {index}")
Output:
The value 30 is found at index 2
If the value you are searching for is not present in the list, the index()
method will raise a ValueError
. To handle this, you can use a try-except block:
my_list = [10, 20, 30, 40, 50] # Find the index of a specific value value_to_find = 60 try: index = my_list.index(value_to_find) print(f"The value {value_to_find} is found at index {index}") except ValueError: print(f"The value {value_to_find} is not present in the list")
Output:
The value 60 is not present in the list
Related Article: How to Use Python's Not Equal Operator
Using a Loop
Another approach to find a value in a Python list is by using a loop to iterate through the elements. Here's an example:
my_list = [10, 20, 30, 40, 50] # Find the index of a specific value using a loop value_to_find = 30 index = None for i, value in enumerate(my_list): if value == value_to_find: index = i break if index is not None: print(f"The value {value_to_find} is found at index {index}") else: print(f"The value {value_to_find} is not present in the list")
Output:
The value 30 is found at index 2
This loop iterates through each element of the list and checks if the value matches the one we are searching for. If a match is found, the loop breaks and the index is stored in the index
variable. If no match is found, the index
variable remains None
.
Alternative Ideas and Best Practices
Related Article: How to Import Files From a Different Folder in Python
- If you want to find all occurrences of a value in a list, you can use list comprehension or a loop to iterate through the list and collect the indices where the value is found. Here's an example using list comprehension:
my_list = [10, 20, 30, 20, 40, 50, 20] # Find all indices of a specific value using list comprehension value_to_find = 20 indices = [i for i, value in enumerate(my_list) if value == value_to_find] print(f"The value {value_to_find} is found at indices: {indices}")
Output:
The value 20 is found at indices: [1, 3, 6]
- If you are working with a large list or need to perform frequent searches, you can consider using a set instead of a list. Sets offer faster lookups as they use hash-based indexing.
- When searching for a value in a list, keep in mind that the index()
method and loop-based approaches have different time complexities. The index()
method has a time complexity of O(n), where n is the length of the list. The loop-based approach has a time complexity of O(n) in the worst case, but it can be more efficient if you only need to find the first occurrence of the value.
- If you are working with nested lists or complex data structures, you can use recursion to search for a value recursively.
- Be cautious when using the index()
method or a loop to find a value in a list that may contain duplicate values. These methods will only return the index of the first occurrence. If you need to find all occurrences, consider using alternative approaches like list comprehension or a loop with a counter.