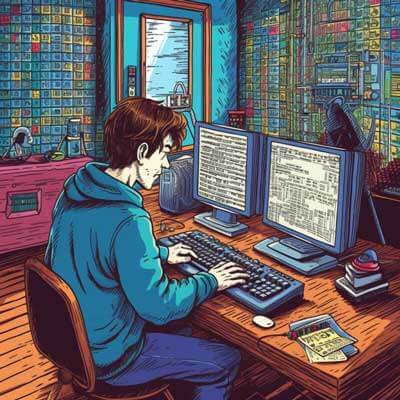
Table of Contents
A KeyError
in Python is an exception that occurs when trying to access a dictionary key that does not exist. This error is commonly encountered when working with dictionaries or other data structures that use key-value pairs. Here are a few ways to solve a KeyError
in Python:
1. Check if the key exists before accessing it
One way to avoid a KeyError
is by checking if the key exists in the dictionary before trying to access it. This can be done using the in
operator or the get()
method.
Using the in
operator:
my_dict = {'key1': 'value1', 'key2': 'value2'} if 'key3' in my_dict: print(my_dict['key3']) else: print('Key does not exist')
Using the get()
method:
my_dict = {'key1': 'value1', 'key2': 'value2'} value = my_dict.get('key3', 'Key does not exist') print(value)
Related Article: Diphthong Detection Methods in Python
2. Use a default value with the get()
method
The get()
method of a dictionary allows you to specify a default value that will be returned if the key does not exist. This can be useful when you want to handle a missing key gracefully without raising a KeyError
.
my_dict = {'key1': 'value1', 'key2': 'value2'} value = my_dict.get('key3', 'Default value') print(value)
3. Use a try-except block to catch the KeyError
Another approach to handle a KeyError
is by using a try-except block to catch the exception and handle it gracefully. This allows you to perform specific actions or provide alternative values when a key is not found.
my_dict = {'key1': 'value1', 'key2': 'value2'} try: print(my_dict['key3']) except KeyError: print('Key does not exist')
4. Check if the key exists using the keys()
method
You can also check if a key exists in a dictionary by using the keys()
method, which returns a view object containing all the keys in the dictionary. This can be useful when you want to perform additional operations on the keys before accessing them.
my_dict = {'key1': 'value1', 'key2': 'value2'} if 'key3' in my_dict.keys(): print(my_dict['key3']) else: print('Key does not exist')
Related Article: How to Execute a Curl Command Using Python
Best Practices
- Always check if a key exists before accessing it to avoid KeyError
exceptions.
- Use the in
operator or the get()
method to check for key existence.
- Consider using a default value with the get()
method to handle missing keys gracefully.
- Use try-except blocks to catch KeyError
exceptions and handle them appropriately.
- Avoid assuming that a key exists without checking, as this can lead to unexpected errors.