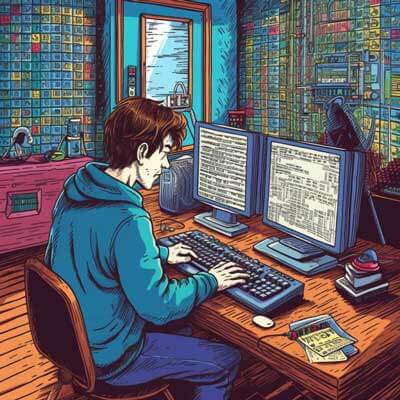
Adding new keys to a Python dictionary is a common operation when working with dictionaries in Python. A dictionary is a built-in data type that allows you to store a collection of key-value pairs. Each key in a dictionary must be unique, and the keys are used to access the corresponding values.
Here are two possible ways to add new keys to a Python dictionary:
Method 1: Using the assignment operator (=)
One way to add a new key to a Python dictionary is by using the assignment operator (=). You can assign a value to a new key by specifying the key inside square brackets and assigning a value to it.
Here’s an example:
# Create an empty dictionary my_dict = {} # Add a new key-value pair my_dict['key1'] = 'value1' # Print the dictionary print(my_dict)
Output:
{'key1': 'value1'}
In this example, we first create an empty dictionary called my_dict
. We then add a new key 'key1'
with the corresponding value 'value1'
using the assignment operator. Finally, we print the dictionary to verify the key-value pair has been added.
Related Article: How To Convert a Dictionary To JSON In Python
Method 2: Using the update() method
Another way to add new keys to a Python dictionary is by using the update()
method. The update()
method allows you to add multiple key-value pairs to a dictionary at once.
Here’s an example:
# Create an empty dictionary my_dict = {} # Add multiple key-value pairs my_dict.update({'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}) # Print the dictionary print(my_dict)
Output:
{'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
In this example, we first create an empty dictionary called my_dict
. We then use the update()
method to add multiple key-value pairs to the dictionary. The key-value pairs are specified as a dictionary inside the update()
method. Finally, we print the dictionary to verify the key-value pairs have been added.
Best practices and additional considerations
– When adding new keys to a dictionary, make sure the keys are unique. If you try to add a key that already exists, the existing value will be replaced with the new value.
– If you want to add a key-value pair only if the key doesn’t already exist in the dictionary, you can use the setdefault()
method. The setdefault()
method sets the value of a key if it doesn’t exist, and returns the value of the key if it does exist. This can be useful in certain scenarios where you want to avoid overwriting existing values.
– If you have a large number of key-value pairs to add to a dictionary, consider using a loop or a list comprehension to automate the process. This can help make your code more concise and easier to maintain.
– Keep in mind that dictionaries in Python are unordered, meaning the order of the key-value pairs may not be preserved. If you need to maintain a specific order, you can use an OrderedDict from the collections
module.
Overall, adding new keys to a Python dictionary is a straightforward operation that can be done using the assignment operator or the update()
method. By following these methods and considering best practices, you can easily add new keys and values to your dictionaries in Python.
Related Article: How to Sort a Dictionary by Key in Python