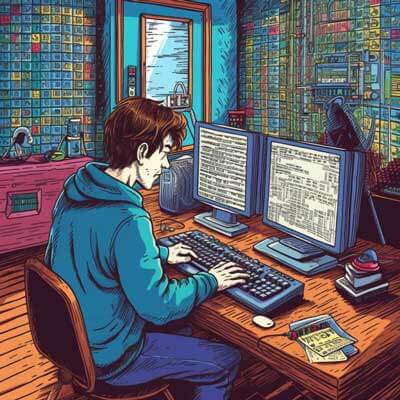
Removing duplicates from a list is a common task in Python programming. There are multiple ways to achieve this, depending on your requirements and the characteristics of the list. In this answer, we will explore two popular and efficient methods to remove duplicates from lists in Python.
Method 1: Using the set() Function
One of the simplest and most straightforward ways to remove duplicates from a list is by converting it to a set. The set data structure in Python does not allow duplicate elements, so converting the list to a set will automatically remove any duplicates. After removing the duplicates, you can convert the set back to a list if needed.
Here’s an example that demonstrates how to use the set() function to remove duplicates from a list:
my_list = [1, 2, 3, 4, 2, 3, 5, 6, 4, 7, 8, 5] unique_list = list(set(my_list)) print(unique_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8]
In this example, we have a list called my_list
that contains duplicate elements. By converting it to a set using the set()
function and then back to a list using the list()
function, we obtain a new list called unique_list
that contains only the unique elements from the original list.
It’s important to note that the order of the elements may change when converting a list to a set and back to a list, as sets do not preserve the order of elements. If you need to preserve the order of the elements, you can use the second method described below.
Related Article: How To Convert a Dictionary To JSON In Python
Method 2: Using a List Comprehension
Another popular method to remove duplicates from a list is by using a list comprehension. List comprehensions provide a concise and efficient way to create new lists based on existing lists. By iterating over the original list and only adding elements to the new list if they have not been added before, we can effectively remove duplicates.
Here’s an example that demonstrates how to use a list comprehension to remove duplicates from a list while preserving the order of the elements:
my_list = [1, 2, 3, 4, 2, 3, 5, 6, 4, 7, 8, 5] unique_list = [] [unique_list.append(x) for x in my_list if x not in unique_list] print(unique_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8]
In this example, we initialize an empty list called unique_list
. The list comprehension iterates over each element x
in the original list my_list
. If the element is not already present in unique_list
, it gets appended to the list. This ensures that only unique elements are added to the new list.
Using a list comprehension can be more memory-efficient compared to converting a list to a set if the original list is large, as it avoids creating a temporary set in memory. However, it may be slightly slower for smaller lists due to the additional check for element presence.
Additional Considerations
– If your list contains mutable objects like lists or dictionaries, the above methods will only remove duplicates based on the object’s identity, not its contents. If you need to remove duplicates based on the contents of mutable objects, you can convert them to immutable objects like tuples before applying the above methods.
– If you want to remove duplicates from a list without changing its order and also preserve the original list, you can create a copy of the list and apply either of the above methods to the copy.
– If you need to remove duplicates from a list while preserving the order and also count the number of occurrences of each element, you can use the collections.Counter
class from the Python standard library.
Related Article: How to Sort a Dictionary by Key in Python