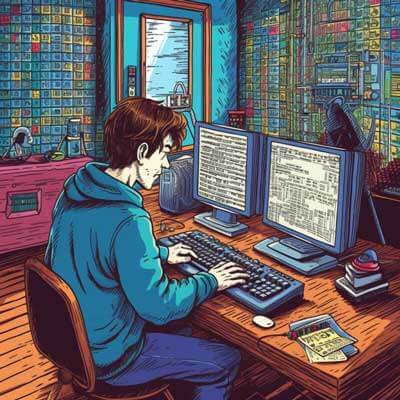
Table of Contents
To find the index of an item in a Python list, you can use the index()
method or a list comprehension. This is a common task when working with lists in Python and can be useful in various scenarios, such as searching for a specific element or determining the position of an item within a list. In this guide, we will explore both methods and provide examples to demonstrate their usage.
Using the index() method
The index()
method is a built-in function in Python that allows you to find the index of an item in a list. It takes a value as an argument and returns the index of the first occurrence of that value in the list. If the value is not found, it raises a ValueError
.
Here is the syntax for using the index()
method:
index(value, start, end)
- value
: The value to search for in the list.
- start
(optional): The index to start searching from. Defaults to the beginning of the list.
- end
(optional): The index to stop searching at (exclusive). Defaults to the end of the list.
Let's see an example:
fruits = ['apple', 'banana', 'orange', 'grape'] index = fruits.index('banana') print(index)
Output:
1
In this example, we have a list of fruits and we want to find the index of the item 'banana'. The index()
method returns the index of the first occurrence of 'banana', which is 1. If the value is not found in the list, a ValueError
will be raised.
Related Article: How to Adjust Font Size in a Matplotlib Plot
Using a list comprehension
Another way to find the index of an item in a Python list is by using a list comprehension. A list comprehension allows you to create a new list based on an existing list, applying a condition to filter the elements. In this case, we can use a list comprehension to filter the elements that match the value we are searching for and then retrieve their indices.
Here is an example:
fruits = ['apple', 'banana', 'orange', 'grape'] indices = [i for i, x in enumerate(fruits) if x == 'banana'] print(indices)
Output:
[1]
In this example, we iterate over the elements of the fruits
list using the enumerate()
function, which returns both the index and the value of each element. We then use a conditional statement (if x == 'banana'
) to filter the elements that match the value we are searching for. Finally, we retrieve the indices of the matching elements using the list comprehension.
Why is the question asked?
The question of how to find the index of an item in a Python list is commonly asked by developers who need to retrieve the position of an element within a list. This information can be useful in various scenarios, such as:
- Updating or deleting specific elements in a list based on their index.
- Sorting a list based on a specific criterion and then accessing the elements in their original order.
- Implementing algorithms or data structures that require knowledge of the position of elements within a list.
By understanding how to find the index of an item in a Python list, developers can perform these operations more efficiently and accurately.
Suggestions and alternative ideas
While the index()
method and list comprehensions are the most straightforward ways to find the index of an item in a Python list, there are alternative approaches that can be used depending on the specific requirements of the task at hand. Here are a few suggestions and alternative ideas:
- Using the find()
method: If you are working with a string list, you can use the find()
method to search for a specific substring within each element. This method returns the index of the first occurrence of the substring in the string, or -1 if it is not found. However, note that this method only works for string lists and not for lists of other data types.
- Handling multiple occurrences: Both the index()
method and list comprehensions return the index of the first occurrence of the value in the list. If you need to find the indices of all occurrences, you can modify the list comprehension approach by returning a list of tuples containing the index and value pairs. For example:
fruits = ['apple', 'banana', 'orange', 'banana'] indices = [(i, x) for i, x in enumerate(fruits) if x == 'banana'] print(indices)
Output:
[(1, 'banana'), (3, 'banana')]
In this modified example, we have a list with multiple occurrences of the value 'banana'. By returning a list of tuples instead of just the indices, we can also retrieve the corresponding values for each occurrence.
- Handling missing values: If you are uncertain whether the value you are searching for exists in the list, you can use a try-except block to handle the ValueError
raised by the index()
method. This allows you to gracefully handle scenarios where the value is not found, without interrupting the execution of your program.
fruits = ['apple', 'banana', 'orange', 'grape'] value = 'pear' try: index = fruits.index(value) print(index) except ValueError: print(f"The value '{value}' was not found in the list.")
Output:
The value 'pear' was not found in the list.
In this example, we attempt to find the index of the value 'pear' in the fruits
list. Since 'pear' is not present in the list, a ValueError
is raised. By using a try-except block, we catch the exception and handle it by printing a custom error message.
Related Article: Converting Integer Scalar Arrays To Scalar Index In Python
Best practices
When finding the index of an item in a Python list, it is important to keep the following best practices in mind:
- Check if the value exists: Before using the index()
method or a list comprehension, it is a good practice to check if the value you are looking for exists in the list. This can be done using the in
operator or the count()
method. By ensuring that the value is present, you can avoid potential ValueError
exceptions.
- Handle potential errors: Since the index()
method raises a ValueError
when the value is not found in the list, it is important to handle this exception to prevent your program from crashing. This can be done using a try-except block, as shown in the previous example.
- Consider performance implications: Both the index()
method and list comprehensions have a time complexity of O(n), where n is the length of the list. If you need to find the index of an item multiple times or in large lists, consider using alternative data structures, such as dictionaries or sets, that offer faster lookup times.
- Be mindful of data types: The index()
method and list comprehensions work with any data type that is comparable for equality. However, keep in mind that certain data types, such as floating-point numbers, may introduce precision issues when comparing for equality. In such cases, consider using tolerance thresholds or custom comparison functions to handle these scenarios.
Overall, understanding how to find the index of an item in a Python list is a fundamental skill for any Python developer. By utilizing the index()
method or list comprehensions, developers can efficiently retrieve the position of elements within a list and perform various operations based on this information.