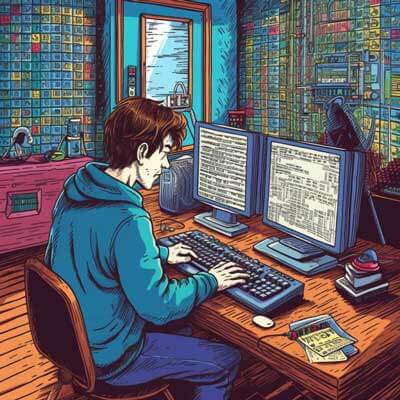
Flattening a list of lists is a common operation in Python, and there are several ways to achieve this. In this answer, we will explore two popular methods: using nested list comprehensions and using the itertools.chain.from_iterable()
function.
Method 1: Nested List Comprehensions
One way to flatten a list of lists in Python is by using nested list comprehensions. This method is concise and efficient, making it a popular choice among Python developers.
Here’s an example of how to use nested list comprehensions to flatten a list of lists:
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] flattened_list = [item for sublist in nested_list for item in sublist] print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In the example above, we have a nested list nested_list
containing three sublists. The nested list comprehension item for sublist in nested_list for item in sublist
iterates over each sublist in nested_list
and then iterates over each item in the sublist, effectively flattening the list.
Related Article: How To Convert a Dictionary To JSON In Python
Method 2: itertools.chain.from_iterable()
Another way to flatten a list of lists in Python is by using the itertools.chain.from_iterable()
function from the itertools
module. This method is more explicit and readable, especially for larger and more complex lists.
Here’s an example of how to use itertools.chain.from_iterable()
to flatten a list of lists:
import itertools nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] flattened_list = list(itertools.chain.from_iterable(nested_list)) print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In the example above, we import the itertools
module and then use the chain.from_iterable()
function to flatten the nested_list
. The function chain.from_iterable()
takes the nested list as an argument and returns an iterator that produces the flattened list. We convert the iterator to a list using the list()
function for easier printing and manipulation.
Best Practices
When flattening a list of lists in Python, consider the following best practices:
1. Use nested list comprehensions for simple and concise code. This method is ideal for small to medium-sized lists.
2. Use itertools.chain.from_iterable()
for larger or more complex lists. This method is more explicit and allows for easier customization or modification.
3. If you only need to iterate over the flattened list and don’t need a new list object, consider using a generator expression instead of a list comprehension. This can save memory and improve performance, especially for large lists.
4. If you need to flatten a list of lists to a specific depth, consider using a recursive function or a third-party library like flatten-list
.
Related Article: How to Sort a Dictionary by Key in Python