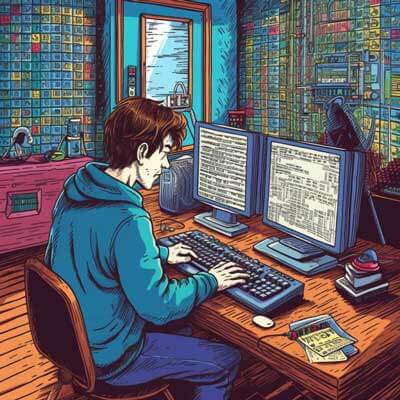
There are multiple ways to extract the file name from a path in Python, regardless of the operating system. Here are two possible approaches:
Using the os module
The os
module in Python provides a platform-independent way to work with operating system functionalities. To extract the file name from a path, you can make use of the os.path
module, which provides functions for manipulating file paths.
Here’s an example of how you can extract the file name from a path using the os.path
module:
import os path = '/path/to/file.txt' file_name = os.path.basename(path) print(file_name) # Output: file.txt
In the example above, we import the os
module and assign the file path to the path
variable. Then, we use the os.path.basename()
function to extract the file name from the path. Finally, we print the file name, which will be 'file.txt'
.
Related Article: How To Convert a Dictionary To JSON In Python
Using the pathlib module
The pathlib
module introduced in Python 3 provides an object-oriented approach for working with file system paths. It offers an easier and more intuitive way to manipulate paths compared to the os.path
module.
Here’s an example of how you can extract the file name from a path using the pathlib
module:
from pathlib import Path path = '/path/to/file.txt' file_name = Path(path).name print(file_name) # Output: file.txt
In the example above, we import the Path
class from the pathlib
module and assign the file path to the path
variable. Then, we create a Path
object with the path and use the name
attribute to extract the file name. Finally, we print the file name, which will be 'file.txt'
.
Alternative Ideas
– Regular expressions: If the file name extraction requires more complex pattern matching, regular expressions can be used. The re
module in Python provides functions for working with regular expressions.
– String manipulation: If the file name extraction is based on a specific pattern or delimiter, you can use string manipulation techniques, such as splitting the path string based on a delimiter or using string slicing.
Best Practices
– When working with file paths, it is recommended to use the os.path
module or the pathlib
module instead of manually manipulating strings. These modules handle platform-specific path separators and provide a more reliable and consistent way to work with file paths.
– If you need to extract both the file name and the file extension from a path, you can use the os.path.splitext()
function (from the os.path
module) or the Path.suffix
attribute (from the pathlib
module).
Here’s an example using os.path.splitext()
:
import os path = '/path/to/file.txt' file_name, file_extension = os.path.splitext(os.path.basename(path)) print(file_name) # Output: file print(file_extension) # Output: .txt
And here’s an example using Path.suffix
:
from pathlib import Path path = '/path/to/file.txt' file_name = Path(path).stem file_extension = Path(path).suffix print(file_name) # Output: file print(file_extension) # Output: .txt
In both examples, we extract both the file name and the file extension from the path.
Related Article: How to Sort a Dictionary by Key in Python