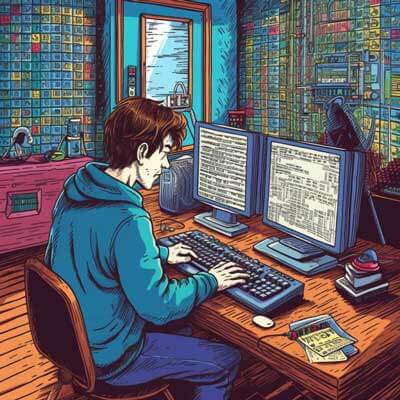
Removing an element from a list by its index is a common operation in Python programming. In this guide, we will explore different ways to remove elements from a list using their index.
Method 1: Using the ‘del’ keyword
One straightforward way to remove an element from a list by index is by using the ‘del’ keyword. The ‘del’ keyword allows us to delete an element or a slice from a list.
Here’s the syntax to remove an element from a list by index using ‘del’:
del list_name[index]
Let’s see an example:
# Define a list my_list = [10, 20, 30, 40, 50] # Remove the element at index 2 del my_list[2] print(my_list) # Output: [10, 20, 40, 50]
In the above example, the element at index 2 (value 30) is removed from the list using the ‘del’ keyword. The resulting list is [10, 20, 40, 50].
Related Article: How To Convert a Dictionary To JSON In Python
Method 2: Using the ‘pop()’ method
Another method to remove an element from a list by index is by using the ‘pop()’ method. The ‘pop()’ method not only removes the element at the specified index but also returns the removed element.
Here’s the syntax to remove an element from a list by index using ‘pop()’:
list_name.pop(index)
Let’s see an example:
# Define a list my_list = [10, 20, 30, 40, 50] # Remove and retrieve the element at index 2 removed_element = my_list.pop(2) print(my_list) # Output: [10, 20, 40, 50] print(removed_element) # Output: 30
In the above example, the element at index 2 (value 30) is removed from the list using the ‘pop()’ method. The resulting list is [10, 20, 40, 50], and the removed element (30) is stored in the variable ‘removed_element’.
Method 3: Using the ‘remove()’ method
If you know the value of the element you want to remove, you can use the ‘remove()’ method to remove it from the list. However, unlike the previous methods, the ‘remove()’ method removes the first occurrence of the specified element rather than a specific index.
Here’s the syntax to remove an element from a list using ‘remove()’:
list_name.remove(element_value)
Let’s see an example:
# Define a list my_list = [10, 20, 30, 40, 50, 30] # Remove the first occurrence of the element with value 30 my_list.remove(30) print(my_list) # Output: [10, 20, 40, 50, 30]
In the above example, the first occurrence of the element with value 30 is removed from the list using the ‘remove()’ method. The resulting list is [10, 20, 40, 50, 30].
Method 4: Using list comprehension
List comprehension is a concise and useful way to manipulate lists in Python. It also provides a way to remove elements from a list based on their index.
Here’s an example of removing an element from a list by index using list comprehension:
# Define a list my_list = [10, 20, 30, 40, 50] # Remove the element at index 2 using list comprehension my_list = [x for i, x in enumerate(my_list) if i != 2] print(my_list) # Output: [10, 20, 40, 50]
In the above example, list comprehension is used to iterate over the elements of the list and exclude the element at index 2. The resulting list is [10, 20, 40, 50].
Related Article: How to Sort a Dictionary by Key in Python
Method 5: Using slicing
Slicing is a useful feature in Python that allows us to access a portion of a list. By combining slicing with concatenation, we can remove an element from a list by index.
Here’s an example of removing an element from a list by index using slicing:
# Define a list my_list = [10, 20, 30, 40, 50] # Remove the element at index 2 using slicing my_list = my_list[:2] + my_list[3:] print(my_list) # Output: [10, 20, 40, 50]
In the above example, the list is sliced into two parts: the elements before the index 2 and the elements after the index 2. Then, the two parts are concatenated to form a new list without the element at index 2. The resulting list is [10, 20, 40, 50].