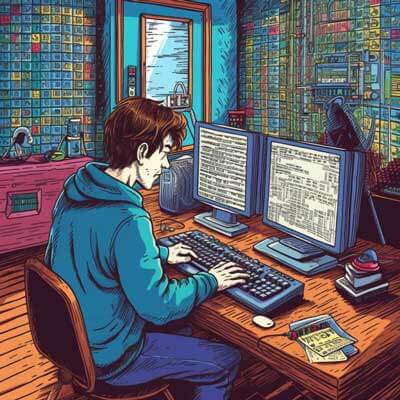
Sorting a dictionary by value in Python can be achieved by using the sorted() function along with a lambda function as the key parameter. This allows us to specify the desired sorting order based on the values of the dictionary. In this answer, we will explore two different approaches to sorting a dictionary by value in Python.
Approach 1: Using the sorted() function with a lambda function
One way to sort a dictionary by value in Python is by using the sorted() function along with a lambda function. The sorted() function takes an iterable and returns a new sorted list based on the specified sorting order. Here’s an example:
my_dict = {'apple': 10, 'banana': 5, 'cherry': 20} sorted_dict = sorted(my_dict.items(), key=lambda x: x[1]) print(sorted_dict)
Output:
[('banana', 5), ('apple', 10), ('cherry', 20)]
In the code above, we first convert the dictionary into a list of tuples using the items() method. Each tuple contains a key-value pair from the dictionary. Then, we pass this list to the sorted() function along with a lambda function as the key parameter. The lambda function extracts the second element from each tuple (the value) and uses it for sorting.
Related Article: How To Convert a Dictionary To JSON In Python
Approach 2: Using the operator module
Another approach to sorting a dictionary by value in Python is by using the operator module. The operator module provides a set of efficient functions that are commonly used in Python programs. In this case, we can use the itemgetter() function from the operator module to specify the sorting order based on the values of the dictionary. Here’s an example:
import operator my_dict = {'apple': 10, 'banana': 5, 'cherry': 20} sorted_dict = sorted(my_dict.items(), key=operator.itemgetter(1)) print(sorted_dict)
Output:
[('banana', 5), ('apple', 10), ('cherry', 20)]
In the code above, we import the operator module and use the itemgetter() function as the key parameter of the sorted() function. The itemgetter() function takes an index as a parameter and returns a callable that fetches the item at that index. In this case, we pass 1 as the index to sort the dictionary based on the second element of each tuple (the value).
Why is this question asked?
The question on how to sort a dictionary by value in Python is a common one because dictionaries in Python are unordered collections of key-value pairs. While dictionaries are efficient for retrieving values based on keys, they do not preserve any specific order. Therefore, there may be situations where we need to sort a dictionary based on the values it contains. Sorting a dictionary by value allows us to retrieve the items in a specific order, which can be useful for various purposes such as displaying the data or performing further calculations.
Potential reasons to sort a dictionary by value:
1. Displaying data: Sorting a dictionary by value can be helpful when we want to display the items in a specific order, such as ranking the items based on their values. This can be useful in scenarios like leaderboard rankings or displaying the top N items.
2. Filtering data: Sorting a dictionary by value can also be useful for filtering the items based on specific criteria. For example, we may want to retrieve the items that have values above a certain threshold or select the items with the highest values.
Related Article: How to Sort a Dictionary by Key in Python
Suggestions and alternative ideas:
1. Reverse sorting order: By default, the sorted() function sorts in ascending order. However, we can reverse the sorting order by setting the reverse parameter to True. This can be useful when we want to sort the dictionary in descending order based on the values.
2. Sorting by key: If we need to sort the dictionary based on the keys instead of the values, we can simply omit the lambda function or itemgetter() function and pass the keys() method as the key parameter to the sorted() function.
3. Using OrderedDict: If we need to preserve the sorted order of a dictionary, we can use the OrderedDict class from the collections module. The OrderedDict class is a subclass of the built-in dict class that remembers the order in which the items were inserted. We can use the sorted() function along with the items() method and OrderedDict to achieve a sorted dictionary by value.
Best practices:
1. Avoid modifying the original dictionary: When sorting a dictionary by value, it is generally recommended to create a new sorted list or dictionary instead of modifying the original dictionary. This helps to preserve the original data and prevents any unexpected behavior that may occur due to modifying the dictionary while iterating over its items.
2. Handle duplicate values: If the dictionary contains duplicate values, the sorting order may not be deterministic. In such cases, we can add additional sorting criteria, such as sorting by key, to ensure a consistent order.