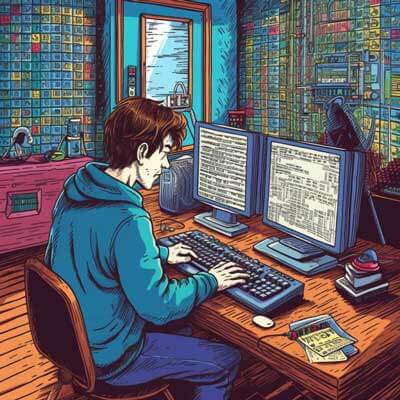
- Introduction to Joining Lists
- Concatenation of Elements
- Use Case: Merging Multiple Lists
- Use Case: Flattening Nested Lists
- Best Practice: Avoiding Memory Overhead
- Best Practice: Preserving Original Lists
- Real World Example: Data Aggregation
- Real World Example: Formatting Output Strings
- Performance Consideration: Time Complexity
- Performance Consideration: Space Complexity
- Advanced Technique: Using Map Function
- Advanced Technique: Using List Comprehension
- Code Snippet: Joining with Empty Separator
- Code Snippet: Joining with Custom Separator
- Code Snippet: Joining with Multiple Separators
- Code Snippet: Joining and Sorting
- Code Snippet: Joining and Removing Duplicates
- Error Handling: TypeError
- Error Handling: MemoryError
Introduction to Joining Lists
Joining lists is a common operation in Python where we concatenate the elements of multiple lists into a single list. This allows us to combine and manipulate data in various ways. In Python, we can use the join()
method to accomplish this task efficiently.
Related Article: How To Convert a Dictionary To JSON In Python
Concatenation of Elements
The join()
method is a string method that concatenates the elements of a list into a single string. It takes a separator as an argument and returns a string that consists of the elements joined by the separator. Here’s an example:
list_of_strings = ['Hello', 'World', '!'] separator = ' ' result = separator.join(list_of_strings) print(result)
Output:
Hello World !
In this example, we join the elements of the list_of_strings
using a space as the separator. The resulting string is 'Hello World !'
.
Use Case: Merging Multiple Lists
The join()
method is particularly useful when we need to merge multiple lists into a single list. Let’s say we have three lists containing the names of fruits, vegetables, and meats respectively. We can use the join()
method to merge them into a single list as follows:
fruits = ['apple', 'banana', 'orange'] vegetables = ['carrot', 'broccoli', 'spinach'] meats = ['chicken', 'beef', 'pork'] merged_list = fruits + vegetables + meats print(merged_list)
Output:
['apple', 'banana', 'orange', 'carrot', 'broccoli', 'spinach', 'chicken', 'beef', 'pork']
In this example, we concatenate the three lists using the +
operator, resulting in a merged list containing all the elements.
Use Case: Flattening Nested Lists
Another use case for joining lists is flattening nested lists. Sometimes, we may have a list of lists where each sublist represents a group of related items. To flatten the nested list and obtain a single list containing all the elements, we can use the join()
method along with list comprehension. Here’s an example:
nested_list = [['a', 'b', 'c'], ['d', 'e', 'f'], ['g', 'h', 'i']] flattened_list = [element for sublist in nested_list for element in sublist] print(flattened_list)
Output:
['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i']
In this example, we use list comprehension to iterate over each sublist in the nested list and then iterate over each element in the sublist. The resulting flattened list contains all the elements from the nested list.
Related Article: How to Sort a Dictionary by Key in Python
Best Practice: Avoiding Memory Overhead
When joining large lists, it’s important to consider the memory overhead. The join()
method creates a new string object for the joined elements, which can consume a significant amount of memory for large lists. To avoid this, we can use generator expressions instead of creating intermediate lists. Here’s an example:
list_of_strings = ['Hello', 'World', '!'] result = ''.join(element for element in list_of_strings) print(result)
Output:
HelloWorld!
In this example, we pass a generator expression to the join()
method instead of a list comprehension. This avoids creating an intermediate list and reduces memory overhead.
Best Practice: Preserving Original Lists
When joining lists, it’s important to note that the original lists remain unchanged. The join()
method returns a new string, but it does not modify the original lists. This is useful when we need to perform multiple join operations without altering the original data. Here’s an example:
fruits = ['apple', 'banana', 'orange'] vegetables = ['carrot', 'broccoli', 'spinach'] merged_list = fruits + vegetables print(merged_list) result = ', '.join(merged_list) print(result) print(fruits) print(vegetables)
Output:
['apple', 'banana', 'orange', 'carrot', 'broccoli', 'spinach'] apple, banana, orange, carrot, broccoli, spinach ['apple', 'banana', 'orange'] ['carrot', 'broccoli', 'spinach']
In this example, we concatenate the fruits
and vegetables
lists to create merged_list
. We then join the elements of merged_list
using a comma and space as the separator. However, the original fruits
and vegetables
lists remain unchanged.
Real World Example: Data Aggregation
Joining lists is commonly used in scenarios where we need to aggregate data from multiple sources. For example, let’s say we have a list of dictionaries where each dictionary represents a person and their respective attributes. We can use the join()
method to extract specific attributes and create a new list. Here’s an example:
person1 = {'name': 'John', 'age': 30} person2 = {'name': 'Jane', 'age': 25} person3 = {'name': 'Bob', 'age': 35} people = [person1, person2, person3] names = ', '.join(person['name'] for person in people) ages = ', '.join(str(person['age']) for person in people) print(f'Names: {names}') print(f'Ages: {ages}')
Output:
Names: John, Jane, Bob Ages: 30, 25, 35
In this example, we extract the names and ages of the people from the list of dictionaries using generator expressions and the join()
method. This allows us to aggregate the data into separate lists for further processing.
Related Article: How to Remove a Key from a Python Dictionary
Real World Example: Formatting Output Strings
Joining lists can also be helpful when formatting output strings. Let’s say we have a list of numbers and we want to display them in a specific format. We can use the join()
method to concatenate the numbers with a custom separator and format the output string. Here’s an example:
numbers = [1, 2, 3, 4, 5] formatted_output = ', '.join(f'Number {num}' for num in numbers) print(formatted_output)
Output:
Number 1, Number 2, Number 3, Number 4, Number 5
In this example, we use a generator expression to iterate over each number in the list and format it as 'Number '
. The resulting string is joined using a comma and space as the separator.
Performance Consideration: Time Complexity
The time complexity of the join()
method is O(n), where n is the total number of characters in the joined elements. This is because the method needs to iterate over each element and concatenate them into a single string. It is important to consider this when joining large lists, as the time taken to join the elements can increase linearly with the size of the input.
Performance Consideration: Space Complexity
The space complexity of the join()
method is O(n), where n is the total number of characters in the joined elements. This is because the method creates a new string object to store the joined elements. When joining large lists, the memory consumed by the resulting string can become a significant factor to consider.
Related Article: How to Remove an Element from a List by Index in Python
Advanced Technique: Using Map Function
An advanced technique for joining lists is to use the map()
function along with the join()
method. The map()
function applies a given function to each element of an iterable and returns an iterator of the results. Here’s an example:
list_of_strings = ['Hello', 'World', '!'] result = ''.join(map(str.upper, list_of_strings)) print(result)
Output:
HELLOWORLD!
In this example, we use the map()
function to apply the str.upper
function to each element in the list_of_strings
. The resulting iterator is then joined using the join()
method. This allows us to perform operations on the elements before joining them.
Advanced Technique: Using List Comprehension
List comprehension is another advanced technique that can be used for joining lists. Instead of using the join()
method directly, we can create a new list by manipulating the elements using list comprehension. Here’s an example:
list_of_strings = ['Hello', 'World', '!'] result = ''.join([element.upper() for element in list_of_strings]) print(result)
Output:
HELLOWORLD!
In this example, we use list comprehension to create a new list where each element is converted to uppercase using the str.upper()
method. The resulting list is then joined using the join()
method.
Code Snippet: Joining with Empty Separator
In some cases, we may want to join the elements of a list without any separator between them. We can achieve this by passing an empty string as the separator to the join()
method. Here’s an example:
list_of_strings = ['Hello', 'World', '!'] result = ''.join(list_of_strings) print(result)
Output:
HelloWorld!
In this example, we join the elements of the list_of_strings
without any separator, resulting in the string 'HelloWorld!'
.
Related Article: How to Solve a Key Error in Python
Code Snippet: Joining with Custom Separator
By default, the join()
method uses a space as the separator. However, we can specify a custom separator by passing it as an argument to the method. Here’s an example:
list_of_strings = ['Hello', 'World', '!'] separator = '-' result = separator.join(list_of_strings) print(result)
Output:
Hello-World-!
In this example, we join the elements of the list_of_strings
using a hyphen as the separator, resulting in the string 'Hello-World-!'
.
Code Snippet: Joining with Multiple Separators
Sometimes, we may need to join the elements of a list using multiple separators. We can achieve this by concatenating multiple join()
methods. Here’s an example:
list_of_strings = ['Hello', 'World', '!'] separator1 = '-' separator2 = ' ' result = separator2.join(separator1.join(list_of_strings).split(separator1)) print(result)
Output:
Hello World !
In this example, we first join the elements of the list_of_strings
using a hyphen as the separator. Then, we split the resulting string using the hyphen as the delimiter and join the elements again using a space as the separator. This allows us to join the elements with multiple separators.
Code Snippet: Joining and Sorting
Joining lists can also be combined with sorting to achieve desired results. Let’s say we have a list of words and we want to join them in alphabetical order. We can use the join()
method along with the sorted()
function to accomplish this. Here’s an example:
list_of_words = ['banana', 'apple', 'orange'] result = ', '.join(sorted(list_of_words)) print(result)
Output:
apple, banana, orange
In this example, we use the sorted()
function to sort the list_of_words
alphabetically. The resulting sorted list is then joined using a comma and space as the separator.
Related Article: How to Add New Keys to a Python Dictionary
Code Snippet: Joining and Removing Duplicates
When joining lists, it’s common to encounter duplicate elements. If we want to remove duplicates before joining, we can use the set()
function along with the join()
method. Here’s an example:
list_with_duplicates = ['apple', 'banana', 'orange', 'banana'] result = ', '.join(set(list_with_duplicates)) print(result)
Output:
banana, apple, orange
In this example, we convert the list_with_duplicates
into a set using the set()
function, which automatically removes duplicate elements. The resulting set is then joined using a comma and space as the separator.
Error Handling: TypeError
When using the join()
method, it’s important to ensure that all elements of the list are strings. Otherwise, a TypeError
will be raised. For example:
list_with_numbers = [1, 2, 3] result = ''.join(list_with_numbers) print(result)
Output:
TypeError: sequence item 0: expected str instance, int found
In this example, the join()
method expects the elements of the list to be strings, but the list_with_numbers
contains integers. To resolve this, we can convert the integers to strings using the str()
function before joining.
Error Handling: MemoryError
When joining extremely large lists, there is a possibility of encountering a MemoryError
due to insufficient memory. This can happen when the resulting string is too large to fit into memory. To avoid this error, it’s important to consider the memory constraints and optimize the join operation if necessary.