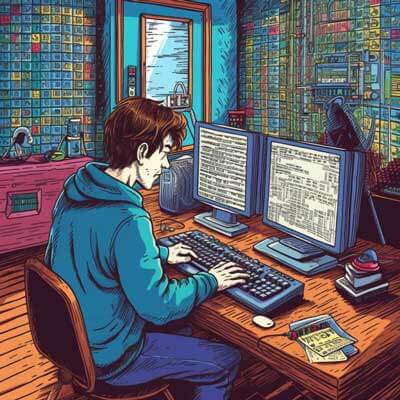
Comparing two lists in Python and returning the matches can be done using several methods. Here are two possible approaches:
Method 1: Using List Comprehension
One way to compare two lists in Python and return the matches is by using list comprehension. List comprehension provides a concise way to create new lists based on existing lists or other iterable objects.
To compare two lists and return the matches using list comprehension, you can iterate over one list and check if each element is present in the other list. If it is, you can add it to a new list.
Here’s an example that demonstrates this approach:
list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] matches = [x for x in list1 if x in list2] print(matches) # Output: [4, 5]
In this example, we have two lists: list1
and list2
. We use list comprehension to iterate over list1
and check if each element is present in list2
. If it is, we add it to the matches
list. Finally, we print the matches
list, which contains the elements that are present in both list1
and list2
.
Related Article: How To Convert a Dictionary To JSON In Python
Method 2: Using the set Intersection
Another way to compare two lists in Python and return the matches is by using the set intersection operation. In Python, sets are unordered collections of unique elements, and the intersection of two sets returns a new set that contains the common elements between them.
To compare two lists and return the matches using the set intersection, you can convert both lists to sets and then perform the intersection operation.
Here’s an example that demonstrates this approach:
list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] set1 = set(list1) set2 = set(list2) matches = list(set1.intersection(set2)) print(matches) # Output: [4, 5]
In this example, we convert list1
and list2
to sets using the set()
function. Then, we use the intersection()
method to find the common elements between the two sets. Finally, we convert the resulting set back to a list using the list()
function and store it in the matches
variable. The matches
list contains the elements that are present in both list1
and list2
, and we print it.
Additional Suggestions
– If the order of the matches is not important, you can use the set intersection method as it provides a faster solution compared to list comprehension, especially for larger lists.
– If you want to find the matches without considering duplicates, you can convert both lists to sets before performing the comparison.
– If you need to compare lists with complex objects or dictionaries, you can define a custom comparison function using the key
parameter of the list.sort()
or sorted()
functions.
– If you want to find the matches and their positions in the original lists, you can use the enumerate()
function along with list comprehension or the set intersection method.
Related Article: How to Sort a Dictionary by Key in Python