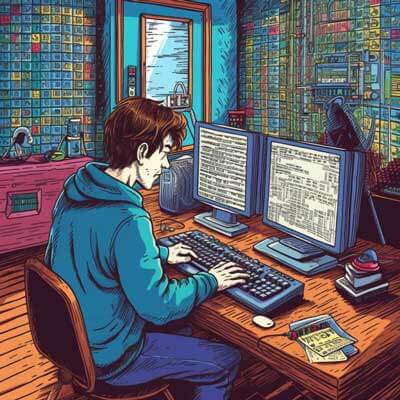
Sorting a dictionary by its keys in Python can be achieved using the built-in sorted()
function. Here are two possible approaches to accomplish this:
Approach 1: Using the sorted() function
The sorted()
function takes an iterable (such as a dictionary) and returns a new sorted list based on the elements in the iterable. To sort a dictionary by its keys, we can pass the dictionary’s keys()
method as the argument to the sorted()
function.
Here’s an example:
my_dict = {'c': 3, 'a': 1, 'b': 2} sorted_dict = {k: my_dict[k] for k in sorted(my_dict.keys())} print(sorted_dict)
Output:
{'a': 1, 'b': 2, 'c': 3}
In the example above, the sorted()
function is applied to the keys of the my_dict
dictionary. The keys are then used to construct a new dictionary sorted_dict
with the same key-value pairs as the original dictionary, but sorted by the keys.
Related Article: How To Convert a Dictionary To JSON In Python
Approach 2: Using the sorted() function with lambda
Another way to sort a dictionary by its keys is to use a lambda function as the key
parameter to the sorted()
function. This lambda function specifies that the keys should be used for sorting.
Here’s an example:
my_dict = {'c': 3, 'a': 1, 'b': 2} sorted_dict = {k: my_dict[k] for k in sorted(my_dict.keys(), key=lambda x: x)} print(sorted_dict)
Output:
{'a': 1, 'b': 2, 'c': 3}
In the above example, the lambda function key=lambda x: x
is used to specify that the keys themselves should be used for sorting. This lambda function returns the keys as-is, resulting in the dictionary being sorted by its keys.
Best Practices
When sorting a dictionary by its keys, it’s important to note that dictionaries in Python are inherently unordered. The order of the keys in a dictionary is not guaranteed to be the same as the order in which they were inserted. Therefore, if you need to maintain a specific order for your dictionary, you should consider using an ordered dictionary, such as the collections.OrderedDict
class.
Here’s an example of how to use collections.OrderedDict
to sort a dictionary by its keys:
from collections import OrderedDict my_dict = {'c': 3, 'a': 1, 'b': 2} sorted_dict = OrderedDict(sorted(my_dict.items(), key=lambda x: x[0])) print(sorted_dict)
Output:
OrderedDict([('a', 1), ('b', 2), ('c', 3)])
In the above example, the sorted()
function is used to sort the items of the dictionary my_dict
based on their keys. The key
parameter is set to lambda x: x[0]
, which specifies that the first element of each item (i.e., the key) should be used for sorting. The sorted items are then used to create a new OrderedDict
object sorted_dict
, which maintains the order of the keys.
Related Article: How to Remove a Key from a Python Dictionary