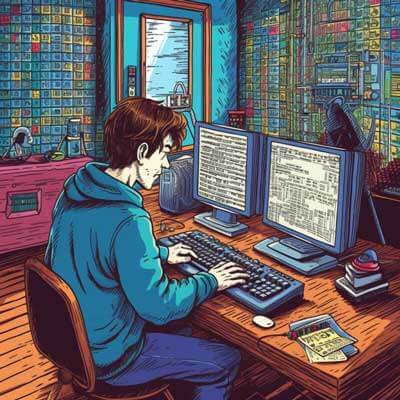
Table of Contents
Chapter 1: Introduction to OOP Concepts
Object-Oriented Programming (OOP) is a programming paradigm that organizes software design around objects rather than functions or logic. It allows for the creation of reusable and modular code, making it easier to maintain and understand large software systems. In this chapter, we will explore the fundamental concepts of OOP.
Related Article: How To Convert Array To List In Java
Example 1.1: Creating a Class
Let's start by creating a simple class in Java. A class is a blueprint for creating objects that have similar attributes and behaviors. Here's an example of a class representing a car:
public class Car { String make; String model; int year; public void startEngine() { System.out.println("Engine started."); } public void accelerate() { System.out.println("Car is accelerating."); } public void brake() { System.out.println("Car is braking."); } }
In the above example, we define a class called "Car" with three instance variables: "make", "model", and "year". We also have three methods: "startEngine()", "accelerate()", and "brake()". These methods represent the behaviors of a car.
Example 1.2: Creating Objects
Once we have a class defined, we can create objects, also known as instances of the class. Each object has its own set of instance variables and can invoke the methods defined in the class. Here's how we can create objects of the "Car" class:
public class Main { public static void main(String[] args) { Car myCar = new Car(); myCar.make = "Toyota"; myCar.model = "Camry"; myCar.year = 2020; Car anotherCar = new Car(); anotherCar.make = "Honda"; anotherCar.model = "Civic"; anotherCar.year = 2019; myCar.startEngine(); myCar.accelerate(); anotherCar.brake(); } }
In the above example, we create two objects of the "Car" class: "myCar" and "anotherCar". We set the values of their instance variables and invoke the methods defined in the class. Each object operates independently and can have different attribute values.
Chapter 2: Overview of Classes and Objects
Classes and objects are the building blocks of object-oriented programming. In this chapter, we will dive deeper into the concepts of classes and objects and explore their features and relationships.
Related Article: Java Adapter Design Pattern Tutorial
Creating a Class
In Java, a class is created using the "class" keyword followed by the class name. It can contain variables, methods, constructors, and other types of members. Here's an example of a class representing a student:
public class Student { String name; int age; public void study() { System.out.println("Student is studying."); } public void sleep() { System.out.println("Student is sleeping."); } }
In the above example, we define a class called "Student" with two instance variables: "name" and "age". We also have two methods: "study()" and "sleep()". These methods represent the behaviors of a student.
Creating Objects
Once we have a class defined, we can create objects of that class using the "new" keyword. Each object has its own set of instance variables and can invoke the methods defined in the class. Here's how we can create objects of the "Student" class:
public class Main { public static void main(String[] args) { Student john = new Student(); john.name = "John Smith"; john.age = 20; Student jane = new Student(); jane.name = "Jane Doe"; jane.age = 19; john.study(); jane.sleep(); } }
In the above example, we create two objects of the "Student" class: "john" and "jane". We set the values of their instance variables and invoke the methods defined in the class. Each object operates independently and can have different attribute values.
Chapter 3: Discussion on Inheritance
Inheritance is a key concept in object-oriented programming that allows one class to inherit the properties and methods of another class. It promotes code reuse and enhances the flexibility and modularity of the software. In this chapter, we will explore the concept of inheritance in Java.
Example 3.1: Creating a Parent Class
In Java, we can create a parent class using the "class" keyword, just like any other class. Here's an example of a parent class called "Animal":
public class Animal { protected String species; public Animal(String species) { this.species = species; } public void eat() { System.out.println("The " + species + " is eating."); } public void sleep() { System.out.println("The " + species + " is sleeping."); } }
In the above example, we define a parent class called "Animal" with an instance variable called "species" and two methods: "eat()" and "sleep()". The constructor initializes the "species" variable.
Related Article: How to Convert a String to Date in Java
Example 3.2: Creating a Child Class
To create a child class that inherits from a parent class, we use the "extends" keyword followed by the name of the parent class. Here's an example of a child class called "Dog" that inherits from the "Animal" class:
public class Dog extends Animal { public Dog() { super("Dog"); } public void bark() { System.out.println("The dog is barking."); } }
In the above example, we define a child class called "Dog" that extends the "Animal" class. The constructor of the "Dog" class calls the constructor of the "Animal" class using the "super" keyword. The "Dog" class also has an additional method called "bark()".
Creating Objects and Accessing Inherited Members
Once we have a child class defined, we can create objects of that class and access both the inherited members from the parent class and the members specific to the child class. Here's an example:
public class Main { public static void main(String[] args) { Dog dog = new Dog(); dog.eat(); dog.sleep(); dog.bark(); } }
In the above example, we create an object of the "Dog" class and invoke the inherited methods "eat()" and "sleep()" from the parent class "Animal". We also invoke the method "bark()" specific to the "Dog" class.
Chapter 4: Explanation of Polymorphism
Polymorphism is a powerful concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. It enables flexibility and extensibility in code design. In this chapter, we will explore the concept of polymorphism in Java.
Example 4.1: Creating a Parent Class
In Java, we can create a parent class using the "class" keyword, just like any other class. Here's an example of a parent class called "Shape":
public class Shape { public double getArea() { return 0; } }
In the above example, we define a parent class called "Shape" with a method called "getArea()". This method returns 0 as a placeholder value.
Related Article: How to Convert List to Array in Java
Example 4.2: Creating Child Classes
To demonstrate polymorphism, we will create two child classes that inherit from the "Shape" class: "Rectangle" and "Circle". Each child class will override the "getArea()" method to provide its own implementation. Here are the child classes:
public class Rectangle extends Shape { private double length; private double width; public Rectangle(double length, double width) { this.length = length; this.width = width; } @Override public double getArea() { return length * width; } } public class Circle extends Shape { private double radius; public Circle(double radius) { this.radius = radius; } @Override public double getArea() { return Math.PI * radius * radius; } }
In the above examples, we define two child classes: "Rectangle" and "Circle". Each child class extends the "Shape" class and overrides the "getArea()" method to provide its own implementation.
Using Polymorphism
Once we have the parent and child classes defined, we can use polymorphism to treat objects of different classes as objects of a common superclass. Here's an example:
public class Main { public static void main(String[] args) { Shape[] shapes = new Shape[2]; shapes[0] = new Rectangle(4, 5); shapes[1] = new Circle(3); for (Shape shape : shapes) { System.out.println("Area: " + shape.getArea()); } } }
In the above example, we create an array of "Shape" objects that can hold objects of both the "Rectangle" and "Circle" classes. We initialize the array with instances of the child classes. In the loop, we invoke the "getArea()" method on each object, and polymorphism ensures that the appropriate implementation is called based on the actual object type.
Chapter 5: Working with Abstraction
Abstraction is a fundamental concept in object-oriented programming that allows us to model real-world entities in a simplified and logical manner. It helps in reducing complexity and focusing on essential features. In this chapter, we will explore the concept of abstraction in Java.
Example 5.1: Creating an Abstract Class
In Java, an abstract class is a class that cannot be instantiated and can contain both abstract and non-abstract methods. Here's an example of an abstract class called "Animal":
public abstract class Animal { protected String species; public Animal(String species) { this.species = species; } public abstract void makeSound(); public void sleep() { System.out.println("The " + species + " is sleeping."); } }
In the above example, we define an abstract class called "Animal" with an instance variable called "species". We have an abstract method called "makeSound()" that does not have an implementation. The class also has a non-abstract method called "sleep()" that provides a default implementation.
Related Article: How to Use the Xmx Option in Java
Example 5.2: Creating Concrete Subclasses
To use an abstract class, we need to create concrete subclasses that extend the abstract class and provide implementations for the abstract methods. Here's an example of two concrete subclasses: "Dog" and "Cat":
public class Dog extends Animal { public Dog() { super("Dog"); } @Override public void makeSound() { System.out.println("The dog is barking."); } } public class Cat extends Animal { public Cat() { super("Cat"); } @Override public void makeSound() { System.out.println("The cat is meowing."); } }
In the above examples, we define two concrete subclasses: "Dog" and "Cat". Each subclass extends the abstract class "Animal" and provides an implementation for the abstract method "makeSound()".
Using Abstraction
Once we have the abstract class and concrete subclasses defined, we can use abstraction to treat objects of different subclasses as objects of the abstract class. Here's an example:
public class Main { public static void main(String[] args) { Animal[] animals = new Animal[2]; animals[0] = new Dog(); animals[1] = new Cat(); for (Animal animal : animals) { animal.makeSound(); animal.sleep(); } } }
In the above example, we create an array of "Animal" objects that can hold objects of both the "Dog" and "Cat" classes. We initialize the array with instances of the concrete subclasses. In the loop, we invoke the "makeSound()" and "sleep()" methods on each object, and abstraction ensures that the appropriate implementation is called based on the actual object type.
Chapter 6: Exploring Encapsulation
Encapsulation is a fundamental principle of object-oriented programming that binds together the data and functions that manipulate the data, ensuring data integrity and providing controlled access to the data. In this chapter, we will explore the concept of encapsulation in Java.
Example 6.1: Creating a Class with Encapsulation
In Java, we can achieve encapsulation by using private access modifiers for the instance variables and providing public getter and setter methods to access and modify the values of the variables. Here's an example of a class called "Person" that demonstrates encapsulation:
public class Person { private String name; private int age; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age >= 0) { this.age = age; } else { System.out.println("Invalid age."); } } }
In the above example, we define a class called "Person" with private instance variables: "name" and "age". We provide public getter and setter methods to access and modify the values of these variables. The setter method for "age" includes a validation check to ensure that the age is not negative.
Related Article: How To Parse JSON In Java
Using Encapsulation
Once we have a class with encapsulation, we can create objects of that class and access or modify the private variables using the getter and setter methods. Here's an example:
public class Main { public static void main(String[] args) { Person person = new Person(); person.setName("John"); person.setAge(25); System.out.println("Name: " + person.getName()); System.out.println("Age: " + person.getAge()); } }
In the above example, we create an object of the "Person" class and use the setter methods to set the values of the private variables. We then use the getter methods to retrieve the values and print them. Encapsulation ensures that the private variables are accessed and modified in a controlled manner.
Chapter 7: Use Case: Inheritance in Action
Inheritance is a powerful concept in object-oriented programming that allows classes to inherit properties and methods from other classes. It promotes code reuse and enhances the flexibility and modularity of the software. In this chapter, we will explore a use case of inheritance in Java.
Use Case Scenario
Suppose we are developing a game that involves different types of vehicles. We want to model these vehicles in our code using inheritance. Here's an example of how we can define the classes using inheritance:
public class Vehicle { protected String brand; public Vehicle(String brand) { this.brand = brand; } public void start() { System.out.println("The " + brand + " is starting."); } public void stop() { System.out.println("The " + brand + " is stopping."); } } public class Car extends Vehicle { private String model; public Car(String brand, String model) { super(brand); this.model = model; } public void accelerate() { System.out.println("The " + brand + " " + model + " is accelerating."); } public void brake() { System.out.println("The " + brand + " " + model + " is braking."); } } public class Motorcycle extends Vehicle { private String type; public Motorcycle(String brand, String type) { super(brand); this.type = type; } public void wheelie() { System.out.println("The " + brand + " " + type + " is doing a wheelie."); } }
In the above example, we define a parent class called "Vehicle" with instance variables and methods common to all vehicles. We then create two child classes: "Car" and "Motorcycle" that inherit from the "Vehicle" class and add their own specific methods and instance variables.
Using Inherited and Specific Methods
Once we have the classes defined, we can create objects of the child classes and use both the inherited methods from the parent class and the methods specific to the child class. Here's an example:
public class Main { public static void main(String[] args) { Car car = new Car("Toyota", "Camry"); car.start(); car.accelerate(); car.brake(); car.stop(); Motorcycle motorcycle = new Motorcycle("Harley-Davidson", "Sportster"); motorcycle.start(); motorcycle.wheelie(); motorcycle.stop(); } }
In the above example, we create objects of the "Car" and "Motorcycle" classes and invoke the inherited methods "start()" and "stop()" from the parent class "Vehicle". We also invoke the methods specific to each child class: "accelerate()", "brake()", and "wheelie()". Inheritance allows us to treat objects of different classes as objects of a common superclass, providing flexibility and code reusability.
Related Article: Java Hashmap Tutorial
Chapter 8: Use Case: Dynamic Polymorphism
Polymorphism is a powerful concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. It enables flexibility and extensibility in code design. In this chapter, we will explore a use case of dynamic polymorphism in Java.
Use Case Scenario
Suppose we are building a system to manage different types of employees in a company. We want to model these employees in our code using polymorphism. Here's an example of how we can define the classes using dynamic polymorphism:
public abstract class Employee { protected String name; public Employee(String name) { this.name = name; } public abstract double calculateSalary(); public void displayInfo() { System.out.println("Name: " + name); System.out.println("Salary: " + calculateSalary()); } } public class FullTimeEmployee extends Employee { private double monthlySalary; public FullTimeEmployee(String name, double monthlySalary) { super(name); this.monthlySalary = monthlySalary; } @Override public double calculateSalary() { return monthlySalary; } } public class PartTimeEmployee extends Employee { private double hourlyRate; private int hoursWorked; public PartTimeEmployee(String name, double hourlyRate, int hoursWorked) { super(name); this.hourlyRate = hourlyRate; this.hoursWorked = hoursWorked; } @Override public double calculateSalary() { return hourlyRate * hoursWorked; } }
In the above example, we define an abstract class called "Employee" with instance variables and methods common to all employees. We then create two concrete subclasses: "FullTimeEmployee" and "PartTimeEmployee" that inherit from the "Employee" class and provide their own implementations for the abstract method "calculateSalary()".
Using Dynamic Polymorphism
Once we have the classes defined, we can create objects of the child classes and use dynamic polymorphism to treat them as objects of the parent class. Here's an example:
public class Main { public static void main(String[] args) { Employee fullTimeEmployee = new FullTimeEmployee("John Smith", 5000); fullTimeEmployee.displayInfo(); Employee partTimeEmployee = new PartTimeEmployee("Jane Doe", 20, 40); partTimeEmployee.displayInfo(); } }
In the above example, we create objects of the "FullTimeEmployee" and "PartTimeEmployee" classes and assign them to variables of the type "Employee". We then invoke the "displayInfo()" method on each object, which calls the appropriate implementation of the "calculateSalary()" method based on the actual object type. Dynamic polymorphism allows us to treat objects of different classes as objects of a common superclass, providing flexibility and extensibility in code design.
Chapter 9: Use Case: Abstraction and Encapsulation
Abstraction and encapsulation are fundamental principles of object-oriented programming that help in reducing complexity, improving code maintainability, and enhancing data integrity. In this chapter, we will explore a use case of abstraction and encapsulation in Java.
Related Article: Saving Tree Data Structure in Java File: A Discussion
Use Case Scenario
Suppose we are developing a banking system that involves different types of bank accounts. We want to model these accounts in our code using abstraction and encapsulation. Here's an example of how we can define the classes:
public abstract class BankAccount { private String accountNumber; protected double balance; public BankAccount(String accountNumber) { this.accountNumber = accountNumber; this.balance = 0; } public String getAccountNumber() { return accountNumber; } public double getBalance() { return balance; } public abstract void deposit(double amount); public abstract void withdraw(double amount); } public class SavingsAccount extends BankAccount { private double interestRate; public SavingsAccount(String accountNumber, double interestRate) { super(accountNumber); this.interestRate = interestRate; } @Override public void deposit(double amount) { balance += amount; } @Override public void withdraw(double amount) { if (balance >= amount) { balance -= amount; } else { System.out.println("Insufficient balance."); } } public void calculateInterest() { double interest = balance * (interestRate / 100); balance += interest; } } public class CheckingAccount extends BankAccount { private double overdraftLimit; public CheckingAccount(String accountNumber, double overdraftLimit) { super(accountNumber); this.overdraftLimit = overdraftLimit; } @Override public void deposit(double amount) { balance += amount; } @Override public void withdraw(double amount) { if (balance + overdraftLimit >= amount) { balance -= amount; } else { System.out.println("Insufficient balance."); } } }
In the above example, we define an abstract class called "BankAccount" that encapsulates the common properties and methods of different types of bank accounts. We provide getter methods for the account number and balance. We also define abstract methods for depositing and withdrawing money, which will be implemented by the concrete subclasses: "SavingsAccount" and "CheckingAccount".
Using Abstraction and Encapsulation
Once we have the classes defined, we can create objects of the concrete subclasses and use the methods provided by the abstract class to perform banking operations. Here's an example:
public class Main { public static void main(String[] args) { SavingsAccount savingsAccount = new SavingsAccount("1234567890", 2.5); savingsAccount.deposit(1000); savingsAccount.calculateInterest(); System.out.println("Account number: " + savingsAccount.getAccountNumber()); System.out.println("Balance: " + savingsAccount.getBalance()); CheckingAccount checkingAccount = new CheckingAccount("0987654321", 500); checkingAccount.deposit(500); checkingAccount.withdraw(800); System.out.println("Account number: " + checkingAccount.getAccountNumber()); System.out.println("Balance: " + checkingAccount.getBalance()); } }
In the above example, we create objects of the "SavingsAccount" and "CheckingAccount" classes and use the methods provided by the abstract class "BankAccount" to deposit, withdraw, and retrieve the account number and balance. Abstraction and encapsulation ensure that the inner workings of the bank accounts are hidden and that the banking operations are performed in a controlled manner.
Chapter 10: Best Practice: Designing Classes and Objects
Designing classes and objects is a crucial aspect of object-oriented programming. It involves identifying the entities, their properties, and the behaviors they exhibit in the real world. In this chapter, we will explore some best practices for designing classes and objects in Java.
Best Practice 10.1: Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. It means that a class should have a single responsibility or do one thing well. This promotes modular and maintainable code. Here's an example:
public class Car { private String make; private String model; private int year; public Car(String make, String model, int year) { this.make = make; this.model = model; this.year = year; } // Getters and setters for make, model, and year public void startEngine() { // Code to start the engine } public void accelerate() { // Code to accelerate the car } public void brake() { // Code to apply the brakes } }
In the above example, the "Car" class has a single responsibility of representing a car and providing methods to interact with it. It does not contain unrelated functionalities, such as handling user input or performing complex calculations.
Related Article: How to Use Spring Restcontroller in Java
Best Practice 10.2: Encapsulation
Encapsulation is the practice of hiding the internal details of a class and providing controlled access to its properties and methods. It helps in maintaining data integrity and reducing code dependencies. Here's an example:
public class Person { private String name; private int age; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age >= 0) { this.age = age; } else { System.out.println("Invalid age."); } } }
In the above example, the instance variables "name" and "age" are private, and access to them is provided through getter and setter methods. This ensures that the values of these variables are accessed and modified in a controlled manner, preventing invalid or inconsistent data.
Best Practice 10.3: Composition over Inheritance
Composition is the practice of building complex objects by combining simpler objects. It promotes code reuse, flexibility, and modularity. In some cases, it is preferable over inheritance to avoid the limitations of a rigid class hierarchy. Here's an example:
public class Engine { public void start() { // Code to start the engine } public void stop() { // Code to stop the engine } } public class Car { private Engine engine; public Car() { engine = new Engine(); } public void startEngine() { engine.start(); } public void stopEngine() { engine.stop(); } }
In the above example, the "Car" class is composed of an "Engine" object. Instead of inheriting the behavior of an engine, the "Car" class uses an instance of the "Engine" class to delegate the responsibility of starting and stopping the engine. This allows for more flexibility in handling different types of engines and avoids the limitations of a fixed class hierarchy.
Best Practice 10.4: Consistent Naming Conventions
Using consistent naming conventions for classes, variables, and methods is essential for code readability and maintainability. It helps in understanding the purpose and functionality of different entities in the codebase. Here are some naming conventions:
- Class names should be in CamelCase and represent a noun or noun phrase, e.g., "Car", "Person".
- Variable and method names should be in camelCase and represent a noun or verb phrase, e.g., "engine", "startEngine()".
- Constants should be in uppercase with underscores separating words, e.g., "MAX_SPEED".
Following a consistent naming convention throughout the codebase improves code clarity and makes it easier for other developers to understand and maintain the code.
Chapter 11: Best Practice: Implementing Inheritance
Inheritance is a powerful concept in object-oriented programming that allows classes to inherit properties and methods from other classes. However, implementing inheritance requires careful consideration to ensure proper code organization and maintainability. In this chapter, we will explore some best practices for implementing inheritance in Java.
Related Article: How to Handle Java's Lack of Default Parameter Values
Best Practice 11.1: Favor Composition over Inheritance
As mentioned earlier, composition is often a better approach than inheritance when it comes to code organization and flexibility. It allows for greater modularity and avoids the limitations of a fixed class hierarchy. Instead of inheriting behavior, a class can use instances of other classes to delegate responsibilities. This promotes code reuse and makes the system more adaptable to change.
Best Practice 11.2: Follow the Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. In other words, subclasses should be able to be used interchangeably with their parent class. This promotes code flexibility and extensibility.
To adhere to the Liskov Substitution Principle, ensure that subclasses do not modify the behavior of inherited methods in a way that violates the expectations of the parent class. The method contracts and preconditions defined by the parent class should still hold true for the subclasses.
Best Practice 11.3: Use Abstract Classes or Interfaces
Abstract classes and interfaces are powerful tools for implementing inheritance in Java. Abstract classes provide a common base for subclasses and can define both abstract and non-abstract methods. Interfaces define a contract that classes must adhere to and can be implemented by multiple classes.
When designing a class hierarchy, consider using abstract classes or interfaces to define common behaviors and create a more modular and maintainable codebase. Abstract classes and interfaces provide a clear contract for subclasses and help in organizing related classes.
Best Practice 11.4: Avoid Deep Inheritance Hierarchies
Deep inheritance hierarchies can lead to complex code and make it harder to understand and maintain the system. It is important to keep the inheritance hierarchy shallow and focused. Aim for a hierarchy that is easy to comprehend and navigate.
If you find yourself building a deep inheritance hierarchy, consider refactoring the code to use composition or interfaces instead. Restructuring the code can simplify the system and make it more flexible and maintainable.
Related Article: Java String Substring Tutorial
Chapter 12: Best Practice: Applying Polymorphism
Polymorphism is a powerful concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. Properly applying polymorphism can lead to more flexible and extensible code. In this chapter, we will explore some best practices for applying polymorphism in Java.
Best Practice 12.1: Use Inheritance and Interfaces
Inheritance and interfaces are key components of polymorphism. Inheritance allows objects of different subclasses to be treated as objects of a common superclass, while interfaces define a contract that classes must adhere to. By using both inheritance and interfaces, you can achieve a higher degree of flexibility and code reuse.
When designing a system, identify common behaviors and define them in a superclass or an interface. Subclasses can then provide their own implementations for these behaviors. This allows for more modular and extensible code.
Best Practice 12.2: Leverage Method Overriding
Method overriding is a core feature of polymorphism that allows subclasses to provide their own implementations for inherited methods. By leveraging method overriding, you can customize the behavior of objects based on their actual types.
When overriding methods, ensure that the overridden method has the same signature (name and parameters) as the parent class method. This ensures that the method can be called polymorphically. Additionally, consider using the "@Override" annotation to indicate that a method is intended to override a superclass method. This helps in avoiding accidental mistakes.
Best Practice 12.3: Favor Composition over Concrete Types
Instead of relying on concrete types, favor composition and program to interfaces or abstract types. This allows for greater flexibility and code extensibility. By programming to interfaces or abstract types, you can switch implementations easily and introduce new behaviors without affecting the rest of the codebase.
For example, instead of directly using a specific implementation class, use an interface or an abstract type to define variables and method parameters. This allows you to pass different implementations at runtime and achieve polymorphic behavior.
Related Article: How to Create Immutable Classes in Java
Best Practice 12.4: Follow the Open-Closed Principle (OCP)
The Open-Closed Principle states that classes should be open for extension but closed for modification. It means that you should be able to extend the behavior of a class without modifying its existing code. This promotes code modularity and maintainability.
To adhere to the Open-Closed Principle, design classes and methods in a way that allows for easy extension through inheritance and interfaces. Avoid making substantial changes to existing code when introducing new behaviors. Instead, focus on adding new code that extends the existing functionality.
Chapter 13: Best Practice: Effective Use of Abstraction
Abstraction is a fundamental concept in object-oriented programming that helps in reducing complexity and improving code maintainability. Effective use of abstraction can lead to more modular and understandable code. In this chapter, we will explore some best practices for using abstraction in Java.
Best Practice 13.1: Identify Relevant Abstractions
When designing a system, it is important to identify the relevant abstractions that represent the essential features and behaviors. Analyze the problem domain and identify the entities, their properties, and the operations they perform. Group related entities and behaviors together to create meaningful abstractions.
For example, in a banking system, relevant abstractions may include "Account", "Transaction", and "Customer". Each abstraction should encapsulate related data and behaviors, providing a clear and focused representation of a real-world entity.
Best Practice 13.2: Define Abstract Classes or Interfaces
Abstract classes and interfaces are powerful tools for implementing abstraction in Java. Abstract classes can define common behaviors and provide a base for subclasses, while interfaces define a contract that classes must adhere to. By defining abstract classes or interfaces, you can create a clear contract for implementing classes.
When designing abstractions, consider whether an abstract class or an interface is more appropriate. Abstract classes are useful when there is a need for common behavior and code reuse, while interfaces are useful when multiple unrelated classes need to adhere to the same contract.
Related Article: How To Convert Java Objects To JSON With Jackson
Best Practice 13.3: Hide Implementation Details
One of the key goals of abstraction is to hide the implementation details of a class or an abstraction. By hiding implementation details, you can reduce complexity and provide a simplified interface for interacting with the abstraction.
To hide implementation details, use access modifiers to control the visibility of variables and methods. Encapsulate the data and behaviors within the abstraction and only expose the necessary functionality to the outside world. This ensures that the internal workings of the abstraction are hidden and that the abstraction can be used in a controlled manner.
Best Practice 13.4: Strive for High Cohesion
Cohesion is a measure of how closely the members of a class or an abstraction are related to each other. High cohesion means that the members of an abstraction work together to achieve a common goal. It promotes code clarity and maintainability.
When designing abstractions, strive for high cohesion by grouping related behaviors and data together. Avoid including unrelated functionalities or properties within an abstraction. This ensures that the abstraction has a focused purpose and is easier to understand and maintain.
Chapter 14: Best Practice: Proper Encapsulation
Encapsulation is a fundamental principle of object-oriented programming that promotes data integrity and controlled access to class members. Proper encapsulation ensures that the internal details of a class are hidden and that data can be accessed and modified in a controlled manner. In this chapter, we will explore some best practices for proper encapsulation in Java.
Best Practice 14.1: Use Access Modifiers
Access modifiers allow you to control the visibility and accessibility of class members. By using access modifiers appropriately, you can enforce encapsulation and prevent unauthorized access to sensitive data and methods.
- Use the "private" access modifier for instance variables that should not be accessed directly from outside the class.
- Use the "public" access modifier for methods that need to be accessible from outside the class.
- Use the "protected" access modifier for methods and variables that need to be accessible from subclasses.
By using the appropriate access modifiers, you can enforce encapsulation and ensure that class members are accessed and modified in a controlled manner.
Related Article: How to Use Public Static Void Main String Args in Java
Best Practice 14.2: Provide Getter and Setter Methods
Getter and setter methods allow controlled access to private instance variables. By providing getter methods, you can retrieve the values of instance variables, and by providing setter methods, you can modify the values of instance variables with validation checks if required.
When defining getter and setter methods, follow the naming conventions and use the "get" and "set" prefixes for the method names. This makes the code more readable and understandable for other developers.
Best Practice 14.3: Validate Data in Setter Methods
Setter methods provide an opportunity to validate the data before assigning it to instance variables. By validating the data in setter methods, you can ensure that only valid and consistent values are stored in the instance variables.
For example, if an instance variable represents a person's age, you can add a validation check in the setter method to ensure that the age is a positive value. If the validation fails, you can handle it appropriately, such as displaying an error message or throwing an exception.
Best Practice 14.4: Avoid Direct Access to Instance Variables
Direct access to instance variables from outside the class can bypass encapsulation and lead to inconsistent or invalid data. It is best to avoid direct access to instance variables and instead use getter and setter methods to access and modify the values.
By using getter and setter methods, you can enforce encapsulation and add additional logic or validation checks if required. This ensures that the data remains consistent and that modifications to the data are controlled.
Chapter 15: Real World Example: OOP in Software Development
Object-oriented programming (OOP) is widely used in software development to build complex and modular systems. OOP provides a set of principles and techniques that help in organizing code, promoting code reuse, and improving code maintainability. In this chapter, we will explore a real-world example of how OOP is used in software development.
Related Article: Can Two Java Threads Access the Same MySQL Session?
Real World Scenario
Suppose we are developing an e-commerce application that allows users to browse and purchase products online. The application needs to handle various entities such as users, products, orders, and payments. Let's see how OOP can be applied in this scenario.
Identifying Entities
The first step is to identify the relevant entities in the problem domain. In this case, we can identify the following entities:
- User: Represents a user of the application.
- Product: Represents a product available for purchase.
- Order: Represents an order placed by a user.
- Payment: Represents a payment made for an order.
Defining Classes
Once we have identified the entities, we can define classes that represent these entities. Each class will have its own properties and methods that model the behavior and characteristics of the entity.
Here's an example of how the classes could be defined:
public class User { private String username; private String email; private String password; // Constructor, getters, and setters } public class Product { private String name; private double price; private int quantity; // Constructor, getters, and setters } public class Order { private User user; private List<Product> products; private double totalAmount; // Constructor, getters, and setters } public class Payment { private Order order; private double amount; private String paymentMethod; // Constructor, getters, and setters }
In the above example, we define four classes: "User", "Product", "Order", and "Payment". Each class has its own set of properties and methods that represent the characteristics and behaviors of the corresponding entity.
Applying Encapsulation and Abstraction
To apply encapsulation and abstraction, we can use access modifiers to control the visibility of class members and provide getter and setter methods to access and modify the values of instance variables.
For example, in the "User" class, we can encapsulate the "email" and "password" properties and provide getter and setter methods to interact with them. This ensures that the sensitive data is accessed and modified in a controlled manner.
Similarly, in the "Order" class, we can hide the implementation details of how the total amount is calculated and provide a getter method to retrieve the calculated value.
By applying encapsulation and abstraction, we can hide the internal details of the classes and provide a simplified interface for interacting with the entities.
Related Article: How To Fix A NullPointerException In Java
Chapter 16: Real World Example: Polymorphism in Design Patterns
Polymorphism is a powerful concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. Polymorphism plays a crucial role in design patterns, which are widely used solutions to common software design problems. In this chapter, we will explore a real-world example of how polymorphism is used in design patterns.
Real World Scenario
Suppose we are developing a messaging application that supports multiple messaging protocols such as SMS, email, and push notifications. Each protocol has its own way of sending messages. Let's see how polymorphism can be applied to handle this scenario.
Identifying Common Behavior
The first step is to identify the common behavior across different messaging protocols. In this case, the common behavior is the ability to send a message. Each protocol may have its own specific implementation of sending a message, but they all share the same interface or base class.
Defining an Interface or Base Class
Once we have identified the common behavior, we can define an interface or a base class that represents this behavior. This interface or base class will define the contract that all messaging protocols must adhere to.
Here's an example of how an interface could be defined:
public interface MessageSender { void sendMessage(String recipient, String message); }
In the above example, we define an interface called "MessageSender" that declares a single method "sendMessage()". This method takes a recipient and a message as parameters and represents the common behavior of sending a message.
Related Article: Java String Comparison: String vs StringBuffer vs StringBuilder
Implementing Specific Protocols
Once we have the interface or base class defined, we can implement it for each specific messaging protocol. Each implementation will provide its own logic for sending a message based on the requirements of the protocol.
Here's an example of how an implementation for the SMS protocol could be defined:
public class SmsSender implements MessageSender { public void sendMessage(String recipient, String message) { // Code to send an SMS message } }
In the above example, we implement the "MessageSender" interface in the "SmsSender" class. The implementation contains the code to send an SMS message.
We can similarly implement the interface or base class for other messaging protocols such as email and push notifications, providing the specific logic for each protocol.
Using Polymorphism
Once we have the implementations for different messaging protocols, we can use polymorphism to treat objects of these implementations as objects of the common interface or base class.
Here's an example of how polymorphism can be used to send messages:
public class MessagingApp { private MessageSender messageSender; public MessagingApp(MessageSender messageSender) { this.messageSender = messageSender; } public void sendMessage(String recipient, String message) { messageSender.sendMessage(recipient, message); } }
In the above example, we define a class called "MessagingApp" that has a dependency on the "MessageSender" interface. The "MessagingApp" class has a method called "sendMessage()" that takes a recipient and a message as parameters and delegates the task of sending the message to the implementation of the "MessageSender" interface.
By using polymorphism, we can pass different implementations of the "MessageSender" interface to the "MessagingApp" class and achieve the desired behavior based on the actual implementation at runtime. This provides flexibility and extensibility in handling different messaging protocols.
Chapter 17: Performance Consideration: Efficient Class Design
Efficient class design is crucial for achieving optimal performance in software systems. By carefully designing classes and their interactions, you can minimize resource usage, reduce bottlenecks, and improve overall system performance. In this chapter, we will explore some considerations for designing classes efficiently in Java.
Consideration 17.1: Minimize Object Creation
Creating objects can be an expensive operation in terms of memory and CPU usage. To improve performance, minimize unnecessary object creation, especially in performance-critical sections of the code.
Avoid creating objects inside loops or frequently invoked methods. Instead, consider reusing existing objects or using primitive types where appropriate. By minimizing object creation, you can reduce memory usage and garbage collection overhead, leading to improved performance.
Related Article: How to Generate Random Integers in a Range in Java
Consideration 17.2: Use Immutable Objects
Immutable objects are objects whose state cannot be modified after creation. They offer several benefits, including improved thread safety, reduced complexity, and enhanced performance.
By designing classes to be immutable where possible, you eliminate the need for synchronization and can safely share instances across multiple threads. Immutable objects also allow for better caching and can be used as keys in hash-based data structures, resulting in faster lookups.
Consideration 17.3: Optimize Memory Usage
Efficient memory usage is crucial for optimal performance. Consider the following techniques to optimize memory usage:
- Avoid unnecessary duplication of data.
- Use primitive types instead of their boxed counterparts (e.g., "int" instead of "Integer") where possible.
- Use data structures and algorithms that minimize memory usage, such as using arrays instead of lists for large collections.
By optimizing memory usage, you can reduce memory footprint, decrease garbage collection overhead, and improve overall performance.
Chapter 18: Performance Consideration: Inheritance and Polymorphism
Inheritance and polymorphism are powerful concepts in object-oriented programming, but they can have performance implications if not used carefully. By understanding the potential performance considerations and applying best practices, you can achieve efficient code design. In this chapter, we will explore some performance considerations related to inheritance and polymorphism in Java.
Consideration 18.1: Method Overhead in Inheritance
Method calls in inheritance introduce additional overhead compared to direct method calls. When invoking a method on a subclass, the JVM needs to perform additional lookups and checks to determine the correct method to execute.
While the performance impact is typically negligible, it can become significant in performance-critical sections of code. If performance is a concern, consider favoring composition over inheritance or using interfaces to achieve the desired behavior.
Related Article: How to Print a Hashmap in Java
Consideration 18.2: Polymorphic Dispatch
Polymorphic dispatch, where an object is treated as an object of a common superclass, incurs a performance cost. The JVM needs to determine the actual type of the object at runtime and dispatch the method call accordingly.
In performance-critical sections of code, where polymorphism is not essential, consider using direct method calls or specialized implementations. This can help avoid the overhead associated with polymorphic dispatch and improve performance.
Consideration 18.3: Method Inlining
Method inlining is an optimization technique used by the JVM to replace a method call with the actual body of the method. This eliminates the overhead of the method call itself.
Inheritance and polymorphism can limit the ability of the JVM to perform method inlining, as the specific method to be called may not be known at compile-time. By reducing the use of inheritance and polymorphism in performance-critical sections of code, you increase the chances of method inlining and improve performance.
Chapter 19: Performance Consideration: Abstraction and Encapsulation
Abstraction and encapsulation are fundamental principles of object-oriented programming, but they can have performance implications if not used carefully. By understanding the potential performance considerations and applying best practices, you can achieve efficient code design. In this chapter, we will explore some performance considerations related to abstraction and encapsulation in Java.
Consideration 19.1: Method Call Overhead in Abstraction
Method calls introduce overhead due to stack-frame setup, parameter passing, and return value handling. When using abstraction, such as interfaces or abstract classes, the JVM needs to perform additional lookups and checks to determine the correct method to execute.
While the performance impact is typically negligible, it can become significant in performance-critical sections of code. If performance is a concern, consider minimizing the use of abstraction in these sections or using more specialized implementations.
Related Article: How to Easily Print a Java Array
Consideration 19.2: Encapsulation and Performance Optimization
Encapsulation can introduce additional method calls and indirection, which can have a minor impact on performance. However, the benefits of encapsulation, such as improved maintainability and data integrity, often outweigh the minor performance impact.
If performance is a critical concern in specific sections of code, you can selectively relax encapsulation for those areas. However, it is important to carefully evaluate the trade-off between performance and code maintainability and ensure that the performance gains justify the loss of encapsulation benefits.
Consideration 19.3: Data Access in Encapsulation
Encapsulation often involves accessing instance variables through getter and setter methods instead of direct access. While this can introduce additional method calls, modern JVMs are optimized to handle such method invocations efficiently.
If performance profiling indicates that getter and setter methods are a bottleneck, you can selectively optimize critical sections of code by directly accessing instance variables. However, be cautious about bypassing encapsulation too extensively, as it may compromise data integrity and maintainability.
Chapter 20: Advanced Technique: Multi-Level Inheritance
Multi-level inheritance is a technique in object-oriented programming that allows a class to inherit properties and methods from its parent class, which in turn may inherit from its parent class. By using multi-level inheritance, you can create a hierarchy of classes that share common characteristics and behaviors. In this chapter, we will explore the concept of multi-level inheritance in Java.
Example 20.1: Creating a Multi-Level Inheritance Hierarchy
To demonstrate multi-level inheritance, let's consider an example where we have a base class called "Animal", a subclass called "Mammal" that inherits from "Animal", and another subclass called "Dog" that inherits from "Mammal".
public class Animal { protected String species; public Animal(String species) { this.species = species; } public void eat() { System.out.println("The " + species + " is eating."); } public void sleep() { System.out.println("The " + species + " is sleeping."); } } public class Mammal extends Animal { protected String habitat; public Mammal(String species, String habitat) { super(species); this.habitat = habitat; } public void giveBirth() { System.out.println("The " + species + " is giving birth to live young."); } } public class Dog extends Mammal { private String breed; public Dog(String species, String habitat, String breed) { super(species, habitat); this.breed = breed; } public void bark() { System.out.println("The " + breed + " is barking."); } }
In the above example, we define three classes: "Animal", "Mammal", and "Dog". The "Animal" class serves as the base class, which provides common properties and methods. The "Mammal" class is a subclass of "Animal" and inherits its properties and methods. The "Dog" class is a subclass of "Mammal" and inherits properties and methods from both "Animal" and "Mammal".
Related Article: How to Implement a Strategy Design Pattern in Java
Using the Multi-Level Inheritance Hierarchy
Once we have the multi-level inheritance hierarchy defined, we can create objects of the subclasses and use inherited methods and properties.
Here's an example of how we can use the multi-level inheritance hierarchy:
public class Main { public static void main(String[] args) { Dog dog = new Dog("Dog", "Land", "Labrador"); dog.eat(); dog.sleep(); dog.giveBirth(); dog.bark(); } }
In the above example, we create an object of the "Dog" class and invoke methods inherited from both the "Animal" and "Mammal" classes. The object operates as an instance of all three classes in the multi-level inheritance hierarchy. Each class in the hierarchy provides additional properties and behaviors specific to that class.
Multi-level inheritance allows for code reuse, promotes modularity, and provides a mechanism to express more complex relationships between classes. However, it is important to use multi-level inheritance judiciously and consider the trade-offs in terms of code complexity and maintainability.
Chapter 21: Advanced Technique: Interface and Abstract Class
Interfaces and abstract classes are powerful tools in Java for defining contracts and providing common behavior across multiple classes. They help in achieving code modularity, promoting code reuse, and enabling future extensibility. In this chapter, we will explore the concepts of interfaces and abstract classes and how they can be used in Java.
Example 21.1: Defining an Interface
An interface in Java defines a contract that classes must adhere to. It specifies a set of methods that participating classes must implement. Here's an example of how an interface could be defined:
public interface Shape { double getArea(); double getPerimeter(); }
In the above example, we define an interface called "Shape" that declares two methods: "getArea()" and "getPerimeter()". Any class that implements this interface must provide implementations for these methods.
Example 21.2: Implementing an Interface
To implement an interface, a class must use the "implements" keyword followed by the interface name. The class must provide implementations for all the methods declared in the interface. Here's an example of how an interface can be implemented:
public class Circle implements Shape { private double radius; public Circle(double radius) { this.radius = radius; } public double getArea() { return Math.PI * radius * radius; } public double getPerimeter() { return 2 * Math.PI * radius; } }
In the above example, we define a class called "Circle" that implements the "Shape" interface. The class provides implementations for the "getArea()" and "getPerimeter()" methods declared in the interface.
Related Article: Top Java Interview Questions and Answers
Example 21.3: Extending an Abstract Class
An abstract class in Java provides a partially implemented class that cannot be instantiated. It can contain abstract methods, concrete methods, instance variables, and constructors. Here's an example of how an abstract class could be defined:
public abstract class Animal { protected String species; public Animal(String species) { this.species = species; } public abstract void makeSound(); public void sleep() { System.out.println("The " + species + " is sleeping."); } }
In the above example, we define an abstract class called "Animal" that declares an abstract method "makeSound()" and a concrete method "sleep()". The abstract class provides a base for subclasses to extend and allows for code reuse.
Example 21.4: Subclassing an Abstract Class
To subclass an abstract class, a class must use the "extends" keyword followed by the abstract class name. The subclass must provide implementations for all the abstract methods declared in the abstract class. Here's an example of how an abstract class can be subclassed:
public class Dog extends Animal { public Dog() { super("Dog"); } public void makeSound() { System.out.println("The dog is barking."); } }
In the above example, we define a subclass called "Dog" that extends the abstract class "Animal". The subclass provides an implementation for the abstract method "makeSound()" declared in the abstract class.
Interfaces and abstract classes provide powerful mechanisms for defining contracts and sharing behavior across multiple classes. They help in achieving code modularity, promoting code reuse, and enabling future extensibility. By using interfaces and abstract classes effectively, you can create more flexible and maintainable code.