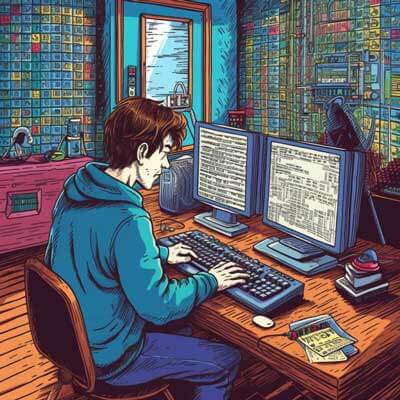
- Chapter 1: Introduction to RestController
- Chapter 2: Explanation of @RestController
- Chapter 3: Setting up @RestController
- Chapter 4: Use Case 1: Creating a Basic GET API
- Chapter 5: Use Case 2: Creating a POST API with @RequestBody
- Chapter 6: Best Practice 1: Using ResponseEntity
- Chapter 7: Best Practice 2: Incorporating @PathVariable
- Chapter 8: Real World Example 1: Implementing CRUD Operations
- Chapter 9: Real World Example 2: Integrating with a Database
- Chapter 10: Performance Consideration 1: Reducing Payload Size
- Chapter 11: Performance Consideration 2: Limiting API Requests
- Chapter 12: Advanced Technique 1: Versioning APIs
- Chapter 13: Advanced Technique 2: Securing APIs
- Chapter 14: Code Snippet 1: GET API with @PathVariable
- Chapter 15: Code Snippet 2: POST API with @RequestBody
- Chapter 16: Code Snippet 3: PUT API with @PathVariable and @RequestBody
- Chapter 17: Code Snippet 4: DELETE API with @PathVariable
- Chapter 18: Code Snippet 5: Exception Handling
- Chapter 19: Handling Errors in RestController
Chapter 1: Introduction to RestController
The RestController is a key component in the Spring Framework that simplifies the development of RESTful web services in Java. It is an annotation-based approach that allows developers to build APIs quickly and easily. RestController combines the functionality of both @Controller and @ResponseBody annotations, making it convenient to handle HTTP requests and generate JSON or XML responses.
Related Article: Spring Boot Integration with Payment, Communication Tools
Chapter 2: Explanation of @RestController
The @RestController annotation is used to mark a class as a RestController in the Spring Framework. It is a specialized version of the @Controller annotation, which is used for traditional Spring MVC applications. When @RestController is applied to a class, it indicates that the class will handle incoming HTTP requests and produce the response directly, without the need for a view resolver.
Here is an example of a basic RestController class:
@RestController public class UserController { // RestController methods go here }
Chapter 3: Setting up @RestController
To set up a RestController in your Java project, you need to follow these steps:
1. Include the necessary dependencies in your project’s build file, such as Maven or Gradle.
2. Create a new class and annotate it with @RestController.
3. Implement the desired RESTful API methods inside the class.
Here is an example of setting up a RestController in a Spring Boot project:
1. Add the following dependency to your Maven project’s pom.xml file:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
2. Create a new class called UserController and annotate it with @RestController:
@RestController public class UserController { // RestController methods go here }
Chapter 4: Use Case 1: Creating a Basic GET API
In this use case, we will create a basic GET API using the @GetMapping annotation. This API will retrieve a list of users from a database and return it as a JSON response.
Here is an example of how to create a basic GET API using RestController:
@RestController public class UserController { @Autowired private UserService userService; @GetMapping("/users") public List<User> getUsers() { return userService.getAllUsers(); } }
In this example, the getUsers() method is annotated with @GetMapping and maps the “/users” URL path to this method. The method retrieves the list of users from the UserService and returns it as a JSON response.
Related Article: Java Spring Security Customizations & RESTful API Protection
Chapter 5: Use Case 2: Creating a POST API with @RequestBody
In this use case, we will create a POST API that accepts a JSON request body and saves the user data to a database. We will use the @PostMapping annotation and the @RequestBody annotation to achieve this.
Here is an example of how to create a POST API using RestController:
@RestController public class UserController { @Autowired private UserService userService; @PostMapping("/users") public ResponseEntity<String> saveUser(@RequestBody User user) { userService.saveUser(user); return ResponseEntity.ok("User saved successfully"); } }
In this example, the saveUser() method is annotated with @PostMapping and maps the “/users” URL path to this method. The method accepts a JSON request body using the @RequestBody annotation and saves the user data to the database using the UserService. It returns a ResponseEntity with a success message.
Chapter 6: Best Practice 1: Using ResponseEntity
Using ResponseEntity is a best practice when working with RestController APIs. It allows you to have more control over the HTTP response, including the status code and headers.
Here is an example of how to use ResponseEntity in a RestController method:
@RestController public class UserController { @Autowired private UserService userService; @GetMapping("/users/{id}") public ResponseEntity<User> getUserById(@PathVariable Long id) { User user = userService.getUserById(id); if (user != null) { return ResponseEntity.ok(user); } else { return ResponseEntity.notFound().build(); } } }
In this example, the getUserById() method retrieves a user by their ID from the UserService. If the user exists, it returns a ResponseEntity with the user data and a status code of 200 (OK). If the user does not exist, it returns a ResponseEntity with a status code of 404 (Not Found).
Chapter 7: Best Practice 2: Incorporating @PathVariable
The @PathVariable annotation allows you to extract path variables from the URL and use them as method parameters in your RestController methods.
Here is an example of how to incorporate @PathVariable in a RestController method:
@RestController public class UserController { @Autowired private UserService userService; @GetMapping("/users/{id}") public User getUserById(@PathVariable Long id) { return userService.getUserById(id); } }
In this example, the getUserById() method retrieves a user by their ID from the UserService. The ID is extracted from the URL using the @PathVariable annotation and passed as a method parameter.
Related Article: Tutorial on Integrating Redis with Spring Boot
Chapter 8: Real World Example 1: Implementing CRUD Operations
In this real-world example, we will implement CRUD (Create, Read, Update, Delete) operations for a user entity using RestController APIs. We will use the appropriate HTTP methods and annotations to handle each operation.
Here is an example of how to implement CRUD operations using RestController:
@RestController public class UserController { @Autowired private UserService userService; @GetMapping("/users/{id}") public User getUserById(@PathVariable Long id) { return userService.getUserById(id); } @PostMapping("/users") public ResponseEntity<String> saveUser(@RequestBody User user) { userService.saveUser(user); return ResponseEntity.ok("User saved successfully"); } @PutMapping("/users/{id}") public ResponseEntity<String> updateUser(@PathVariable Long id, @RequestBody User user) { user.setId(id); userService.updateUser(user); return ResponseEntity.ok("User updated successfully"); } @DeleteMapping("/users/{id}") public ResponseEntity<String> deleteUser(@PathVariable Long id) { userService.deleteUser(id); return ResponseEntity.ok("User deleted successfully"); } }
In this example, we have implemented the getUserById(), saveUser(), updateUser(), and deleteUser() methods to handle the respective CRUD operations. The appropriate HTTP methods and annotations are used for each operation.
Chapter 9: Real World Example 2: Integrating with a Database
In this real-world example, we will integrate our RestController APIs with a database using the Spring Data JPA framework. We will use the JpaRepository interface to perform database operations.
Here is an example of how to integrate with a database using RestController:
@RestController public class UserController { @Autowired private UserRepository userRepository; @GetMapping("/users/{id}") public User getUserById(@PathVariable Long id) { return userRepository.findById(id).orElse(null); } @PostMapping("/users") public ResponseEntity<String> saveUser(@RequestBody User user) { userRepository.save(user); return ResponseEntity.ok("User saved successfully"); } // Other CRUD methods go here }
In this example, the UserRepository interface is autowired into the RestController. The getUserById() method retrieves a user by their ID using the findById() method provided by JpaRepository. The saveUser() method saves a user to the database using the save() method.
Chapter 10: Performance Consideration 1: Reducing Payload Size
Reducing payload size is an important performance consideration when working with RestController APIs. By minimizing the amount of data transferred over the network, we can improve the overall performance of our application.
One way to reduce payload size is by using data transfer objects (DTOs) instead of directly transferring domain objects. DTOs are lightweight objects that contain only the necessary data for a specific API request or response.
Here is an example of how to reduce payload size using DTOs:
@RestController public class UserController { @Autowired private UserService userService; @GetMapping("/users") public List<UserDto> getUsers() { List<User> users = userService.getAllUsers(); List<UserDto> userDtos = new ArrayList<>(); for (User user : users) { userDtos.add(new UserDto(user.getId(), user.getName())); } return userDtos; } }
In this example, we have created a UserDto class that contains only the necessary data for the API response. The getUsers() method retrieves a list of users from the UserService and maps them to UserDto objects. Only the required data is transferred over the network, reducing the payload size.
Related Article: Identifying the Version of Your MySQL-Connector-Java
Chapter 11: Performance Consideration 2: Limiting API Requests
Limiting API requests is another important performance consideration when working with RestController APIs. By restricting the number of requests made to our application, we can reduce the load on our servers and improve performance.
One way to limit API requests is by implementing rate limiting. Rate limiting allows us to set a maximum number of requests that can be made within a certain time period.
Here is an example of how to implement rate limiting in a RestController:
@RestController public class UserController { private static final int MAX_REQUESTS_PER_MINUTE = 100; private static final long ONE_MINUTE_IN_MILLISECONDS = 60000; private AtomicInteger requestCount = new AtomicInteger(0); private long lastResetTime = System.currentTimeMillis(); @GetMapping("/users") public List<User> getUsers() { if (isRateLimitExceeded()) { throw new RateLimitExceededException("Rate limit exceeded"); } // Rest of the logic goes here } private boolean isRateLimitExceeded() { long currentTime = System.currentTimeMillis(); if (currentTime - lastResetTime > ONE_MINUTE_IN_MILLISECONDS) { requestCount.set(0); lastResetTime = currentTime; } return requestCount.incrementAndGet() > MAX_REQUESTS_PER_MINUTE; } }
In this example, we have implemented rate limiting using an AtomicInteger to keep track of the number of requests made within a minute. If the rate limit is exceeded, a RateLimitExceededException is thrown.
Chapter 12: Advanced Technique 1: Versioning APIs
Versioning APIs is an advanced technique that allows you to manage changes to your RestController APIs over time. It ensures backward compatibility and allows clients to choose the desired API version.
There are different approaches to versioning APIs, such as URL versioning, request header versioning, or media type versioning. Let’s take a look at URL versioning as an example:
@RestController @RequestMapping("/v1/users") public class UserControllerV1 { // RestController methods for version 1 go here } @RestController @RequestMapping("/v2/users") public class UserControllerV2 { // RestController methods for version 2 go here }
In this example, we have created two different versions of the UserController class by using different URL paths. The first version is accessed using “/v1/users” and the second version is accessed using “/v2/users”. This allows clients to choose the appropriate version of the API.
Chapter 13: Advanced Technique 2: Securing APIs
Securing RestController APIs is essential to protect sensitive data and prevent unauthorized access. There are various security mechanisms available, such as authentication, authorization, and encryption.
One common approach to securing APIs is by using JSON Web Tokens (JWT). JWT is an open standard for securely transmitting information between parties as a JSON object. It can be used to authenticate and authorize users accessing your APIs.
Here is an example of how to secure a RestController API using JWT:
@RestController public class UserController { @Autowired private UserService userService; @PostMapping("/login") public ResponseEntity<String> login(@RequestBody LoginRequest loginRequest) { // Authenticate user and generate JWT token String token = userService.authenticate(loginRequest.getUsername(), loginRequest.getPassword()); if (token != null) { return ResponseEntity.ok(token); } else { return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build(); } } @GetMapping("/users/{id}") public ResponseEntity<User> getUserById(@PathVariable Long id, @RequestHeader("Authorization") String token) { // Validate JWT token and retrieve user data User user = userService.getUserById(id); if (user != null && userService.validateToken(token)) { return ResponseEntity.ok(user); } else { return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build(); } } // Rest of the methods go here }
In this example, we have implemented a /login API for user authentication. Upon successful authentication, a JWT token is generated and returned to the client. Subsequent API requests require the token to be included in the Authorization header. The getUserById() method validates the token and retrieves the user data.
Related Article: Proper Placement of MySQL Connector JAR File in Java
Chapter 14: Code Snippet 1: GET API with @PathVariable
Here is a code snippet demonstrating how to create a GET API with @PathVariable in a RestController:
@GetMapping("/users/{id}") public User getUserById(@PathVariable Long id) { return userService.getUserById(id); }
In this example, the getUserById() method maps the “/users/{id}” URL path to this method. The @PathVariable annotation extracts the ID from the URL and passes it as a method parameter.
Chapter 15: Code Snippet 2: POST API with @RequestBody
Here is a code snippet demonstrating how to create a POST API with @RequestBody in a RestController:
@PostMapping("/users") public ResponseEntity<String> saveUser(@RequestBody User user) { userService.saveUser(user); return ResponseEntity.ok("User saved successfully"); }
In this example, the saveUser() method maps the “/users” URL path to this method. The @RequestBody annotation is used to accept a JSON request body and bind it to the User object.
Chapter 16: Code Snippet 3: PUT API with @PathVariable and @RequestBody
Here is a code snippet demonstrating how to create a PUT API with @PathVariable and @RequestBody in a RestController:
@PutMapping("/users/{id}") public ResponseEntity<String> updateUser(@PathVariable Long id, @RequestBody User user) { user.setId(id); userService.updateUser(user); return ResponseEntity.ok("User updated successfully"); }
In this example, the updateUser() method maps the “/users/{id}” URL path to this method. The @PathVariable annotation extracts the ID from the URL and the @RequestBody annotation accepts a JSON request body and binds it to the User object.
Related Article: How to Connect Java with MySQL
Chapter 17: Code Snippet 4: DELETE API with @PathVariable
Here is a code snippet demonstrating how to create a DELETE API with @PathVariable in a RestController:
@DeleteMapping("/users/{id}") public ResponseEntity<String> deleteUser(@PathVariable Long id) { userService.deleteUser(id); return ResponseEntity.ok("User deleted successfully"); }
In this example, the deleteUser() method maps the “/users/{id}” URL path to this method. The @PathVariable annotation extracts the ID from the URL.
Chapter 18: Code Snippet 5: Exception Handling
Here is a code snippet demonstrating how to handle exceptions in a RestController:
@RestControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(UserNotFoundException.class) public ResponseEntity<String> handleUserNotFoundException(UserNotFoundException ex) { return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage()); } @ExceptionHandler(Exception.class) public ResponseEntity<String> handleException(Exception ex) { return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("An error occurred"); } }
In this example, we have created a GlobalExceptionHandler class annotated with @RestControllerAdvice. It contains methods annotated with @ExceptionHandler to handle specific exceptions. In case of a UserNotFoundException, a custom response with a status code of 404 (Not Found) is returned. For other exceptions, a generic error message with a status code of 500 (Internal Server Error) is returned.
Chapter 19: Handling Errors in RestController
When working with RestController APIs, it is important to handle errors properly to provide meaningful responses to clients. Errors can occur due to various reasons, such as validation failures, database errors, or system failures.
One approach to handling errors in RestController is by using exception handling. You can create custom exceptions for different error scenarios and handle them using appropriate exception handlers.
Here is an example of how to handle errors in a RestController:
@RestController public class UserController { @Autowired private UserService userService; @GetMapping("/users/{id}") public ResponseEntity<User> getUserById(@PathVariable Long id) { User user = userService.getUserById(id); if (user != null) { return ResponseEntity.ok(user); } else { throw new UserNotFoundException("User not found"); } } }
In this example, the getUserById() method checks if the user exists in the UserService. If the user is found, a ResponseEntity with the user data is returned. If the user is not found, a UserNotFoundException is thrown. This exception can be handled by a global exception handler to provide a custom error response to the client.