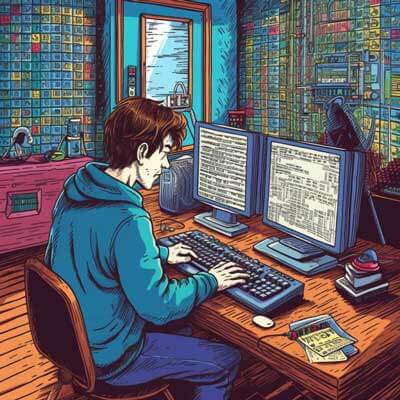
- Concurrency and Multithreading
- Java Synchronized Keyword
- Java Thread Safety
- Database Locking
- MySQL Connection Pool
- MySQL Transaction
- Java ExecutorService
- Java Thread Synchronization
- Java Thread Communication
- Handling Concurrent Database Access in Java
- Difference between Synchronized and Volatile in Java
- How Database Locking Works in MySQL
- Understanding Connection Pool in MySQL
- Ensuring Thread Safety in Java
- Synchronizing Threads in Java
- Understanding Transactions in MySQL
- ExecutorService in Java
- Communicating Between Threads in Java
- Best Practices for Concurrent Programming in Java
- Additional Resources
Concurrency and Multithreading
Concurrency and multithreading are fundamental concepts in modern software development. Concurrency refers to the ability of a program to execute multiple tasks simultaneously, while multithreading involves dividing a program into multiple threads that can run concurrently. In Java, threads are used to achieve concurrent execution of code.
Java provides robust support for multithreading through its built-in Thread class and related APIs. By creating multiple threads, Java enables developers to write programs that can perform multiple tasks concurrently, improving performance and responsiveness.
Let’s look at an example that demonstrates how to create and run multiple threads in Java:
public class MyThread extends Thread { @Override public void run() { // Code to be executed by the thread System.out.println("Hello from thread " + getId()); } } public class Main { public static void main(String[] args) { // Create and start multiple threads MyThread thread1 = new MyThread(); MyThread thread2 = new MyThread(); thread1.start(); thread2.start(); } }
In this example, we define a custom MyThread
class that extends the Thread
class. The run()
method contains the code that will be executed when the thread is started. We then create two instances of MyThread
and start them using the start()
method.
When we run this program, we may see the output “Hello from thread 1” and “Hello from thread 2” in any order, indicating that the threads are executing concurrently.
It’s important to note that while multithreading can bring significant benefits, it also introduces challenges related to thread safety and concurrent access to shared resources. In the context of accessing a MySQL session, these challenges become particularly relevant.
Related Article: How to Use the Xmx Option in Java
Java Synchronized Keyword
In Java, the synchronized
keyword is used to provide thread safety by ensuring that only one thread can execute a synchronized block of code or method at a time. This prevents concurrent access to shared resources and helps avoid data inconsistencies and race conditions.
To demonstrate how the synchronized
keyword works, consider the following example:
public class Counter { private int count = 0; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } } public class Main { public static void main(String[] args) { Counter counter = new Counter(); // Create multiple threads that increment the counter Thread thread1 = new Thread(() -> { for (int i = 0; i { for (int i = 0; i < 1000; i++) { counter.increment(); } }); thread1.start(); thread2.start(); // Wait for the threads to complete try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } // Print the final count System.out.println("Count: " + counter.getCount()); } }
In this example, we have a Counter
class with an increment()
method that increments the count
variable and a getCount()
method that returns the current count. Both methods are marked as synchronized
, ensuring that only one thread can execute them at a time.
We create two threads that concurrently call the increment()
method of the Counter
object. Each thread increments the count variable 1000 times. After both threads have completed, we print the final count. The output will always be 2000, demonstrating that the synchronized
keyword provides thread safety by preventing concurrent access to the shared count variable.
Java Thread Safety
Thread safety refers to the property of a program or object being able to handle multiple threads accessing it concurrently without causing data inconsistencies or other unexpected behaviors. Achieving thread safety is crucial when developing multithreaded applications to ensure correct and predictable results.
Java provides several mechanisms for ensuring thread safety, including the synchronized
keyword, atomic variables, and thread-safe data structures. Let’s explore these mechanisms with examples:
1. Synchronized Methods:
public class Counter { private int count = 0; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } }
In this example, the increment()
and getCount()
methods are marked as synchronized
, ensuring that only one thread can execute them at a time. This guarantees thread safety by preventing concurrent access to the count
variable.
2. Atomic Variables:
import java.util.concurrent.atomic.AtomicInteger; public class Counter { private AtomicInteger count = new AtomicInteger(0); public void increment() { count.incrementAndGet(); } public int getCount() { return count.get(); } }
In this example, we use the AtomicInteger
class from the java.util.concurrent.atomic
package. This class provides atomic operations for int
values, ensuring that incrementing and getting the count are performed atomically, without the need for explicit synchronization.
3. Thread-Safe Data Structures:
import java.util.concurrent.ConcurrentHashMap; import java.util.concurrent.ConcurrentMap; public class DataStore { private ConcurrentMap map = new ConcurrentHashMap(); public void put(String key, String value) { map.put(key, value); } public String get(String key) { return map.get(key); } }
In this example, we use the ConcurrentHashMap
class, which is a thread-safe implementation of the Map
interface. This data structure allows multiple threads to access and modify the map concurrently without explicit synchronization.
Database Locking
Database locking is a mechanism used to control access to shared resources within a database system. It allows multiple transactions to interact with the database concurrently while ensuring data integrity and consistency.
In the context of MySQL, there are two main types of locks: shared locks and exclusive locks. Shared locks allow concurrent transactions to read the same data, while exclusive locks prevent other transactions from reading or modifying the locked data.
Let’s consider an example to understand how database locking works in MySQL:
Suppose we have a table called employees
with columns id
, name
, and salary
. We want to update the salary of an employee with a given ID. We can use the following SQL statement:
UPDATE employees SET salary = 50000 WHERE id = 1;
When this statement is executed, MySQL will acquire an exclusive lock on the row with id = 1
to ensure that no other transaction can modify the salary while the update is in progress. Once the update is complete, the lock is released.
If another transaction tries to read or update the same row while the lock is held, it will be blocked until the lock is released. This ensures that data integrity is maintained and prevents conflicts between concurrent transactions.
MySQL also supports more granular locking mechanisms, such as table-level locks and row-level locks, which allow for finer control over concurrent access to data.
It’s important to note that while database locking provides concurrency control, excessive or improper use of locks can lead to performance issues, such as lock contention and deadlocks. Therefore, it’s crucial to design database schemas and queries in a way that minimizes the need for locks and optimizes concurrent access.
Related Article: How to Implement Recursion in Java
MySQL Connection Pool
A MySQL connection pool is a cache of database connections that can be reused by multiple threads to improve performance and scalability. It allows applications to reduce the overhead of establishing and closing connections to the database, making the overall system more efficient.
When a connection is requested, the connection pool checks if there are any available connections in the pool. If a connection is available, it is returned to the requesting thread. If not, a new connection is created and added to the pool.
Let’s look at an example of how to use a connection pool in Java with the help of the Apache Commons DBCP library:
1. Add the Apache Commons DBCP dependency to your project:
org.apache.commons commons-dbcp2 2.9.0
2. Create a DataSource
object configured with the necessary connection details:
import org.apache.commons.dbcp2.BasicDataSource; public class ConnectionPoolExample { public static void main(String[] args) { BasicDataSource dataSource = new BasicDataSource(); dataSource.setDriverClassName("com.mysql.jdbc.Driver"); dataSource.setUrl("jdbc:mysql://localhost:3306/mydb"); dataSource.setUsername("username"); dataSource.setPassword("password"); // Use the dataSource to obtain connections // ... } }
In this example, we create a BasicDataSource
object from the Apache Commons DBCP library. We set the necessary connection details such as the driver class name, URL, username, and password.
3. Obtain connections from the connection pool:
import org.apache.commons.dbcp2.BasicDataSource; public class ConnectionPoolExample { public static void main(String[] args) { BasicDataSource dataSource = new BasicDataSource(); // ... // Obtain a connection from the pool try (Connection connection = dataSource.getConnection()) { // Use the connection // ... } catch (SQLException e) { e.printStackTrace(); } } }
In this example, we use the getConnection()
method of the BasicDataSource
object to obtain a connection from the connection pool. We wrap the connection in a try-with-resources block to ensure it is automatically closed when no longer needed.
MySQL Transaction
A transaction in MySQL represents a sequence of database operations that are executed as a single unit. It ensures that either all the operations within the transaction are successfully completed, or none of them are applied, maintaining data integrity and consistency.
MySQL supports the ACID properties for transactions, which stand for Atomicity, Consistency, Isolation, and Durability:
– Atomicity: A transaction is treated as a single, indivisible unit of work. Either all the operations within the transaction are successfully completed, or none of them are applied. If any operation fails, the entire transaction is rolled back, and the database is left unchanged.
– Consistency: A transaction brings the database from one consistent state to another. It enforces integrity constraints, such as foreign key relationships and unique indexes, ensuring that the data remains consistent.
– Isolation: Transactions are executed in isolation from each other, ensuring that concurrent transactions do not interfere with each other. MySQL provides different isolation levels, such as Read Uncommitted, Read Committed, Repeatable Read, and Serializable, allowing developers to choose the level of isolation appropriate for their application.
– Durability: Once a transaction is committed, its changes are permanent and will survive system failures, such as power outages or crashes. MySQL ensures durability by writing the transaction’s changes to disk before acknowledging the commit.
Let’s see an example of how to use transactions in MySQL with Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.sql.Statement; public class TransactionExample { public static void main(String[] args) { String jdbcUrl = "jdbc:mysql://localhost:3306/mydb"; String username = "username"; String password = "password"; try (Connection connection = DriverManager.getConnection(jdbcUrl, username, password)) { // Set autocommit to false to start a transaction connection.setAutoCommit(false); try (Statement statement = connection.createStatement()) { // Execute multiple SQL statements within the transaction statement.executeUpdate("INSERT INTO employees (name, salary) VALUES ('John', 50000)"); statement.executeUpdate("UPDATE employees SET salary = salary + 1000 WHERE name = 'John'"); // Commit the transaction connection.commit(); } catch (SQLException e) { // Roll back the transaction if an exception occurs connection.rollback(); e.printStackTrace(); } } catch (SQLException e) { e.printStackTrace(); } } }
In this example, we start a transaction by setting autocommit
to false on the connection object. We then execute multiple SQL statements within the transaction, such as inserting a new employee and updating their salary. If any of the statements fail, we roll back the transaction using the rollback()
method. If all the statements succeed, we commit the transaction using the commit()
method.
Java ExecutorService
The ExecutorService
is a higher-level API in Java that provides a framework for executing and managing asynchronous tasks. It simplifies the process of creating and executing threads, allowing developers to focus on the logic of their tasks rather than the low-level details of thread management.
The ExecutorService
interface extends the Executor
interface and provides additional methods for managing the execution of tasks, such as submitting tasks for execution, shutting down the executor, and obtaining the results of completed tasks.
Let’s look at an example that demonstrates how to use the ExecutorService
in Java:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class ExecutorServiceExample { public static void main(String[] args) { // Create an ExecutorService with a fixed thread pool size ExecutorService executor = Executors.newFixedThreadPool(2); // Submit tasks for execution executor.submit(() -> { System.out.println("Task 1 executed by thread: " + Thread.currentThread().getName()); }); executor.submit(() -> { System.out.println("Task 2 executed by thread: " + Thread.currentThread().getName()); }); // Shut down the executor executor.shutdown(); } }
In this example, we create an ExecutorService
using the Executors.newFixedThreadPool()
method, which creates a thread pool with a fixed number of threads. We submit two tasks for execution using the submit()
method, which takes a Runnable
or Callable
object representing the task. The tasks are executed concurrently by the threads in the thread pool.
Once all the tasks have been submitted, we call the shutdown()
method to gracefully shut down the executor. This ensures that no new tasks can be submitted, and the executor will terminate when all the tasks have completed.
The ExecutorService
provides a useful and flexible way to manage the execution of tasks in Java, allowing developers to control thread creation, reuse, and termination efficiently.
Related Article: Java Adapter Design Pattern Tutorial
Java Thread Synchronization
Thread synchronization is the process of coordinating the execution of multiple threads to ensure that they access shared resources in a safe and orderly manner. It prevents data inconsistencies and race conditions that can occur when multiple threads access shared data concurrently.
Java provides several mechanisms for thread synchronization, including the synchronized
keyword, locks, and condition variables. These mechanisms allow developers to control access to shared resources and enforce critical sections of code.
Let’s explore an example that demonstrates how to use thread synchronization in Java:
public class Counter { private int count = 0; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } } public class Main { public static void main(String[] args) { Counter counter = new Counter(); // Create multiple threads that increment the counter Thread thread1 = new Thread(() -> { for (int i = 0; i { for (int i = 0; i < 1000; i++) { counter.increment(); } }); thread1.start(); thread2.start(); // Wait for the threads to complete try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } // Print the final count System.out.println("Count: " + counter.getCount()); } }
In this example, we have a Counter
class with an increment()
method that increments the count
variable and a getCount()
method that returns the current count. Both methods are marked as synchronized
, ensuring that only one thread can execute them at a time.
We create two threads that concurrently call the increment()
method of the Counter
object. Each thread increments the count variable 1000 times. After both threads have completed, we print the final count. The output will always be 2000, demonstrating that thread synchronization prevents data inconsistencies when multiple threads access the shared count variable.
Java Thread Communication
Thread communication is the process of coordinating the execution of multiple threads to allow them to exchange information, synchronize their actions, and work together towards a common goal. It enables threads to cooperate and coordinate their behavior, leading to more efficient and effective concurrent programs.
Java provides several mechanisms for thread communication, including shared objects, wait and notify methods, and higher-level constructs such as locks and condition variables. These mechanisms allow threads to communicate and synchronize their actions in a controlled and orderly manner.
Let’s explore an example that demonstrates how to use thread communication in Java:
public class Message { private String content; private boolean empty = true; public synchronized String read() { while (empty) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } empty = true; notifyAll(); return content; } public synchronized void write(String message) { while (!empty) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } empty = false; this.content = message; notifyAll(); } } public class Reader implements Runnable { private Message message; public Reader(Message message) { this.message = message; } @Override public void run() { String receivedMessage = message.read(); System.out.println("Received message: " + receivedMessage); } } public class Writer implements Runnable { private Message message; private String content; public Writer(Message message, String content) { this.message = message; this.content = content; } @Override public void run() { message.write(content); System.out.println("Sent message: " + content); } } public class Main { public static void main(String[] args) { Message message = new Message(); Thread readerThread = new Thread(new Reader(message)); Thread writerThread = new Thread(new Writer(message, "Hello, world!")); readerThread.start(); writerThread.start(); try { readerThread.join(); writerThread.join(); } catch (InterruptedException e) { e.printStackTrace(); } } }
In this example, we have a Message
class that represents a shared message between a reader and a writer thread. The read()
method is called by the reader thread to read the message, while the write()
method is called by the writer thread to write a new message.
Both the read()
and write()
methods are marked as synchronized
, ensuring that only one thread can access the message at a time. The wait()
method is used to suspend the calling thread until another thread calls notify()
or notifyAll()
, indicating that a certain condition has been met.
The reader thread waits until the message is not empty and then reads the content. The writer thread waits until the message is empty and then writes the new content. After the read or write operation is complete, the threads notify all other waiting threads to resume execution.
Handling Concurrent Database Access in Java
Handling concurrent database access in Java requires careful consideration of thread safety, transaction management, and performance optimization. It’s important to ensure that multiple threads can access the database concurrently without causing data inconsistencies or performance bottlenecks.
Here are some best practices for handling concurrent database access in Java:
1. Use Connection Pooling: Utilize a connection pool, such as Apache Commons DBCP or HikariCP, to manage database connections efficiently. Connection pooling reduces the overhead of establishing and closing connections, improves performance, and enables concurrent access to the database.
2. Ensure Thread Safety: Make sure that your code is thread-safe when accessing shared resources such as database connections or data objects. Use synchronization mechanisms, such as the synchronized
keyword or locks, to prevent concurrent access and data inconsistencies.
3. Use Transactions: Wrap database operations within transactions to ensure atomicity, consistency, isolation, and durability. Begin a transaction, execute the necessary database operations, and commit the transaction if all operations are successful. Roll back the transaction if an error occurs.
4. Optimize Database Queries: Analyze and optimize database queries to minimize the time spent in the database. Use indexes, query optimization techniques, and appropriate join strategies to ensure efficient retrieval and manipulation of data. Consider using prepared statements or parameterized queries to prevent SQL injection attacks and improve performance.
5. Fine-Tune Isolation Levels: Choose the appropriate isolation level for your database transactions. Higher isolation levels provide stronger data consistency but may impact performance due to increased locking. Lower isolation levels offer better performance but may introduce anomalies and data inconsistencies.
6. Handle Deadlocks: Deadlocks can occur when two or more threads are waiting for resources that are held by each other, resulting in a deadlock situation where none of the threads can proceed. Implement deadlock detection and resolution strategies, such as timeout mechanisms or resource ordering, to handle and prevent deadlocks.
7. Monitor and Tune Performance: Regularly monitor database performance using profiling tools, database monitoring tools, or logging. Identify performance bottlenecks, such as slow queries or lock contention, and optimize them accordingly. Consider using caching mechanisms, query optimization, or denormalization techniques to improve performance.
Related Article: How to Print a Hashmap in Java
Difference between Synchronized and Volatile in Java
In Java, both the synchronized
keyword and the volatile
keyword are used to ensure thread safety and prevent data inconsistencies in multithreaded programs. However, they serve different purposes and have different behaviors.
Here are the key differences between synchronized
and volatile
in Java:
1. Usage and Scope:
– synchronized
: Used to create mutually exclusive blocks of code or methods to ensure that only one thread can execute them at a time. The synchronization is based on an object’s monitor, and it can synchronize on different objects, such as instance methods, static methods, or blocks.
– volatile
: Used to declare a variable as volatile, indicating that its value may be modified by multiple threads. It ensures that changes to the variable are immediately visible to other threads, preventing thread-local caching of the variable’s value.
2. Atomicity:
– synchronized
: Provides atomicity by ensuring that a synchronized block or method is executed as a single, indivisible unit of work. It prevents thread interference and memory consistency errors by enforcing a happens-before relationship between the entrance and exit of the synchronized block or method.
– volatile
: Does not provide atomicity. It only guarantees that changes to the volatile variable are immediately visible to other threads. If multiple threads are updating the same volatile variable concurrently, volatile alone is not sufficient to ensure atomicity.
3. Memory Consistency:
– synchronized
: Provides strong memory consistency by enforcing a happens-before relationship. It ensures that changes made by one thread in a synchronized block or method are visible to other threads when they subsequently enter a synchronized block or method on the same object’s monitor.
– volatile
: Provides weaker memory consistency compared to synchronized
. It guarantees that changes made to the volatile variable are immediately visible to other threads, but it does not enforce a happens-before relationship for other operations.
4. Performance Impact:
– synchronized
: Involves acquiring and releasing locks, which can introduce overhead and potentially lead to contention and reduced performance in highly concurrent scenarios.
– volatile
: Generally has lower performance impact compared to synchronized
as it does not involve acquiring and releasing locks. However, excessive use of volatile
can result in increased memory traffic and reduced performance due to frequent updates and reads of volatile variables.
5. Applicability:
– synchronized
: Suitable for protecting critical sections of code or methods that access shared resources. It ensures exclusive access to the synchronized block or method, preventing concurrent modifications and data inconsistencies.
– volatile
: Suitable for variables that are read and written by multiple threads but do not require atomicity. It ensures that changes to the volatile variable are immediately visible to other threads, enabling efficient communication and synchronization between threads.
How Database Locking Works in MySQL
Database locking is a mechanism used by MySQL to control access to shared resources and ensure data consistency in the presence of concurrent transactions. When multiple transactions attempt to modify the same data concurrently, database locks are used to prevent conflicts and maintain data integrity.
MySQL supports various types of locks, including shared locks (S-locks) and exclusive locks (X-locks). A shared lock allows multiple transactions to read the same data simultaneously, while an exclusive lock allows only one transaction to modify the locked data.
Here’s how database locking works in MySQL:
1. Requesting and Acquiring Locks:
– When a transaction needs to read or modify a piece of data, it requests the appropriate lock from the database. The type of lock requested depends on the operation being performed. For example, a read operation requests a shared lock, while a write operation requests an exclusive lock.
– If the requested lock is not already held by another transaction, it is immediately granted, and the transaction proceeds. If the lock is already held by another transaction, the requesting transaction is placed in a wait state until the lock is released.
2. Lock Compatibility:
– MySQL uses a lock compatibility matrix to determine whether a lock can be granted or if a transaction must wait. The matrix defines the compatibility between different types of locks. For example, a shared lock is compatible with other shared locks but incompatible with an exclusive lock.
3. Lock Duration:
– Locks in MySQL can be either lock-while-using or lock-until-commit locks.
– Lock-while-using locks are released as soon as the transaction completes the operation for which the lock was granted. For example, a shared lock acquired for reading data is released after the read operation is complete.
– Lock-until-commit locks are held until the transaction is committed or rolled back. For example, an exclusive lock acquired for modifying data is held until the transaction is committed or rolled back.
4. Deadlocks:
– A deadlock occurs when two or more transactions are waiting indefinitely for each other to release the locks they hold. Deadlocks can lead to a complete halt in transaction processing.
– MySQL employs a deadlock detection mechanism to identify and resolve deadlocks. If a deadlock is detected, MySQL automatically chooses a victim transaction to abort, releasing its locks and allowing the other transactions to proceed. The aborted transaction can be rolled back, and the application can retry the transaction if desired.
Database locking is a fundamental aspect of transaction processing in MySQL. It ensures data consistency and prevents conflicts when multiple transactions access and modify shared data concurrently. Understanding how database locking works is crucial for designing efficient and scalable database systems.
Understanding Connection Pool in MySQL
A connection pool is a cache of database connections that can be reused to improve performance and scalability. It allows applications to reduce the overhead of establishing and closing connections to the database, resulting in faster response times and improved efficiency.
In the context of MySQL, a connection pool maintains a pool of established connections to the database. When a new connection is requested, the pool checks if there are any available connections. If a connection is available, it is reused. If not, a new connection is created and added to the pool.
Here’s how a connection pool works in MySQL:
1. Connection Pool Configuration:
– The connection pool is typically configured with a set of parameters, such as the minimum and maximum number of connections in the pool, the maximum time a connection can remain idle before being closed, and the maximum time a connection can be used before being closed.
– These parameters can be adjusted based on the expected workload and performance requirements of the application.
2. Connection Acquisition:
– When a connection is requested, the connection pool first checks if there are any idle connections in the pool. An idle connection is a connection that is currently not being used by any client.
– If an idle connection is available, it is returned to the requesting client. The connection is marked as “in use” to prevent other clients from using it simultaneously.
– If no idle connection is available and the maximum number of connections in the pool has not been reached, a new connection is created and added to the pool. This ensures that the pool always has a certain number of connections available for clients.
3. Connection Reuse:
– Once a connection is obtained from the pool, the client can use it to execute database operations.
– After the client finishes using the connection, it releases the connection back to the pool. The connection is marked as idle and becomes available for other clients to use.
– Reusing connections eliminates the need to establish a new connection for each client request, reducing the overhead of connection establishment and improving performance.
4. Connection Validation and Cleanup:
– The connection pool may periodically validate idle connections to ensure that they are still valid and can be reused. This can involve executing a simple query or sending a ping request to the database.
– If a connection fails the validation check, it is closed and removed from the pool. This ensures that the pool only contains valid and usable connections.
– The pool may also close idle connections that have been idle for too long, based on the configured maximum idle time. This prevents the pool from being filled with idle connections that are not being used.
Related Article: How to Manage Collections Without a SortedList in Java
Ensuring Thread Safety in Java
Ensuring thread safety is crucial when developing multithreaded applications in Java. Thread safety refers to the property of a program or object being able to handle multiple threads accessing it concurrently without causing data inconsistencies or other unexpected behaviors.
Here are some best practices for ensuring thread safety in Java:
1. Synchronization:
– Use the synchronized
keyword to create mutually exclusive blocks of code or methods. By synchronizing critical sections of code, you ensure that only one thread can execute them at a time, preventing concurrent modifications and data inconsistencies.
– Example:
public synchronized void increment() { count++; }
2. Atomic Variables:
– Use atomic variables from the java.util.concurrent.atomic
package, such as AtomicInteger
or AtomicLong
, to perform atomic operations on shared variables without the need for explicit synchronization.
– Example:
private AtomicInteger count = new AtomicInteger(0); public void increment() { count.incrementAndGet(); }
3. Thread-Safe Data Structures:
– Utilize thread-safe data structures, such as ConcurrentHashMap
or CopyOnWriteArrayList
, to handle concurrent access to shared data. These data structures provide built-in synchronization mechanisms that ensure thread safety.
– Example:
private ConcurrentMap map = new ConcurrentHashMap(); public void increment(String key) { map.compute(key, (k, v) -> v == null ? 1 : v + 1); }
4. Immutability:
– Design objects to be immutable whenever possible. Immutable objects are inherently thread-safe because their state cannot be modified once created. They can be safely shared between multiple threads without the risk of data inconsistencies.
– Example:
public final class ImmutablePoint { private final int x; private final int y; public ImmutablePoint(int x, int y) { this.x = x; this.y = y; } public int getX() { return x; } public int getY() { return y; } }
5. Thread-Local Variables:
– Use thread-local variables to store thread-specific data that should not be shared across threads. Each thread has its own copy of the variable, ensuring thread safety without the need for synchronization.
– Example:
private static ThreadLocal dateFormat = ThreadLocal.withInitial(() -> new SimpleDateFormat("yyyy-MM-dd")); public String formatDate(Date date) { return dateFormat.get().format(date); }
6. Volatile Variables:
– Use the volatile
keyword to declare variables that are accessed by multiple threads. Volatile ensures that changes to the variable are immediately visible to other threads, preventing thread-local caching of the variable’s value.
– Example:
private volatile boolean isRunning = true; public void stop() { isRunning = false; } public void run() { while (isRunning) { // Perform work } }
Synchronizing Threads in Java
Synchronizing threads in Java is essential for coordinating their execution and ensuring that they access shared resources in a safe and orderly manner. Synchronization prevents data inconsistencies, race conditions, and other issues that can arise when multiple threads access shared data concurrently.
Here are some techniques for synchronizing threads in Java:
1. Synchronized Blocks:
– Use the synchronized
keyword to create mutually exclusive blocks of code. By synchronizing critical sections of code, you ensure that only one thread can execute them at a time, preventing concurrent modifications and data inconsistencies.
– Example:
public void doSomething() { synchronized (lock) { // Code to be executed by a single thread at a time } }
2. Synchronized Methods:
– Mark methods as synchronized
to ensure that only one thread can execute them at a time. This simplifies synchronization by automatically locking the object on which the method is called.
– Example:
public synchronized void increment() { count++; }
3. Locks:
– Use explicit lock objects, such as ReentrantLock
or Lock
, to synchronize threads. Locks provide more flexibility and functionality compared to synchronized blocks, such as the ability to specify lock fairness or use multiple conditions for signaling.
– Example:
private Lock lock = new ReentrantLock(); public void doSomething() { lock.lock(); try { // Code to be executed by a single thread at a time } finally { lock.unlock(); } }
4. Condition Variables:
– Use condition variables, such as Condition
or java.util.concurrent.locks.Condition
, to coordinate the execution of threads. Condition variables allow threads to wait for a certain condition to be met before proceeding, enabling more advanced synchronization patterns.
– Example:
private Lock lock = new ReentrantLock(); private Condition condition = lock.newCondition(); public void doSomething() { lock.lock(); try { while (!conditionMet) { condition.await(); } // Code to be executed when the condition is met } catch (InterruptedException e) { Thread.currentThread().interrupt(); } finally { lock.unlock(); } } public void signalCondition() { lock.lock(); try { conditionMet = true; condition.signalAll(); } finally { lock.unlock(); } }
5. Thread Joining:
– Use the join()
method to wait for a thread to complete before proceeding. By joining threads, you ensure that their execution is synchronized, allowing one thread to wait for another to finish its work.
– Example:
Thread thread1 = new Thread(() -> { // Code executed by thread1 }); Thread thread2 = new Thread(() -> { // Code executed by thread2 }); thread1.start(); thread2.start(); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { Thread.currentThread().interrupt(); }
Understanding Transactions in MySQL
Transactions in MySQL are a fundamental concept for ensuring data integrity and consistency in database operations. A transaction represents a sequence of database operations that are executed as a single unit, either entirely or not at all. Transactions provide the ACID properties: Atomicity, Consistency, Isolation, and Durability.
Let’s explore the key aspects of transactions in MySQL:
1. Atomicity:
– Atomicity ensures that a transaction is treated as a single, indivisible unit of work. Either all the operations within the transaction are successfully completed, or none of them are applied. If any operation fails, the entire transaction is rolled back, and the database is left unchanged.
– The COMMIT
statement is used to commit a transaction, making its changes permanent. The ROLLBACK
statement is used to roll back a transaction, undoing its changes.
– Example:
START TRANSACTION; -- Database operations COMMIT;
2. Consistency:
– Consistency ensures that a transaction brings the database from one consistent state to another. It enforces integrity constraints, such as foreign key relationships and unique indexes, ensuring that the data remains consistent.
– If any operation within a transaction violates a constraint or integrity rule, the entire transaction is rolled back to maintain consistency.
3. Isolation:
– Isolation ensures that transactions are executed in isolation from each other, preventing interference and conflicts. MySQL provides different isolation levels, such as Read Uncommitted, Read Committed, Repeatable Read, and Serializable, allowing developers to choose the level of isolation appropriate for their application.
– Isolation levels define the behavior of transactions in terms of concurrency, consistency, and performance. Higher isolation levels provide stronger consistency but may impact performance due to increased locking. Lower isolation levels offer better performance but may introduce anomalies and data inconsistencies.
– Example:
SET TRANSACTION ISOLATION LEVEL SERIALIZABLE; START TRANSACTION; -- Database operations COMMIT;
4. Durability:
– Durability ensures that once a transaction is committed, its changes are permanent and will survive system failures, such as power outages or crashes. MySQL ensures durability by writing the transaction’s changes to disk before acknowledging the commit.
– Once a transaction is committed, its changes are stored in the database’s transaction log and can be recovered in the event of a failure.
Transactions provide a useful mechanism for managing database operations in a reliable and consistent manner. By using transactions, developers can ensure data integrity, handle complex operations as a single unit, and protect against data corruption in the event of failures.
Related Article: Java String Comparison: String vs StringBuffer vs StringBuilder
ExecutorService in Java
The ExecutorService
is a higher-level API in Java that provides a framework for executing and managing asynchronous tasks. It simplifies the process of creating and executing threads, allowing developers to focus on the logic of their tasks rather than the low-level details of thread management.
The ExecutorService
interface extends the Executor
interface and provides additional methods for managing the execution of tasks, such as submitting tasks for execution, shutting down the executor, and obtaining the results of completed tasks.
Here’s an overview of how to use the ExecutorService
in Java:
1. Creating an ExecutorService:
– Use the ExecutorService
factory methods in the Executors
class to create an instance of ExecutorService
. The factory methods provide various types of executors, such as fixed-size thread pools, cached thread pools, or scheduled thread pools.
– Example:
ExecutorService executor = Executors.newFixedThreadPool(5);
2. Submitting Tasks for Execution:
– Use the submit()
method of the ExecutorService
to submit tasks for execution. The submit()
method takes a Runnable
or Callable
object representing the task and returns a Future
object that can be used to obtain the result of the task.
– Example:
Future future = executor.submit(() -> { // Task logic return "Task completed"; });
3. Executing Tasks Asynchronously:
– The ExecutorService
executes tasks asynchronously, meaning that it assigns tasks to worker threads and returns immediately. The tasks are executed concurrently by the worker threads, allowing for parallel processing and improved performance.
– Example:
executor.submit(() -> { // Task 1 logic }); executor.submit(() -> { // Task 2 logic });
4. Obtaining Results of Completed Tasks:
– Use the get()
method of the Future
object to obtain the result of a completed task. The get()
method blocks until the task is completed and returns the result or throws an exception if the task failed.
– Example:
Future future = executor.submit(() -> { // Task logic return "Task completed"; }); try { String result = future.get(); System.out.println(result); } catch (InterruptedException | ExecutionException e) { e.printStackTrace(); }
5. Shutting Down the Executor:
– When you are done using the ExecutorService
, call the shutdown()
method to gracefully shut down the executor. The shutdown()
method initiates the shutdown process and allows the already submitted tasks to complete execution, but it does not accept new tasks.
– Example:
executor.shutdown();
The ExecutorService
provides a useful and flexible way to manage the execution of tasks in Java. It simplifies thread management, improves performance, and enables concurrent programming with ease.
Communicating Between Threads in Java
Communicating between threads in Java is crucial for coordination, synchronization, and data exchange between concurrent threads. Thread communication allows threads to work together, share information, and synchronize their actions.
Here are some techniques for communicating between threads in Java:
1. Shared Objects:
– Use shared objects to facilitate communication between threads. Shared objects are objects that can be accessed and modified by multiple threads. Synchronization mechanisms, such as the synchronized
keyword or locks, are used to ensure that shared objects are accessed safely.
– Example:
public class SharedObject { private int sharedData; public synchronized void setData(int data) { this.sharedData = data; } public synchronized int getData() { return sharedData; } }
2. Wait and Notify:
– Use the wait()
and notify()
methods of the Object
class to coordinate the execution and synchronization of threads. The wait()
method suspends the calling thread until another thread calls notify()
or notifyAll()
, indicating that a certain condition has been met.
– Example:
public class SharedObject { private boolean conditionMet = false; public synchronized void waitForCondition() { while (!conditionMet) { try { wait(); } catch (InterruptedException e) { Thread.currentThread().interrupt(); } } // Code to be executed when the condition is met } public synchronized void signalCondition() { conditionMet = true; notifyAll(); } }
3. Thread Joining:
– Use the join()
method to wait for a thread to complete before proceeding. By joining threads, you ensure that their execution is synchronized, allowing one thread to wait for another to finish its work.
– Example:
Thread thread1 = new Thread(() -> { // Code executed by thread1 }); Thread thread2 = new Thread(() -> { thread1.join(); // Wait for thread1 to complete // Code executed by thread2 }); thread1.start(); thread2.start();
4. Thread Signaling:
– Use flags or variables to signal between threads. A flag can be set by one thread to indicate that a certain condition has been met, and other threads can check the flag periodically to detect the signal.
– Example:
public class SharedObject { private volatile boolean flag = false; public void setFlag() { flag = true; } public boolean isFlagSet() { return flag; } }
5. Blocking Queues:
– Use blocking queues, such as BlockingQueue
or SynchronousQueue
, to exchange data between threads. Blocking queues provide blocking methods that allow threads to wait until there is data available or space available in the queue.
– Example:
BlockingQueue queue = new ArrayBlockingQueue(10); // Producer thread queue.put("Data"); // Blocks if the queue is full // Consumer thread String data = queue.take(); // Blocks if the queue is empty
Best Practices for Concurrent Programming in Java
Concurrency is a challenging aspect of software development, requiring careful consideration of thread safety, performance, and synchronization. Here are some best practices for concurrent programming in Java:
1. Understand Thread Safety:
– Gain a deep understanding of thread safety and the consequences of concurrent access to shared resources. Be aware of the risks of data inconsistencies, race conditions, and deadlock situations.
– Use synchronization mechanisms, such as the synchronized
keyword, locks, or atomic variables, to protect shared resources and ensure thread safety.
2. Minimize Shared Mutable State:
– Reduce the amount of shared mutable state in your application. Immutable objects and thread-local variables are inherently thread-safe and can simplify concurrent programming.
– Prefer immutability and design objects to be immutable whenever possible. Immutable objects can be safely shared between multiple threads without the risk of data inconsistencies.
3. Use Thread-Safe Data Structures:
– Utilize thread-safe data structures, such as ConcurrentHashMap
or CopyOnWriteArrayList
, to handle concurrent access to shared data. These data structures provide built-in synchronization mechanisms that ensure thread safety.
4. Choose the Right Concurrency API:
– Familiarize yourself with the various concurrency APIs in Java, such as the low-level synchronized
keyword, the higher-level ExecutorService
, or the advanced java.util.concurrent
package.
– Choose the API that best suits your needs in terms of performance, flexibility, and ease of use.
5. Optimize Lock Granularity:
– Use fine-grained locking whenever possible to minimize lock contention and improve concurrency. Instead of locking an entire object or method, consider locking only the critical sections that require synchronization.
– Analyze your code for potential lock bottlenecks and consider using locks with different levels of granularity, such as read-write locks, to improve performance.
6. Avoid Deadlocks:
– Deadlocks occur when two or more threads are waiting for each other to release resources, resulting in a complete halt in transaction processing. Understand the causes and symptoms of deadlocks and implement deadlock detection and resolution strategies.
– Use timeouts, resource ordering, or other deadlock prevention techniques to handle and prevent deadlocks.
7. Profile and Optimize Performance:
– Regularly profile your concurrent code to identify performance bottlenecks, such as slow queries, lock contention, or excessive synchronization. Use profiling tools, database monitoring tools, or logging to identify areas for optimization.
– Consider using caching mechanisms, query optimization techniques, or denormalization to improve performance in concurrent scenarios.
8. Thoroughly Test Concurrency:
– Test your concurrent code thoroughly to uncover race conditions, data inconsistencies, or other concurrency-related issues. Use testing frameworks, stress testing, or load testing to simulate concurrent scenarios and validate the correctness and performance of your code.
Related Article: How to Fix the Java NullPointerException
Additional Resources
– MySQL Connection Pooling in Java with HikariCP
– Java MySQL Connection Tutorial
– Java Database Connection: Pooling vs. Connection per Request