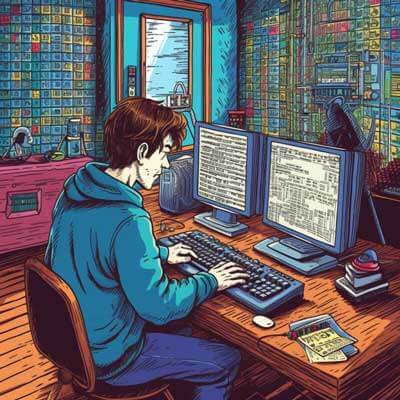
Table of Contents
A NullPointerException
is a common and often frustrating error that can occur in Java programs. It is thrown when a program tries to access or call a method on an object reference that is currently null
, meaning it does not point to any object in memory. This typically happens when a variable is not properly initialized or when an object reference is not assigned to an actual object.
To fix a NullPointerException
in Java, you can follow these steps:
1. Identify the location of the exception
The first step in fixing a NullPointerException
is to identify the exact location in your code where the exception is being thrown. This can be done by examining the exception stack trace, which provides information about the sequence of method calls that led to the exception. Look for the line number in the stack trace that corresponds to your code and identify the variable or object reference that is null
.
Related Article: Resolving MySQL Connection Issues in Java
2. Check for uninitialized variables
One common reason for a NullPointerException
is when a variable is not properly initialized before it is used. Make sure that all variables are assigned a value before they are accessed. For example, if you have a variable myObject
of type MyClass
, make sure to instantiate it before using any of its methods or accessing its properties:
MyClass myObject = new MyClass(); // Now you can use myObject safely
3. Verify object references
Another common cause of NullPointerException
is when an object reference is not assigned to an actual object. Check if the object reference you are trying to access is properly assigned and not null
. If the reference is null
, you can initialize it with a new object or make sure it is assigned a valid object reference before using it.
MyClass myObject; // ... myObject = new MyClass(); // Assign a valid object reference // Now you can use myObject without causing a NullPointerException
4. Handle null
conditions
In some cases, it is expected that an object reference may be null
at certain points in your code. To avoid NullPointerException
, you can add conditional checks to handle these null
conditions appropriately. For example, if you have a method that accepts an object reference as a parameter, you can check if the reference is null
before performing any operations on it:
public void doSomething(MyClass myObject) { if (myObject != null) { // Perform operations on myObject } else { // Handle the null condition } }
Related Article: How to Use the Java Command Line Arguments
5. Use the null-safe operator
Java provides the null-safe operator (?.
) as of Java 8, which allows you to safely access methods or properties of an object reference that may be null
. This operator acts as a short-circuit and prevents a NullPointerException
from being thrown. It is particularly useful when dealing with complex object hierarchies or when chaining method calls. Here's an example:
String result = myObject?.getNestedObject()?.getProperty();
In the above example, if any of the object references (myObject
, getNestedObject()
, or getProperty()
) is null
, the null-safe operator will return null
instead of throwing a NullPointerException
. You can then handle the null
condition accordingly.
6. Debugging and logging
When troubleshooting a NullPointerException
, it can be helpful to use debugging techniques and logging statements to trace the flow of your program and identify the root cause of the issue. Debuggers allow you to step through your code line by line and inspect the values of variables at runtime. Logging statements can help track the execution flow and provide information about the state of your program.
7. Review your code and design
In some cases, a NullPointerException
may indicate a deeper flaw in your code or design. It could be a result of improper handling of object lifecycles, incorrect usage of APIs, or inadequate error handling. Take the opportunity to review your code and design to ensure that objects are properly initialized, dependencies are correctly managed, and error conditions are handled gracefully.
8. Common pitfalls to avoid
There are several common pitfalls that can lead to NullPointerException
in Java programs. Avoid the following practices to minimize the occurrence of this error:
- Not initializing variables: Always initialize variables before using them to avoid NullPointerException
.
- Ignoring return values: Make sure to capture and handle the return values of methods that can potentially return null
.
- Incorrect comparison: Use the equals()
method instead of ==
to compare object references, as ==
compares the memory addresses and not the actual values.
- Overlooking dependencies: Ensure that all dependencies are properly initialized and injected.
- Incorrect array access: Verify that array indexes are within the bounds before accessing elements.