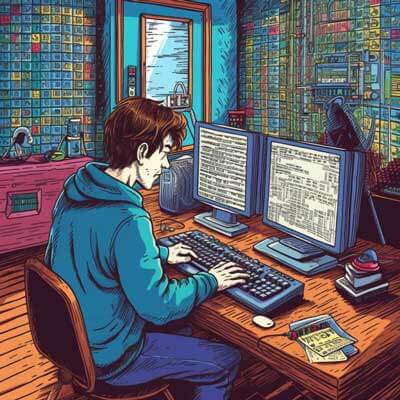
To print a HashMap in Java, you can follow one of the following approaches:
Approach 1: Using a for-each loop
One way to print the contents of a HashMap is by using a for-each loop to iterate over the key-value pairs and printing them one by one. Here’s an example:
import java.util.HashMap; import java.util.Map; public class PrintHashMapExample { public static void main(String[] args) { // Create a HashMap Map<String, Integer> hashMap = new HashMap<>(); // Add some key-value pairs hashMap.put("Apple", 10); hashMap.put("Orange", 5); hashMap.put("Banana", 8); // Print the HashMap for (Map.Entry<String, Integer> entry : hashMap.entrySet()) { System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue()); } } }
This will output:
Key: Apple, Value: 10 Key: Orange, Value: 5 Key: Banana, Value: 8
In this approach, we use the entrySet()
method of the HashMap to get a set of all key-value pairs. Then, we iterate over this set using a for-each loop and print each key-value pair using the getKey()
and getValue()
methods of the Map.Entry
interface.
Related Article: How to Use the Xmx Option in Java
Approach 2: Using Java 8 Streams
Another way to print the contents of a HashMap is by using Java 8 Streams. Here’s an example:
import java.util.HashMap; import java.util.Map; public class PrintHashMapExample { public static void main(String[] args) { // Create a HashMap Map<String, Integer> hashMap = new HashMap<>(); // Add some key-value pairs hashMap.put("Apple", 10); hashMap.put("Orange", 5); hashMap.put("Banana", 8); // Print the HashMap using Streams hashMap.entrySet().stream() .forEach(entry -> System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue())); } }
This will produce the same output as the previous approach:
Key: Apple, Value: 10 Key: Orange, Value: 5 Key: Banana, Value: 8
In this approach, we use the entrySet()
method of the HashMap to get a set of all key-value pairs. Then, we convert this set into a Stream using the stream()
method. Finally, we use the forEach()
method on the Stream to print each key-value pair.
Alternative Ideas:
– If you only want to print the keys or values of the HashMap, you can use the keySet()
or values()
methods respectively, instead of the entrySet()
method. For example:
// Print the keys for (String key : hashMap.keySet()) { System.out.println("Key: " + key); } // Print the values for (Integer value : hashMap.values()) { System.out.println("Value: " + value); }
– If you want to print the HashMap in a specific order, you can use a TreeMap
instead of a HashMap
. A TreeMap
sorts the elements based on their natural order or a specified comparator. Here’s an example:
import java.util.Map; import java.util.TreeMap; public class PrintTreeMapExample { public static void main(String[] args) { // Create a TreeMap Map<String, Integer> treeMap = new TreeMap<>(); // Add some key-value pairs treeMap.put("Apple", 10); treeMap.put("Orange", 5); treeMap.put("Banana", 8); // Print the TreeMap for (Map.Entry<String, Integer> entry : treeMap.entrySet()) { System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue()); } } }
This will output the key-value pairs in alphabetical order:
Key: Apple, Value: 10 Key: Banana, Value: 8 Key: Orange, Value: 5
Best Practices:
– When printing a HashMap, it’s recommended to use the entrySet()
method to iterate over the key-value pairs. This approach is more efficient than getting the keys or values separately, especially when the HashMap is large.
– To ensure a specific order when printing a HashMap, you can use a LinkedHashMap
. A LinkedHashMap
preserves the order of insertion, so the elements will be printed in the same order they were added.
– If you need to print a HashMap in a specific format or customize the output, you can override the toString()
method of the HashMap or create a separate method to format the output as desired.
– When working with large HashMaps, consider using parallel streams (parallelStream()
) to process the elements in parallel and potentially improve performance.
These are some general best practices to consider, but the best approach may vary depending on your specific requirements and use case.
Related Article: Can Two Java Threads Access the Same MySQL Session?
Conclusion:
Printing the contents of a HashMap in Java can be achieved using various approaches. Two common approaches are using a for-each loop to iterate over the key-value pairs and using Java 8 Streams. Additionally, there are alternative ideas such as printing only the keys or values, or using a TreeMap
for a specific order. It’s important to follow best practices, such as using the entrySet()
method for efficiency and considering the use of parallel streams for large HashMaps.