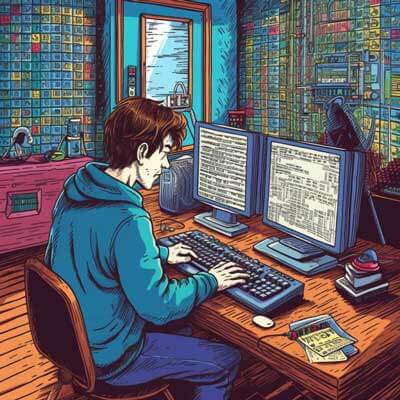
Java is a widely used programming language that has gained popularity due to its simplicity and robustness. However, one drawback of Java is its lack of default parameter values. Unlike some other programming languages, Java does not provide a built-in mechanism to specify default values for method parameters. This can sometimes lead to code duplication and make the code harder to read and maintain. In this answer, we will explore a few possible solutions to handle this limitation in Java.
1. Method Overloading
One way to handle Java’s lack of default parameter values is by using method overloading. Method overloading allows us to define multiple methods with the same name but different parameters. By providing overloaded methods with different parameter sets, we can mimic the behavior of default parameters.
Here’s an example:
public class Calculator { public int add(int a, int b) { return a + b; } public int add(int a) { // Assuming default value of b is 0 return add(a, 0); } }
In the above example, we have defined two overloaded versions of the add
method. The first method takes two integers and returns their sum. The second method takes only one integer and uses a default value of 0 for the second parameter. This way, callers can choose to provide both parameters or just one, and the method will work accordingly.
Using method overloading for default parameter values has its limitations. It can quickly become cumbersome if there are multiple parameters with default values, resulting in a large number of overloaded methods. Additionally, it may not be feasible if the number of possible combinations of default values is large.
Related Article: How to Convert JsonString to JsonObject in Java
2. Builder Pattern
Another approach to handle Java’s lack of default parameter values is by using the Builder pattern. The Builder pattern is a creational design pattern that allows us to construct complex objects step by step. It provides a fluent API for configuring an object’s properties, including setting default values.
Here’s an example:
public class Person { private String name; private int age; private String address; private Person(Builder builder) { this.name = builder.name; this.age = builder.age; this.address = builder.address; } public static class Builder { private String name; private int age; private String address; public Builder() { // Set default values this.name = ""; this.age = 0; this.address = ""; } public Builder name(String name) { this.name = name; return this; } public Builder age(int age) { this.age = age; return this; } public Builder address(String address) { this.address = address; return this; } public Person build() { return new Person(this); } } // Getters and other methods... }
In the above example, we have a Person
class with three properties: name
, age
, and address
. The Person
class has a private constructor that takes a Builder
object and initializes its properties. The Builder
class provides methods for setting the properties, including default values. To create a Person
object, we use the Builder
class to set the desired properties and then call the build
method.
Using the Builder pattern for default parameter values provides more flexibility compared to method overloading. It allows us to set default values for any number of parameters and provides a clear and readable API for constructing objects. However, it requires an extra layer of abstraction and may not be suitable for simple scenarios.
Alternative Ideas
While method overloading and the Builder pattern are commonly used approaches to handle Java’s lack of default parameter values, there are alternative ideas worth exploring:
- Annotation-based Configuration: Use annotations to specify default parameter values in a separate configuration file or class. During runtime, the application can read the annotations and apply the default values accordingly.
- Configuration Properties: Use a configuration file or external properties to specify default values for parameters. The application can read the configuration and use the default values when necessary.
- Optional Parameters: Instead of default values, use the
Optional
class introduced in Java 8 to handle optional parameters. This allows callers to explicitly indicate whether a parameter is present or absent.
Best Practices
When handling Java’s lack of default parameter values, it’s important to follow some best practices:
- Keep the code readable and maintainable. Use meaningful method and variable names to clearly indicate the purpose of the code.
- Choose the approach that best suits the specific requirements of the project. Consider factors such as code complexity, scalability, and maintainability.
- Avoid excessive method overloading or builder methods. Too many options can make the code harder to understand and maintain.
- Consider backward compatibility when introducing default parameter values. Existing code may rely on the absence of default values.
Related Article: How to Implement a Delay in Java Using java wait seconds