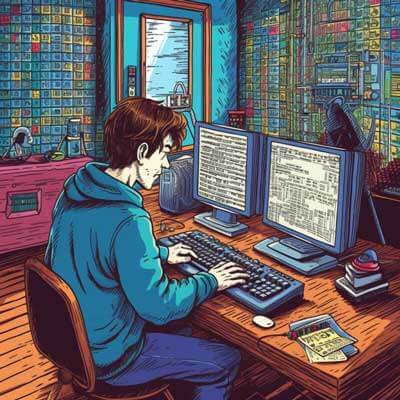
Table of Contents
Introduction to Nullpointerexception
A Nullpointerexception is a common exception that occurs in Java when you try to perform an operation on a null object reference. It is one of the most frequently encountered runtime exceptions and can cause your program to crash if not handled properly.
The Nullpointerexception is thrown when you try to access or invoke a method on an object that is not initialized or has a null value. This can happen when you forget to assign a value to an object or when you mistakenly assume that an object is not null.
To better understand Nullpointerexception, let's look at some of its root causes.
Related Article: How to Change the Date Format in a Java String
The Root Causes of Nullpointerexception
Nullpointerexception can be caused by various factors. Some of the common root causes include:
1. Uninitialized Variables:
If you try to access a variable that has not been initialized, it will have a null value by default, leading to a Nullpointerexception. For example:
String name; System.out.println(name.length()); // Nullpointerexception
2. Null Assignments:
Assigning a null value to an object reference can also result in a Nullpointerexception. For instance:
String name = null; System.out.println(name.length()); // Nullpointerexception
Use Case: Nullpointerexception in Array
Nullpointerexception can also occur when working with arrays. Consider the following code snippet:
int[] numbers = null; System.out.println(numbers[0]); // Nullpointerexception
In this example, we have declared an integer array but have not initialized it. When we try to access the first element of the array, a Nullpointerexception is thrown since the array reference is null.
Use Case: Nullpointerexception in Object References
Nullpointerexception can also be encountered when working with object references. For example:
Person person = null; System.out.println(person.getName()); // Nullpointerexception
Here, we have a Person object reference that is not initialized. When we try to invoke the getName()
method on the null object reference, a Nullpointerexception is thrown.
Related Article: How to Fix the java.lang.reflect.InvocationTargetException
Use Case: Nullpointerexception in Multi-threading
Nullpointerexception can manifest in multi-threading scenarios as well. When multiple threads access shared objects concurrently, there is a possibility of a Nullpointerexception occurring if one thread modifies the object while another thread is using it. For example:
class SharedData { private String data; public void setData(String data) { this.data = data; } public void printData() { System.out.println(data.length()); // Nullpointerexception } } SharedData sharedData = new SharedData(); Thread thread1 = new Thread(() -> { sharedData.setData("Hello"); }); Thread thread2 = new Thread(() -> { sharedData.printData(); }); thread1.start(); thread2.start();
In this example, thread1
sets the data
field to a non-null value, while thread2
tries to access the data
field without proper synchronization. This can result in a Nullpointerexception if thread2
executes before thread1
sets the value.
Best Practice: Null Checks
To avoid Nullpointerexception, it is essential to perform null checks before accessing or invoking methods on object references. By validating that an object reference is not null, you can prevent potential Nullpointerexception scenarios.
Consider the following example:
String name = null; if (name != null) { System.out.println(name.length()); } else { System.out.println("Name is null"); }
In this example, we first check if the name
object reference is null before accessing its length()
method. If the reference is null, we handle the scenario accordingly, preventing a Nullpointerexception.
Best Practice: Optional Class Usage
Java 8 introduced the Optional
class as a best practice for handling nullable object references. It provides a way to explicitly indicate that a value may be absent, allowing you to avoid Nullpointerexception scenarios.
Here's an example of using Optional
:
Optional<String> name = Optional.ofNullable(null); if (name.isPresent()) { System.out.println(name.get().length()); } else { System.out.println("Name is absent"); }
In this example, we create an Optional
object with a potentially null value. We can then check if the value is present using the isPresent()
method and retrieve it using the get()
method. This approach helps prevent Nullpointerexception by explicitly handling the absence of a value.
Best Practice: Utilizing try-catch
Another best practice for handling Nullpointerexception is to utilize try-catch blocks. By catching the exception, you can gracefully handle the scenario and prevent your program from crashing.
Consider the following example:
String name = null; try { System.out.println(name.length()); } catch (NullPointerException e) { System.out.println("Nullpointerexception occurred"); }
In this example, we attempt to access the length()
method of the name
object reference. If a Nullpointerexception occurs, it is caught in the catch block, and we can handle the exception accordingly.
Related Article: How To Convert Array To List In Java
Real World Example: Nullpointerexception in a Web Application
Nullpointerexception can pose challenges in web application development. For example, consider the following scenario:
@WebServlet("/user") public class UserServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) { String username = request.getParameter("username"); User user = getUserByUsername(username); request.setAttribute("user", user); request.getRequestDispatcher("user.jsp").forward(request, response); } }
In this example, the getUserByUsername
method retrieves a User
object based on the provided username. If the username
parameter is null or if no user is found, a Nullpointerexception can occur when forwarding the request to the user.jsp
page.
To prevent this, it is crucial to handle null cases and validate inputs before performing operations that could lead to Nullpointerexception.
Real World Example: Nullpointerexception in a Database Connection
Nullpointerexception can also occur when working with database connections. Consider the following example:
public class DatabaseService { private Connection connection; public void connect() { // Establish database connection } public ResultSet executeQuery(String query) { Statement statement; try { statement = connection.createStatement(); return statement.executeQuery(query); } catch (SQLException e) { e.printStackTrace(); } return null; } }
In this example, the executeQuery
method attempts to create a statement and execute a query on the connection
object. If the connection
object is null or not properly initialized, a Nullpointerexception can occur when executing the query.
To avoid this, it is essential to ensure that the connection
object is properly established before executing any queries.
Performance Consideration: Impact of Nullpointerexception on CPU Usage
Nullpointerexception can have performance implications, particularly in scenarios where it occurs frequently. When a Nullpointerexception is thrown, it adds overhead to the program execution due to the exception handling process.
To mitigate the impact on CPU usage, it is crucial to handle Nullpointerexception scenarios efficiently and avoid unnecessary exception handling in performance-critical sections of your code.
Performance Consideration: Nullpointerexception and Memory Allocation
Nullpointerexception can also impact memory allocation in your program. When a Nullpointerexception occurs, the Java Virtual Machine (JVM) allocates memory for the exception object and associated stack trace, which can contribute to memory consumption.
To minimize the memory impact of Nullpointerexception, it is essential to handle the exception appropriately and prevent unnecessary exception creation in situations where it can be avoided.
Related Article: How To Convert Java Objects To JSON With Jackson
Advanced Technique: Nullpointerexception and Reflection
Reflection is a powerful feature in Java that allows you to inspect and manipulate the structure of classes at runtime. However, it can also introduce Nullpointerexception scenarios if not used carefully.
Consider the following example:
class MyClass { private String name; public void setName(String name) { this.name = name; } } public class ReflectionExample { public static void main(String[] args) throws Exception { Class<?> clazz = Class.forName("MyClass"); Object instance = clazz.getDeclaredConstructor().newInstance(); Method method = clazz.getDeclaredMethod("setName", String.class); method.invoke(instance, null); // Nullpointerexception } }
In this example, we use reflection to dynamically invoke the setName
method on an instance of the MyClass
class. However, if we pass a null argument to the method invocation, a Nullpointerexception will occur.
When using reflection, it is crucial to handle null cases and ensure that the necessary checks are in place to prevent Nullpointerexception scenarios.
Advanced Technique: Nullpointerexception and Streams
Java Streams provide a powerful way to perform functional-style operations on collections and arrays. However, when working with streams, it is essential to be mindful of potential Nullpointerexception scenarios.
Consider the following example:
List<String> names = Arrays.asList("Alice", "Bob", null, "Charlie"); long count = names.stream() .filter(Objects::nonNull) .count(); System.out.println(count); // Nullpointerexception
In this example, we have a list of names that includes a null value. When we try to count the non-null elements using the count()
operation, a Nullpointerexception occurs.
To handle this scenario, it is crucial to filter out null values before performing operations that could lead to Nullpointerexception.
Code Snippet: Nullpointerexception in an Array
int[] numbers = null; System.out.println(numbers[0]); // Nullpointerexception
In this code snippet, we declare an integer array but do not initialize it. When we try to access the first element of the array, a Nullpointerexception is thrown because the array reference is null.
Code Snippet: Nullpointerexception in an Object Reference
Person person = null; System.out.println(person.getName()); // Nullpointerexception
In this code snippet, we have a Person object reference that is not initialized. When we try to invoke the getName()
method on the null object reference, a Nullpointerexception is thrown.
Related Article: How to Implement a Strategy Design Pattern in Java
Code Snippet: Nullpointerexception in a List
List<String> names = null; names.add("Alice"); // Nullpointerexception
In this code snippet, we attempt to add an element to a null list reference. Since the list reference is null and not properly initialized, a Nullpointerexception is thrown when trying to add an element.
Code Snippet: Nullpointerexception in a Map
Map<String, Integer> scores = null; scores.put("Alice", 100); // Nullpointerexception
In this code snippet, we try to put a key-value pair into a null map reference. Since the map reference is null and not properly initialized, a Nullpointerexception is thrown when trying to add the pair.
Code Snippet: Nullpointerexception in a Set
Set<String> names = null; names.contains("Alice"); // Nullpointerexception
In this code snippet, we attempt to check if a null set reference contains a specific element. Since the set reference is null and not properly initialized, a Nullpointerexception is thrown when trying to perform the containment check.
Error Handling: Nullpointerexception in try-catch
String name = null; try { System.out.println(name.length()); } catch (NullPointerException e) { System.out.println("Nullpointerexception occurred"); }
In this example, we attempt to access the length()
method of the name
object reference. If a Nullpointerexception occurs, it is caught in the catch block, and we can handle the exception accordingly.
Related Article: Java Exception Handling Tutorial
Error Handling: Nullpointerexception in a Custom Exception Class
class CustomException extends Exception { public CustomException(String message) { super(message); } } public class ExceptionExample { public static void main(String[] args) { try { throw new CustomException("Nullpointerexception occurred"); } catch (CustomException e) { System.out.println(e.getMessage()); } } }
In this example, we define a custom exception class CustomException
that extends the Exception
class. We then throw an instance of this custom exception when a Nullpointerexception occurs. By catching the custom exception, we can handle the Nullpointerexception scenario in a more specific way.
It is crucial to validate inputs, initialize objects properly, and handle potential null cases to ensure the stability and reliability of your Java applications.