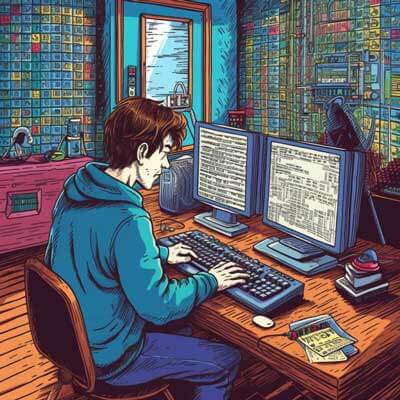
Converting an array to a list in Java is a common operation when working with collections. In this guide, we will explore different methods to achieve this conversion and discuss their advantages and use cases.
Why convert an array to a list?
The need to convert an array to a list often arises when we want to take advantage of the additional functionality provided by the List interface. Arrays in Java have a fixed length and limited methods for manipulation, while lists offer dynamic resizing and a wide range of operations such as adding, removing, and sorting elements.
Converting an array to a list allows us to leverage the flexibility and convenience of the List interface, enabling us to perform various operations on the elements of the array.
Related Article: How To Parse JSON In Java
Method 1: Using the Arrays.asList() method
One straightforward way to convert an array to a list is to use the Arrays.asList()
method provided by the Java API. This method takes the array as an argument and returns a list backed by the original array.
Here’s an example of how to use the Arrays.asList()
method:
String[] array = { "apple", "banana", "orange" }; List list = Arrays.asList(array);
In the above example, we create an array of strings and then pass it to the Arrays.asList()
method. The method returns a list that contains the elements of the array. Note that the list returned by Arrays.asList()
is a fixed-size list, meaning we cannot add or remove elements from it.
Method 2: Using the ArrayList constructor
Another way to convert an array to a list is by using the ArrayList
constructor that accepts a collection as a parameter. This approach allows us to create a mutable list that can be modified after creation.
Here’s an example of how to use the ArrayList
constructor to convert an array to a list:
String[] array = { "apple", "banana", "orange" }; List list = new ArrayList(Arrays.asList(array));
In the above example, we create an array of strings and pass it to the Arrays.asList()
method to obtain a fixed-size list. We then pass this list as a parameter to the ArrayList
constructor, which creates a new ArrayList
instance containing the elements of the original array. This resulting list can be modified by adding or removing elements.
Method 3: Using Java 8 Streams
Starting from Java 8, we can also use streams to convert an array to a list. The Arrays.stream()
method allows us to create a stream from the elements of the array, and then we can collect these elements into a list using the Collectors.toList()
method.
Here’s an example of how to convert an array to a list using Java 8 streams:
String[] array = { "apple", "banana", "orange" }; List list = Arrays.stream(array).collect(Collectors.toList());
In the above example, we use the Arrays.stream()
method to create a stream from the elements of the array. We then use the collect()
method with Collectors.toList()
as the collector to convert the stream into a list.
Related Article: How To Iterate Over Entries In A Java Map
Considerations and best practices
When converting an array to a list, it is important to keep the following considerations in mind:
1. Arrays.asList() creates a fixed-size list: The Arrays.asList()
method returns a fixed-size list, which means that we cannot add or remove elements from it. If we need a mutable list, we should use the ArrayList
constructor or Java 8 streams.
2. Array elements may be modified through the list: When converting an array to a list, keep in mind that modifying an element in the list will also modify the corresponding element in the original array. This is because the list and the array share the same underlying data.
3. Performance implications: Converting an array to a list using the Arrays.asList()
method or the ArrayList
constructor has a time complexity of O(n), where n is the length of the array. If performance is a concern, it is worth considering using Java 8 streams, as it can provide better performance for large arrays.
4. Handling primitive arrays: The methods discussed above work for arrays of objects. If we have a primitive array, such as int[]
or double[]
, we can use the corresponding wrapper class (Integer[]
or Double[]
) to convert it to a list.