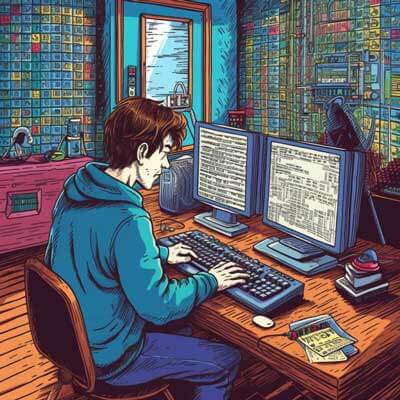
To generate random integers within a specific range in Java, you can use the java.util.Random
class or the ThreadLocalRandom
class. Both classes provide methods to generate random numbers, and you can specify the range by using the nextInt
or nextLong
methods with the desired minimum and maximum values.
Using the java.util.Random class
To generate random integers in a range using the java.util.Random
class, you can follow these steps:
1. Create an instance of the Random
class:
Random rand = new Random();
2. Specify the minimum and maximum values for the range. For example, if you want to generate random numbers between 1 and 100 (inclusive), you can set the minimum value to 1 and the maximum value to 101:
int min = 1; int max = 101;
3. Use the nextInt
method to generate a random integer within the specified range:
int randomNumber = rand.nextInt(max - min) + min;
The nextInt
method returns a random integer between 0 (inclusive) and the specified range (exclusive). By adding the minimum value, you shift the range to start from the minimum value and end at the maximum value.
Here’s the complete code snippet:
import java.util.Random; public class RandomNumberGenerator { public static void main(String[] args) { Random rand = new Random(); int min = 1; int max = 101; int randomNumber = rand.nextInt(max - min) + min; System.out.println(randomNumber); } }
This code will generate and print a random integer between 1 and 100 (inclusive).
Related Article: How To Parse JSON In Java
Using the ThreadLocalRandom class
Alternatively, you can use the ThreadLocalRandom
class, which is a subclass of Random
specifically designed for concurrent use. It provides a more efficient way to generate random numbers in a range.
To generate random integers in a range using the ThreadLocalRandom
class, follow these steps:
1. Import the ThreadLocalRandom
class:
import java.util.concurrent.ThreadLocalRandom;
2. Specify the minimum and maximum values for the range, as before:
int min = 1; int max = 101;
3. Use the nextInt
method of ThreadLocalRandom
to generate a random integer within the specified range:
int randomNumber = ThreadLocalRandom.current().nextInt(min, max);
The nextInt
method of ThreadLocalRandom
takes the minimum and maximum values as parameters and returns a random integer within that range.
Here’s the complete code snippet:
import java.util.concurrent.ThreadLocalRandom; public class RandomNumberGenerator { public static void main(String[] args) { int min = 1; int max = 101; int randomNumber = ThreadLocalRandom.current().nextInt(min, max); System.out.println(randomNumber); } }
This code will generate and print a random integer between 1 and 100 (inclusive).
Best Practices
When generating random integers in a range, keep the following best practices in mind:
– If you need to generate random numbers multiple times, it is recommended to reuse the same Random
or ThreadLocalRandom
instance instead of creating a new one each time. Creating a new instance can lead to less random results due to the seed being based on the current time.
– If you are using the ThreadLocalRandom
class, note that it is designed for use within a single thread. If you need to generate random numbers in a concurrent environment, consider using a different approach, such as creating multiple instances of Random
within each thread.
– Make sure to properly define the range by considering inclusive or exclusive boundaries. For example, if you want to generate random numbers between 1 and 100 (inclusive), you should set the maximum value to 101
to include 100
in the possible outcomes.
– If you need to generate random floating-point numbers, you can use the nextFloat
or nextDouble
methods of the Random
or ThreadLocalRandom
class, respectively. Make sure to adjust the range accordingly.
– Keep in mind that the random numbers generated by these methods are pseudorandom, meaning that they are determined by an algorithm and a seed value. If you require true randomness, consider using external sources, such as hardware random number generators.
Related Article: How To Convert Array To List In Java