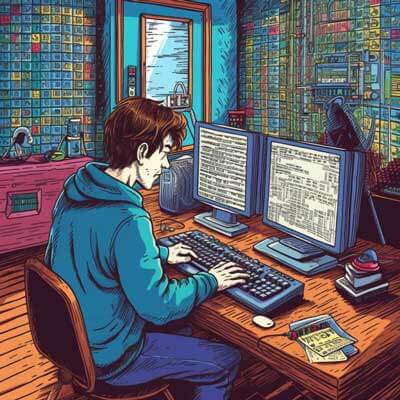
Printing an ArrayList in Java
To print the elements of an ArrayList in Java, you can use various approaches depending on your specific requirements and the version of Java you are using. Here are two possible approaches:
Related Article: How to Convert List to Array in Java
1. Using a for-each loop
One simple way to print the elements of an ArrayList is by using a for-each loop. This approach works well if you just want to iterate over the elements and print them one by one. Here’s an example:
ArrayList arrayList = new ArrayList(); arrayList.add("Apple"); arrayList.add("Banana"); arrayList.add("Orange"); for (String element : arrayList) { System.out.println(element); }
In this example, we create an ArrayList of strings and add three elements to it. Then, we use a for-each loop to iterate over the elements and print each element using the System.out.println()
method.
2. Using the Java 8 Stream API
If you are using Java 8 or a later version, you can use the Stream API to print the elements of an ArrayList. This approach provides more flexibility and allows you to apply various operations to the elements before printing them. Here’s an example:
ArrayList arrayList = new ArrayList(); arrayList.add("Apple"); arrayList.add("Banana"); arrayList.add("Orange"); arrayList.stream().forEach(System.out::println);
In this example, we create an ArrayList of strings, add three elements to it, and then convert the ArrayList to a stream using the stream()
method. We then use the forEach()
method to print each element using a method reference (System.out::println
).
Using the Stream API, you can also apply various operations to the elements before printing them. For example, you can filter the elements based on a condition, sort them, or transform them before printing.
Alternative Ideas and Best Practices
– If you want to print the elements of the ArrayList in a specific format or with additional information, you can modify the print statement accordingly. For example, you can use System.out.printf()
to format the output.
– If you need to print the elements of the ArrayList in reverse order, you can use the Collections.reverse()
method to reverse the ArrayList before printing.
– It is generally considered a good practice to check if the ArrayList is empty before trying to print its elements. You can use the isEmpty()
method to check if the ArrayList is empty and handle the situation accordingly.
– If you are dealing with a large ArrayList or frequently printing its elements, consider using a StringBuilder to efficiently concatenate the elements before printing, instead of using the println()
method for each element individually.
Overall, the choice of approach depends on your specific needs and the complexity of the task at hand. The for-each loop approach is simple and straightforward, while the Stream API approach offers more flexibility and allows for additional operations on the elements.
Related Article: Tutorial: Sorted Data Structure Storage in Java