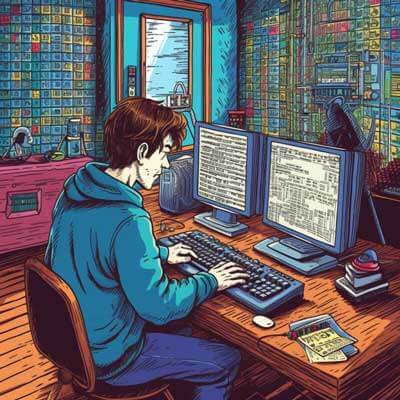
Table of Contents
Printing a Java Array
Printing the contents of a Java array is a simple task that can be accomplished using various techniques. In this answer, we will explore two common approaches to print a Java array.
Related Article: How to Use and Manipulate Arrays in Java
1. Using a loop
One way to print a Java array is by using a loop to iterate over each element and printing it individually. Here's an example:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { System.out.println(numbers[i]); }
In the above code snippet, we declare an array of integers called "numbers" and initialize it with some values. We then use a for loop to iterate over each element of the array and print it using the System.out.println()
method.
This approach allows you to have more control over how the array is printed. For instance, you can format the output by adding separators between the elements or printing them on the same line.
2. Using the Arrays class
Another way to print a Java array is by using the Arrays
class from the java.util
package, which provides a convenient method called toString()
. Here's an example:
import java.util.Arrays; int[] numbers = {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(numbers));
In the above code snippet, we import the Arrays
class and then use the toString()
method to convert the array into a string representation. The resulting string is then printed using the System.out.println()
method.
The advantage of using the Arrays.toString()
method is that it simplifies the code and provides a concise way to print the array. Additionally, it handles null arrays gracefully and prints "null" instead of throwing a NullPointerException.
Best practices
When printing a Java array, it's important to consider the following best practices:
1. Use a loop for more control: If you need to perform additional operations on each element of the array before printing, using a loop gives you the flexibility to do so.
2. Use Arrays.toString()
for simplicity: If you simply want to print the array without any additional processing, using the Arrays.toString()
method is a concise and convenient option.
3. Handle null arrays gracefully: Make sure to handle null arrays properly to avoid NullPointerExceptions. The Arrays.toString()
method handles null arrays gracefully by printing "null".
4. Consider formatting options: If you want to format the output in a specific way, such as adding separators between elements or printing them on the same line, using a loop gives you more control over the formatting.