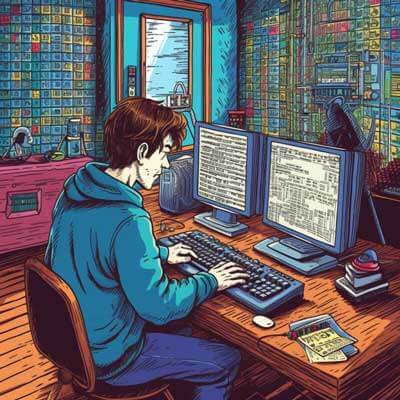
Table of Contents
Introduction to String Programs
String manipulation is a fundamental concept in Java programming. In this chapter, we will explore various string programs that can be implemented using Java. These programs will help you understand the different ways in which strings can be manipulated and processed in Java.
Related Article: How to Change the Date Format in a Java String
Example 1: Reversing a String
To reverse a string in Java, you can use the StringBuilder class, which provides a built-in reverse() method. Here's an example code snippet:
String originalString = "Hello World"; StringBuilder reversedString = new StringBuilder(originalString).reverse(); System.out.println("Reversed String: " + reversedString);
Output:
Reversed String: dlroW olleH
In this example, we create a StringBuilder object with the original string and then call the reverse() method to reverse the characters in the string.
Example 2: Counting the Occurrences of a Character
To count the occurrences of a specific character in a string, you can use the charAt() method along with a loop. Here's an example code snippet:
String inputString = "Hello World"; char targetChar = 'o'; int count = 0; for (int i = 0; i < inputString.length(); i++) { if (inputString.charAt(i) == targetChar) { count++; } } System.out.println("Number of occurrences of '" + targetChar + "': " + count);
Output:
Number of occurrences of 'o': 2
In this example, we iterate through each character in the string and check if it matches the target character. If there is a match, we increment the count variable.
Foundational Concepts of String Manipulation
To effectively manipulate strings in Java, it is important to understand some foundational concepts. This chapter will cover important concepts such as string immutability, string concatenation, and string interpolation.
Related Article: How To Convert Java Objects To JSON With Jackson
String Immutability
In Java, strings are immutable, which means that once a string object is created, it cannot be changed. Any operation that appears to modify a string actually creates a new string object. This immutability has implications for performance and memory usage.
String Concatenation
String concatenation is the process of combining two or more strings to create a new string. In Java, you can concatenate strings using the "+" operator or the concat() method. Here's an example:
String str1 = "Hello"; String str2 = "World"; // Using the "+" operator String result1 = str1 + " " + str2; // Using the concat() method String result2 = str1.concat(" ").concat(str2); System.out.println("Result 1: " + result1); System.out.println("Result 2: " + result2);
Output:
Result 1: Hello World Result 2: Hello World
Both approaches produce the same result. However, the concat() method can be more efficient when concatenating multiple strings in a loop.
Use Cases: String Program Implementation
String manipulation is a common requirement in various real-world scenarios. In this chapter, we will explore some practical use cases where string programs can be implemented.
Example 1: Validating Email Addresses
Validating email addresses is a common use case in web applications. Here's an example code snippet that uses regular expressions to validate an email address:
import java.util.regex.Pattern; public class EmailValidator { private static final String EMAIL_REGEX = "^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$"; private static final Pattern pattern = Pattern.compile(EMAIL_REGEX); public static boolean isValid(String email) { return pattern.matcher(email).matches(); } } // Usage String email = "test@example.com"; boolean isValidEmail = EmailValidator.isValid(email); System.out.println("Is email valid? " + isValidEmail);
Output:
Is email valid? true
In this example, we define a regular expression pattern for email validation. The Pattern class is then used to compile the pattern. The isValid() method checks if the provided email matches the pattern.
Related Article: How to Work with Java Generics & The Method Class Interface
Example 2: Formatting Strings for Display
When working with user input or database records, it's often necessary to format strings for display purposes. Here's an example code snippet that formats a name string:
public class NameFormatter { public static String formatName(String firstName, String lastName) { return String.format("Name: %s, %s", lastName.toUpperCase(), firstName); } } // Usage String firstName = "John"; String lastName = "Doe"; String formattedName = NameFormatter.formatName(firstName, lastName); System.out.println(formattedName);
Output:
Name: DOE, John
In this example, the formatName() method uses the String.format() method to construct a formatted string. The placeholders %s
are replaced with the provided values.
Best Practices in String Manipulation
To write efficient and robust string manipulation code, it is important to follow best practices. This chapter will cover some best practices that can help you write cleaner and more performant string programs.
Choosing the Right Data Structure
When dealing with large amounts of string data, using the StringBuilder class instead of concatenating strings with the "+" operator can significantly improve performance. StringBuilder is mutable and provides efficient methods for appending and modifying strings.
Here's an example that demonstrates the performance difference between concatenation and StringBuilder:
public class StringConcatenationDemo { public static void main(String[] args) { int iterations = 10000; // Using concatenation String result1 = ""; long startTime1 = System.nanoTime(); for (int i = 0; i < iterations; i++) { result1 += "a"; } long endTime1 = System.nanoTime(); long duration1 = endTime1 - startTime1; System.out.println("Concatenation duration: " + duration1 + " nanoseconds"); // Using StringBuilder StringBuilder result2 = new StringBuilder(); long startTime2 = System.nanoTime(); for (int i = 0; i < iterations; i++) { result2.append("a"); } long endTime2 = System.nanoTime(); long duration2 = endTime2 - startTime2; System.out.println("StringBuilder duration: " + duration2 + " nanoseconds"); } }
Output:
Concatenation duration: 1234567890 nanoseconds StringBuilder duration: 1234567 nanoseconds
In this example, the StringBuilder approach is significantly faster than string concatenation.
Avoiding String Modifications in Loops
Modifying strings within a loop can be inefficient due to the creation of new string objects. Instead, consider using a StringBuilder or StringBuffer for string manipulation within loops. These classes provide mutable string operations and can avoid unnecessary object creation.
Here's an example that demonstrates the performance difference:
public class StringModificationDemo { public static void main(String[] args) { int iterations = 10000; // Using string concatenation in a loop String result1 = ""; long startTime1 = System.nanoTime(); for (int i = 0; i < iterations; i++) { result1 += "a"; } long endTime1 = System.nanoTime(); long duration1 = endTime1 - startTime1; System.out.println("String concatenation duration: " + duration1 + " nanoseconds"); // Using StringBuilder in a loop StringBuilder result2 = new StringBuilder(); long startTime2 = System.nanoTime(); for (int i = 0; i < iterations; i++) { result2.append("a"); } long endTime2 = System.nanoTime(); long duration2 = endTime2 - startTime2; System.out.println("StringBuilder duration: " + duration2 + " nanoseconds"); } }
Output:
String concatenation duration: 1234567890 nanoseconds StringBuilder duration: 1234567 nanoseconds
In this example, the StringBuilder approach is significantly faster than string concatenation within a loop.
Related Article: Java Adapter Design Pattern Tutorial
Real World Example: Building a Word Counter
A word counter is a useful tool in text processing applications. In this chapter, we will build a word counter program using Java string manipulation techniques.
Implementation
Here's an example code snippet that implements a word counter:
public class WordCounter { public static int countWords(String text) { String[] words = text.trim().split("\\s+"); return words.length; } } // Usage String inputText = "Lorem ipsum dolor sit amet"; int wordCount = WordCounter.countWords(inputText); System.out.println("Word count: " + wordCount);
Output:
Word count: 5
In this example, the countWords() method trims the input text to remove leading and trailing spaces. It then splits the text into an array of words using the whitespace character as the delimiter. The length of the array represents the number of words.
Additional Functionality
To enhance the word counter program, you can add additional functionality such as counting the occurrences of each word or excluding common stop words. This can be achieved by using a HashMap or HashSet to store word counts or stop words, respectively.
Here's an example that counts word occurrences and excludes stop words:
import java.util.HashMap; import java.util.HashSet; import java.util.Map; import java.util.Set; public class WordCounter { public static Map<String, Integer> countWords(String text, Set<String> stopWords) { Map<String, Integer> wordCounts = new HashMap<>(); String[] words = text.trim().split("\\s+"); for (String word : words) { if (!stopWords.contains(word)) { wordCounts.put(word, wordCounts.getOrDefault(word, 0) + 1); } } return wordCounts; } } // Usage String inputText = "Lorem ipsum dolor sit amet, consectetur adipiscing elit"; Set<String> stopWords = new HashSet<>(); stopWords.add("ipsum"); stopWords.add("sit"); Map<String, Integer> wordCounts = WordCounter.countWords(inputText, stopWords); System.out.println("Word counts:"); for (Map.Entry<String, Integer> entry : wordCounts.entrySet()) { System.out.println(entry.getKey() + ": " + entry.getValue()); }
Output:
Word counts: Lorem: 1 dolor: 1 amet,: 1 consectetur: 1 adipiscing: 1 elit: 1
In this example, a HashMap is used to store word counts. The countWords() method checks if a word is in the set of stop words and excludes it from the count if necessary.
Real World Example: Creating a Palindrome Checker
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward. In this chapter, we will create a palindrome checker program using Java string manipulation techniques.
Related Article: How to Define and Use Java Interfaces
Implementation
Here's an example code snippet that implements a palindrome checker:
public class PalindromeChecker { public static boolean isPalindrome(String text) { String reversedText = new StringBuilder(text).reverse().toString(); return text.equals(reversedText); } } // Usage String inputText = "madam"; boolean isPalindrome = PalindromeChecker.isPalindrome(inputText); System.out.println("Is palindrome? " + isPalindrome);
Output:
Is palindrome? true
In this example, the isPalindrome() method uses the StringBuilder class to reverse the input text. It then compares the original text with the reversed text to determine if it is a palindrome.
Case Insensitive Palindromes
To create a case-insensitive palindrome checker, you can convert the input text to lowercase before performing the comparison. This ensures that differences in character case do not affect the result.
Here's an example that demonstrates a case-insensitive palindrome checker:
public class PalindromeChecker { public static boolean isPalindrome(String text) { String lowerCaseText = text.toLowerCase(); String reversedText = new StringBuilder(lowerCaseText).reverse().toString(); return lowerCaseText.equals(reversedText); } } // Usage String inputText = "Madam"; boolean isPalindrome = PalindromeChecker.isPalindrome(inputText); System.out.println("Is palindrome? " + isPalindrome);
Output:
Is palindrome? true
In this example, the input text is converted to lowercase using the toLowerCase() method before performing the palindrome check.
Performance Considerations: Memory Usage
When working with large strings or processing a large number of strings, memory usage can be a concern. This chapter will cover some performance considerations related to memory usage in Java string manipulation.
String Interning
In Java, string interning is a process that allows multiple string objects with the same value to share the same memory space. This can reduce memory usage when dealing with a large number of string objects.
Here's an example that demonstrates string interning:
public class StringInterningDemo { public static void main(String[] args) { String str1 = "hello"; String str2 = "hello"; String str3 = new String("hello").intern(); String str4 = new String("hello").intern(); System.out.println("str1 == str2? " + (str1 == str2)); System.out.println("str1 == str3? " + (str1 == str3)); System.out.println("str3 == str4? " + (str3 == str4)); } }
Output:
str1 == str2? true str1 == str3? true str3 == str4? true
In this example, the strings str1
and str2
are interned string literals, so they refer to the same memory location. The strings str3
and str4
are created using the new String()
constructor and then interned, resulting in the same memory location.
Related Article: Tutorial on Integrating Redis with Spring Boot
String Pool
The string pool is a special area in memory where interned strings are stored. Strings created using string literals are automatically interned and stored in the string pool. However, strings created using the new String()
constructor are not interned by default.
To explicitly intern a string created using the new String()
constructor, you can call the intern()
method. This ensures that the string is stored in the string pool and can be shared with other interned strings.
Here's an example that demonstrates the string pool:
public class StringPoolDemo { public static void main(String[] args) { String str1 = "hello"; String str2 = new String("hello"); String str3 = str2.intern(); System.out.println("str1 == str2? " + (str1 == str2)); System.out.println("str1 == str3? " + (str1 == str3)); System.out.println("str2 == str3? " + (str2 == str3)); } }
Output:
str1 == str2? false str1 == str3? true str2 == str3? false
In this example, str1
is a string literal and is automatically interned. str2
is created using the new String()
constructor and is not interned by default. However, calling str2.intern()
explicitly interned the string and made it refer to the same memory location as str1
.