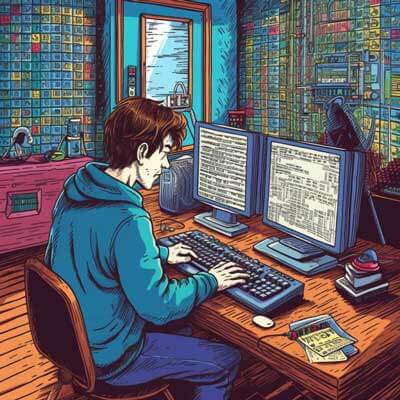
Converting a List to an Array in Java is a common task that you may encounter in your programming journey. In Java, a List is an ordered collection of elements, while an Array is a fixed-size container that holds a sequence of elements of the same type. There are several ways to convert a List to an Array in Java, and in this answer, we will explore two commonly used methods.
Method 1: Using toArray() Method
The first method to convert a List to an Array in Java is by using the toArray()
method provided by the List interface. The toArray()
method returns an array containing all of the elements in the List in the correct order.
Here’s an example of how you can use the toArray()
method to convert a List to an Array:
List list = new ArrayList(); list.add("Apple"); list.add("Banana"); list.add("Orange"); String[] array = list.toArray(new String[0]); System.out.println(Arrays.toString(array));
In the above example, we first create a List of String objects and add three elements to it. Then, we use the toArray()
method to convert the List to an Array. We pass an empty String array as the argument to the toArray()
method. The toArray()
method will create a new String array with the same size as the List and populate it with the elements of the List. Finally, we print the contents of the array using the Arrays.toString()
method.
Related Article: Tutorial: Sorted Data Structure Storage in Java
Method 2: Using Stream API
Another method to convert a List to an Array in Java is by using the Stream API introduced in Java 8. The Stream API provides a useful way to manipulate collections in a functional style. You can use the stream()
method provided by the List interface to obtain a Stream representation of the List, and then convert it to an Array using the toArray()
method provided by the Stream interface.
Here’s an example of how you can use the Stream API to convert a List to an Array:
List list = new ArrayList(); list.add(1); list.add(2); list.add(3); Integer[] array = list.stream().toArray(Integer[]::new); System.out.println(Arrays.toString(array));
In the above example, we first create a List of Integer objects and add three elements to it. Then, we use the stream()
method to obtain a Stream representation of the List. We then use the toArray()
method on the Stream, passing Integer[]::new
as a method reference to create a new Integer array with the same size as the Stream. The toArray()
method will populate the array with the elements of the Stream. Finally, we print the contents of the array using the Arrays.toString()
method.
Best Practices
When converting a List to an Array in Java, it’s important to keep the following best practices in mind:
1. Specify the array type explicitly: When using the toArray()
method, it’s recommended to specify the type of the resulting array explicitly. This allows the compiler to perform type checking and ensures type safety at compile time.
2. Handle incompatible types: If the List contains elements that are not compatible with the type of the array, a ClassCastException
will be thrown at runtime. To avoid this, make sure the List and the array have compatible types.
3. Consider using ArrayList or LinkedList: If you are working with a List implementation that preserves the order of elements, such as ArrayList or LinkedList, the order of the elements in the resulting array will be the same as the order in the List. However, if you are using a Set implementation, the order of the elements in the resulting array may not be the same as the order in the Set.
4. Be mindful of null elements: If the List contains null elements, they will be preserved in the resulting array. Make sure to handle null elements appropriately in your code.
5. Use the appropriate method: Choose the method that best suits your requirements. If you need a flexible solution that works with any List implementation, the toArray()
method is a good choice. If you prefer a more concise and functional approach, the Stream API method may be more suitable.
Related Article: Tutorial: Enumeration Types and Data Structures in Java