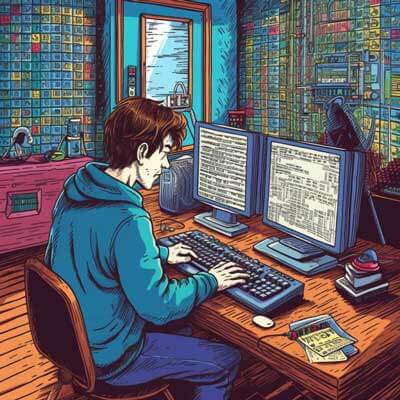
- Introduction to String, StringBuffer and StringBuilder
- Defining String, StringBuffer and StringBuilder
- Use Cases: When to Use String
- Use Cases: When to Use StringBuffer
- Use Cases: When to Use StringBuilder
- Comparing Performance: String vs StringBuffer
- Comparing Performance: StringBuffer vs StringBuilder
- Best Practices: Efficient Use of String
- Best Practices: Efficient Use of StringBuffer
- Best Practices: Efficient Use of StringBuilder
- Real World Examples: String Usage
- Real World Examples: StringBuffer Usage
- Real World Examples: StringBuilder Usage
- Advanced Techniques: Optimizing String Operations
- Advanced Techniques: Optimizing StringBuffer Operations
- Advanced Techniques: Optimizing StringBuilder Operations
- Code Snippet: String Initialization and Manipulation
- Code Snippet: StringBuffer Initialization and Manipulation
- Code Snippet: StringBuilder Initialization and Manipulation
- Code Snippet: String to StringBuffer Conversion
- Code Snippet: String to StringBuilder Conversion
- Error Handling in String Operations
- Error Handling in StringBuffer Operations
- Error Handling in StringBuilder Operations
Introduction to String, StringBuffer and StringBuilder
Strings are a fundamental data type in Java that represent sequences of characters. In Java, there are three classes available for manipulating strings: String, StringBuffer, and StringBuilder.
The String class is immutable, meaning its value cannot be changed once it is created. This immutability ensures that once a String object is created, its value remains the same throughout its lifetime. This can be advantageous in situations where the value of a string should not be modified.
On the other hand, StringBuffer and StringBuilder are mutable classes that allow modifying the value of a string. They provide methods for appending, inserting, deleting, and modifying characters in a string.
Related Article: How to Use the Xmx Option in Java
Defining String, StringBuffer and StringBuilder
– String: The String class represents an immutable sequence of characters. Strings are created using double quotes, like “Hello, World!”.
Example of String initialization:
String str = "Hello, World!";
– StringBuffer: The StringBuffer class is a mutable sequence of characters. It provides methods for modifying the value of a string without creating a new object. StringBuffer is thread-safe, meaning it can be safely used in multi-threaded environments.
Example of StringBuffer initialization:
StringBuffer buffer = new StringBuffer("Hello"); buffer.append(", World!");
– StringBuilder: The StringBuilder class is similar to StringBuffer in that it is a mutable sequence of characters. However, StringBuilder is not thread-safe, which makes it more efficient in single-threaded environments.
Example of StringBuilder initialization:
StringBuilder builder = new StringBuilder("Hello"); builder.append(", World!");
Use Cases: When to Use String
The String class is suitable for use cases where the value of a string is not intended to be modified. Some common use cases for String include:
1. Storing and manipulating constant values or literals.
2. Comparing string values using the equals()
method.
3. Concatenating strings using the +
operator or the concat()
method.
Example 1: Storing constant values or literals
String appName = "My Application";
Example 2: Comparing strings
String password = "password123"; if (password.equals("password123")) { System.out.println("Access granted"); } else { System.out.println("Access denied"); }
Use Cases: When to Use StringBuffer
The StringBuffer class is ideal for scenarios where the value of a string needs to be modified frequently, such as in a loop or when building large strings. Use StringBuffer when:
1. Modifying strings frequently using methods like append()
, insert()
, or delete()
.
2. Building long strings dynamically.
3. Performing string manipulations in multi-threaded environments.
Example 1: Modifying strings frequently
StringBuffer buffer = new StringBuffer("Hello"); for (int i = 0; i < 1000; i++) { buffer.append(" World"); } String result = buffer.toString();
Example 2: Building long strings dynamically
StringBuffer query = new StringBuffer("SELECT * FROM users WHERE"); query.append(" age > 18"); query.append(" AND gender = 'female'");
Related Article: Can Two Java Threads Access the Same MySQL Session?
Use Cases: When to Use StringBuilder
The StringBuilder class is similar to StringBuffer but provides better performance in single-threaded environments where thread-safety is not a concern. Use StringBuilder when:
1. Modifying strings frequently in a single-threaded environment.
2. Building long strings dynamically without the need for thread-safety.
Example 1: Modifying strings frequently
StringBuilder builder = new StringBuilder("Hello"); for (int i = 0; i < 1000; i++) { builder.append(" World"); } String result = builder.toString();
Example 2: Building long strings dynamically
StringBuilder query = new StringBuilder("SELECT * FROM users WHERE"); query.append(" age > 18"); query.append(" AND gender = 'female'");
Comparing Performance: String vs StringBuffer
The performance of String and StringBuffer can differ significantly in scenarios involving frequent string modifications.
When using the String class for concatenation or modification operations, a new String object is created each time, resulting in unnecessary memory allocation and garbage collection. This can lead to performance degradation, especially when dealing with large strings or in a loop.
On the other hand, StringBuffer provides a more efficient way to modify strings since it modifies the existing object without creating new ones. StringBuffer achieves this by internally using a resizable character array to store the string’s value.
To compare the performance of String and StringBuffer, consider the following example:
Example: Concatenating strings using String and StringBuffer
String str = ""; for (int i = 0; i < 1000; i++) { str += "a"; }
The above code snippet concatenates the string “a” to the variable str
in a loop. In this case, using String leads to poor performance due to the creation of new String objects in each iteration. To improve performance, we can use StringBuffer as follows:
Example: Concatenating strings using StringBuffer
StringBuffer buffer = new StringBuffer(); for (int i = 0; i < 1000; i++) { buffer.append("a"); } String result = buffer.toString();
By using StringBuffer, the performance of string concatenation is significantly improved, as the existing object is modified instead of creating new ones.
Comparing Performance: StringBuffer vs StringBuilder
The performance difference between StringBuffer and StringBuilder is minimal. Both classes provide similar functionality for modifying strings, but StringBuilder is faster because it is not thread-safe.
In a single-threaded environment, where thread-safety is not a concern, using StringBuilder is recommended for better performance. However, in a multi-threaded environment, where multiple threads may access or modify the same StringBuffer object, it is safer to use StringBuffer to ensure thread-safety.
Example: Appending strings using StringBuffer and StringBuilder
StringBuilder builder = new StringBuilder(); for (int i = 0; i < 1000; i++) { builder.append("a"); } String result1 = builder.toString(); StringBuffer buffer = new StringBuffer(); for (int i = 0; i < 1000; i++) { buffer.append("a"); } String result2 = buffer.toString();
In the above example, both StringBuilder and StringBuffer are used to append the string “a” in a loop. The performance difference between the two is negligible, and the choice between them depends on the specific requirements of the application.
Related Article: How to Implement Recursion in Java
Best Practices: Efficient Use of String
To use the String class efficiently, consider the following best practices:
1. Avoid unnecessary string concatenation using the +
operator in a loop. Instead, use StringBuffer or StringBuilder.
2. Use the equals()
method to compare string values, rather than using the ==
operator.
3. When performing multiple string manipulations, consider converting the string to a mutable form (StringBuffer or StringBuilder) and then converting it back to a String after the manipulations are complete.
Example: Efficient use of String
String str = "Hello"; str += " World";
In the above example, the string concatenation using the +=
operator can be replaced with a StringBuilder for better performance:
StringBuilder builder = new StringBuilder("Hello"); builder.append(" World"); String result = builder.toString();
Best Practices: Efficient Use of StringBuffer
To make efficient use of StringBuffer, keep the following best practices in mind:
1. Pre-allocate the initial capacity of the StringBuffer to avoid frequent resizing.
2. Use the append()
method to concatenate strings instead of using the +
operator.
3. Use the insert()
method to insert characters or strings at specific positions.
Example: Efficient use of StringBuffer
StringBuffer buffer = new StringBuffer(100); buffer.append("Hello"); buffer.append(" World");
In the above example, by pre-allocating the initial capacity of the StringBuffer, we avoid unnecessary resizing operations.
Best Practices: Efficient Use of StringBuilder
When working with StringBuilder, consider the following best practices for efficient usage:
1. Pre-allocate the initial capacity of the StringBuilder to avoid resizing.
2. Use the append()
method to concatenate strings instead of using the +
operator.
3. Use the insert()
method to insert characters or strings at specific positions.
Example: Efficient use of StringBuilder
StringBuilder builder = new StringBuilder(100); builder.append("Hello"); builder.append(" World");
By pre-allocating the initial capacity of the StringBuilder, we reduce the number of resizing operations, resulting in improved performance.
Related Article: Java Adapter Design Pattern Tutorial
Real World Examples: String Usage
Strings are widely used in various real-world scenarios. Here are a couple of examples:
Example 1: Parsing user input
String userInput = getUserInput(); if (userInput.equals("quit")) { System.exit(0); } else { processInput(userInput); }
In this example, the String class is used to compare user input and perform an action based on the input value.
Example 2: Formatting output
String name = "John"; int age = 30; String formattedOutput = "Name: " + name + ", Age: " + age; System.out.println(formattedOutput);
In this example, the String class is used to concatenate multiple strings and create a formatted output.
Real World Examples: StringBuffer Usage
StringBuffer is commonly used in scenarios where frequent string modifications are required. Here are a couple of real-world examples:
Example 1: Building SQL queries dynamically
StringBuffer query = new StringBuffer("SELECT * FROM users WHERE"); if (filterByAge) { query.append(" age > 18"); } if (filterByGender) { query.append(" AND gender = 'female'"); }
In this example, the StringBuffer class is used to dynamically build an SQL query based on optional filter conditions.
Example 2: Generating log messages
StringBuffer logMessage = new StringBuffer("Error occurred at "); logMessage.append(new Date()); logMessage.append(": "); logMessage.append(errorMessage);
In this example, the StringBuffer class is used to create log messages by appending timestamp and error messages.
Real World Examples: StringBuilder Usage
StringBuilder is commonly used in scenarios where frequent string modifications are required, and thread-safety is not a concern. Here are a couple of real-world examples:
Example 1: Building JSON strings
StringBuilder json = new StringBuilder(); json.append("{"); json.append("\"name\": \"John\","); json.append("\"age\": 30"); json.append("}");
In this example, the StringBuilder class is used to construct a JSON string by appending different key-value pairs.
Example 2: Generating HTML content
StringBuilder html = new StringBuilder(); html.append("<html>"); html.append("<head><title>My Webpage</title></head>"); html.append("<body>"); html.append("<h1>Welcome to my webpage!</h1>"); html.append("</body>"); html.append("</html>");
In this example, the StringBuilder class is used to build an HTML page dynamically by appending various HTML tags.
Related Article: How to Print a Hashmap in Java
Advanced Techniques: Optimizing String Operations
To optimize string operations, consider the following techniques:
1. Use the substring()
method instead of creating new String objects.
2. Utilize the replace()
or replaceAll()
methods for efficient string replacements.
3. Use the toLowerCase()
or toUpperCase()
methods to convert the case of strings.
Example: Optimizing string operations
String str = "Hello, World!"; String substring = str.substring(7); String replaced = str.replace("Hello", "Hi"); String lowercase = str.toLowerCase();
In the above example, the substring()
, replace()
, and toLowerCase()
methods are used to optimize string operations.
Advanced Techniques: Optimizing StringBuffer Operations
To optimize StringBuffer operations, follow these techniques:
1. Set the initial capacity of the StringBuffer appropriately to avoid unnecessary resizing.
2. Use the setCharAt()
method to modify individual characters at specific positions.
3. Utilize the reverse()
method to reverse the characters in the StringBuffer.
Example: Optimizing StringBuffer operations
StringBuffer buffer = new StringBuffer(100); buffer.append("Hello"); buffer.setCharAt(1, 'a'); buffer.reverse();
In the above example, the initial capacity is set for the StringBuffer, the setCharAt()
method is used to modify a specific character, and the reverse()
method is used to reverse the characters.
Advanced Techniques: Optimizing StringBuilder Operations
To optimize StringBuilder operations, consider the following techniques:
1. Set the initial capacity of the StringBuilder appropriately to avoid unnecessary resizing.
2. Use the setCharAt()
method to modify individual characters at specific positions.
3. Utilize the reverse()
method to reverse the characters in the StringBuilder.
Example: Optimizing StringBuilder operations
StringBuilder builder = new StringBuilder(100); builder.append("Hello"); builder.setCharAt(1, 'a'); builder.reverse();
In the above example, the initial capacity is set for the StringBuilder, the setCharAt()
method is used to modify a specific character, and the reverse()
method is used to reverse the characters.
Related Article: How to Manage Collections Without a SortedList in Java
Code Snippet: String Initialization and Manipulation
String str = "Hello, World!"; String substring = str.substring(7); String replaced = str.replace("Hello", "Hi"); String lowercase = str.toLowerCase();
In this code snippet, a String is initialized with the value “Hello, World!”. The substring()
, replace()
, and toLowerCase()
methods are then used to manipulate the string.
Code Snippet: StringBuffer Initialization and Manipulation
StringBuffer buffer = new StringBuffer(100); buffer.append("Hello"); buffer.setCharAt(1, 'a'); buffer.reverse();
In this code snippet, a StringBuffer is initialized with an initial capacity of 100. The append()
, setCharAt()
, and reverse()
methods are then used to manipulate the StringBuffer.
Code Snippet: StringBuilder Initialization and Manipulation
StringBuilder builder = new StringBuilder(100); builder.append("Hello"); builder.setCharAt(1, 'a'); builder.reverse();
In this code snippet, a StringBuilder is initialized with an initial capacity of 100. The append()
, setCharAt()
, and reverse()
methods are then used to manipulate the StringBuilder.
Related Article: How to Fix the Java NullPointerException
Code Snippet: String to StringBuffer Conversion
String str = "Hello, World!"; StringBuffer buffer = new StringBuffer(str); buffer.append(" Welcome"); String result = buffer.toString();
In this code snippet, a String is converted to a StringBuffer using its constructor. The StringBuffer is then modified by appending ” Welcome”, and the final result is converted back to a String.
Code Snippet: String to StringBuilder Conversion
String str = "Hello, World!"; StringBuilder builder = new StringBuilder(str); builder.append(" Welcome"); String result = builder.toString();
In this code snippet, a String is converted to a StringBuilder using its constructor. The StringBuilder is then modified by appending ” Welcome”, and the final result is converted back to a String.
Error Handling in String Operations
String operations in Java may encounter various errors, such as null values or index out of bounds exceptions. To handle these errors effectively, consider the following best practices:
1. Always check for null values before performing string operations.
2. Use try-catch blocks to handle exceptions that may occur during string operations.
Example: Error handling in string operations
String str = null; try { String substring = str.substring(7); } catch (NullPointerException e) { System.out.println("Error: String is null"); } catch (IndexOutOfBoundsException e) { System.out.println("Error: Index out of bounds"); }
In this example, a try-catch block is used to handle potential NullPointerException and IndexOutOfBoundsException that may occur during string operations.
Related Article: How to Convert a String to an Array in Java
Error Handling in StringBuffer Operations
StringBuffer operations can also encounter errors, such as null values or index out of bounds exceptions. To handle these errors, follow these best practices:
1. Always check for null values before performing StringBuffer operations.
2. Use try-catch blocks to handle exceptions that may occur during StringBuffer operations.
Example: Error handling in StringBuffer operations
StringBuffer buffer = null; try { buffer.append("Hello"); } catch (NullPointerException e) { System.out.println("Error: StringBuffer is null"); } catch (IndexOutOfBoundsException e) { System.out.println("Error: Index out of bounds"); }
In this example, a try-catch block is used to handle potential NullPointerException and IndexOutOfBoundsException that may occur during StringBuffer operations.
Error Handling in StringBuilder Operations
StringBuilder operations can also encounter errors, such as null values or index out of bounds exceptions. To handle these errors, consider the following best practices:
1. Always check for null values before performing StringBuilder operations.
2. Use try-catch blocks to handle exceptions that may occur during StringBuilder operations.
Example: Error handling in StringBuilder operations
StringBuilder builder = null; try { builder.append("Hello"); } catch (NullPointerException e) { System.out.println("Error: StringBuilder is null"); } catch (IndexOutOfBoundsException e) { System.out.println("Error: Index out of bounds"); }
In this example, a try-catch block is used to handle potential NullPointerException and IndexOutOfBoundsException that may occur during StringBuilder operations.