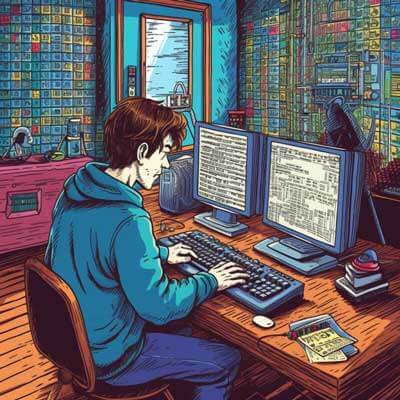
Table of Contents
Converting a string to an integer in Java is a common task that you may encounter in your programming journey. There are various scenarios where you may need to convert a string to an integer, such as reading user input, parsing data from a file, or performing mathematical operations. In this answer, we will explore different approaches to convert a string to an int in Java.
Approach 1: Using Integer.parseInt()
One of the simplest and most widely used methods to convert a string to an int in Java is by using the Integer.parseInt()
method. This method takes a string as input and returns the corresponding int value. Here's an example:
String str = "123";int num = Integer.parseInt(str);System.out.println(num); // Output: 123
In this example, we have a string str
containing the value "123". By calling Integer.parseInt(str)
, we convert the string to an int and store it in the variable num
. Finally, we print the value of num
, which is 123.
It's important to note that the Integer.parseInt()
method throws a NumberFormatException
if the string cannot be parsed as an int. For example, if you try to convert the string "abc" to an int, it will throw an exception. To handle this, you can wrap the conversion code in a try-catch block as shown below:
String str = "abc";try { int num = Integer.parseInt(str); System.out.println(num);} catch (NumberFormatException e) { System.out.println("Invalid input");}
In this modified example, if the string "abc" cannot be parsed as an int, the NumberFormatException
will be caught and the message "Invalid input" will be printed.
Related Article: Java Do-While Loop Tutorial
Approach 2: Using Integer.valueOf()
Another approach to convert a string to an int in Java is by using the Integer.valueOf()
method. This method is similar to Integer.parseInt()
, but instead of returning a primitive int, it returns an Integer
object. Here's an example:
String str = "456";Integer num = Integer.valueOf(str);System.out.println(num); // Output: 456
In this example, we convert the string "456" to an Integer
object and store it in the variable num
. The Integer
class is a wrapper class for the primitive int
type and provides additional methods and functionality.
Like Integer.parseInt()
, Integer.valueOf()
also throws a NumberFormatException
if the string cannot be parsed as an int. You can handle this exception in the same way as shown in the previous example.
Why is the question asked?
The question "How to convert string to int in Java?" is commonly asked by programmers who need to perform operations that involve converting user input or data from external sources into integer values. Understanding how to convert strings to ints is fundamental for handling and manipulating numerical data in Java programs.
Potential reasons for asking the question
There are several potential reasons why someone may ask this question:
1. Input Validation: When accepting user input, it's important to validate and convert the input into the appropriate data type. By converting a string to an int, you can ensure that the user has entered a valid numerical value.
2. Data Processing: In many cases, data is stored in files or databases as strings. To perform mathematical operations or comparisons on this data, it needs to be converted to numerical types such as int.
3. Error Handling: When dealing with external data sources or APIs, there is a possibility of receiving unexpected or invalid data. Converting strings to ints allows you to handle such scenarios gracefully and provide appropriate error messages.
Related Article: How to Convert JsonString to JsonObject in Java
Suggestions and alternative ideas
While the approaches discussed above are the standard and recommended ways to convert a string to an int in Java, there are a few alternative methods and suggestions worth mentioning:
1. Using Integer.parseInt()
with Radix: The Integer.parseInt()
method also allows you to specify the radix or base of the number you are converting. By passing a second parameter to the method, you can convert strings that represent numbers in different bases, such as binary, octal, or hexadecimal. For example:
String binaryStr = "1010";int binaryNum = Integer.parseInt(binaryStr, 2); // Convert binary to intSystem.out.println(binaryNum); // Output: 10
2. Handling NumberFormatException: As mentioned earlier, both Integer.parseInt()
and Integer.valueOf()
methods throw a NumberFormatException
if the string cannot be parsed as an int. When handling this exception, consider providing a meaningful error message to the user or logging the error for debugging purposes.
Best practices
When converting a string to an int in Java, it's important to keep the following best practices in mind:
1. Input Validation: Always validate user input or data from external sources before attempting to convert it to an int. Use appropriate error handling mechanisms to handle invalid input gracefully.
2. Exception Handling: Handle the NumberFormatException
that may be thrown when converting a string to an int. This will prevent your program from crashing and allow you to handle invalid input appropriately.
3. Radix Conversion: If you need to convert strings representing numbers in different bases, use the Integer.parseInt()
method with the radix parameter to ensure correct conversion.
4. Use Wrapper Classes: Consider using the Integer.valueOf()
method if you need to work with Integer
objects instead of primitive int
values. This allows you to leverage the additional methods and functionality provided by the Integer
class.
5. Code Readability: Choose meaningful variable names that accurately represent the purpose of the string and int variables. This will make your code more readable and easier to understand for other developers.