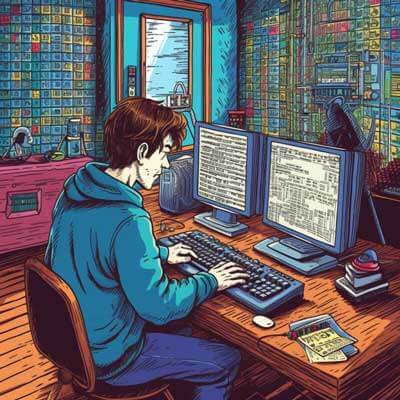
Table of Contents
Introduction to Exception Handling
Exception handling is a fundamental concept in Java programming that allows developers to handle and manage errors or exceptional situations that may occur during the execution of a program. An exception is an object that represents an error or an exceptional condition.
In Java, exceptions are thrown when an abnormal condition occurs, such as an arithmetic error, a null reference, or an input/output failure. By using exception handling, developers can gracefully handle these exceptional situations and prevent their programs from crashing.
Let's take a look at an example that demonstrates the basic structure of exception handling in Java:
try { // Code that may throw an exception} catch (ExceptionType1 e1) { // Exception handling code for ExceptionType1} catch (ExceptionType2 e2) { // Exception handling code for ExceptionType2} finally { // Code that will always be executed, regardless of whether an exception occurs or not}
In the above code snippet, the code that might throw an exception is enclosed within a try block. If an exception occurs within the try block, it is caught by one of the catch blocks based on the type of the exception. The finally block is optional and is used to specify code that will be executed regardless of whether an exception occurs or not.
Related Article: Java Design Patterns Tutorial
Example 1: Handling ArithmeticException
try { int result = 10 / 0; // ArithmeticException: division by zero} catch (ArithmeticException e) { System.out.println("An arithmetic error occurred: " + e.getMessage());}
In this example, we attempt to divide the number 10 by zero, which results in an ArithmeticException. The exception is caught by the catch block, and the error message is printed to the console.
Example 2: Handling NullPointerException
try { String text = null; int length = text.length(); // NullPointerException: text is null} catch (NullPointerException e) { System.out.println("A null pointer error occurred: " + e.getMessage());}
In this example, we are trying to call the length()
method on a null object text
, which throws a NullPointerException. The catch block catches the exception and displays an appropriate error message.
Exception handling allows developers to gracefully recover from errors and take appropriate actions. It is an essential aspect of writing robust and reliable Java programs.
The Hierarchy of Exceptions
In Java, exceptions are organized into a hierarchical structure, with the base class being java.lang.Throwable
. This hierarchy allows for more specific exception classes to be caught and handled separately from more general exceptions.
The Throwable
class has two direct subclasses: Error
and Exception
. Errors are exceptional conditions that usually cannot be recovered from and indicate serious problems in the JVM or the underlying system. Exceptions, on the other hand, represent exceptional conditions that can be caught and handled by the program.
Related Article: How to Easily Print a Java Array
Checked and Unchecked Exceptions
Exceptions in Java are further classified as either checked or unchecked. Checked exceptions are exceptions that must be declared in the method signature using the throws
keyword, or caught and handled within the method. Examples of checked exceptions include IOException
and SQLException
.
Unchecked exceptions, also known as runtime exceptions, do not need to be declared or caught explicitly. They are subclasses of RuntimeException
and typically represent programming errors or unexpected conditions. Examples of unchecked exceptions include NullPointerException
and ArrayIndexOutOfBoundsException
.
Example 1: Handling a Checked Exception
import java.io.FileInputStream;import java.io.FileNotFoundException;public class FileReadExample { public static void main(String[] args) { try { FileInputStream file = new FileInputStream("file.txt"); // FileNotFoundException } catch (FileNotFoundException e) { System.out.println("The file was not found: " + e.getMessage()); } }}
In this example, we attempt to open a file that does not exist, resulting in a FileNotFoundException
. Since FileNotFoundException
is a checked exception, we need to either declare it in the method signature or catch and handle it within the method.
Example 2: Handling an Unchecked Exception
public class DivisionExample { public static void main(String[] args) { int result = divide(10, 0); // ArithmeticException: division by zero System.out.println("Result: " + result); } public static int divide(int dividend, int divisor) { return dividend / divisor; }}
In this example, we define a method divide()
that performs division. If the divisor is zero, an ArithmeticException
is thrown. Since ArithmeticException
is an unchecked exception, we are not required to declare or catch it explicitly. However, if we don't handle the exception, the program will terminate abruptly.
Understanding the hierarchy of exceptions and the distinction between checked and unchecked exceptions is crucial for effective exception handling in Java. By properly organizing and handling exceptions, developers can write more robust and maintainable code.