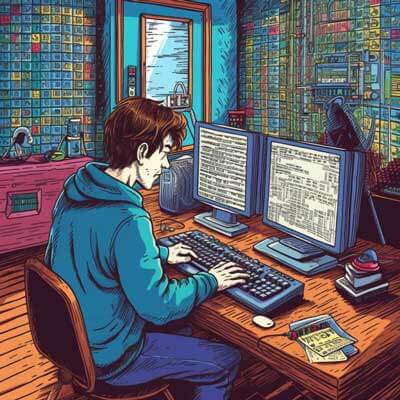
Parsing JSON in Java is a common task when working with web services or data exchange formats. JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. In Java, there are several libraries available for parsing JSON, including the built-in JSON APIs provided by Java SE, as well as third-party libraries like Jackson, Gson, and JSON.simple. In this guide, we will explore different approaches to parse JSON in Java and discuss their pros and cons.
1. Using the built-in JSON APIs in Java SE
Java SE provides built-in APIs for parsing and generating JSON. These APIs are part of the javax.json package and were introduced in Java SE 7. Here is an example of how to parse JSON using these APIs:
import javax.json.Json; import javax.json.JsonObject; import javax.json.JsonReader; import javax.json.JsonStructure; import javax.json.JsonValue; import java.io.StringReader; public class JsonParserExample { public static void main(String[] args) { String jsonString = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}"; try (JsonReader reader = Json.createReader(new StringReader(jsonString))) { JsonStructure jsonStructure = reader.read(); if (jsonStructure.getValueType() == JsonValue.ValueType.OBJECT) { JsonObject jsonObject = (JsonObject) jsonStructure; String name = jsonObject.getString("name"); int age = jsonObject.getInt("age"); String city = jsonObject.getString("city"); System.out.println("Name: " + name); System.out.println("Age: " + age); System.out.println("City: " + city); } } } }
In this example, we create a JsonReader
using a StringReader
and pass the JSON string to the reader
‘s read()
method. The resulting JsonStructure
represents the JSON object. We can then extract values from the JSON object using the appropriate getter methods.
Pros of using the built-in JSON APIs:
– No external dependencies, as these APIs are part of Java SE.
– Simple and lightweight.
Cons of using the built-in JSON APIs:
– The API is relatively low-level and requires manual handling of JSON structures.
– Limited functionality compared to third-party libraries.
Related Article: How To Convert Array To List In Java
2. Using Jackson
Jackson is a popular JSON library for Java that provides a high-performance JSON processor. It offers a powerful and flexible API for parsing, generating, and manipulating JSON data. To parse JSON using Jackson, you need to add the Jackson dependencies to your project’s build configuration. Here is an example of how to parse JSON using Jackson:
import com.fasterxml.jackson.databind.JsonNode; import com.fasterxml.jackson.databind.ObjectMapper; public class JsonParserExample { public static void main(String[] args) throws Exception { String jsonString = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}"; ObjectMapper objectMapper = new ObjectMapper(); JsonNode jsonNode = objectMapper.readTree(jsonString); String name = jsonNode.get("name").asText(); int age = jsonNode.get("age").asInt(); String city = jsonNode.get("city").asText(); System.out.println("Name: " + name); System.out.println("Age: " + age); System.out.println("City: " + city); } }
In this example, we create an ObjectMapper
instance and use its readTree()
method to parse the JSON string into a JsonNode
object. We can then extract values from the JsonNode
using the appropriate getter methods.
Pros of using Jackson:
– High-performance and efficient JSON processing.
– Rich feature set and flexibility.
– Wide community support and active development.
Cons of using Jackson:
– Requires external dependencies to be added to the project.
– May have a steeper learning curve compared to the built-in JSON APIs.
Why is JSON parsing in Java important?
The question of how to parse JSON in Java arises because JSON has become a widely used data interchange format in web services and APIs. Many web services and APIs return data in JSON format, and Java developers often need to extract and manipulate this data in their applications. Parsing JSON allows Java developers to convert JSON data into Java objects or extract specific values from the JSON structure.
Some potential reasons for asking this question include:
– Needing to consume JSON data from an external API.
– Needing to process and manipulate JSON data within a Java application.
– Wanting to convert JSON data into Java objects for further processing.
Alternative approaches and suggestions
While the examples provided above cover the most common approaches to parse JSON in Java, there are alternative libraries and techniques available. Some popular alternatives to consider are Gson and JSON.simple.
– Gson: Gson is a Java library provided by Google for converting Java Objects into their JSON representation and vice versa. It provides a simple and flexible API for parsing and generating JSON. To parse JSON using Gson, you need to add the Gson dependency to your project’s build configuration and use the Gson API to deserialize the JSON data into Java objects.
– JSON.simple: JSON.simple is a lightweight Java library for parsing and generating JSON data. It provides a simple and intuitive API for parsing and generating JSON. To parse JSON using JSON.simple, you need to add the JSON.simple dependency to your project’s build configuration and use the JSON.simple API to parse the JSON data.
When choosing a JSON parsing library for your Java project, consider factors such as performance, ease of use, community support, and compatibility with your project’s requirements.
Related Article: How To Iterate Over Entries In A Java Map
Best practices
When parsing JSON in Java, it is important to follow some best practices to ensure efficient and reliable code:
– Handle exceptions: JSON parsing can throw exceptions, such as JsonParsingException
. Make sure to handle these exceptions properly to prevent your application from crashing or producing unexpected results.
– Use appropriate data structures: Choose the appropriate data structures to represent the JSON data in your Java code. For simple JSON structures, using maps or Java objects can be sufficient. For complex JSON structures, consider using custom Java classes or libraries like Jackson or Gson to deserialize the JSON into strongly-typed objects.
– Validate JSON: Before parsing JSON, it is a good practice to validate the JSON data to ensure its correctness. You can use JSON schema validation libraries or validate the JSON against a predefined schema to ensure that it meets the expected structure and data types.
– Be mindful of performance: JSON parsing can be a resource-intensive operation, especially for large JSON documents. Consider using streaming APIs provided by libraries like Jackson to parse JSON incrementally and avoid loading the entire JSON into memory at once.
– Handle null values: JSON data can contain null values. Make sure to handle null values appropriately in your Java code to prevent null pointer exceptions.